Mastering Spring Rest Exception Handling: Common Pitfalls
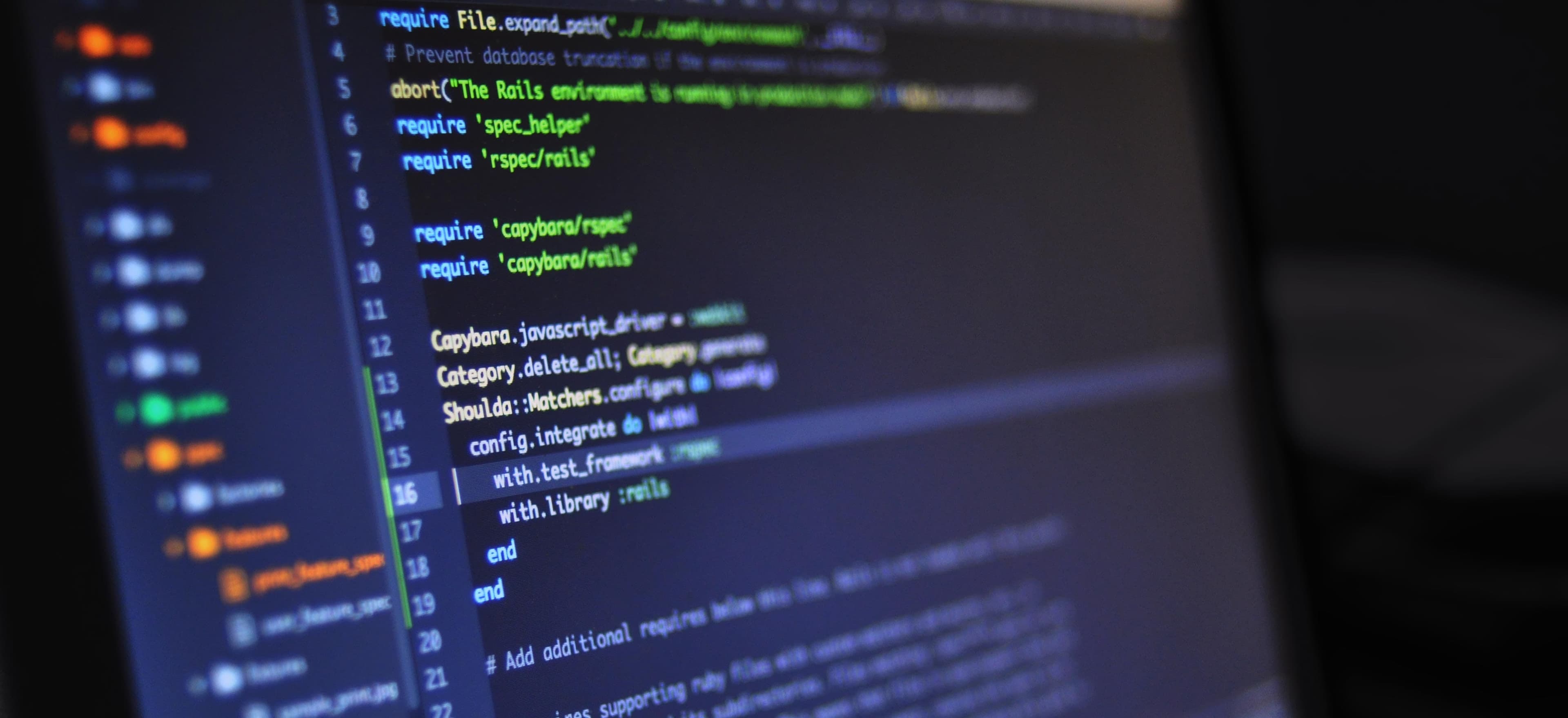
- Published on
Mastering Spring Rest Exception Handling: Common Pitfalls
When building a RESTful application in Spring, one of the most crucial considerations is how to handle exceptions effectively. Properly managing exceptions can lead to better user experience, more maintainable code, and easier debugging. However, many developers encounter pitfalls along the journey of implementing exception handling in their Spring applications. In this post, we will explore some common pitfalls and provide you with insights on how to master exception handling in your Spring REST applications.
Understanding Exception Handling in Spring
Before diving into pitfalls, it's essential to understand the basic components of exception handling in Spring:
- Controller Advice: Using
@ControllerAdvice
, you can create a global exception handler that intercepts exceptions thrown by any controller. - ResponseEntityExceptionHandler: A convenient base class that you can extend to customize the response structure of your exceptions.
- Exception Mappings: Mapping specific exceptions to appropriate HTTP status codes and responses.
With that foundation, let’s explore some common pitfalls.
Pitfall 1: Not Using @ControllerAdvice
The Issue
Many developers forget to use @ControllerAdvice
for global exception handling. Instead, they either handle exceptions locally in each controller or rely on default Spring Boot error responses. This can lead to inconsistent error responses across different parts of your application.
The Solution
Implement a global exception handler using @ControllerAdvice
. This allows you to centralize your error handling.
