Mastering Java Serialization: Common Pitfalls Explained
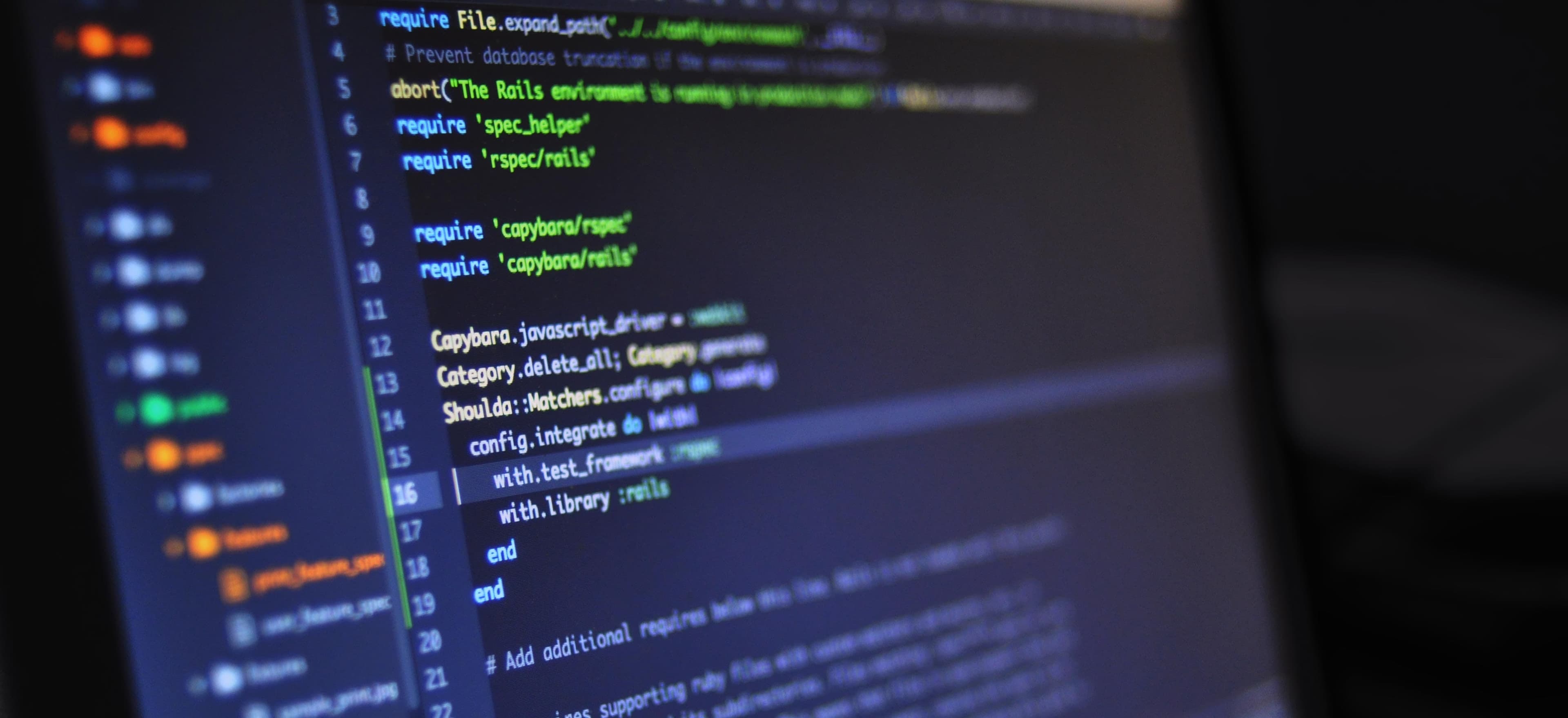
- Published on
Mastering Java Serialization: Common Pitfalls Explained
Java serialization is a crucial aspect of Java programming, especially when it comes to object persistence, remote communication, and frameworks like RMI (Remote Method Invocation). While it seems straightforward, many developers stumble upon common pitfalls. In this blog post, we will explore what serialization is, its importance, and the typical mistakes developers make, along with code snippets and best practices.
What is Serialization?
Serialization is the process of converting an object into a byte stream, allowing the object to be easily saved to a file or transmitted over the network. Deserialization is the reverse process, where you convert the byte stream back into a copy of the object.
Why is Serialization Important?
Serialization is particularly important for:
- Persistence: Saving the state of an object to a file so that it can be read later.
