Simplifying Java-Based Spring Configurations for Better Clarity
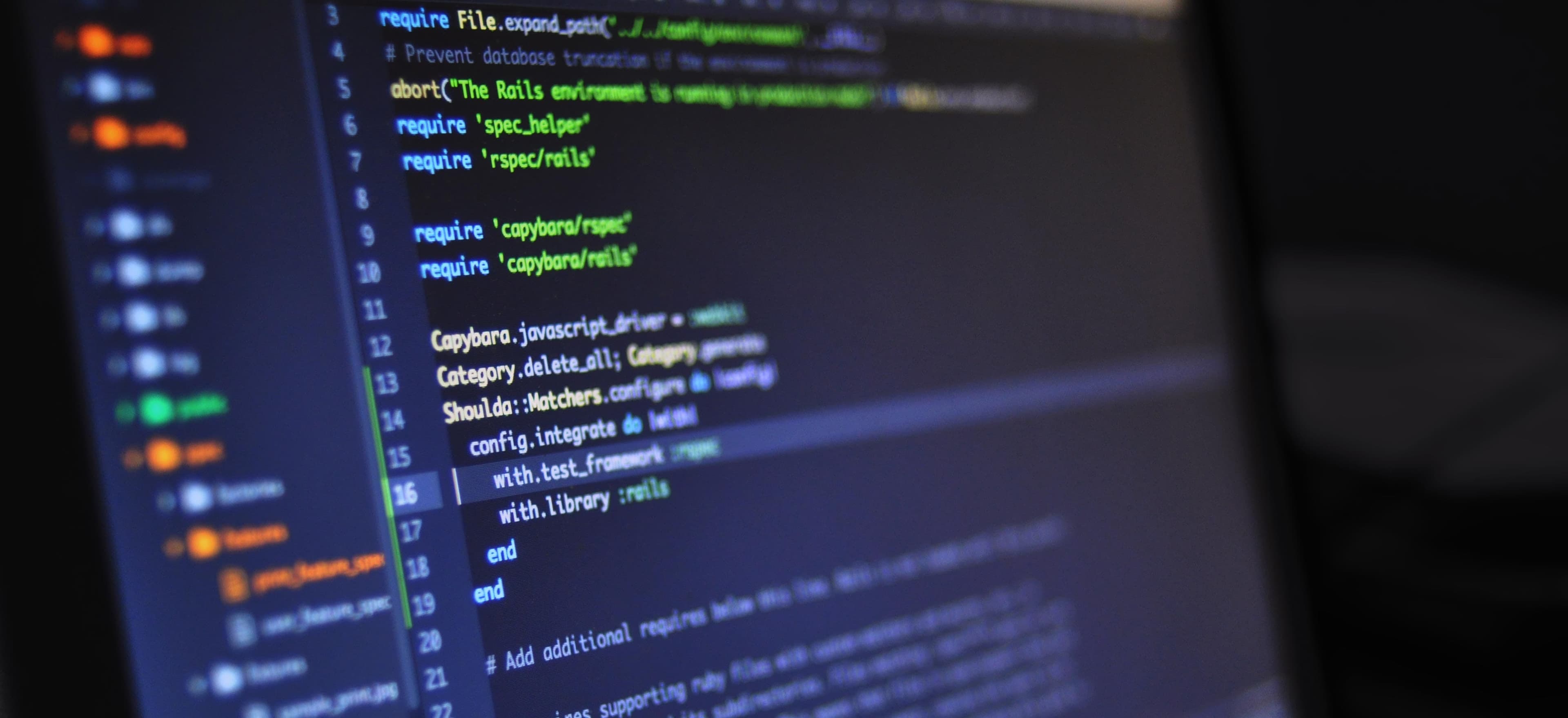
- Published on
Simplifying Java-Based Spring Configurations for Better Clarity
Java-based Spring configurations can often appear overwhelming, especially for beginners stepping into the world of Spring Framework. The sheer volume of settings, options, and configurations can lead to confusion, making it challenging to maintain or update applications. This blog post aims to demystify Java-based Spring configurations by simplifying them and providing clear, actionable insights.
What is Spring Configuration?
Spring Framework, renowned for its robustness, offers various ways to configure applications. Historically, developers turned to XML configurations. However, with Java-based configuration introduced in Spring 3.0, a more intuitive, type-safe approach emerged.
Why Use Java-Based Configuration?
Java-based configuration provides several benefits:
- Type Safety: Compile-time checking helps catch errors early.
