Overcoming Common Issues with Pub/Sub Local Emulator
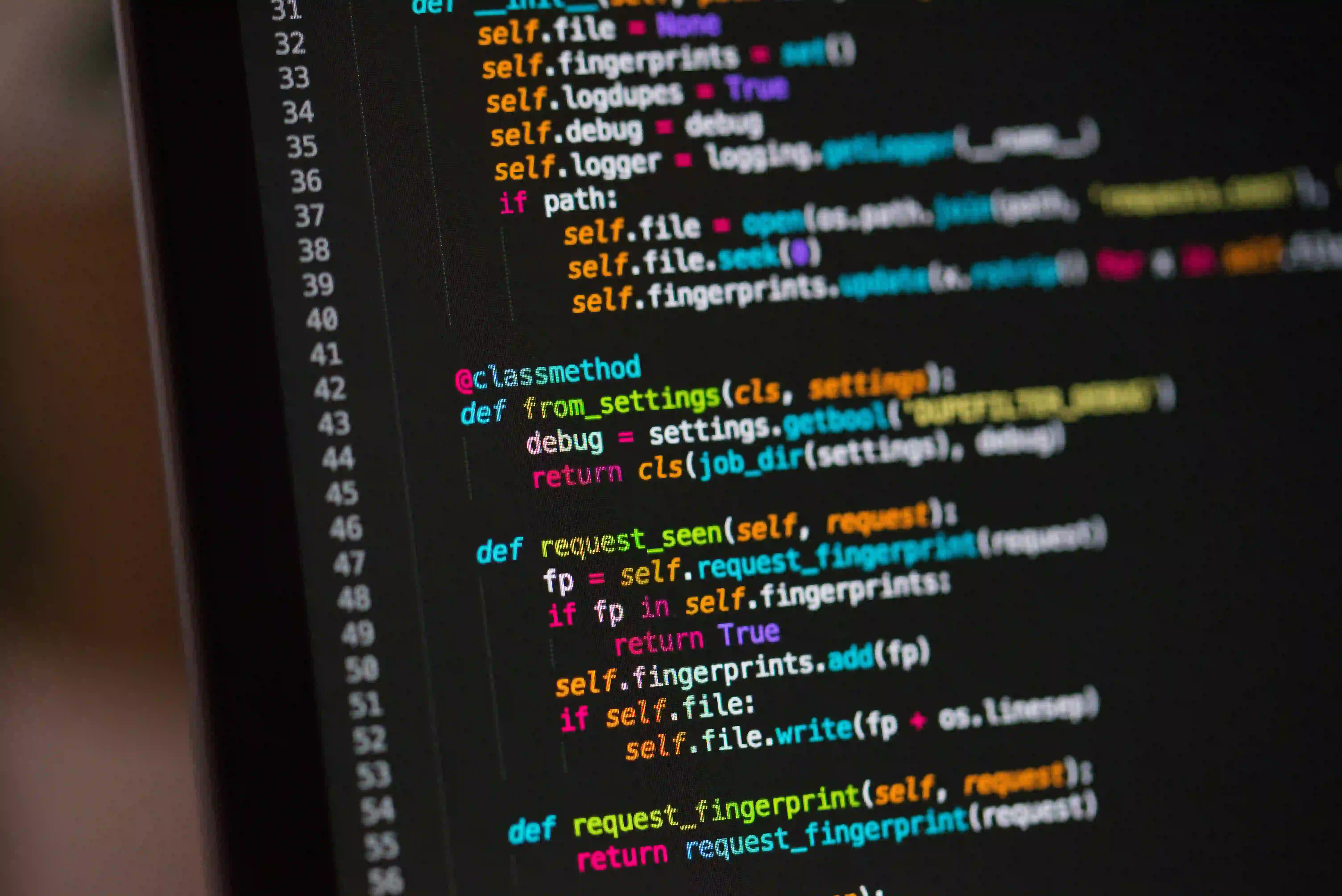
Overcoming Common Issues with Pub/Sub Local Emulator
Google Cloud Pub/Sub is an essential service for building event-driven architectures, allowing applications to communicate with one another asynchronously. As developers, we often require a local environment to test and simulate these interactions before deploying them in a production setting. Enter the Pub/Sub Local Emulator.
In this blog post, we will explore common issues developers face while using the Pub/Sub Local Emulator and provide solutions to overcome them. This discussion aims to aid both novice and experienced developers to establish a smooth local testing environment.
What is the Pub/Sub Local Emulator?
The Pub/Sub Local Emulator is a tool that allows developers to run a local version of the Google Cloud Pub/Sub API. It is beneficial for testing and development without incurring costs on the actual Google Cloud services. The emulator simulates the behavior of Google Cloud Pub/Sub to a large extent, providing a playground where you can develop your applications.
Why Use the Local Emulator?
- Cost-Effective: You incur no charges while developing and testing.
- Faster Development Cycle: Local testing allows for quicker debugging and iteration.
- No Internet Required: You can run the emulator without internet access, making it ideal for situations with limited connectivity.
Setting Up the Local Emulator
To get started, you first need to install the Google Cloud SDK. If you haven't done so, follow the installation guide.
