Mastering JSON Merge Patch: Common Pitfalls to Avoid
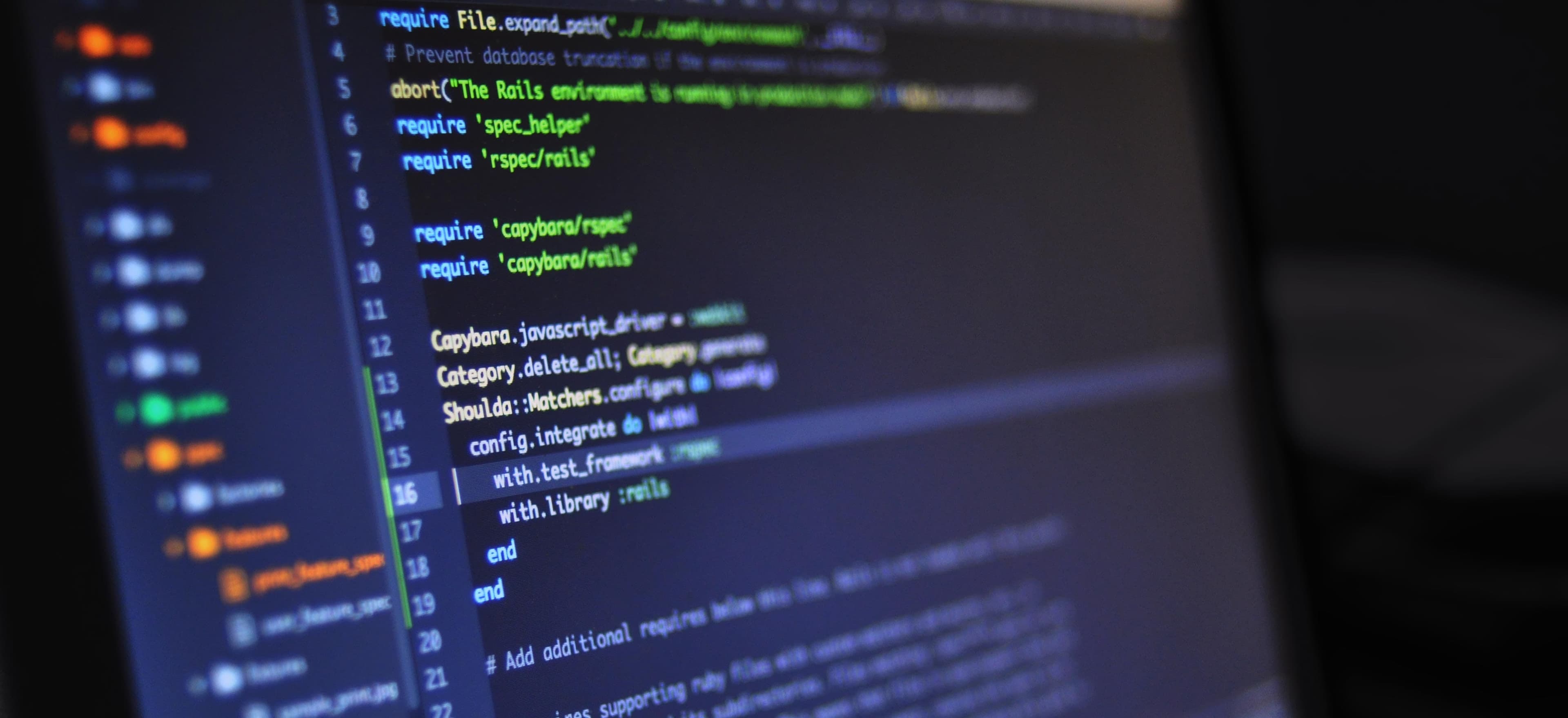
- Published on
Mastering JSON Merge Patch: Common Pitfalls to Avoid
In today's interconnected software landscape, the need for efficient data interchange formats has never been more crucial. One such format that has gained prominence is JSON (JavaScript Object Notation). With its lightweight nature and easy readability, it's no wonder that developers gravitate towards JSON in many applications, especially in RESTful APIs. However, when dealing with JSON documents, especially in scenarios requiring partial updates, errors can arise unless proper handling is employed. This is where JSON Merge Patch comes into play.
What is JSON Merge Patch?
