Mastering SQL Aggregations in Java 8: Common Pitfalls
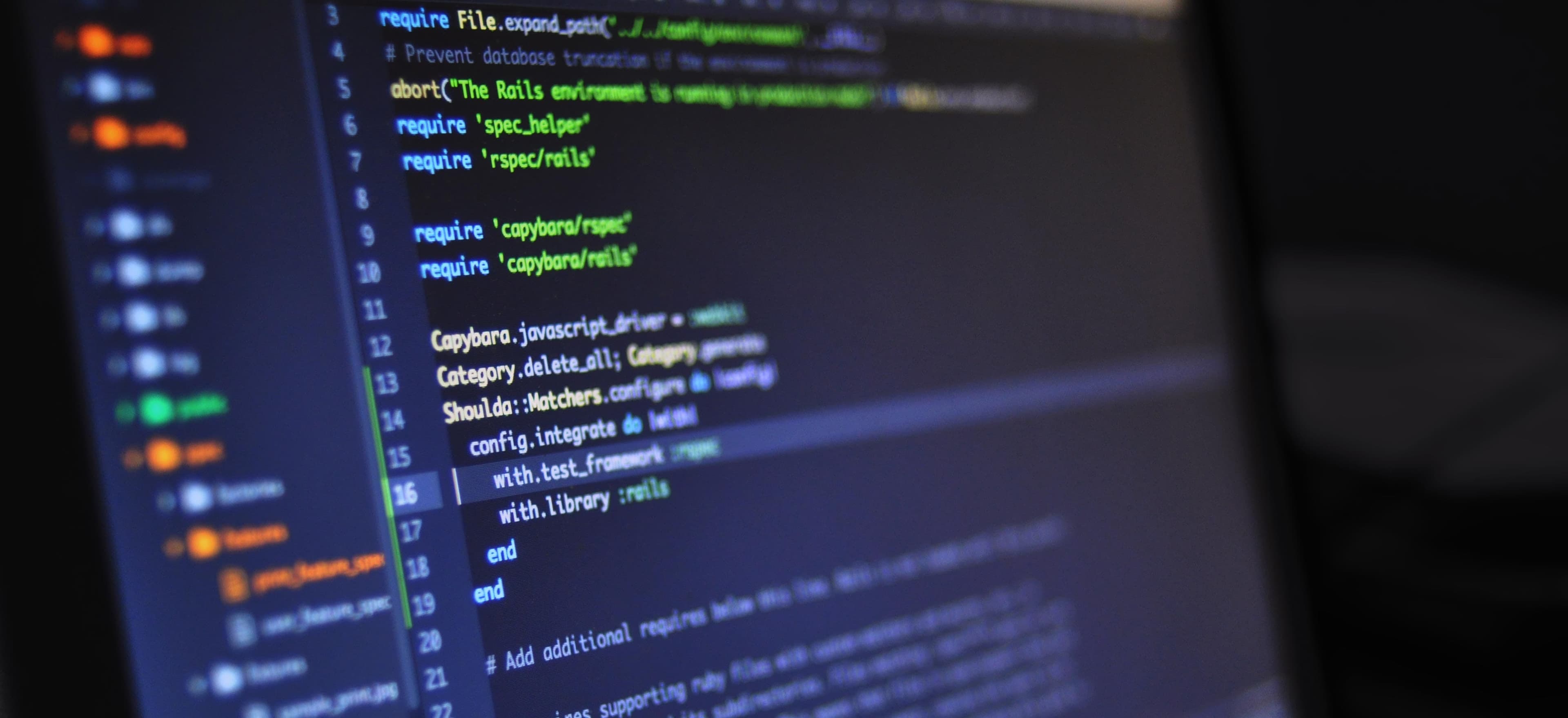
- Published on
Mastering SQL Aggregations in Java 8: Common Pitfalls
SQL aggregations are a fundamental part of database management, allowing developers to summarize and analyze data effectively. With the introduction of Java 8, handling data using the Stream API has become more intuitive, but it also comes with its own set of hurdles. In this blog post, we'll dive deep into SQL aggregations in the context of Java 8 while exploring common pitfalls developers face and how to tackle them.
What is SQL Aggregation?
SQL aggregation functions are used to compute a single result value from a set of input values. Common aggregation functions include:
- COUNT: Counts the number of rows.
- SUM: Sums up the numeric data of a column.
- AVG: Calculates the average value of a numeric column.
