Common Mistakes When Releasing Maven to Nexus
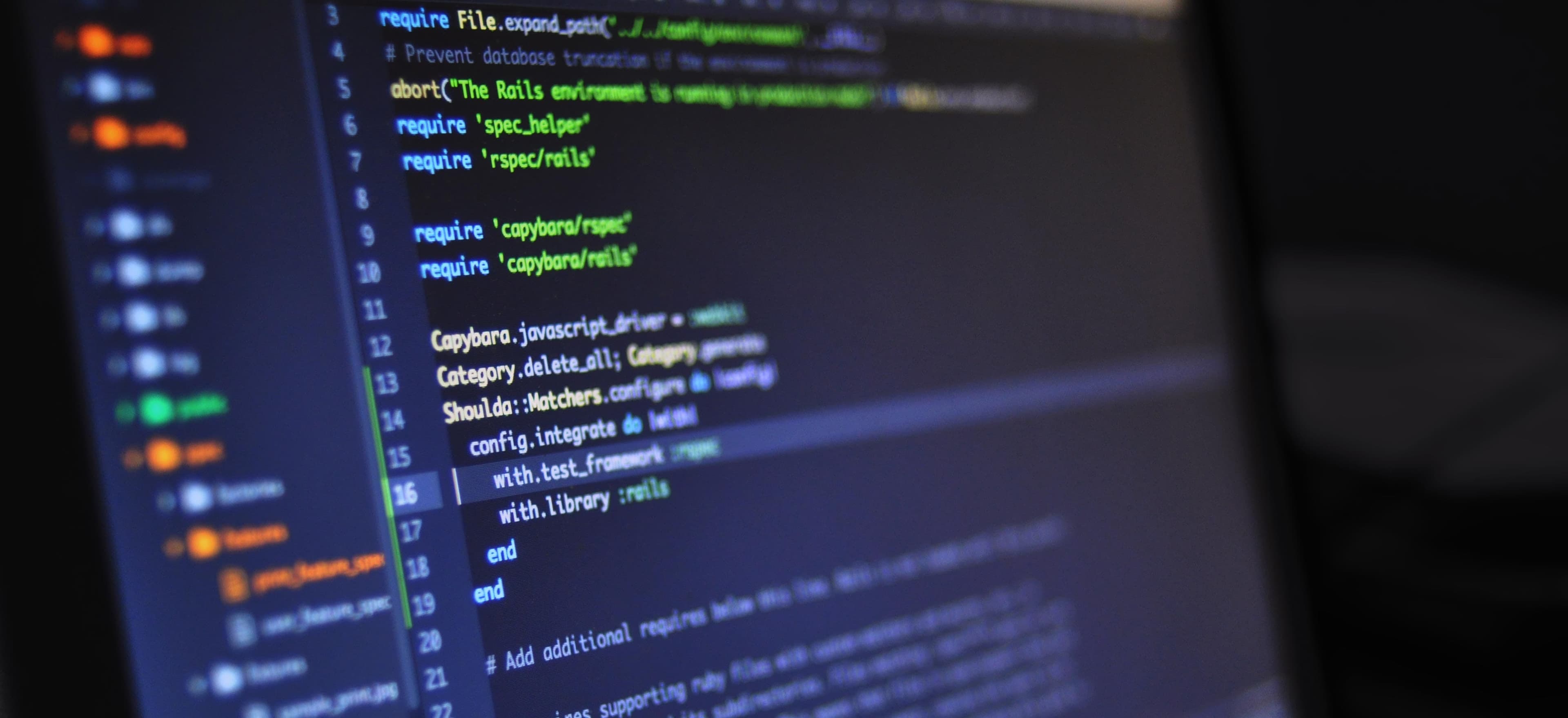
- Published on
Common Mistakes When Releasing Maven to Nexus
Releasing a Maven artifact to a Nexus repository can sometimes feel like a daunting task, especially for those who are relatively new to the Java ecosystem. Few things are as satisfying as successfully deploying a library or module that you’ve painstakingly crafted, only to find that it didn’t go smoothly due to minor missteps. This post discusses common pitfalls and mistakes developers make during the Maven release process and how to avoid them.
Understanding Maven and Nexus
Before diving into the common mistakes, let's clarify what Maven and Nexus are.
Maven is a build automation tool used primarily for Java projects. It simplifies the build process, dependency management, and project configuration.
