Mastering Alpha Channel: Solving JavaFX Clipping Issues
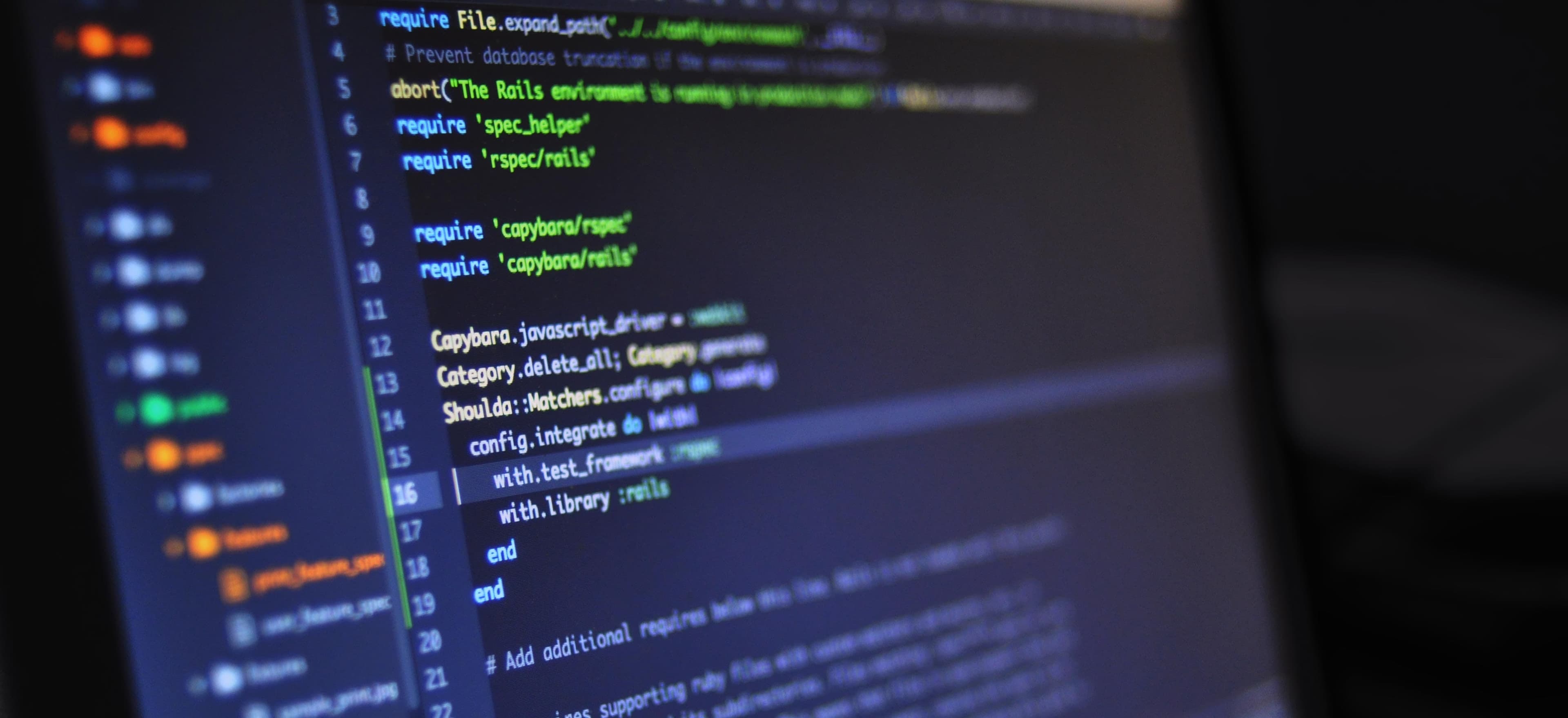
- Published on
Mastering Alpha Channel: Solving JavaFX Clipping Issues
JavaFX offers a robust toolkit for building rich client applications. One of its powerful features is its alpha channel, which allows for transparency and sophisticated visual effects. However, mastering the alpha channel can be tricky, especially when it comes to clipping. In this blog post, we will explore the nuances of alpha channels, delve into common clipping issues in JavaFX, and provide solutions to enhance your application’s visual fidelity.
Understanding Alpha Channels in JavaFX
An alpha channel defines the transparency of a color. In JavaFX, each color can be represented using an RGBA format, where "A" stands for alpha. The alpha value ranges from 0 (completely transparent) to 1 (completely opaque). This feature is crucial for layering visual elements and creating compelling interfaces.
