Understanding the Ternary Operator: Common Pitfalls Explained
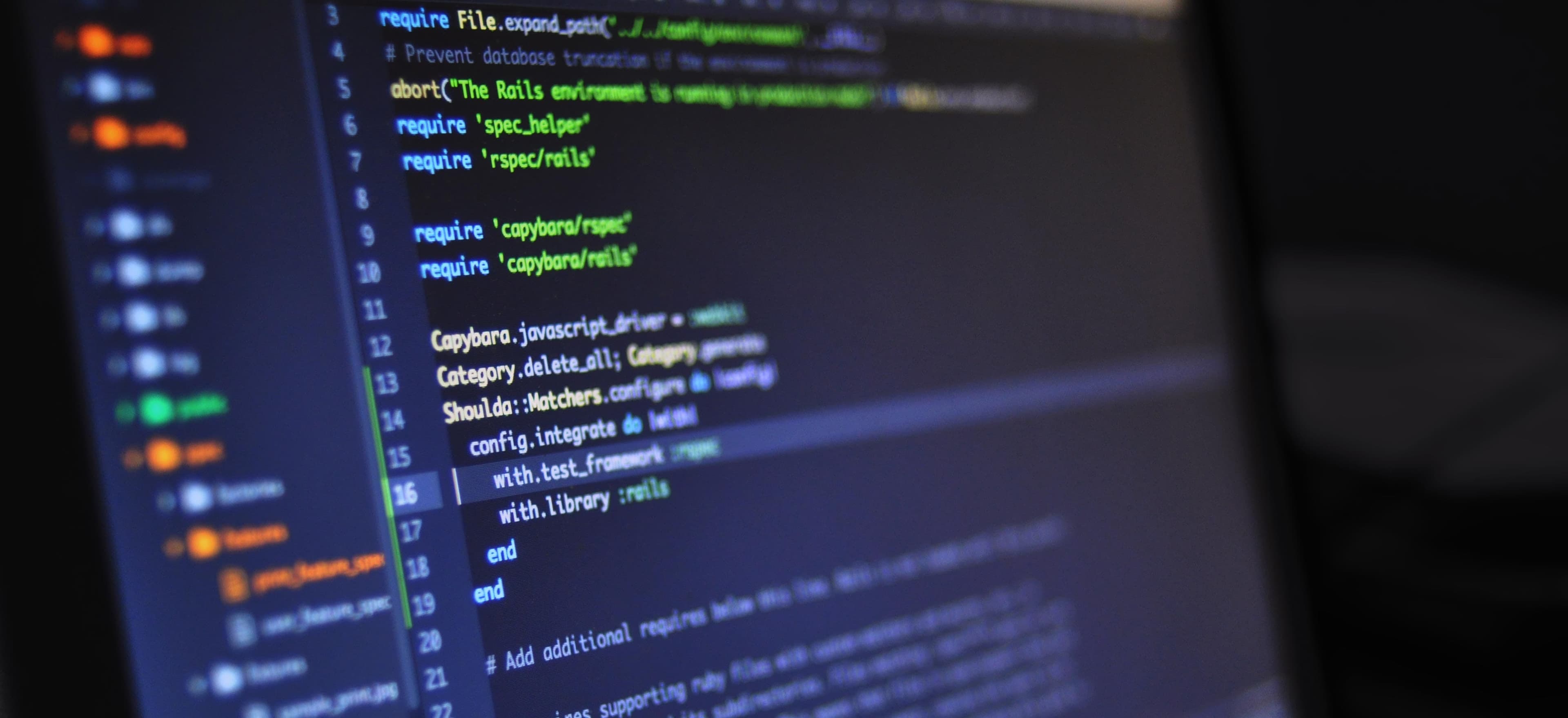
- Published on
Understanding the Ternary Operator: Common Pitfalls Explained
In Java, the ternary operator is a powerful, concise expression that can significantly optimize your code. However, many developers, especially novices, can stumble when utilizing this seemingly straightforward operator. In this blog post, we will delve into the intricacies of the ternary operator, its usage, and, most importantly, the common pitfalls that can occur. By the end, you should have a more profound understanding of effective ternary operator usage in your Java applications.
