Mastering Top-N Queries: Avoiding Priority Queue Pitfalls
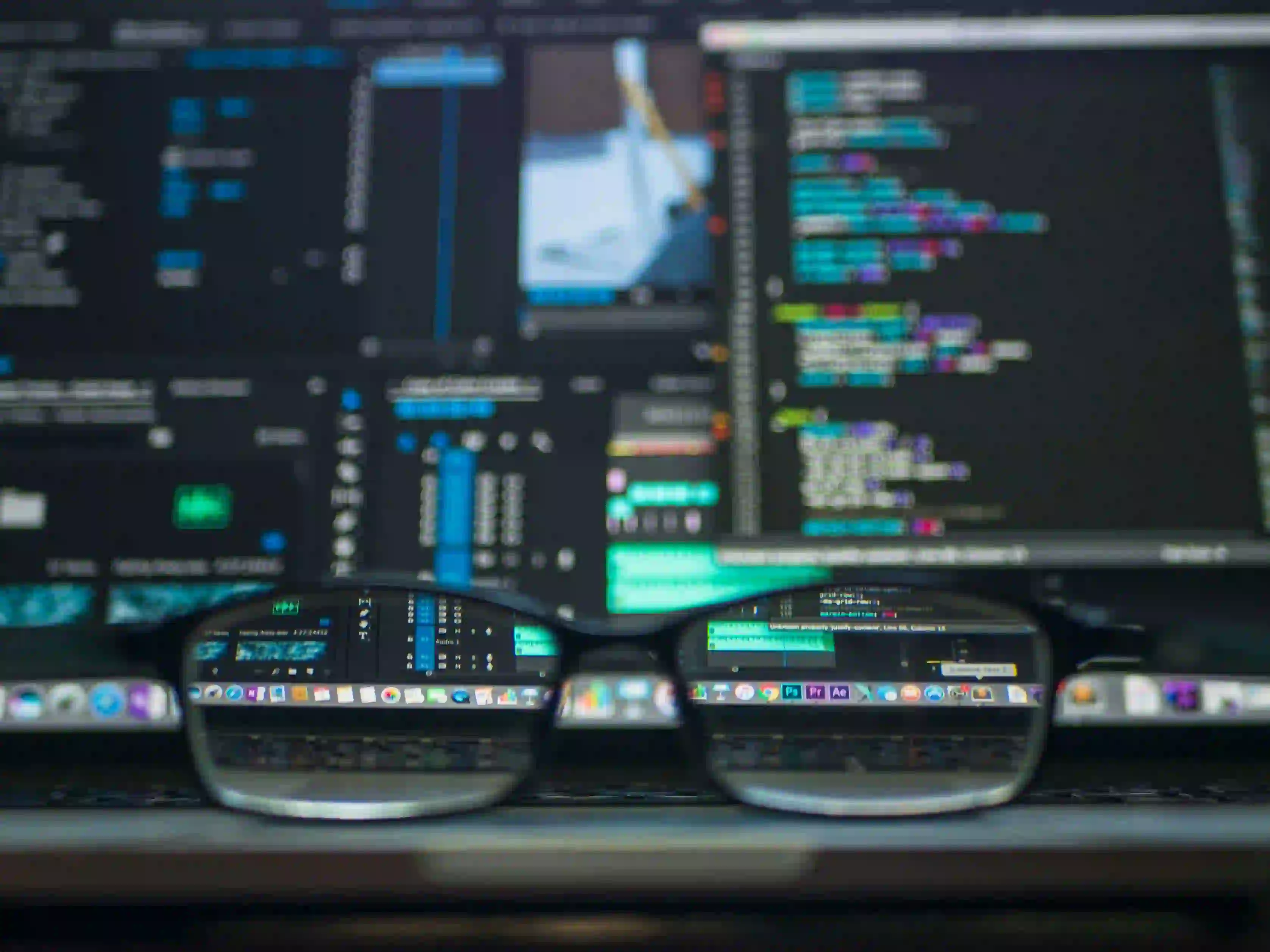
Mastering Top-N Queries: Avoiding Priority Queue Pitfalls in Java
In today's data-driven world, we frequently need to extract valuable insights from large sets of information. Whether you are building a recommendation system, aggregating reporting statistics, or conducting performance analysis, the ability to efficiently execute Top-N queries is paramount. Top-N queries allow you to fetch the top N elements from a dataset based on certain criteria, such as scores, sales, or ratings.
