Troubleshooting Apache Camel Plugin Issues in IntelliJ IDEA
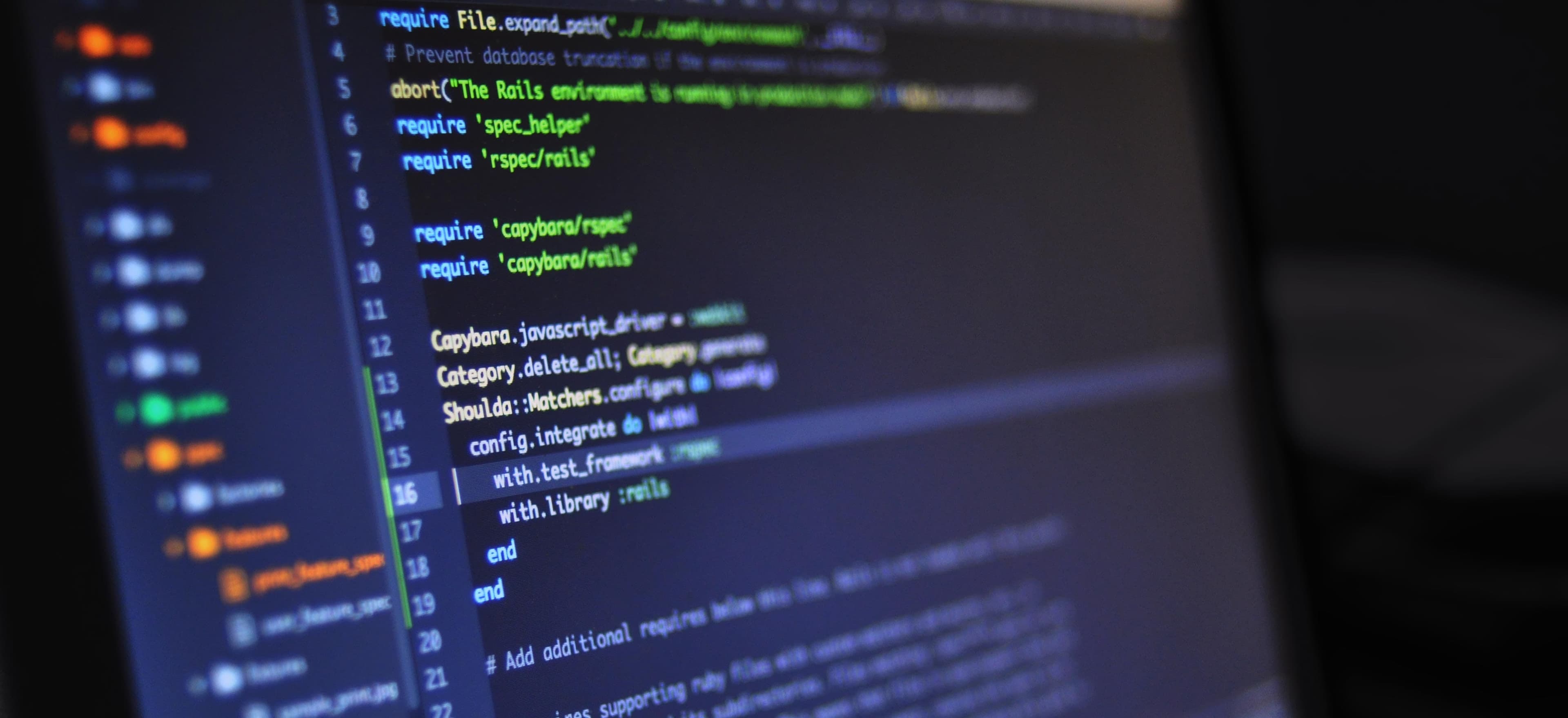
- Published on
Troubleshooting Apache Camel Plugin Issues in IntelliJ IDEA
Apache Camel is a powerful integration framework that allows developers to connect different applications, protocols, and data formats seamlessly. When using IntelliJ IDEA for Apache Camel development, you may come across several issues with the Camel plugin. In this article, we will explore common problems and demonstrate effective troubleshooting techniques to help you get back on track.
