Mastering Android's Basic Structure: Common Rookie Mistakes
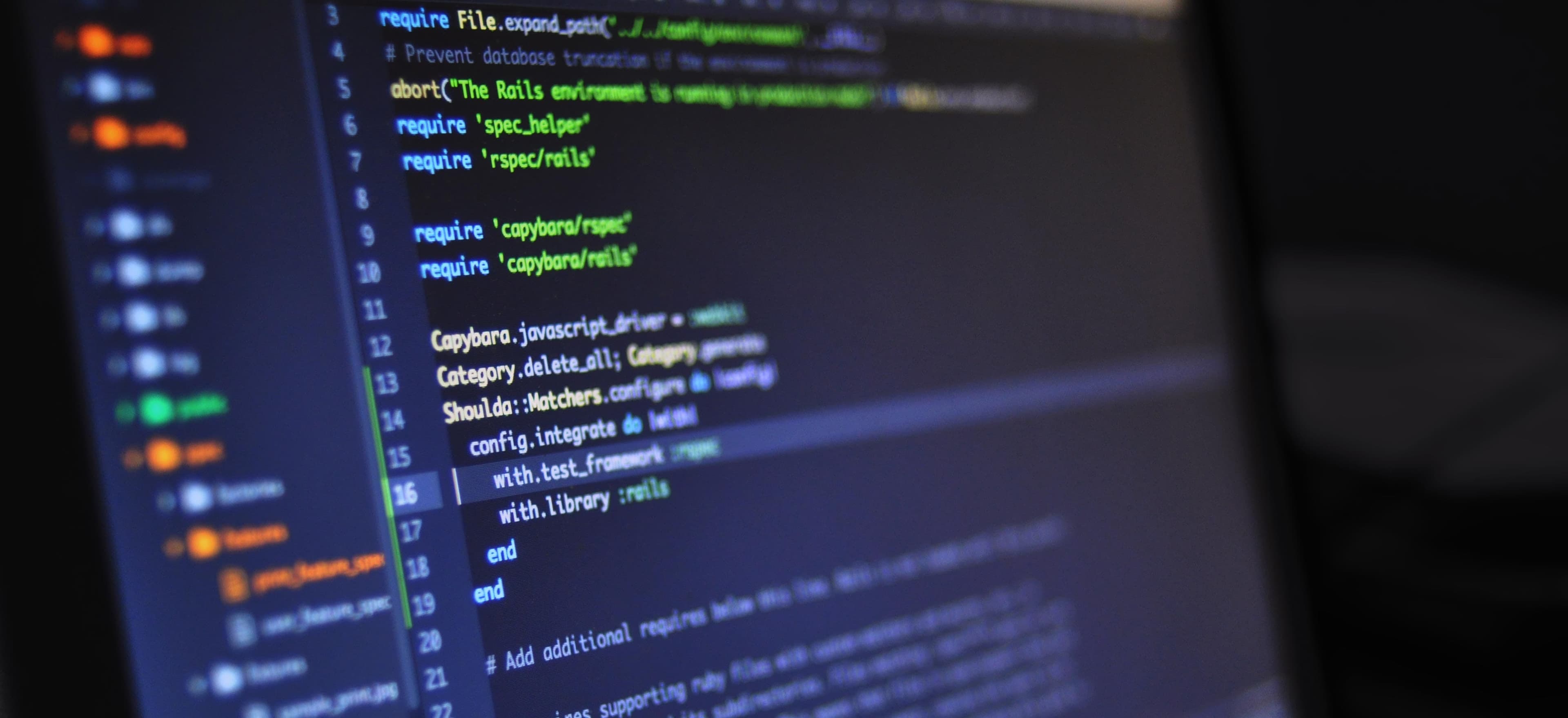
- Published on
Mastering Android's Basic Structure: Common Rookie Mistakes
When diving into Android development, many newcomers stumble over the same foundational concepts. Understanding the basic structure of Android applications is crucial for building efficient and effective apps. This blog post will outline some of the common rookie mistakes developers make when they start with Android, along with tips and code examples to ensure you don’t end up in the same boat.
Understanding the Android Application Structure
Before we delve into the common mistakes, let's quickly review the architecture of an Android app. An Android application is primarily composed of Activities, Fragments, Services, Content Providers, and Broadcast Receivers.
- Activities: The entry point for users; they represent a single screen.
