Common JAXB Errors and How to Fix Them
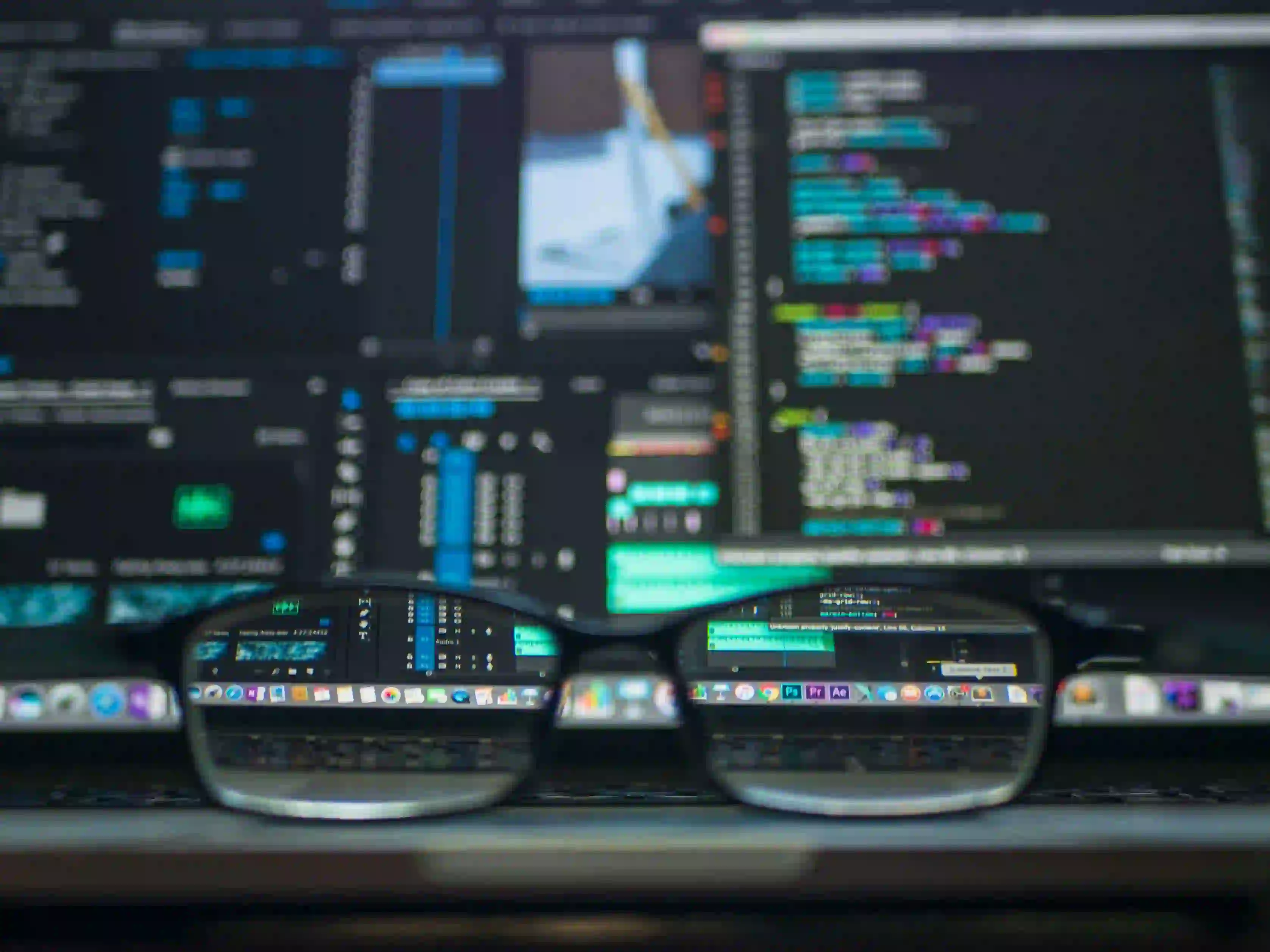
Common JAXB Errors and How to Fix Them
Java Architecture for XML Binding (JAXB) is a powerful tool that enables Java developers to easily bind XML schemas and Java representations. This facilitates the handling of XML data by allowing for smooth serialization and deserialization. However, JAXB is not free from common pitfalls, and developers often face several errors. In this blog post, we will discuss some common JAXB errors and their solutions, ensuring that you can work more effectively with JAXB in your Java projects.
