Overcoming Common Issues in JSP Custom Tag Development
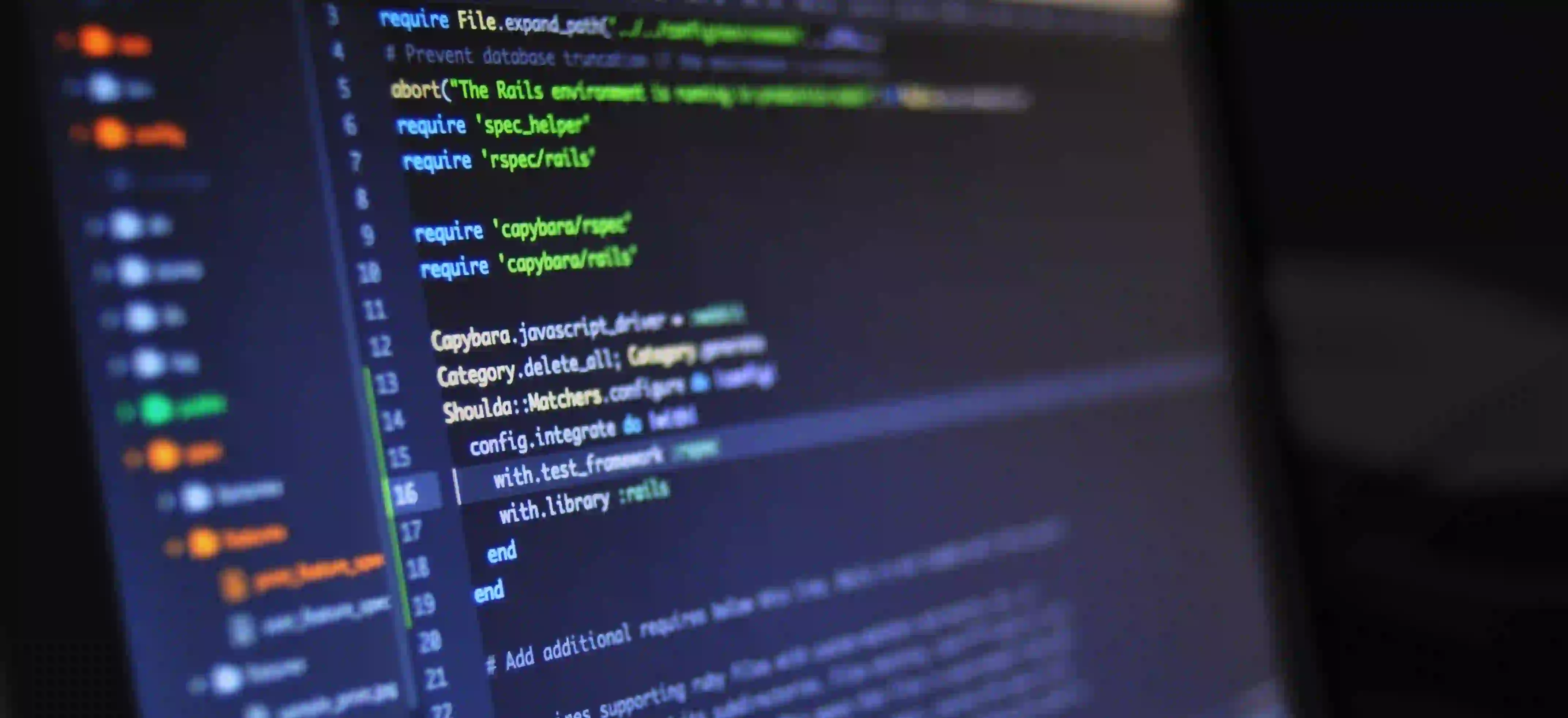
Overcoming Common Issues in JSP Custom Tag Development
JavaServer Pages (JSP) is a powerful technology for creating dynamic web content. One of its standout features is the ability to create custom tags. However, developing these tags can sometimes introduce challenges. In this article, we will explore common issues in JSP custom tag development and how to overcome them effectively.
Understanding JSP Custom Tags
Before diving into the common issues, letβs clarify what JSP custom tags are. Custom tags allow you to encapsulate reusable pieces of code that can be used within your JSP files. They enhance code clarity and maintainability while promoting the DRY (Don't Repeat Yourself) principle.
