Understanding Idempotency: Why It Matters in APIs
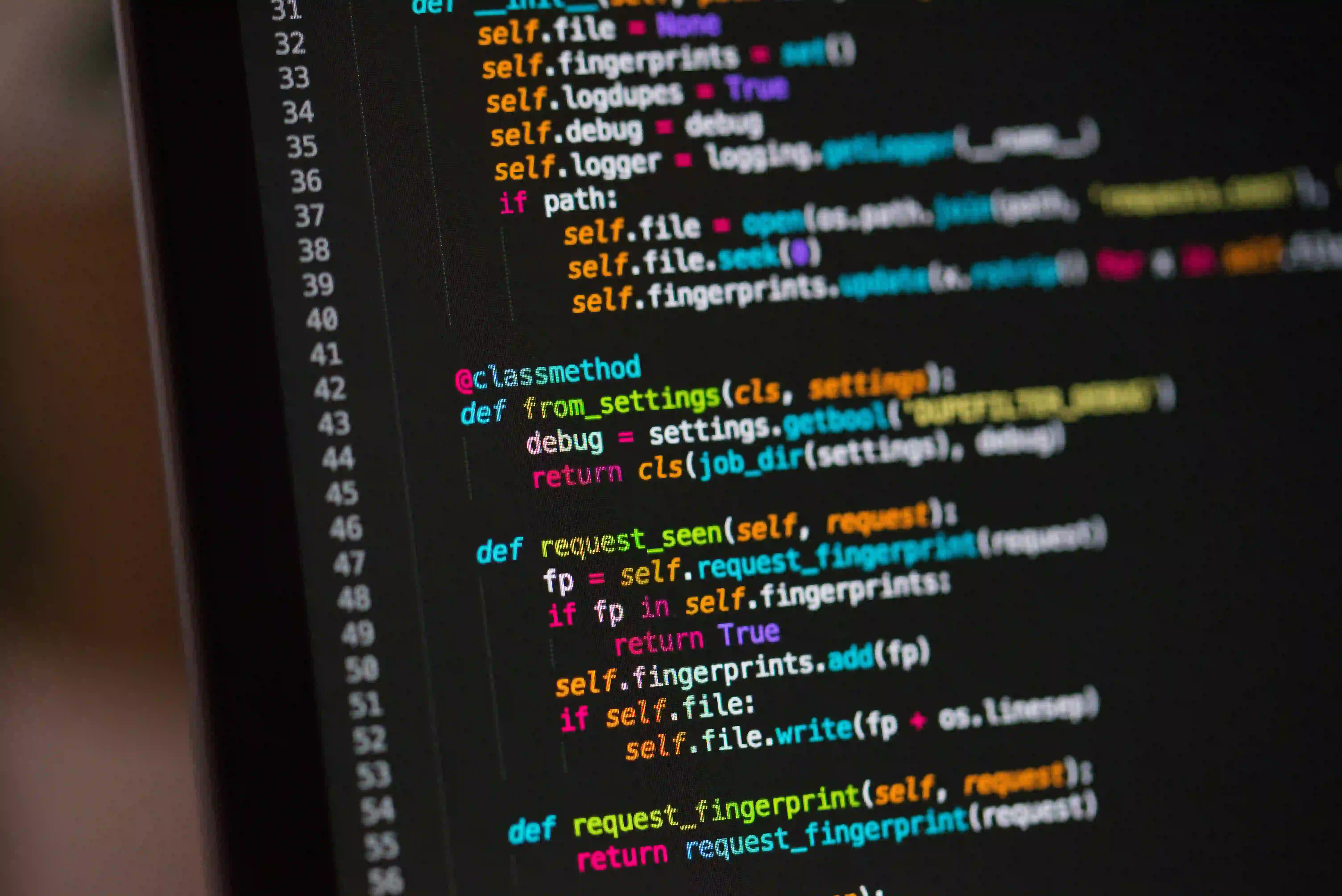
Understanding Idempotency: Why It Matters in APIs
In the realm of web development and API design, concepts become crucial for ensuring robust, efficient, and predictable interactions between clients and servers. One such concept is idempotency. This blog post will take a deep dive into what idempotency means, why it matters in APIs, and how it can be implemented effectively.
What is Idempotency?
At its core, idempotency refers to an operation that can be performed multiple times without changing the result beyond the initial application. In simple terms, making a request once is equivalent to making it multiple times.
Consider the HTTP methods:
- GET is idempotent. Fetching a resource multiple times yields the same result.
