Troubleshooting Java Mail with Gmail SMTP Configuration
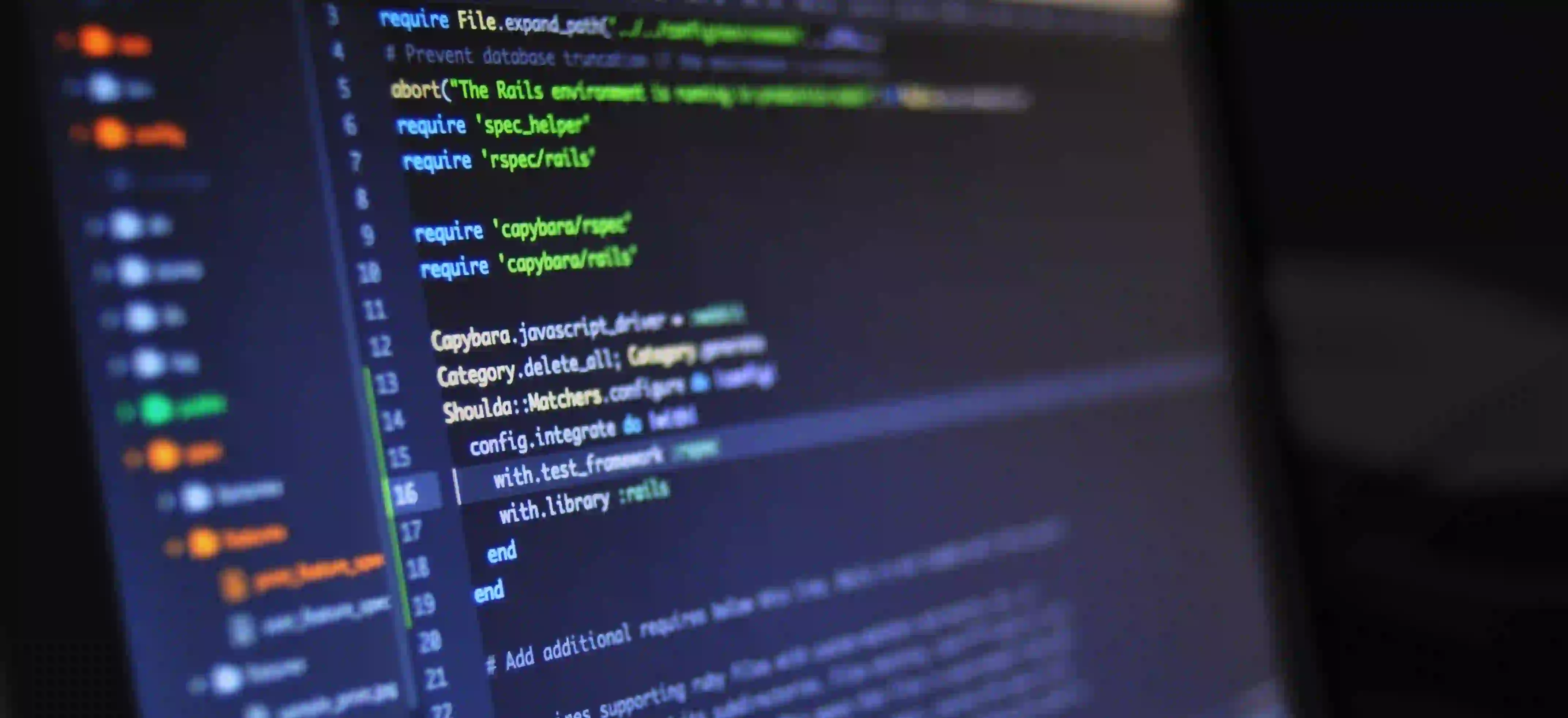
Troubleshooting Java Mail with Gmail SMTP Configuration
Sending emails from a Java application can enhance user experience significantly. However, configuring Java Mail with Gmail's SMTP server can be daunting. This blog post will guide you through the troubleshooting process, drawing on common issues and providing solutions.
Table of Contents
- Understanding JavaMail API
- Gmail SMTP Settings
- Common Issues and Solutions
- Code Example
- Conclusion
1. Understanding JavaMail API
The JavaMail API provides a platform-independent framework to create, send, receive, and read emails. It supports common email protocols like SMTP, POP3, and IMAP. To work with JavaMail, you will typically need the JavaMail library and an SMTP server to send your emails.