Nexus is a repository manager that allows developers to manage their software artifacts. It provides a storage solution for artifacts produced by the build process.
Mistake 1: Misconfigured Settings.xml
One of the most frequent issues arises from a misconfigured settings.xml
file. This XML file is critical as it defines repository configurations, proxy settings, server authentication, and more.
Example
<servers>
<server>
<id>nexus-releases</id>
<username>your-username</username>
<password>your-password</password>
</server>
</servers>
Why It Matters
Inaccurate entries here can prevent Maven from uploading artifacts to your Nexus repository. Always ensure that the <id>
corresponds perfectly with the server setting in the Maven pom.xml
. Any typos or mismatches will result in authentication failures.
Mistake 2: Incorrect Versioning
Maven relies heavily on versioning. If you forget to increment the version number before a release, you may face issues.
Example
In your pom.xml
, you have:
<version>1.0.0</version>
If you try to release this version again without changing it, Nexus will reject your request since it already exists.
Why It Matters
Versioning enables better dependency management, avoiding jar hell
. Always make sure to increment your version using either semantic versioning (e.g., from 1.0.0 to 1.1.0) or following the appropriate versioning strategy for your team.
Mistake 3: Not Using the Release Plugin
While many developers try to automate the release process, some overlook the Maven Release Plugin. Failing to use this tool might lead to an inconsistent state in your repository.
Example Command
mvn release:prepare release:perform
Why It Matters
Using the Release Plugin automatically performs necessary actions like tagging the version in your version control and updating your pom.xml
with the new version. It encapsulates everything into a single process that is less error-prone.
Mistake 4: Release Without Testing
In the rush to publish new features or fixes, developers often skip crucial testing steps before the release.
Solution
Make it a best practice to run your entire test suite before releasing. You can also leverage:
mvn clean install
Why It Matters
Running tests ensures that your code meets quality standards and doesn't introduce new bugs. Releases should only go out if they are reliable and perform as expected.
Mistake 5: Ignoring Dependency Management
Not managing dependencies properly can create headaches. It is vital to ensure that all required dependencies are present and correctly defined.
Example
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>example-artifact</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
Why It Matters
Incorrect or missing dependencies can lead to runtime failures or class not found exceptions when the artifact is used in other projects. Always ensure that your dependencies are properly referenced and are the correct versions.
Mistake 6: Forgetting Sonatype Staging Profile
If you're using Sonatype’s OSS Nexus, many developers will accidentally skip the staging profile, which is essential for pushing artifacts to the central repository.
Example Steps
- Configure your
pom.xml
to include the<distributionManagement>
section with the correct URLs.
<distributionManagement>
<repository>
<id>sonatype-nexus-staging</id>
<url>https://oss.sonatype.org/repository/staging/</url>
</repository>
</distributionManagement>
- Run:
mvn clean deploy
Why It Matters
Skipping the staging repository might lead to artifacts not being available in the central repository after deployment. Always validate that you follow the necessary steps if you’re using a service like Sonatype.
Mistake 7: Configuring Proxy Settings
Proxy settings can be troublesome, especially in corporate environments. If your network config requires a proxy and it’s not set correctly, you won’t be able to connect to Nexus.
Example Configuration
<proxies>
<proxy>
<id>example-proxy</id>
<active>true</active>
<protocol>http</protocol>
<host>proxy.yourcompany.com</host>
<port>8080</port>
</proxy>
</proxies>
Why It Matters
Without the proper proxy settings, Maven cannot access external repositories or Nexus, thus making it impossible to complete any download or upload actions.
Final Thoughts
The journey of releasing a Maven artifact to Nexus doesn't need to be littered with common mistakes. By avoiding configuration errors, while embracing proper versioning and using essential plugins, you can streamline your deployments.
Always remember:
- Review your
settings.xml
carefully. - Increment your version after each release.
- Utilize the Maven Release Plugin for a smoother process.
- Rigorously test your code.
- Properly manage your dependencies.
By keeping these considerations in mind, you'll find the release process to be both rewarding and efficient. Happy coding!
For more in-depth guides and documentation, please check:
- Maven Official Documentation
- Sonatype Nexus Repository Documentation
Feel free to share your experiences or pitfalls you've encountered in your Maven and Nexus journey.
Checkout our other articles