Top 5 Ways to Secure Your Groovy Objects from Bugs
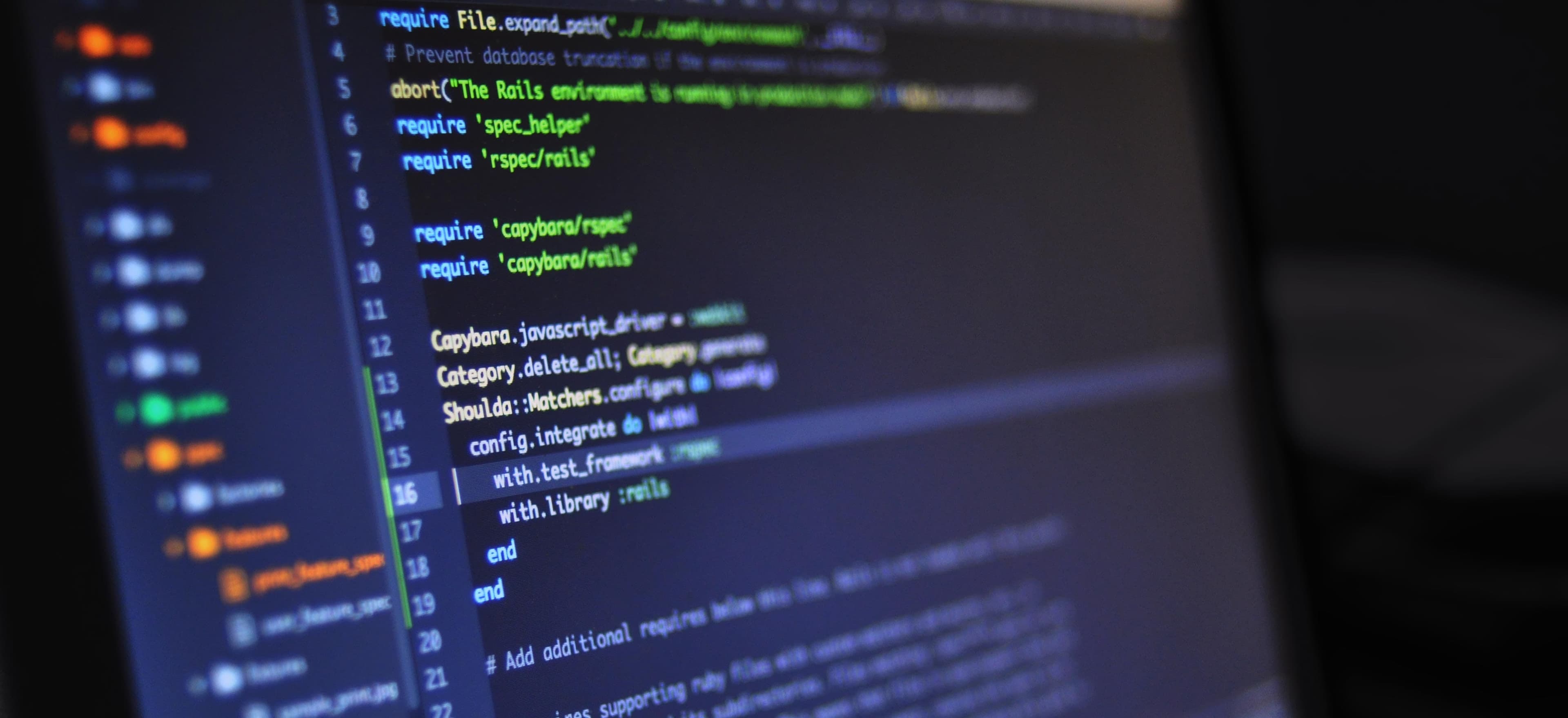
- Published on
Top 5 Ways to Secure Your Groovy Objects from Bugs
Groovy, a powerful dynamic language for the Java platform, offers developers a concise and expressive syntax. However, like any programming environment, it is not immune to bugs and vulnerabilities. In this article, we'll delve into the top five methods to secure your Groovy objects from common pitfalls and effectively minimize bugs in your applications.
1. Leverage Type Checking
One of Groovy's most significant winning points is its dynamic nature. However, this flexibility can also lead to runtime errors. Utilizing type checking allows you to catch these errors early, enhancing the robustness of your applications.
Why Type Checking?
Type checking helps enforce constraints at compile time, enabling you to identify type mismatches before the code is executed.