- Communication: Sending objects over the network in distributed applications.
- Caching: Storing objects for quick retrieval.
To perform serialization in Java, a class must implement the java.io.Serializable
interface. Let's look at a simple example.
import java.io.*;
public class Employee implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private transient String password; // A field we don't want to serialize
public Employee(String name, String password) {
this.name = name;
this.password = password;
}
// Getters and toString() method omitted for brevity
}
In this Employee
class, we marked the password
field as transient
to prevent it from being serialized. This is critical for security reasons, as sensitive information should never be transmitted or stored in an unencrypted format.
Common Pitfalls with Java Serialization
Despite its utility, serialization can lead to a range of errors if not handled carefully. Let's explore some of the most common pitfalls.
1. Not Defining serialVersionUID
One of the most common mistakes is failing to declare a serialVersionUID
. This unique identifier helps ensure the compatibility of serialized objects.
private static final long serialVersionUID = 1L;
If you do not specify a serialVersionUID
, Java will generate one at runtime based on various class attributes. However, changes such as adding new fields or methods can alter the default UID and lead to InvalidClassException
during deserialization. Always define this ID explicitly to maintain compatibility across different versions of your class.
2. Failing to Handle Transient Fields
Transient fields are not serialized, which can lead to loss of critical information when the object is deserialized. Consider this example:
import java.io.*;
class User implements Serializable {
private String username;
private transient String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Getters and toString() method omitted for brevity
}
Here, if we attempt to serialize and later deserialize our User
object, the password
will be lost. A better approach would be to utilize a custom serialization method.
3. Ignoring the readObject
and writeObject
Methods
When you need custom behavior during serialization or deserialization, you can implement the writeObject
and readObject
methods. These methods allow for better control over how specific fields are handled.
private void writeObject(ObjectOutputStream oos) throws IOException {
oos.defaultWriteObject(); // Regular serialization
oos.writeObject(encrypt(password)); // Custom logic for sensitive fields
}
private void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException {
ois.defaultReadObject(); // Regular deserialization
password = decrypt((String) ois.readObject()); // Custom logic for sensitive fields
}
In this case, we are encrypting sensitive information before serialization and decrypting it during deserialization, thus enhancing security.
4. Serializing Non-Serializable Objects
If a class contains non-serializable fields, it can lead to NotSerializableException
. For instance:
class Order implements Serializable {
private String orderId;
private Payment payment; // Let's assume Payment isn't serializable
// Constructor and methods omitted for brevity
}
To avoid such issues, ensure all fields in a serializable class are either primitive types or implement Serializable
. If a field cannot be made serializable and is not essential for maintaining the object's state, mark it as transient.
5. Updating Class Definitions
If the class definition changes after serializing an object, you might run into compatibility issues. Changes like adding fields or method signatures can render older serialized objects unusable.
Always consider the implications of class modification. When making changes, increment the serialVersionUID
to indicate a new version of the class.
6. Serialization Performance
Serialization can be performance-heavy, especially for large objects. When working with large datasets or in high-performance applications, consider using alternatives like JSON or Protobuffer for serialization. These options are often faster and more efficient in terms of space.
For additional performance tuning, consider using ObjectOutputStream
buffering techniques.
7. Be Cautious with Inheritance
In cases of class inheritance, serialized fields might lead to unexpected behaviors. The subclass must maintain de/serialization consistency with its superclass, which necessitates careful management of the serialVersionUID
. Always define it in every class involved in inheritance.
Best Practices for Serialization
To avoid common pitfalls, adhere to the following best practices:
- Always define a
serialVersionUID
. - Handle sensitive information with
transient
and custom serialization methods. - Ensure all non-primitive fields are serializable or marked transient.
- Increment
serialVersionUID
when modifying class definitions. - Consider performance trade-offs and potential alternatives for serialization.
For comprehensive knowledge on Java serialization techniques, check out the official Java documentation here.
Closing the Chapter
Mastering Java serialization entails understanding the prerequisites, potential pitfalls, and best practices in managing your object state. By adhering to these guidelines, you can prevent many common errors associated with serialization and enhance your application's data handling capabilities.
Remember, serialization is powerful, but its pitfalls can be traps for the unwary. Always proceed with caution, validate your designs, and keep learning.
If you have any questions or comments regarding Java serialization, feel free to leave your thoughts below. Happy coding!
Checkout our other articles