Once you have the SDK installed, you can start the emulator using the command:
gcloud beta emulators pubsub start
This command will provide you with environment variables to set, which is crucial for your applications to communicate with the emulator.
export PUBSUB_EMULATOR_HOST=localhost:8085
You should also run the command to create project and subscriptions:
gcloud pubsub topics create my-topic
gcloud pubsub subscriptions create my-sub --topic my-topic
Common Issues and Solutions
While using the Pub/Sub local emulator is advantageous, you may encounter some common issues. Below are some of these issues followed by suggested solutions.
Issue 1: Connection Refused
Symptom: When your application tries to connect to the Pub/Sub Local Emulator, you receive a "connection refused" error.
Solution: First, ensure that the emulator is running. Check the terminal where you started the emulator. If you see error messages or notifications that the emulator has stopped unexpectedly, restart it. Additionally, verify that you have set the PUBSUB_EMULATOR_HOST
environment variable correctly.
Issue 2: Messages Not Being Received
Symptom: You publish messages to a topic, but the subscriber does not receive them.
Solution: This might occur due to improperly configured subscriptions or topics. Always make sure that your publisher and subscriber are correctly pointed to the same topic and subscription.
Here's an example of how to configure a publisher and subscriber in Java:
import com.google.cloud.pubsub.v1.Publisher;
import com.google.pubsub.v1.PubsubMessage;
import java.util.concurrent.TimeUnit;
public class PubSubPublisher {
private final Publisher publisher;
public PubSubPublisher(String topicId) throws Exception {
publisher = Publisher.newBuilder(topicId).build();
}
public void publishMessage(String messageContent) {
PubsubMessage message = PubsubMessage.newBuilder()
.setData(ByteString.copyFromUtf8(messageContent))
.build();
publisher.publish(message);
}
public void shutdown() throws InterruptedException {
publisher.shutdown();
publisher.awaitTermination(1, TimeUnit.MINUTES);
}
}
Commentary: This code initializes a publisher for a given topic ID. It constructs a message with the provided content and publishes it. Ensuring you have one instance of a publisher is crucial, as continually creating new publisher instances can cause concurrency issues.
Issue 3: Incorrect Data Format
Symptom: The data sent to Pub/Sub does not match the expected format, causing subscribers to fail processing messages.
Solution: Verify the serialization format being used for messages. Google Cloud Pub/Sub supports several formats, including JSON, Avro, and Protocol Buffers. It's essential to ensure that both the publisher and subscriber are using compatible formats.
Here's an example of sending a JSON formatted message:
import com.google.pubsub.v1.PubsubMessage;
String jsonData = "{\"eventType\":\"user_signup\",\"userId\":\"12345\"}";
PubsubMessage message = PubsubMessage.newBuilder()
.setData(ByteString.copyFromUtf8(jsonData))
.build();
Commentary: Here, we create a PubsubMessage by serializing a JSON object. Correct serialization ensures that subscribers can deserialize and interpret the message as intended.
Issue 4: Topic and Subscription Not Found
Symptom: When attempting to publish or subscribe to a topic, you receive a "topic not found" error.
Solution: Make sure you have created the topic and subscription before trying to use them. You can confirm this by listing existing topics and subscriptions:
gcloud pubsub topics list
gcloud pubsub subscriptions list
Issue 5: Throttling and Quotas
Symptom: After running tests, you may see throttling or quota-related issues.
Solution: Ensure that you configure your tests to avoid excessive messaging rates that you might not encounter in a local environment. The emulator caters to set limitations on the number of messages that can be published or consumed per second.
Integrating with Java
As we work with the Pub/Sub Local Emulator, integrating it into your Java application is a necessary step. Here's how we can set up a subscriber to listen for our messages:
import com.google.api.core.ApiFuture;
import com.google.cloud.pubsub.v1.MessageReceiver;
import com.google.cloud.pubsub.v1.Subscriber;
import com.google.pubsub.v1.PubsubMessage;
public class PubSubSubscriber {
public static void main(String[] args) {
String subscriptionId = "my-subscription-id";
MessageReceiver receiver =
(PubsubMessage message, AckReplyConsumer consumer) -> {
System.out.println("Received message: " + message.getData().toStringUtf8());
consumer.ack();
};
Subscriber subscriber = Subscriber.newBuilder(subscriptionId, receiver).build();
subscriber.startAsync().awaitRunning();
}
}
Commentary: In this Java snippet, we set up a simple subscriber that listens to messages. Upon receiving a message, it prints the message data to the console and acknowledges it. This code gives a practical demonstration of how subscribers in Java work with the Pub/Sub service.
Bringing It All Together
By now, you should feel equipped to tackle the common issues associated with the Pub/Sub Local Emulator. A thorough understanding of your application's development environment is critical to successful debugging and testing.
Remember to leverage the Google Cloud documentation for deeper insights, and don't hesitate to check forums or communities for shared developer experiences. The Pub/Sub Local Emulator, when properly utilized, provides a robust framework for testing event-driven applications.
For more information on Pub/Sub, visit the official Google Cloud Pub/Sub documentation.
Happy coding!