Overcoming the Hidden Costs of Technical Debt
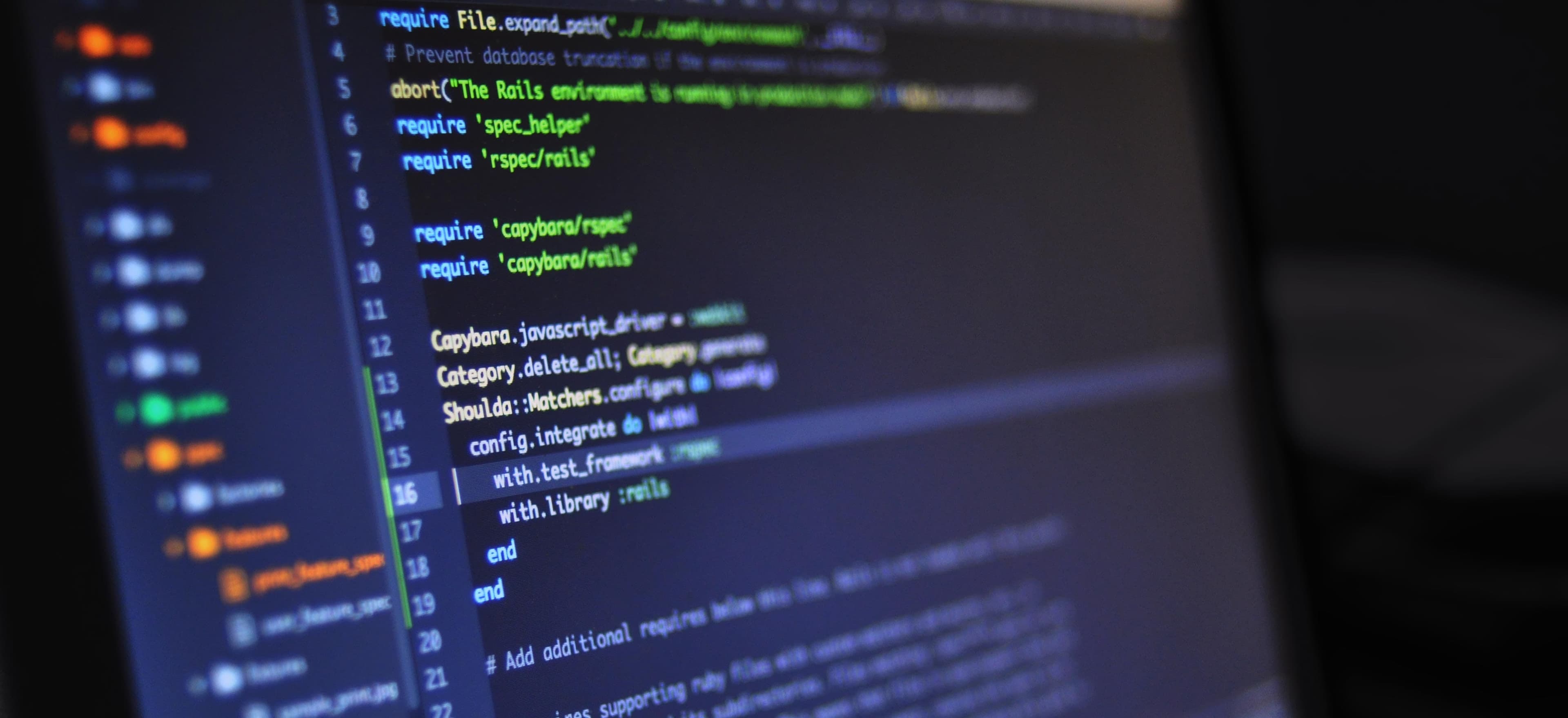
- Published on
Overcoming the Hidden Costs of Technical Debt
Technical debt is a term every software developer has likely heard. But what does it really mean, and why should we care? In this blog post, we'll delve into the depths of technical debt, addressing its hidden costs, and provide strategies to effectively manage and overcome it. Let's unpack this concept systematically.
What is Technical Debt?
Technical debt is akin to financial debt; it refers to the implied cost of future refactoring or redoing work due to the shortcuts taken in the present. Imagine a developer opting for a quick fix instead of a long-term solution. Initially, this may result in faster delivery. However, that fix may introduce complications later, leading to more significant issues down the line.
Why It Matters
The real kicker with technical debt is that it's often invisible until it manifests as bugs, performance degradation, or decreased developer productivity. According to a 2017 report by Stripe, about 60% of developers spend more time fixing existing code than writing new code. Understanding and managing technical debt is not just about code quality, but also about improving team dynamics and productivity.