Table of Contents
- 1. Missing JAXB Annotations
- 2. Class Not Found Exception
- 3. Incompatible XML Schema
- 4. JAXBContext Initialization Issues
- 5. Unexpected End of The Stream
- 6. Conclusion
1. Missing JAXB Annotations
One of the most common errors when working with JAXB is the omission of necessary annotations in your Java classes. JAXB requires specific annotations to properly marshal (convert Java objects to XML) and unmarshal (convert XML data back to Java objects).
Example
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class Employee {
private String name;
private String role;
@XmlElement
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlElement
public String getRole() {
return role;
}
public void setRole(String role) {
this.role = role;
}
}
Why It Matters: Without annotations like @XmlRootElement
and @XmlElement
, JAXB doesn’t know how to process your Java objects. Always ensure you annotate your classes appropriately.
Fix: Check your Java classes for missing JAXB annotations and add them as needed based on how you want your XML data structured.
2. Class Not Found Exception
When you run your JAXB-related code and encounter a ClassNotFoundException
, it usually stems from a missing class that's referenced in your XML. This can happen if a class type doesn't match the expected XML structure.
Example Error
java.lang.ClassNotFoundException: com.example.Employee
Why It Matters: JAXB relies on specific class references to perform marshalling and unmarshalling. Missing classes can lead to failures in these operations.
Fix: Ensure that all classes are correctly packaged and included in your classpath. If using Maven, verify that your dependencies are correctly specified in your pom.xml
.
3. Incompatible XML Schema
When working with JAXB, you may generate Java classes from an XML schema (XSD). If you modify your XML schema but don't regenerate your classes, you may run into compatibility issues.
Example
You generate classes from an old version of your XSD and make changes in the schema later. As a result, you might see unexpected behavior when you marshal or unmarshal XML.
Why It Matters: Any mismatch between the XML structure and the Java class representations can lead to serialization errors.
Fix: Always regenerate your Java classes after making changes to your XML schema. Use the xjc
tool for this purpose, which can be run via command line.
xjc -d src/main/java -p com.example.generated src/main/resources/schema.xsd
4. JAXBContext Initialization Issues
Another common issue developers encounter is JAXBContext
initialization problems. Incorrect usage can prevent your application from creating the context necessary for JAXB operations.
Example
JAXBContext context = JAXBContext.newInstance(Employee.class);
Why It Matters: JAXBContext
must know about every class it should work with, so attempting to instantiate it with an incorrect class will lead to a JAXBException
.
Fix: Ensure that you pass the correct class or classes that you intend to use in the JAXBContext
.
JAXBContext context = JAXBContext.newInstance(Employee.class, Department.class);
This way, you're ensuring that the context is aware of all relevant classes.
5. Unexpected End of The Stream
This error often occurs during unmarshalling when JAXB expects more data but encounters the end of the document unexpectedly.
Example Error
javax.xml.bind.UnmarshalException: unexpected end of the stream
Why It Matters: This might indicate that the XML data is corrupt or truncated, leading JAXB to throw an exception.
Fix: Validate the XML input before attempting to unmarshal. You can use a schema validator to ensure that the XML document conforms to its specified schema.
Example of XML Validation
SchemaFactory schemaFactory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
Schema schema = schemaFactory.newSchema(new File("schema.xsd"));
Unmarshal = context.createUnmarshaller();
unmarshaller.setSchema(schema);
This code snippet incorporates schema validation before unmarshalling the data, reducing the likelihood of encountering an Unexpected End of The Stream
error.
6. Conclusion
Working with JAXB can greatly simplify XML processing in your Java applications. However, like any technology, it is not without its challenges. By understanding these common JAXB errors—ranging from missing annotations to context initialization issues—you can develop more robust applications.
For further reading on JAXB and XML processing in Java, check out the official JAXB documentation and consider exploring related tools like Jackson for JSON if you work with JSON data.
With these insights, you can confidently tackle any issues that may arise during your JAXB development endeavors. Happy coding!