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFound(ResourceNotFoundException ex) {
ErrorResponse error = new ErrorResponse(HttpStatus.NOT_FOUND.value(), ex.getMessage());
return new ResponseEntity<>(error, HttpStatus.NOT_FOUND);
}
}
Commentary
Using the @ExceptionHandler
annotation allows you to specify which exception types you want this method to handle. In the above example, if a ResourceNotFoundException
is thrown, it will return a JSON response with a 404 status code. Centralizing error handling helps maintain consistency in your API response structure and improves maintainability.
Pitfall 2: Confusing HTTP Status Codes
The Issue
Using incorrect HTTP status codes can confuse clients consuming your API. A common mistake is to return a 200 OK status for error conditions, which does not communicate the failure adequately.
The Solution
Map your exceptions to appropriate HTTP status codes. Use the appropriate status codes for each scenario.
@ExceptionHandler(IllegalArgumentException.class)
public ResponseEntity<ErrorResponse> handleIllegalArgument(IllegalArgumentException ex) {
ErrorResponse error = new ErrorResponse(HttpStatus.BAD_REQUEST.value(), ex.getMessage());
return new ResponseEntity<>(error, HttpStatus.BAD_REQUEST);
}
Commentary
In the example above, we use HttpStatus.BAD_REQUEST
(400) for handling an IllegalArgumentException
. This clearly communicates the issue to the client, making it easier for them to understand the nature of the error.
Pitfall 3: Not Returning a Consistent Error Response Structure
The Issue
Inconsistent error response structures can confuse API consumers and lead to incorrect error handling on their part. Developers may return different formats for errors in different scenarios, which is not advisable.
The Solution
Define a standard error response structure and use it consistently across your application.
public class ErrorResponse {
private int status;
private String message;
public ErrorResponse(int status, String message) {
this.status = status;
this.message = message;
}
// Getters and setters
}
Commentary
The ErrorResponse
class above provides a uniform structure for error responses. A consistent error structure allows API consumers to anticipate what they will receive, reducing confusion and improving integration.
Pitfall 4: Ignoring Runtime Exceptions
The Issue
Developers often overlook runtime exceptions. Uncaught exceptions can lead to server errors (500 Internal Server Error) that do not provide useful information back to the client.
The Solution
Handle general runtime exceptions at the global level.
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleAllExceptions(Exception ex) {
ErrorResponse error = new ErrorResponse(HttpStatus.INTERNAL_SERVER_ERROR.value(), "An error occurred: " + ex.getMessage());
return new ResponseEntity<>(error, HttpStatus.INTERNAL_SERVER_ERROR);
}
Commentary
By handling all exceptions with a general @ExceptionHandler
, you ensure that no exception goes unhandled. However, it's still good practice to log these exceptions, so that you can troubleshoot issues in your application easily.
Pitfall 5: Not Logging Exceptions
The Issue
Failing to log exceptions is another common pitfall. If an exception occurs, but there is no logging in place, it can be challenging to diagnose the cause later.
The Solution
Integrate logging in your exception handlers. Use frameworks like SLF4J or Log4j for effective logging.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@ControllerAdvice
public class GlobalExceptionHandler {
private static final Logger logger = LoggerFactory.getLogger(GlobalExceptionHandler.class);
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleAllExceptions(Exception ex) {
logger.error("An error occurred: ", ex);
ErrorResponse error = new ErrorResponse(HttpStatus.INTERNAL_SERVER_ERROR.value(), "An error occurred: " + ex.getMessage());
return new ResponseEntity<>(error, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Commentary
In the above example, we log the entire stack trace of the exception. This not only helps you understand the errors happening in production but also aids in maintenance and debugging.
Pitfall 6: Not Testing Exception Handling
The Issue
Developers often forget to write tests for exception handling logic. As a result, any changes to the error handling code can inadvertently break the intended behavior.
The Solution
Create a suite of tests specifically for your exception handling logic.
@Test
public void testHandleResourceNotFound() throws Exception {
// Create a mock of the controller that throws ResourceNotFoundException
ResponseEntity<ErrorResponse> response = globalExceptionHandler.handleResourceNotFound(new ResourceNotFoundException("Resource not found"));
assertEquals(HttpStatus.NOT_FOUND, response.getStatusCode());
assertEquals("Resource not found", response.getBody().getMessage());
}
Commentary
Testing your exception handling ensures that the error responses remain correct as your application evolves. In this test, we validate the behavior of our handleResourceNotFound
method to ensure it returns the expected result.
To Wrap Things Up
Mastering exception handling in your Spring REST applications is vital for a robust user experience and effective API design. By avoiding these common pitfalls—using @ControllerAdvice
, correctly mapping HTTP status codes, maintaining a consistent error response structure, logging exceptions, and testing your error handling—you can create a resilient application that communicates clearly with its users.
For further reading, you can explore the official Spring documentation on exception handling and consider diving into best practices for RESTful APIs to enhance your design decisions.
By being aware of these pitfalls and implementing the necessary solutions, you’ll be well on your way to mastering exception handling in your Spring applications. Happy coding!
Checkout our other articles