Unlocking the Mystery of Java Wrapper Class Limitations
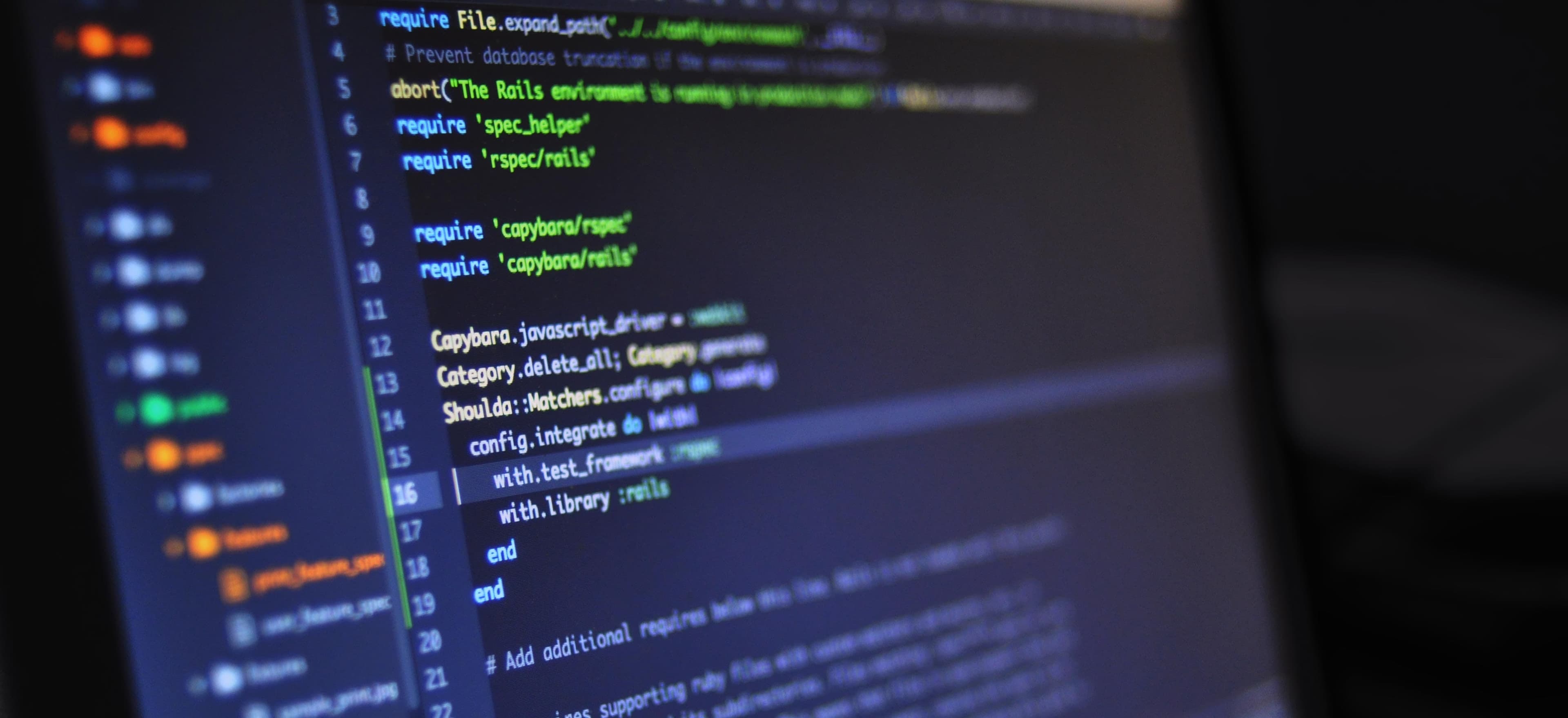
- Published on
Unlocking the Mystery of Java Wrapper Class Limitations
Java, as an object-oriented programming language, provides a variety of features to enhance the robustness and flexibility of your applications. One such feature is the use of wrapper classes. These classes allow you to convert primitive data types into objects, enabling additional functionalities. However, while wrapper classes are useful in many scenarios, they also come with limitations. In this blog post, we will explore the Java wrapper classes, their significance, and their inherent limitations.
What Are Java Wrapper Classes?