JSON Merge Patch is a document format used to apply updates to a JSON document. It allows a client to send a patch of the original JSON document, which makes it a powerful tool for updating resources without needing to resend the entire document. The official specification can be found in RFC 7396.
Why Use JSON Merge Patch?
- Reduced Payload Size: By only sending the changes rather than the entire document, network bandwidth is preserved, which is essential for mobile and low-bandwidth environments.
- Simplicity: The format is intuitive and easy to understand. This allows developers to implement and troubleshoot more efficiently.
- Rich Semantics: JSON Merge Patch supports a rich set of operations, allowing for a wide variety of updates, including object additions, deletions, and modifications.
Basic Structure
Here’s a simple example of a JSON Merge Patch:
Original JSON Document
{
"title": "My Blog Post",
"content": "This is the content of the post.",
"tags": ["programming", "json", "api"]
}
Merge Patch
{
"content": "Updated content of the post.",
"tags": ["tutorial"]
}
Resulting JSON Document After Merge
{
"title": "My Blog Post",
"content": "Updated content of the post.",
"tags": ["tutorial"]
}
Commentary: In this example, the content
field was updated, and the tags
array was replaced entirely. Notice the importance of going beyond simple updates to fully understand implications, such as array replacement versus item updating.
Common Pitfalls to Avoid
While JSON Merge Patch simplifies the process of updating JSON documents, there are common pitfalls that developers should watch out for. Let’s dive deeper into these obstacles and discuss how to avoid them.
1. Overwriting vs. Merging Arrays
One significant aspect of JSON Merge Patch is that it replaces an entire array instead of merging it. Newcomers often mistakenly believe it merges content.
Example of the Pitfall
Suppose you start with the following JSON document:
{
"tags": ["programming", "json"]
}
And your merge patch is:
{
"tags": ["api"]
}
The resulting document will be:
{
"tags": ["api"]
}
Correct Approach: If your intent is simply to add "api" to the tags, you need to merge them manually, or consider using the JSON Patch format which does support array operations.
2. Handling Null Values
In JSON Merge Patch, setting a field to null means to remove that field from the document entirely. This can sometimes lead to unexpected data loss if not handled properly.
Example
Starting JSON document:
{
"username": "john_doe",
"age": 30
}
Merge patch parsed as:
{
"age": null
}
This leads to:
{
"username": "john_doe"
}
Solution: Document your APIs clearly so consumers know that sending null will delete fields. Consider alternatives or implement safeguards against such exposures.
3. Field Typing Mismatch
Merging patches that inadvertently change the type of data can lead to runtime errors or data corruption. This often happens during schema evolution.
Example of the Pitfall
Consider the original document:
{
"id": 123,
"active": true
}
And the merge patch:
{
"id": "123"
}
Here, the id
has been changed from an integer to a string, leading to possible type errors downstream.
Best Practice: Ensure that any updates maintain the types consistent with the data model. Utilize validation frameworks to enforce correct types.
4. Non-standard JSON Structures
Developers sometimes use complex structures that are non-conventional or heavily nested. Understand the JSON Merge Patch implications before applying it to these structures.
Example
Starting Document:
{
"user": {
"name": "Alice",
"contact": {
"email": "alice@example.com"
}
}
}
If one sends:
{
"user": {
"contact": null
}
}
The entire contact information is lost.
Solution: Understand the structure deeply before implementing. If deletion is required, specify exactly which field you want to remove.
5. Ignoring Security Considerations
When implementing a patching mechanism, security is paramount. JSON Merge Patch can be less secure if sensitive data is accidentally exposed or altered through incorrect merge operations.
Best Practice: Always validate incoming patches against your permissions model and sanitize data. Implement thorough logging to track changes for auditing purposes.
Final Considerations
JSON Merge Patch is a powerful technique for efficiently updating JSON documents with minimal data transfer. However, it comes with various pitfalls that developers must navigate to ensure integrity and correctness of the data. By staying aware of array replacement issues, careful handling of null values, type consistency, and the complexity of structures, developers can implement JSON Merge Patch more effectively and securely.
Further Reading
Mastering JSON Merge Patch opens the door to better data handling and efficiency. By learning from common pitfalls, you can avoid them in your applications and build more robust and reliable systems.
Checkout our other articles