Types of Technical Debt
Technical debt can be classified into several categories:
- Deliberate Debt: This stems from consciously chosen shortcuts, like rushing to meet a deadline.
- Inadvertent Debt: This arises from lack of knowledge or awareness, such as using outdated libraries.
- Bit Rot: Over time, code can degrade if not maintained; what was once a solid solution may become ineffective.
- Outdated Architectures: When the underlying systems become obsolete or can't scale with current needs.
Recognizing these types is vital for understanding their unique impact and developing targeted strategies to address them.
The Hidden Costs of Technical Debt
Technical debt can have far-reaching consequences. Here are some hidden costs to consider:
1. Slower Development Speed
Adding features becomes cumbersome as the underlying codebase grows more complex. Developers may hesitate to touch certain areas of code, fearing that their changes could cause breakages.
2. Increased Bug Rate
With a tangled codebase, the likelihood of introducing new bugs skyrockets. Even minor changes can lead to significant unforeseen issues, which ultimately consumes more development time.
3. Lower Morale
Developers often feel demotivated when perpetually dealing with junky code. This can lead to burnout and, quite possibly, higher turnover.
4. Difficulties in Onboarding
New developers may struggle to understand intricate or poorly written systems, leading to longer onboarding processes and decreased productivity.
5. Technical Reviews Become More Time-Consuming
As technical debt accumulates, code reviews take longer. Reviewers may need to sift through nested conditions or outdated practices to ensure quality, which can lead to friction among team members.
Strategies to Address Technical Debt
Addressing technical debt requires a proactive approach. Here are several strategies that can help alleviate its hidden costs.
1. Regular Refactoring
Scheduled refactoring sessions can combat the accumulation of technical debt. Refactoring involves restructuring existing code without changing its external behavior, which keeps the system clean and manageable.
// This method is too complex; let's refactor it.
public void processOrders(List<Order> orders) {
for (Order order : orders) {
if (order.getStatus().equals("COMPLETE")) {
// Logic for completed orders
} else if (order.getStatus().equals("PENDING")) {
// Logic for pending orders
}
}
}
// A simple refactor makes it more readable and maintainable.
public void processOrder(Order order) {
switch (order.getStatus()) {
case "COMPLETE":
completeOrder(order);
break;
case "PENDING":
pendingOrder(order);
break;
}
}
The above code is streamlined, enhancing readability and maintainability.
2. Create a Technical Debt Register
Maintaining a list of known technical debt allows teams to prioritize and track debt effectively. Use a tool like Jira or a simple spreadsheet to log items you need to address.
3. Set Time Aside for Debt Repayment
Encourage teams to allocate a fixed percentage of their time to address technical debt. This could be done during sprint planning, where a specific velocity is reserved for refactoring tasks.
4. Enforce Code Reviews
Establish a strong code review culture. Code reviews help catch potential debt before it becomes a problem, improving overall code quality.
5. Utilize Automated Testing
Establishing a robust automated testing framework ensures code is tested efficiently before deployment. It also acts as a safety net when making changes or refactoring.
import static org.junit.Assert.assertEquals;
public class OrderTest {
@Test
public void testProcessOrder_Complete() {
Order order = new Order("1", "COMPLETE");
OrderProcessor processor = new OrderProcessor();
processor.processOrder(order);
assertEquals("Processed Complete", order.getStatus());
}
}
The above unit test ensures that our processOrder function behaves correctly when handling completed orders. Automated tests catch issues early, reducing the risk of incurring additional debt.
6. Invest in Continued Learning
Encourage teams to invest time in learning about new technologies, frameworks, and methodologies. This can help prevent accidental accumulation of technical debt due to outdated practices.
Final Considerations
Technical debt might feel like a necessary evil in the fast-paced world of software development, but it doesn't have to be. By recognizing the hidden costs and employing strategic management techniques, teams can maintain high code quality and stay agile in their development efforts.
Key Takeaways:
- Understand the concept and types of technical debt.
- Recognize the hidden costs it can impose on your projects.
- Implement continuous refactoring and establish a culture focused on technical debt management.
Navigating the world of technical debt can seem daunting at first, but the rewards—improved productivity, higher morale, and cleaner code—are well worth the effort. Start implementing these strategies today and turn debt into an asset rather than a liability.
Additional Resources
For further reading on technical debt, consider exploring the following articles:
Harnessing your understanding of technical debt can transform your approach to software development, leading to more maintainable and robust applications. Let's tackle it together!