However, when implementing Top-N queries within your Java applications, it's important to avoid the pitfalls associated with using priority queues and to master alternative strategies that can lead to improved performance.
Understanding Top-N Queries
A Top-N query retrieves the highest (or lowest) N items from a large collection based on a defined criteria. The challenge lies not only in retrieving the correct elements but also doing so efficiently. As datasets grow, the naive implementations can quickly become suboptimal.
The Naive Approach
The naive method for implementing a Top-N query might involve sorting the entire dataset and then selecting the top N items. Here's how that looks in Java:
import java.util.Arrays;
public class TopNQuery {
public static int[] getTopN(int[] numbers, int n) {
Arrays.sort(numbers); // Step 1: Sort the entire array
return Arrays.copyOfRange(numbers, numbers.length - n, numbers.length); // Step 2: Select top N
}
}
While this code works fine for small datasets, sorting isn't efficient for larger ones — it operates in O(N log N) time complexity.
Priority Queue Pitfalls
To improve efficiency, one might consider using a priority queue, specifically a min-heap. This data structure allows you to maintain the top N elements as you iterate through the dataset. However, there are several pitfalls to be aware of:
- Memory Overhead: Maintaining a priority queue can require additional memory.
- Unintentional Sorting: The structure maintains only the top N elements, but it can be less intuitive than sorting.
- Performance Fluctuations: The performance may vary depending on the input data characteristics.
Using PriorityQueue in Java
Here’s an example of using a PriorityQueue
to implement a Top-N query:
import java.util.PriorityQueue;
public class TopNWithPriorityQueue {
public static int[] getTopN(int[] numbers, int n) {
PriorityQueue<Integer> minHeap = new PriorityQueue<>(n); // Initialize a min-heap
for (int number : numbers) {
if (minHeap.size() < n) {
minHeap.offer(number); // Add the number if the heap has less than N elements
} else if (number > minHeap.peek()) {
minHeap.poll(); // Remove the minimum element (the root)
minHeap.offer(number); // Add the new number
}
}
// Convert the priority queue to an array
int[] topN = new int[minHeap.size()];
for (int i = 0; i < topN.length; i++) {
topN[i] = minHeap.poll();
}
return topN;
}
}
Commentary on Priority Queue Approach
- Efficiency: This implementation operates in O(N log N) time complexity as well, but it has a better space complexity of O(N), which is more efficient for larger datasets.
- Use Cases: Priority queues work particularly well in streaming data scenarios where continuous data flow allows for incremental analysis.
Alternative Approaches to Top-N Queries
While priority queues can be advantageous, they still come with challenges that may not fit all situations. Therefore, it’s essential to consider alternative methods such as:
- Sorting and Slicing: For static datasets, sorting once and slicing is often enough.
- Bucket Sort: If the range of the data is known (like ranking scores), this can significantly reduce complexity.
- Using Java Streams: For Java 8 and above, streams can provide an elegant way to achieve Top-N queries with less boilerplate.
Here's a simplified approach using Java Streams:
import java.util.Arrays;
public class TopNWithStreams {
public static int[] getTopN(int[] numbers, int n) {
return Arrays.stream(numbers)
.boxed()
.sorted((a, b) -> b.compareTo(a)) // Sort in descending order
.limit(n) // Limit to top N elements
.mapToInt(Integer::intValue)
.toArray(); // Convert back to array
}
}
Streaming Advantages
- Elegance: The stream API provides a more readable and functional style of programming.
- Inline Processing: You can process data on-the-fly without the need for additional storage.
Best Practices for Top-N Queries
- Choose the Right Data Structure: Depending on your use case, analyze if a priority queue, sorted list, or different technique fits your needs.
- Data Characteristics: Understand the nature of your dataset—its size, distribution, and frequency of queries—to choose the most efficient approach.
- Performance Testing: Always test performance with datasets of various sizes to ensure your implementation is scalable.
To Wrap Things Up
Mastering Top-N queries is essential for building efficient applications that deal with large datasets. While priority queues offer a balance of simplicity and performance, exploring alternatives like sorting and bucket sort can yield better results based on your specific context. Always prioritize efficiency and clarity in your code to make it maintainable and scalable.
For more insights on managing data efficiently, you might find these resources helpful:
- Big O Notation: Sorting Algorithms - A guide in understanding the efficiency of various algorithms.
- Java Collections Framework - A comprehensive overview of data structures Java has to offer.
As you venture into implementing Top-N queries, keep these strategies in mind to craft robust Java applications that handle your data adeptly. Happy coding!