In Java, every primitive data type has a corresponding wrapper class. Here’s a quick overview:
- byte: Byte
- short: Short
- int: Integer
- long: Long
- float: Float
- double: Double
- char: Character
- boolean: Boolean
These wrapper classes are located in the java.lang
package. The primary purpose of these classes is to provide a way to use primitive data types as objects. This is essential for working with Java's Collection framework, which only accepts objects, not primitive types.
Example of Wrapper Classes
Integer intValue = new Integer(42);
Double doubleValue = new Double(3.14);
Boolean booleanValue = new Boolean(true);
In the above example, we create instances of the wrapper classes Integer
, Double
, and Boolean
. Each instance wraps a primitive data type into an object.
Benefits of Using Wrapper Classes
Though wrapper classes have limitations, their advantages cannot be overlooked:
-
Object Manipulation: You can treat primitive types as objects, which is particularly useful in scenarios like collections.
-
Utility Methods: Each wrapper class provides utility methods for converting between types and manipulating values. For instance, Integer class provides methods like
parseInt()
,toString()
, etc. -
Null Values: Wrapper classes can hold null values, which is beneficial when working with databases, as you can differentiate between an uninitialized variable and one that has been set to a primitive value.
Limitations of Java Wrapper Classes
While wrapper classes extend functionalities, they come with certain drawbacks. Let's break down some common limitations.
1. Performance Overhead
Creating instances of wrapper classes incurs a performance penalty compared to working with primitives directly. This is due to object creation and garbage collection.
Example
Consider a scenario where you need to sum an array of integers:
int[] primitiveArray = {1, 2, 3, 4, 5};
int sum = 0;
for (int num : primitiveArray) {
sum += num; // Performance is higher
}
In contrast, using integers as wrapper objects might look similar but will be slower:
Integer[] integerArray = {1, 2, 3, 4, 5};
int sum = 0;
for (Integer num : integerArray) {
sum += num.intValue(); // Slower due to method call and boxing
}
2. Immutability
Wrapper classes in Java are immutable. This means once they are created, their values cannot be changed. Any operation that seems to alter a wrapper object will actually generate a new instance.
Example
Integer original = 5;
original++; // This does not modify original
System.out.println(original); // Still prints: 5
In this case, original
remains 5 after the increment operation due to immutability. This might lead to unintentional bugs if you rely on modifying the original values.
3. NullPointerExceptions
Since wrapper classes can hold null values, you may encounter NullPointerExceptions
if you try to access a method on a null wrapper object.
Example
Integer value = null;
int result = value + 1; // This throws NullPointerException
Such issues can be frustrating as they can arise during runtime, making debugging challenging.
4. Boxing and Unboxing
When using wrapper classes, you often perform boxing (converting a primitive to a wrapper) and unboxing (converting a wrapper back to a primitive). While Java does provide auto-boxing and unboxing, it can lead to performance inefficiencies when used excessively.
Example
Integer boxedValue = 10; // Auto-boxing
int primitiveValue = boxedValue; // Auto-unboxing
Even though Java handles these operations surprisingly well, frequent boxing and unboxing can contribute to unnecessary object creation and affect performance.
Best Practices When Using Wrapper Classes
To maximize efficiency and mitigate issues related to wrapper classes, consider the following best practices:
-
Use Primitives When Possible: For calculations and operations where null handling is not needed, leverage primitive types over wrapper classes.
-
Utilize Collections Wisely: When using collections that require objects, minimize the use of wrapper classes if they don’t require object-level methods or handling null.
-
Check for Null: When using wrapper classes, always check for null before performing operations to avoid
NullPointerExceptions
. -
Leverage Optional Class: Java 8 introduced the
Optional
class which offers a more graceful way to handle nullable values by avoiding direct null checks.
Example of Using Optional
Optional<Integer> optionalValue = Optional.ofNullable(null);
optionalValue.ifPresent(System.out::println); // Safe handling of optional value
Final Considerations
Java wrapper classes play a crucial role in bridging the gap between primitivism and object-oriented programming paradigms. They provide versatility, especially when working with data structures. However, it’s essential to understand their limitations, particularly related to performance, immutability, null handling, and the boxing/unboxing mechanism.
By following best practices, you can harness the power of wrapper classes in Java while minimizing potential pitfalls. If you want to dive deeper into Java best practices and features, check out the official Java documentation.
Happy coding!
Checkout our other articles