Understanding Apache Camel and IntelliJ IDEA
Apache Camel provides a set of tools to simplify the creation of integration solutions. Its rich ecosystem of components enables developers to connect and transform data across various technologies. IntelliJ IDEA, being a robust Integrated Development Environment (IDE), offers a dedicated Camel plugin designed to streamline Camel development with visual editing, auto-completion, and validation capabilities.
Before diving into troubleshooting, it's essential to check that you have the latest version of IntelliJ IDEA and the Apache Camel plugin installed. Running outdated software can lead to compatibility and functionality issues.
Common Issues with Apache Camel Plugin
1. Plugin Not Installed or Enabled
The first issue that developers face is the Apache Camel plugin not being installed or enabled in IDEA. Without this plugin, you lose access to many of the Camel features.
Solution: Here's how to check:
- Go to
File
>Settings
(orPreferences
if you are on macOS). - In the left pane, locate
Plugins
. - Search for "Apache Camel.” If it isn’t installed, click “Marketplace” and search for the Apache Camel plugin to install it.
2. Unable to Create Camel Context
Creating a Camel context is a fundamental task. However, sometimes developers encounter errors when trying to set up a new route.
Example Code:
import org.apache.camel.CamelContext;
import org.apache.camel.impl.DefaultCamelContext;
public class Main {
public static void main(String[] args) {
CamelContext context = new DefaultCamelContext();
try {
// Starting the Camel context
context.start();
System.out.println("Camel Context started.");
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
context.stop();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
Why Use This Code?: The above code initializes a Camel context using the DefaultCamelContext
for routing and processing. The try-catch block ensures that any exceptions raised during context handling are properly logged. This is crucial for diagnosing what went wrong.
3. Route Configuration Errors
When routes are not defined correctly, the Camel plugin may not recognize them, leading to runtime errors. The issues may stem from XML DSL or Java DSL configurations.
Common XML Example:
<camelContext xmlns="http://camel.apache.org/schema/spring">
<route id="myRoute">
<from uri="direct:start"/>
<log message="Received message: ${body}"/>
</route>
</camelContext>
Why This Matters: This XML configuration establishes a route that listens on a direct:start
endpoint and logs incoming messages. Properly defining routes ensures that the Camel context can manage messages correctly. Misconfigured URI or missing required attributes can lead to problems, so always validate your configuration against the schema.
4. No Camel Icons or Syntax Highlighting in Routes
Occasionally, you might notice that the Camel-specific icons or syntax highlighting in your routes are missing. This often indicates an issue with the Camel plugin’s recognition of your project.
Solution:
- Ensure that your project setup includes the necessary dependencies in your
pom.xml
orbuild.gradle
file if you're using Maven or Gradle.
Example for Maven Project:
<dependencies>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-core</artifactId>
<version>3.x.x</version> <!-- Use the appropriate version -->
</dependency>
</dependencies>
Why This is Important: Listing the necessary dependencies ensures that the Camel plugin can recognize the classes and configurations in your project. It’s a basic yet integral step for plugin functionality.
5. Debugging Apache Camel Routes
Debugging Camel routes can sometimes be tricky without a clear understanding of the framework’s workings. Here are some basic debugging tips:
- Use
log
components wisely within the route definitions to output or inspect message processing. - Utilize breakpoints in your IDE, as it allows you to step through the code execution.
Example for Logging:
from("direct:start")
.log("Received message: ${body}")
.to("mock:result");
Why Use Logging?: Including logging statements within routes can help trace the flow of messages and identify where issues might arise. This is integral to effective troubleshooting and development.
Additional Resources and Help
If you continue to face challenges even after applying the solutions mentioned above, consider utilizing additional resources. The official Apache Camel documentation provides crucial insights and comprehensive tutorials about different components and integrations.
Communities like Stack Overflow can also be incredibly resourceful when looking for assistance. Here, you can find solutions to problems faced by others or post your unique issue for community help.
To Wrap Things Up
Troubleshooting issues with the Apache Camel Plugin in IntelliJ IDEA does not have to be a daunting task. By following proper installation procedures, ensuring correct code configurations, and utilizing effective debugging practices, you can resolve many common problems swiftly.
As you enhance your integration skills with Apache Camel, don’t forget to keep your tools updated and take advantage of community knowledge. Happy coding!