Example of RGBA Representation
In JavaFX, colors can be defined with alpha using the Color
class.
import javafx.scene.paint.Color;
// Creating a semi-transparent red color
Color semiTransparentRed = new Color(1, 0, 0, 0.5); // RGB and Alpha
Here, 0.5
sets the red color to 50% transparency. Understanding this flexibility allows developers to create eye-catching visual effects, but it also introduces complexity, particularly regarding clipping.
What is Clipping in JavaFX?
Clipping is the process of controlling the visible area of a node. It restricts what part of the node is displayed, effectively cropping out the unwanted sections. While this can be visually beneficial, issues can arise when transparency is involved.
How Clipping Works
When clipping, JavaFX creates a mask based on a provided shape, and only the portions of the node inside that shape are rendered. However, if you use transparency opaquely, unintended visual artifacts can emerge.
Example of a Clipping Path
To create a clip in JavaFX, you can use the setClip
method:
import javafx.scene.shape.Circle;
import javafx.scene.Node;
// Example of clipping a node to a circular shape
Node someNode = ...; // Your JavaFX Node (e.g., ImageView)
Circle clipCircle = new Circle(50, 50, 50); // Circle positioned at (50,50) with a radius of 50
someNode.setClip(clipCircle);
In this example, someNode
will only display the area within the circle. While this provides powerful UI capabilities, let's explore common issues and solutions when working with alpha channels and clipping.
Common Clipping Issues in JavaFX
-
Unwanted Artifacts and Edges: Transparent areas might not clip correctly, leading to edges that are visually unattractive.
-
Performance Overheads: Deeply nested nodes or complex shapes can cause performance drainage, especially when alpha is involved.
-
Color Blending Issues: Colors can appear differently depending on the alpha value, leading to inconsistencies in visual outputs.
Solutions to Clipping Issues
1. Properly Manage Node Hierarchy
One simple approach for resolving clipping issues is to ensure your node hierarchy is well-structured. JavaFX renders nodes from the bottom up, meaning transparency can behave differently based on the order of nodes.
Example of Order Importance:
If you are layering UI components, reassess the stacking order.
Pane root = new StackPane();
ImageView imageView = new ImageView("image.png");
Rectangle clipRectangle = new Rectangle(100, 100); // Clipping area
imageView.setClip(clipRectangle);
root.getChildren().addAll(imageView);
Putting imageView
at the bottom ensures it serves as the background, and therefore, it can be clipped properly.
2. Avoid Complex Paths
While complex shapes allow for creativity, they can result in performance issues. Where possible, use simpler shapes for clipping.
Polygon starShape = new Polygon();
starShape.getPoints().addAll(new Double[]{
50.0, 0.0,
61.8, 35.0,
98.0, 35.0,
68.0, 57.0,
79.0, 91.0,
50.0, 70.0,
21.0, 91.0,
32.0, 57.0,
2.0, 35.0,
38.2, 35.0 });
someNode.setClip(starShape);
While the star shape is visually interesting, consider the performance implications before using it.
3. Use Two Layers for Transparent Effects
When blending transparent images or shapes, consider creating a duplicate node—one for the clip and one for the display. This separation reduces clipping artifacts and improves visual quality.
Node displayNode = new ImageView("image.png");
Node clipNode = new Rectangle(100, 100);
displayNode.setClip(clipNode);
Here, clipNode
handles the clipping while displayNode
represents the visual output, reducing the artifact issues we discussed earlier.
4. Add Background Colors Carefully
Ensure that your background colors provide enough contrast. If you are using transparent shapes over a similarly colored background, this can lead to a flat appearance.
Rectangle background = new Rectangle(200, 200, Color.LIGHTGRAY);
Using a contrasting color can change how transparencies blend, making your interface more engaging.
Final Considerations
Mastering the alpha channel in JavaFX can significantly enhance your application's aesthetic appeal. Understanding the implications of clipping and alpha channels is crucial for achieving visually striking interfaces without compromising performance.
Further Learning
- To dive deeper into JavaFX graphics, consider checking out the official JavaFX Documentation.
- For more advanced clipping techniques, review this JavaFX Clipping Tutorial.
- Investigating Shapes and Paths in JavaFX can also provide valuable insights into demonstrating complex graphics.
By honing your skills in managing alpha channels and clipping, you will enhance not only the functionality but also the artistic flair of your applications. Happy coding!
Checkout our other articles