Overcoming Common Spock Mocking Pitfalls in Spring Beans
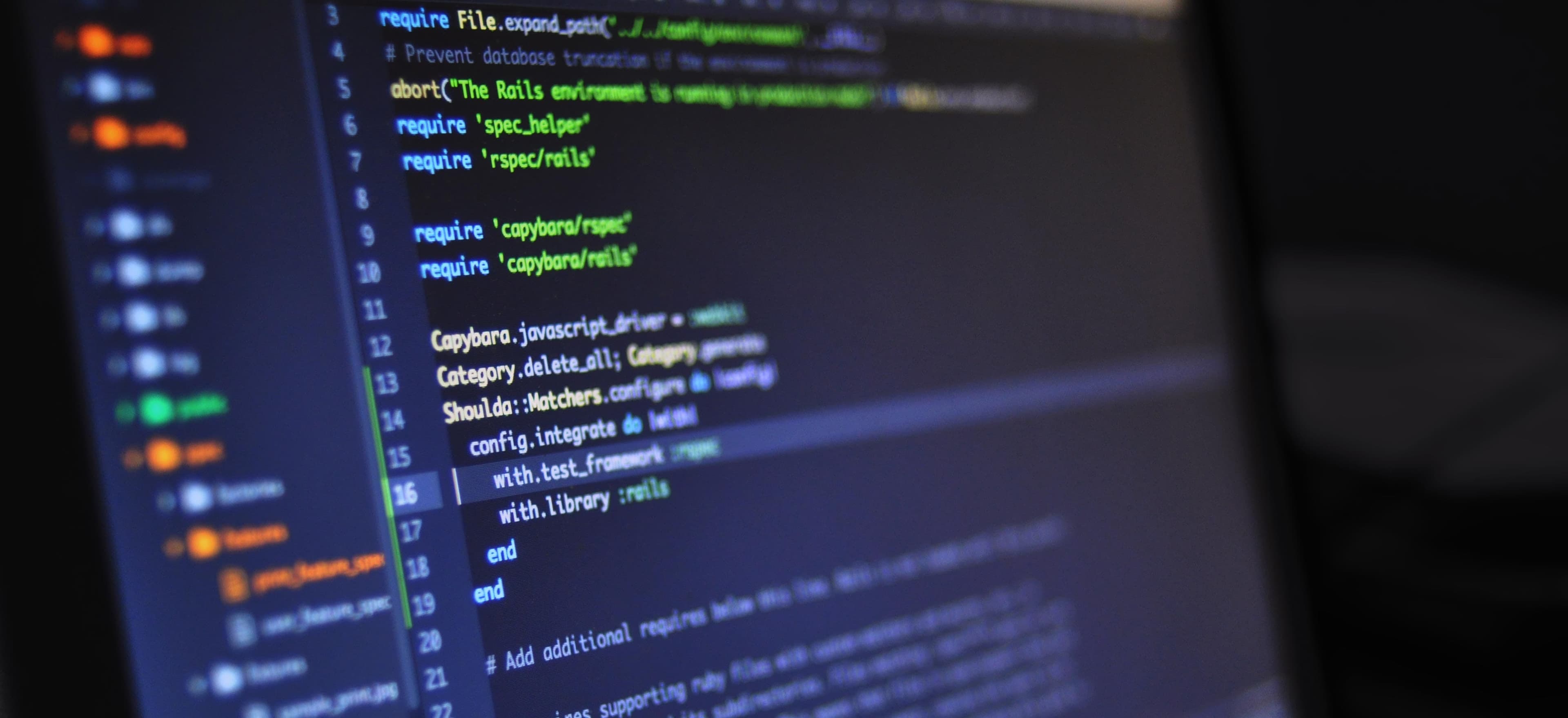
- Published on
Overcoming Common Spock Mocking Pitfalls in Spring Beans
When developing applications in Java, using a robust testing framework is key to delivering high-quality software. Spock, a testing and specification framework for Java and Groovy applications, has gained popularity due to its expressive and readable syntax. In the context of Spring applications, Spock enables developers to test Spring Beans effectively. However, mocking can often lead to pitfalls that could compromise our tests' reliability. In this article, we will explore common Spock mocking pitfalls when testing Spring Beans and how to overcome them, ensuring your tests remain robust and clear.
Understanding Spock and Spring
Before diving into pitfalls, let’s establish a foundation.
-
Spock: This framework uses a Groovy DSL to create tests that are both human-readable and concise. It offers unique features like data-driven testing, mocking, and more.
-
Spring: Spring is a powerful framework that facilitates dependency injection, making it easier to manage components and services in an application.