- MAX: Gets the maximum value in a column.
- MIN: Retrieves the minimum value in a column.
In Java 8, we use the Stream API to perform similar operations on collections. But with power comes complexity. Let's explore some of the common pitfalls to avoid.
Common Pitfalls in SQL Aggregations with Java 8
1. Forgetting to Use Terminal Operations
One of the most common mistakes developers make is neglecting to call a terminal operation on a stream. Streams in Java are lazy; meaning if you don't execute a terminal operation, nothing happens.
Here's an example:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
Stream<Integer> numberStream = numbers.stream().map(n -> n * 2);
// Forgot to use a terminal operation
Why: It’s crucial to remember that without a terminal operation like forEach()
, collect()
, or reduce()
, the stream does not execute any operations.
Correct Implementation:
List<Integer> result = numberStream.collect(Collectors.toList());
System.out.println(result); // Outputs: [2, 4, 6, 8, 10]
2. Not Handling Null Values
Null values can cause unexpected results when working with aggregation functions. For instance, consider you are calculating the average of a list that contains null values.
List<Integer> values = Arrays.asList(1, 2, null, 4, 5);
Double average = values.stream()
.mapToInt(val -> val == null ? 0 : val) // Handling null values
.average() // Averages the values
.orElse(0.0);
System.out.println(average); // Outputs: 2.5
Why: By replacing nulls with default values or skipping them, we ensure the aggregation functions behave as expected.
3. Misunderstanding Grouping Operations
When performing grouping operations, developers often overlook how to collect results. Using the Collectors.groupingBy()
collector can aggregate data based on certain criteria. Here's an example:
List<Person> people = Arrays.asList(
new Person("John", 25),
new Person("Jane", 35),
new Person("John", 30)
);
Map<String, List<Person>> groupedByName = people.stream()
.collect(Collectors.groupingBy(Person::getName));
System.out.println(groupedByName);
// Outputs: {John=[Person{name='John', age=25}, Person{name='John', age=30}], Jane=[Person{name='Jane', age=35}]}
Why: Grouping without specifying how to handle resultant values (e.g., summing ages) can lead to unstructured data.
4. Inefficient Use of Collectors
Understanding how to use different collectors effectively can increase the performance of your aggregation. For instance, using Collectors.toMap()
can efficiently transform a list into a map.
Map<String, Integer> nameToAge = people.stream()
.collect(Collectors.toMap(
Person::getName,
Person::getAge,
Integer::sum // In case of duplicates, sum their ages
));
System.out.println(nameToAge); // Outputs: {John=55, Jane=35}
Why: Clearly defining duplicate handling (such as summing or finding max/min) is crucial for maintaining data integrity.
5. Mixing Stream and Collection Operations
Sometimes, developers may forget that streams are different from collections. They cannot be reused. Each stream can only be traversed once.
Stream<Integer> numbersStream = numbers.stream();
numbersStream.forEach(System.out::println);
// Attempting to reuse the stream will throw an exception
numbersStream.forEach(System.out::println); // IllegalStateException: stream has already been operated upon or closed
Why: A stream must be created anew if you wish to iterate again. This distinction is paramount for optimal performance.
Best Practices for SQL Aggregations in Java 8
1. Embrace Immutability
When possible, leverage immutable collections. This helps prevent state-related bugs and promotes more predictable behavior.
2. Utilize Optional Class Effectively
Use Optional
to avoid dealing with nullable values directly, streamlining your aggregations and enhancing readability.
Optional<Double> maxAge = people.stream()
.map(Person::getAge)
.reduce(Math::max);
// Handle missing values
maxAge.ifPresent(age -> System.out.println("Max Age: " + age)); // Outputs: Max Age: 35
3. Document Your Code
Always leave comments explaining why certain decisions were made, especially when it comes to handling edge cases in your aggregations.
4. Benchmarking and Profiling
Before deploying your application, ensure that you benchmark different approaches for performance. Sometimes, optimizing the way you aggregate can significantly improve efficiency.
Final Thoughts
Understanding SQL aggregations through the lens of Java 8 can greatly enhance your data manipulation capabilities. By avoiding common pitfalls, you can leverage the power of the Stream API to perform insightful data analysis while maintaining code quality.
For further reading, consider looking into Java 8's Stream API and Collectors. With practice and diligence, mastering SQL aggregations in Java 8 will become second nature.
Happy coding!
Checkout our other articles