What is the Ternary Operator?
The ternary operator, also referred to as the conditional operator, is a shorthand way to perform conditional statements in Java. It takes three operands, making it unique among operators. The syntax is as follows:
conditionalExpression ? resultIfTrue : resultIfFalse;
Here is a simplified breakdown of the syntax:
conditionalExpression
is a boolean expression that evaluates to either true or false.resultIfTrue
is the value returned if the conditional expression evaluates to true.resultIfFalse
is the value returned if the conditional expression evaluates to false.
Example of the Ternary Operator
Let’s look at a simple example to understand how it works:
int num = 10;
String result = (num % 2 == 0) ? "Even" : "Odd";
System.out.println(result); // Output: Even
In this snippet, we check whether num
is even or odd. If num
is even, it returns "Even"; otherwise, it returns "Odd." The ternary operator compresses what could be a multi-line if-else statement into a single line, enhancing readability.
Common Pitfalls of the Ternary Operator
While the ternary operator can make your code cleaner and more concise, improper usage can lead to confusion, bugs, and maintenance headaches. Below are some common pitfalls you should be mindful of:
1. Misunderstanding Operator Precedence
When using the ternary operator alongside other operators, misunderstanding operator precedence can lead to unexpected results. For instance:
int a = 5;
int b = 10;
int maxValue = (a > b) ? a : (b < 15 ? b : 15);
Here, the lines may seem straightforward, but the second condition is nested within the first. This can confuse developers who expect a simpler outcome. To clarify intent, consider breaking this into more explicit statements:
int maxValue = (a > b) ? a : b;
maxValue = (maxValue < 15) ? maxValue : 15;
2. Returning Incompatible Types
A major pitfall arises when the true
and false
branches of a ternary expression return incompatible types. For instance:
Object result = (condition) ? "String Value" : 10;
// This will compile, but often leads to confusion.
While Java's automatic boxing features allow this code to compile, it results in a vague return type. It's generally advisable to ensure that both branches return the same type, to maintain clarity and type safety.
3. Nested Ternary Operators
While nesting ternary operators is possible, it's often ill-advised due to reduced code readability. Consider the following example:
int a = 5, b = 10, c = 15;
String result = (a > b) ? "a" : (b > c) ? "b" : "c";
This can quickly become hard to read and maintain. Instead, opt for multi-line statements, even if they are lengthier:
String result;
if (a > b) {
result = "a";
} else if (b > c) {
result = "b";
} else {
result = "c";
}
4. Using Ternary for Side Effects
It's crucial to remember that the ternary operator is meant for assignments and evaluations, not for executing statements with side effects. Consider this example:
(1 < 2) ? System.out.println("True") : System.out.println("False"); // Compiler error
This won't compile since you cannot use the ternary operator to execute methods that produce side effects directly. Always use statements where side effects are necessary.
5. Overusing the Ternary Operator
Finally, the most subtle pitfall is overusing the ternary operator to the detriment of code clarity. While the operator can simplify some scenarios, in more complex situations, it can clutter code:
String userType = (role.equals("admin")) ? "Administrator" :
(role.equals("user")) ? "Regular User" : "Guest";
While this compacts logic, it sacrifices readability. In this case, using a switch-case structure might deliver superior clarity.
In Conclusion
The ternary operator can enhance your Java code efficiency when used correctly. However, a deeper understanding of its workings and potential pitfalls will make you a more competent programmer. You must prioritize code readability and maintainability as much as brevity.
For further exploration of better Java practices, consider checking out Effective Java by Joshua Bloch and Java: The Complete Reference by Herbert Schildt.
Remember, coding is not just about writing the least amount of code; it’s about writing code that is easy to read, maintain, and debug. Adopting best practices in your use of the ternary operator will bolster your Java skillset. Happy coding!
Checkout our other articles