Here's a simple example of a custom tag definition:
<tag>
<name>helloWorld</name>
<tag-class>com.example.HelloWorldTag</tag-class>
<body-content>empty</body-content>
</tag>
In this definition, we create a custom tag called helloWorld
which is linked to a Java class HelloWorldTag
. This tag can now be used in JSP files as <mytags:helloWorld />
.
Common Issues in JSP Custom Tag Development
-
Incorrect Tag Handler Class Configuration
One of the most frequent issues developers encounter is an incorrectly configured tag handler class. Itβs crucial to ensure that your tag handler class correctly implements the
SimpleTag
orTag
interface, depending on the requirements of your custom tag.Here's a basic implementation using
SimpleTag
:βsnippet.javapackage com.example; import javax.servlet.jsp.tagext.SimpleTagSupport; import java.io.IOException; public class HelloWorldTag extends SimpleTagSupport { @Override public void doTag() throws IOException { getJspContext().getOut().write("Hello World!"); } }
Why: This structure ensures that your tag handler follows the JSP engine's expectations and allows you to define the logic for rendering your custom tag.
-
Classpath Issues
JSP custom tags require the proper inclusion of the tag handler classes in your classpath. If your JSP page cannot find the class, it will lead to a
ClassNotFoundException
.Make sure to:
- Place your compiled classes in the
WEB-INF/classes
directory or package them as a JAR file inWEB-INF/lib
. - Declare your custom tag library in your JSP file accurately.
Example of including the tag library:
πsnippet.txt<%@ taglib prefix="mytags" uri="WEB-INF/tags/mytags.tld" %>
Why: Properly placing and referencing class files ensures that your server can locate and execute your custom tag code when the JSP pages are requested.
- Place your compiled classes in the
-
Null Pointer Exceptions
A common pitfall when using custom tags is failing to correctly pass parameters or set attributes. Misconfigured or uninitialized attributes can lead to
NullPointerException
.Here's an example of a custom tag that takes an attribute:
βsnippet.javapublic class GreetingTag extends SimpleTagSupport { private String userName; public void setUserName(String userName) { this.userName = userName; } @Override public void doTag() throws IOException { if (userName != null && !userName.isEmpty()) { getJspContext().getOut().write("Hello, " + userName + "!"); } else { getJspContext().getOut().write("Hello, Guest!"); } } }
Why: By checking for null or empty values, we prevent the application from crashing and improve the overall user experience.
-
Tag Library Descriptor (TLD) Syntax Errors
The TLD file is vital for registering and using custom tags. Typos or syntax errors will cause the tags not to load, often resulting in confusing error messages.
A correctly formatted TLD file should resemble this:
πsnippet.txt<taglib> <uri>http://example.com/tags</uri> <short-name>mytags</short-name> <tag> <name>greeting</name> <tag-class>com.example.GreetingTag</tag-class> <body-content>empty</body-content> <attribute> <name>userName</name> <required>false</required> </attribute> </tag> </taglib>
Why: Adhering to the correct structure allows the JSP engine to recognize and compile your custom tags without issues.
-
Improper Attribute Declaration
Always define attributes in your tag by creating getter and setter methods. Not declaring an attribute will lead to default values or
null
.For instance, the
userName
must have both set and get methods:βsnippet.javapublic void setUserName(String userName) { this.userName = userName; } public String getUserName() { return userName; }
Why: The JSP engine utilizes these methods to assign and retrieve the attribute values, ensuring proper functionality of your custom tag.
Best Practices in JSP Custom Tag Development
To avoid the most common issues, consider these best practices:
-
Thoroughly Test Your Tags: Always test your tags in different scenarios, especially with edge cases.
-
Use Debugging Logs: Implement logging within your tag handlers to help trace errors during execution.
-
Keep Tags Simple: Aim for a single responsibility principle. A custom tag should ideally do one thing well.
-
Documentation: Document your tags extensively, especially the attributes and return values. This practice aids future maintenance and scalability.
-
Consult the Standards: Familiarize yourself with the JSP 2.0 specification for additional insights into tag development.
Lessons Learned
Developing custom tags in JSP can significantly enhance code reusability and clarity. However, as we've discussed, common issues can arise. By adhering to best practices, following proper configuration guidelines, and being mindful of potential pitfalls, you can create robust and effective custom tags for your Java applications.
Further Reading
- JSP Custom Tags Tutorial
- JavaServer Pages (JSP) Documentation
- Java EE Tutorial
Happy coding!