Code Example
class Person {
String name
int age
}
def person = new Person(name: 'John Doe', age: 25) // Correct usage
def invalidPerson = new Person(name: 'Jane Doe', age: 'twenty-five') // Runtime error
Commentary: In this example, the wrong assignment to the age
field will lead to a runtime error. To avoid such issues, you can utilize static type checking in your Groovy configuration:
@TypeChecked
class Person {
String name
int age
}
By applying the static type checking annotation, you can ensure the compiled code adheres to the defined data types.
2. Implement Defensive Programming Practices
Defensive programming is the practice of writing code to handle unforeseen situations gracefully. This approach can safeguard your application against bugs that could arise from unexpected inputs or states.
Why Defensive Programming?
By anticipating potential failure points, you can significantly reduce the chances of runtime exceptions and improve code maintainability.
Code Example
class Calculator {
int divide(int dividend, int divisor) {
if (divisor == 0) {
throw new IllegalArgumentException("Divisor cannot be zero.")
}
return dividend / divisor
}
}
def calc = new Calculator()
println calc.divide(10, 2) // Outputs 5
println calc.divide(10, 0) // Throws IllegalArgumentException
Commentary: In the Calculator
class, we anticipate a division by zero scenario and handle it proactively. This approach is not just about capturing bugs but also about providing clear feedback to the developers and users of your code.
3. Use Groovy's Built-In Exception Handling
Groovy provides flexible exception handling that can be used to manage and log potential bugs. By utilizing try-catch blocks, you can gracefully handle errors, allowing your application to continue running while also providing necessary debugging information.
Why Exception Handling?
Handling exceptions cleanly can prevent application crashes, guiding developers toward fixing the underlying problems.
Code Example
try {
// Some code that may throw an exception
String result = riskyOperation()
println "Result: $result"
} catch (Exception e) {
println "An error occurred: ${e.message}"
} finally {
println "Clean up resources if needed."
}
Commentary: This example showcases a typical exception handling flow. Implementing this structure in your code can shield your applications from abrupt crashes and provide insights into what went wrong.
4. Use Unit Testing
Unit testing is an essential practice in software development that involves testing individual components of your code in isolation. Groovy’s integration with popular testing frameworks like JUnit makes it straightforward to ensure your objects behave as expected.
Why Unit Testing?
Creating a suite of unit tests can help catch bugs early and design your code better. Testing ensures your objects maintain the expected behavior as your codebase evolves.
Code Example
import spock.lang.Specification
class CalculatorSpec extends Specification {
def "should divide two numbers"() {
expect:
new Calculator().divide(10, 2) == 5
}
def "should throw exception when divisor is zero"() {
when:
new Calculator().divide(10, 0)
then:
thrown(IllegalArgumentException)
}
}
Commentary: In this example, we define two tests for the Calculator
class. The first verifies the correct division, while the second asserts that dividing by zero correctly throws an exception. By regularly running these tests, you ensure your code remains reliable as changes occur.
5. Utilize Dependency Injection
Dependency injection (DI) is a design pattern that allows for decoupling component dependencies. In Groovy, leveraging dependency injection frameworks can contribute to a clean architecture and help prevent bugs that arise from tightly coupled codebases.
Why Dependency Injection?
DI enhances the modularity and testability of your code, making it easier to manage dependencies and reducing the risk of bugs due to interlinked components.
Code Example
class UserService {
MessageService messageService
void notifyUser(String user) {
messageService.send(user, "Notification")
}
}
class MessageService {
void send(String recipient, String message) {
println "Sending '$message' to $recipient"
}
}
// Using a Dependency Injection framework like Spring
def messageService = new MessageService()
def userService = new UserService(messageService: messageService)
userService.notifyUser('john.doe@example.com')
Commentary: Here, UserService
depends on MessageService
to function. By injecting MessageService
instead of instantiating it, you decouple the two classes. This approach aids in maintaining and testing each part independently.
Closing Remarks
In summary, while Groovy offers great flexibility and capabilities, ensuring the security and bug-resilience of your Groovy objects through careful practices is crucial. By leveraging type checking, implementing defensive programming, utilizing robust exception handling, adopting unit testing, and employing dependency injection, you can create more stable and maintainable applications.
For further reading, consider exploring resources on Groovy Documentation, or dive into the world of unit testing with JUnit and Spock Framework. Implementing these principles will undoubtedly help you guard your Groovy applications against bugs and improve overall code quality. Happy coding!
Checkout our other articles