- Refactoring Support: IDE features such as auto-complete make refactoring easier.
- Reduced Boilerplate: Eliminates the verbose XML syntax used in traditional configurations.
However, with great power comes great responsibility. Mismanagement of configurations can lead to a convoluted spaghetti code structure. Therefore, organizing your configurations effectively is crucial.
Getting Started with Java-Based Configuration
Let's consider a simple example where we will create a Spring application with services and a repository.
Step 1: Basic Setup
Ensure that you have the following dependencies in your pom.xml
if you're using Maven:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.19</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.3.19</version>
</dependency>
</dependencies>
Step 2: Create a Simple Domain Model
Let's say you are building a simple application to manage books. A Book
entity can look like this:
// Book.java
public class Book {
private String title;
private String author;
public Book(String title, String author) {
this.title = title;
this.author = author;
}
// Getters and Setters
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
}
Step 3: Create a Book Repository
Now we need a repository to handle our book data:
// BookRepository.java
import java.util.ArrayList;
import java.util.List;
public class BookRepository {
private List<Book> books = new ArrayList<>();
public void addBook(Book book) {
books.add(book);
}
public List<Book> getAllBooks() {
return books;
}
}
Step 4: Create a Service for Business Logic
The service layer processes the data and communicates with the repository:
// BookService.java
import org.springframework.stereotype.Service;
@Service
public class BookService {
private final BookRepository bookRepository;
public BookService(BookRepository bookRepository) {
this.bookRepository = bookRepository;
}
public void addBook(String title, String author) {
Book book = new Book(title, author);
bookRepository.addBook(book);
}
public List<Book> listBooks() {
return bookRepository.getAllBooks();
}
}
Step 5: Java-Based Spring Configuration
With the model, repository, and service in place, we can now configure Spring to wire everything together.
// AppConfig.java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan(basePackages = "com.example") // Adjust accordingly
public class AppConfig {
@Bean
public BookRepository bookRepository() {
return new BookRepository();
}
}
Here, we use the @Configuration
annotation to define our configuration class. The @ComponentScan
annotation allows Spring to discover and register components. We also explicitly declare a BookRepository
bean, which is sufficient for simple applications.
Step 6: Bootstrap the Application
Now let's set up a main application class to run the Spring context:
// MainApplication.java
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class MainApplication {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
BookService bookService = context.getBean(BookService.class);
bookService.addBook("1984", "George Orwell");
bookService.listBooks().forEach(book -> System.out.println(book.getTitle() + " by " + book.getAuthor()));
}
}
This application initializes the Spring context based on your AppConfig
class and retrieves the BookService
bean. If you run this application, you will get the output for the books managed by your service.
Best Practices for Simplifying Java-Based Configurations
1. Use Component Scanning Wisely
Instead of declaring every bean explicitly, leverage annotations like @Service
, @Repository
, and @Controller
. This reduces boilerplate code significantly.
2. Modularize Your Configurations
If your application grows, consider breaking your configuration into manageable parts using multiple configuration classes. For example:
// DatabaseConfig.java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class DatabaseConfig {
@Bean
public DataSource dataSource() {
// Configure and return the DataSource
}
}
// ServiceConfig.java
import org.springframework.context.annotation.Configuration;
@Configuration
public class ServiceConfig {
// Service Beans
}
This not only adds clarity but also aids maintenance.
3. Leverage Profiles
Use Spring's profiles to separate configurations based on different environments (development, testing, production). This can minimize human error and enhance clarity:
import org.springframework.context.annotation.Profile;
@Configuration
@Profile("dev")
public class DevConfig {
// Dev-specific beans
}
4. Documentation and Comments
Always document your configuration classes to explain the purpose and usage of beans. Comments can be especially crucial for future maintainability.
5. Review Your Dependencies
Regularly review and audit your dependencies in the pom.xml
. This prevents unnecessary clutter and potential conflicts.
Closing Remarks
Java-based Spring configurations, while potent, can sometimes lead to confusion. However, simplifying configurations through best practices—such as effective component scanning, modularization, and using profiles—can make development smoother and more intuitive.
By following the examples and recommendations in this post, your Java Spring configurations will not only maintain clarity but also foster a better coding experience. You can further enhance your understanding of Spring configurations by exploring official Spring documentation and Spring Framework in Action, which provide deeper insights and real-world scenarios.
Embrace the power of Spring, and let clarity be your guiding principle! Happy coding!
Checkout our other articles