- PUT is also idempotent. When updating a resource to the same state, it won't cause any additional changes on subsequent updates.
Conversely, the HTTP method POST is generally not idempotent because it creates a new resource each time it is invoked.
Why Does Idempotency Matter?
Idempotency is essential for several reasons:
-
Fault Tolerance: Network issues can lead to duplicate requests. Idempotent operations ensure that retrying requests won't cause unintended consequences.
-
Consistency: In distributed systems, multiple services interact. Idempotent APIs enhance data consistency by preventing duplicate entries or modifications.
-
Simplified Client Logic: Clients will have less complexity to manage when they can retry requests safely. This makes client implementations simpler and more robust.
-
Improved User Experience: If a user submits a form and doesn't receive immediate feedback, they might resubmit the form. Idempotent APIs prevent duplicate submissions and improve feedback loops.
Idempotency in Practice
To further explore idempotency, let’s look at a simple API design scenario.
Designing an Idempotent API
Imagine a profile update API where users can update their contact details. This update should be idempotent. Here’s how we can structure the API:
@RestController
@RequestMapping("/api/v1/profiles")
public class ProfileController {
@PutMapping("/{id}/updateContact")
public ResponseEntity<ProfileResponse> updateContact(
@PathVariable("id") Long id,
@RequestBody ContactDetails contactDetails) {
Profile profile = findProfileById(id); // Fetch profile
if (profile == null) {
return ResponseEntity.notFound().build();
}
profile.setContactDetails(contactDetails); // Update details
saveProfile(profile); // Persist changes
return ResponseEntity.ok(new ProfileResponse(profile));
}
}
Analyzing the Code
-
@PutMapping: This annotation indicates that our method responds to HTTP PUT requests, aligning with idempotent operations.
-
Response Entity: We return an appropriate response entity that provides meaningful feedback, including a possible
404 Not Found
for invalid IDs. -
Profile Manipulation: The core idea here is that any valid update request with the same contact details would not change the final state for that profile, maintaining idempotency.
Handling Edge Cases
Despite our best intentions, real-world scenarios present various challenges. Consider a situation where a client performs a PUT request with a different set of data:
@PutMapping("/{id}/updateContact")
public ResponseEntity<ProfileResponse> updateContact(
@PathVariable("id") Long id,
@RequestBody ContactDetails contactDetails) {
Profile profile = findProfileById(id);
if (profile == null) {
return ResponseEntity.notFound().build();
}
// Check if incoming contact details differ from the current value
if (!profile.getContactDetails().equals(contactDetails)) {
profile.setContactDetails(contactDetails);
saveProfile(profile);
}
return ResponseEntity.ok(new ProfileResponse(profile));
}
In this update, we added a check to avoid unnecessary database operations if the incoming details are the same as the existing ones. This optimizes performance further, minimizing redundant writes.
Implementing Idempotency Tokens
To bolster idempotency in use cases like POST requests, many APIs implement idempotency tokens. Here's an example in a hypothetical order placement API:
@PostMapping("/orders")
public ResponseEntity<OrderResponse> createOrder(
@RequestHeader("Idempotency-Key") String idempotencyKey,
@RequestBody OrderRequest orderRequest) {
Optional<Order> existingOrder = findOrderByIdempotencyKey(idempotencyKey);
if (existingOrder.isPresent()) {
return ResponseEntity.ok(new OrderResponse(existingOrder.get()));
}
Order newOrder = createNewOrder(orderRequest);
saveOrder(newOrder, idempotencyKey); // Save the token with the order
return ResponseEntity.status(HttpStatus.CREATED).body(new OrderResponse(newOrder));
}
Key Takeaways from the Code
-
Idempotency-Key Header: Clients send an idempotency key with the request. If the request is sent multiple times with the same key, the API can return the existing order response without creating a new order.
-
Optional Handling: The logic checks for an existing order before proceeding to create one, reinforcing idempotency.
The Closing Argument
Idempotency is a cornerstone of robust API design, facilitating fault tolerance, consistency, and improved user experiences. By understanding and applying idempotency in our API methods, we can create systems that are resilient and predictable.
Further Reading
- For an in-depth understanding, check out RESTful API Design.
- Explore the importance of HTTP Methods in web APIs.
- Read more on application design patterns that involve idempotency.
By integrating idempotency into API design, you're not just adding an extra layer of robustness; you're ultimately building trust in the client-server relationship. Embrace idempotency today for more resilient APIs.