Spock works seamlessly with Spring, allowing for easy mocking of Spring Beans and dependencies. However, challenges can arise when mocking the interactions between them.
Common Spock Mocking Pitfalls
- Inadequate Mock Configuration
- Incorrect Use of Mocks vs Stubs
- Over-Mocking
- Skipping Setup Blocks
- Failure to Expect Proper Interactions
Let’s break these pitfalls down and provide examples for better understanding.
1. Inadequate Mock Configuration
Mocking without proper configuration leads to unexpected behaviors in tests. In Spock, it's essential to define mocks that correctly simulate the behavior of the Spring Beans they replace. Failing to do so might cause your tests to pass when they should fail (or vice versa).
Example:
class UserService {
private final UserRepository userRepository
UserService(UserRepository userRepository) {
this.userRepository = userRepository
}
String getUserName(Long userId) {
return userRepository.findById(userId)?.name
}
}
class UserServiceSpec extends spock.lang.Specification {
UserRepository userRepository = Mock()
UserService userService = new UserService(userRepository)
def "get user name returns correct name"() {
given:
userRepository.findById(1) >> new User(name: "John Doe")
when:
String result = userService.getUserName(1)
then:
result == "John Doe"
}
def "get user name returns null for non-existent user"() {
given:
userRepository.findById(2) >> null
when:
String result = userService.getUserName(2)
then:
result == null
}
}
Why It's Effective: In the example above, we define the behavior of userRepository
when calling findById()
, ensuring we are simulating realistic conditions. This setup maximizes test accuracy.
2. Incorrect Use of Mocks vs Stubs
In Spock, there is a clear distinction between mocks and stubs. Mocks are used to verify interactions, while stubs are used to provide pre-defined responses. Misusing them can lead to confusion.
Example:
def "should save user"() {
given:
UserRepository repository = Mock()
UserService service = new UserService(repository)
when:
service.saveUser(new User(name: "Jane"))
then:
1 * repository.save(_)
}
Why It Matters: In this test, we verify that saveUser()
interacts with the repository exactly once. Using a mock here is appropriate as we care about the interaction.
3. Over-Mocking
Over-mocking can make your tests less reliable and harder to read. When every dependency is mocked, you may lose sight of what the actual system under test (SUT) does.
Best Practice: Only mock what you need to.
Example:
def "should compute profile"() {
given:
ProfileService profileService = new ProfileService(Mock(UserService))
when:
profileService.computeProfile(1)
then:
// Assertions here
}
Why It's Important: Here, if UserService
has many dependencies, mocking all of them makes it hard to validate the actual behavior of ProfileService
. Only mock dependencies critical for the test scenario.
4. Skipping Setup Blocks
Failing to set up using the setup()
and cleanup()
blocks can lead to test duplication and maintenance difficulties.
Example:
class UserServiceSpec extends spock.lang.Specification {
UserRepository repository
UserService service
def setup() {
repository = Mock()
service = new UserService(repository)
}
def "test case"() {
given:
repository.findById(1) >> new User(name: "Sample User")
when:
String name = service.getUserName(1)
then:
name == "Sample User"
}
}
Why It Is Necessary: The setup()
method helps initialize test objects before each test, promoting code reuse and clarity.
5. Failure to Expect Proper Interactions
Spock allows us to specify the expected interactions with mocks. Forgetting to assert these interactions can lead to missed bugs.
Example:
def "should call repository on user creation"() {
given:
UserRepository repository = Mock()
UserService service = new UserService(repository)
when:
service.createUser(new User(name: "New User"))
then:
1 * repository.save(_)
}
Key Takeaway: Explicitly define expectations for interactions in every test case. This practice enhances the test's clarity and ensures that your dependencies behave as intended.
Tips for Effective Mocking with Spock
-
Limit the Scope of Mocking: Only mock components that impact the behavior you want to test.
-
Structure Your Tests: Utilize
setup()
,cleanup()
, andwhere:
blocks effectively for clarity. -
Use Annotations: Leverage Spock's annotations like
@Mock
,@Subject
, and@Shared
to better organize and maintain tests. -
Static Mocking: For scenarios requiring mocking of static methods, Spock's built-in capability allows you to mock those methods, but use it judiciously.
Wrapping Up
Overcoming common mocking pitfalls in Spock can dramatically increase the reliability and maintainability of your tests for Spring Beans. By understanding the framework's features, adhering to best practices, and avoiding the aforementioned pitfalls, developers can write cleaner and more effective tests.
Implementing these strategies will not only enhance your current tests but will cement good practices for future development. As with any testing framework, knowledge and experience are key to making the most of Spock’s powerful features. Happy testing!
For additional context on Spock and its documentation, be sure to check out the official Spock Framework documentation.