- Fragments: Modular sections of an Activity, allowing for UI reusability.
- Services: Background processes that don't interact directly with UI.
- Content Providers: Facilitate data sharing between applications.
- Broadcast Receivers: Listen for system-wide broadcast announcements.
Now, let’s look at the common pitfalls associated with these components.
Common Rookie Mistakes
1. Misusing Activity Lifecycle
One of the most frequent mistakes is misunderstanding the Activity lifecycle. Each Activity goes through states like onCreate
, onStart
, onResume
, and others that need to be correctly managed.
Example Mistake
Here’s a common mistake:
@Override
protected void onStart() {
super.onStart();
// Starting a network call here
fetchData();
}
Why is this a mistake?
Starting long-running operations in onStart
can lead to performance issues, especially if the Activity's lifecycle changes, such as being paused or stopped.
Best Practice
Perform heavy operations in onCreate
or onResume
, and manage them effectively in onPause
or onStop
. This helps in ensuring your app remains responsive.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Fetch data in a background thread
new Thread(() -> fetchData()).start();
}
2. Ignoring UI Thread Management
Another rookie mistake is performing UI updates on background threads, which can crash the app.
Example Mistake
// Wrong: This is unsafe
new Thread(() -> {
// Fetch data
updateUi(someData); // Called on background thread
}).start();
Why is this a mistake?
Android requires that UI updates occur on the main thread. Failing to do so can lead to CalledFromWrongThreadException
.
Best Practice
Use runOnUiThread
or better yet, use AsyncTask
or LiveData
. Here's an updated version:
new Thread(() -> {
// Fetch data
runOnUiThread(() -> updateUi(someData));
}).start();
3. Hardcoding Strings
Hardcoding strings can lead to localized issues as your audience grows. It’s a common oversight that can compound maintenance difficulties.
Example Mistake
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Welcome to My App!" /> <!-- Hardcoded string -->
Why is this a mistake? Hardcoded strings cannot be easily translated, making localization efforts tedious.
Best Practice
Always use string resources. Update the previous example to:
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/welcome_message" /> <!-- Reference string resource -->
Make sure to define welcome_message
in res/values/strings.xml
:
<resources>
<string name="welcome_message">Welcome to My App!</string>
</resources>
4. Not Following MVVM Architecture
A common rookie mistake is neglecting proper architectural patterns like MVVM (Model-View-ViewModel).
Example Mistake
Developers often put business logic inside Activities, leading to tight coupling.
public class MainActivity extends AppCompatActivity {
// Business logic here
}
Why is this a mistake? This makes unit testing difficult and harms maintainability.
Best Practice
Separate your concerns. Use ViewModels for the presentation logic.
public class MainViewModel extends ViewModel {
// Business logic here
}
Now the MainActivity
can just observe changes:
MainViewModel viewModel = new ViewModelProvider(this).get(MainViewModel.class);
viewModel.getData().observe(this, data -> updateUi(data));
5. Overusing Memory with Static References
Rookie developers might be unaware of memory leaks caused by static references, particularly to Activities or Views.
Example Mistake
public class MyApplication extends Application {
private static MainActivity mainActivity;
// Static reference holding the Activity
}
Why is this a mistake? Static references can prevent garbage collection of Activities, leading to memory leaks.
Best Practice
Use WeakReference
for maintaining references when necessary.
public class MyApplication extends Application {
private static WeakReference<MainActivity> mainActivityRef;
}
6. Skipping Gradle Optimizations
Failing to optimize your Gradle files is another common error seen in rookie projects.
Example Mistake
implementation 'com.squareup.retrofit2:retrofit:2.9.0' // Include individual dependencies
Why is this a mistake? Over time, Gradle can slow down builds if not managed correctly.
Best Practice
Use Gradle’s dependency management features effectively.
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0' // Group dependencies
Organizing your dependencies can help manage the complexity of your project.
A Final Look
Ensuring a solid grasp on Android’s basic structure will go a long way in avoiding common pitfalls. Avoid these rookie mistakes, and you will not only build better applications but also gain greater confidence as a developer.
For more comprehensive insights, check out the official Android Developer Documentation. Understanding how to effectively manage the Android application structure and lifecycle will enable you to create responsive and maintainable apps.
By following these tips and best practices, you can pave the way for a smoother development experience and a more robust application. Happy coding!