Understanding how JavaMail integrates with Java applications is crucial. If you are looking for more information on the JavaMail API, consider checking Oracle’s official documentation for deeper insights.
2. Gmail SMTP Settings
Gmail's SMTP server requires specific configurations to allow Java applications to send emails. Here are the essential SMTP settings you'll need:
- SMTP Server: smtp.gmail.com
- Port:
- 465 (SSL)
- 587 (TLS)
- Authentication: Required
- Secure Connection: Required (SSL/TLS)
Before proceeding, ensure that you have enabled Less secure app access in your Google account settings if you are not using OAuth2 authentication. Please note that this may pose a security risk.
3. Common Issues and Solutions
Issue 1: Authentication Failed
Description: You receive an "Authentication failed" error message.
Solution: A common cause is that your email or password is incorrect, or Less secure app access is not enabled. Verify your credentials by logging into Gmail through a browser. If two-factor authentication (2FA) is enabled, you'll need to create an App Password for use with JavaMail.
To create an App Password:
- Go to your Google Account.
- Navigate to Security.
- Under "Signing in to Google", select App passwords.
- Follow the prompts to generate a new password.
Issue 2: SMTP Server Timeout
Description: You receive a connection timeout error.
Solution: This usually indicates that your application cannot connect to Gmail’s SMTP server due to a firewall or connection issue.
- Check your network connection.
- Ensure your firewall settings are not blocking the Java application.
- Try using a different network to see if it resolves the issue.
For more detailed instructions on troubleshooting connection errors, check Google’s official support page here.
Issue 3: Security Issues and Firewalls
Description: Connection refused by the SMTP server or blocked by your ISP.
Solution: If you are behind a firewall or your ISP blocks certain ports, connections may fail.
- Ensure that the ports 465 or 587 are open.
- Adjust your firewall settings to allow Java applications to send data through these ports.
4. Code Example
Now let's delve into a simple code example demonstrating how to configure JavaMail with Gmail's SMTP settings. This example illustrates the process of sending an email using JavaMail.
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class EmailSender {
public static void main(String[] args) {
// Step 1: Set the SMTP server properties
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.gmail.com");
props.put("mail.smtp.port", "587");
// Step 2: Create a session with an authenticator
Session session = Session.getInstance(props, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("your_email@gmail.com", "your_app_password");
}
});
try {
// Step 3: Create a default MimeMessage object
Message message = new MimeMessage(session);
// Step 4: Set From: header field of the header
message.setFrom(new InternetAddress("your_email@gmail.com"));
// Step 5: Set To: header field of the header
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse("recipient_email@example.com"));
// Step 6: Set Subject: header field
message.setSubject("Hello from Java!");
// Step 7: Now set the actual message
message.setText("This is a test email sent from Java application!");
// Step 8: Send message
Transport.send(message);
System.out.println("Email sent successfully!");
} catch (MessagingException e) {
e.printStackTrace();
}
}
}
Code Explanation
-
SMTP Properties: The properties configured in the
props
variable define how to connect to Gmail's SMTP server. Themail.smtp.starttls.enable
property ensures a secure connection. -
Session Object: The
Session
object is created with the defined properties along with authentication using your Gmail credentials. This is where the App Password comes into play, ensuring a more secure means of authentication. -
MimeMessage: A
MimeMessage
object represents the email message itself. Here, you can set the sender, recipient, subject, and body of the email. -
Sending the Message: Finally, the
Transport.send(message)
method sends the email, concluding the process.
Make sure to replace your_email@gmail.com
and your_app_password
with your actual email and generated App Password. Also, update recipient_email@example.com
to the address you want to send the email to.
5. Conclusion
Configuring JavaMail with Gmail's SMTP server can be intricate, but understanding the common issues and their solutions will make the process smoother. Always ensure you have the correct SMTP settings, and be mindful of security features when dealing with authentication.
Moreover, do not ignore the importance of error handling in your production-grade applications. Proper logging will help identify and troubleshoot any future issues effectively.
For more advanced configurations like using OAuth2, consider exploring additional resources on JavaMail or refer to the Gmail API documentation. Happy coding!