Finding the Right Number of Java Threads for Your App
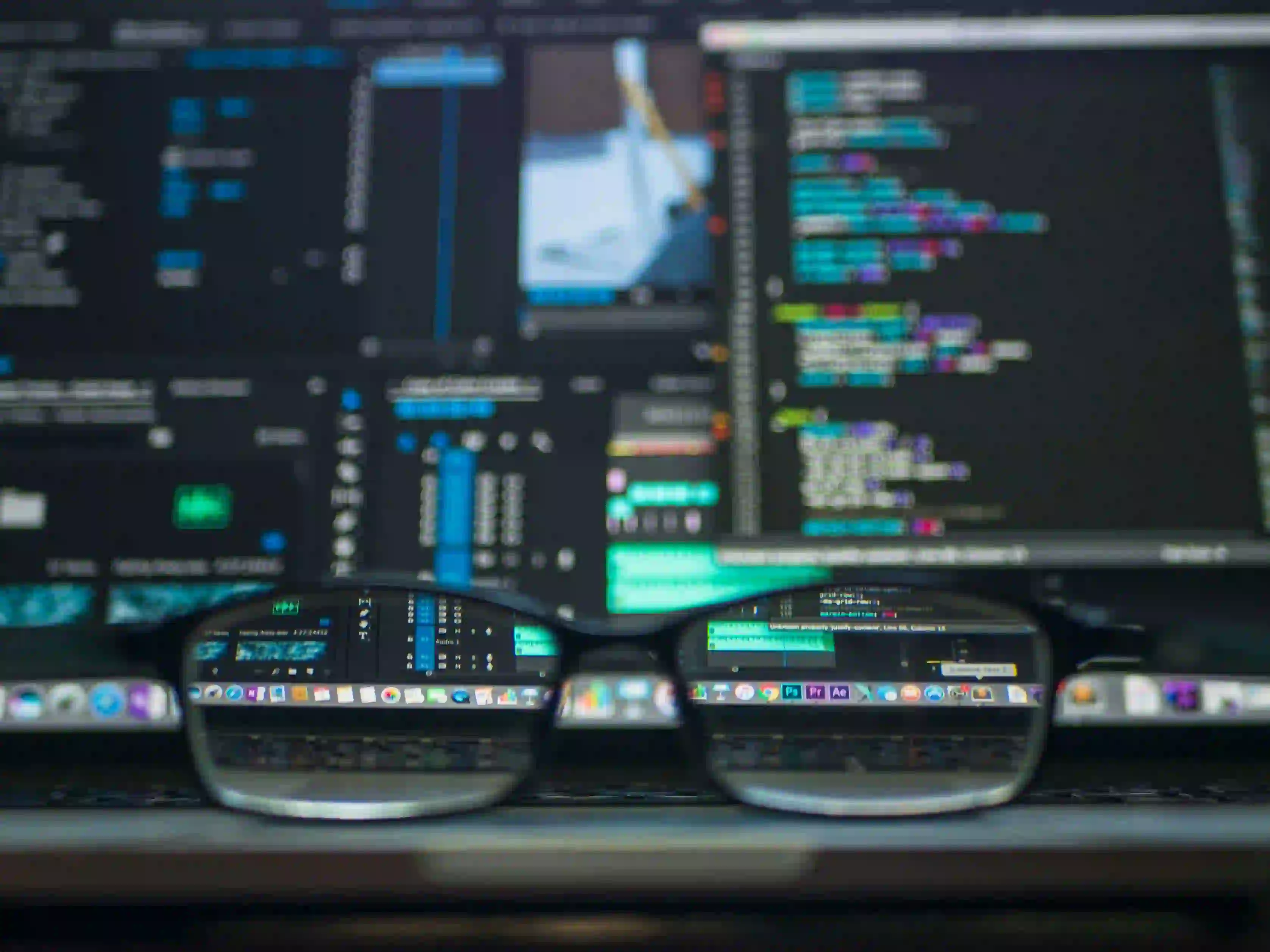
Finding the Right Number of Java Threads for Your App
When developing Java applications, performance optimization is crucial. One key factor that significantly impacts the performance of an application is the number of threads it utilizes. This post will explore how to determine the right number of Java threads for your application, help you understand the threading model in Java, and offer practical examples along the way.
Understanding Java Threads
Java threads are lightweight processes that enable concurrent execution of tasks within a program. By using threads, developers can enhance the responsiveness of applications and make better use of CPU resources. However, mismanagement of threads can lead to various issues such as reduced performance due to context switching, increased memory usage, and even deadlocks.
Why Use Threads?
- Concurrency: Threads allow multiple tasks to run simultaneously, improving the application's performance by utilizing available CPU cores effectively.
- Responsiveness: In UI applications, using threads helps keep the user interface responsive while performing background operations.
- Resource Utilization: Properly managed threads can significantly increase an application's throughput by allowing concurrent operations.
The Threading Model in Java
Java's threading model is built around the Java Virtual Machine (JVM) and is designed to be platform-independent. The core classes associated with threading in Java include:
Thread
: Represents a single thread of execution.Runnable
: An interface that should be implemented by any class whose instance is intended to be executed by a thread.ExecutorService
: A higher-level interface for managing pools of threads.
Understanding Thread
and Runnable
When creating threads in Java, developers have two primary options: extending the Thread
class or implementing the Runnable
interface. However, it is generally recommended to prefer the Runnable
interface due to its flexibility, particularly when working with thread pools. Here's a brief example:
class MyRunnable implements Runnable {
@Override
public void run() {
System.out.println("Thread is running.");
}
}
// Creating a thread with the Runnable implementation
Thread thread = new Thread(new MyRunnable());
thread.start();
Why use Runnable
?
Using Runnable
allows you to separate the logic of the task from the execution. This is particularly useful when you want to pass task objects to executors or when you need to extend a class, something not possible when extending Thread
.
Choosing the Right Number of Threads
Determining the optimal number of threads for your application is not straightforward. Below are some considerations to help guide your decision:
1. Measure Maximum Concurrent Workload
Understand the maximum workload your application needs to handle simultaneously. For example, in web server applications, you might need to handle multiple requests. Start by monitoring existing application performance metrics.
2. Consider CPU Bound vs. I/O Bound Tasks
Tasks can generally be classified as CPU-bound (tasks that require significant computation) or I/O-bound (tasks that wait for external resources, such as file or database access).
- For CPU-bound tasks, the optimal number of threads is often around the number of available processor cores. You can use the following Java snippet to find the number of cores:
int numberOfCores = Runtime.getRuntime().availableProcessors();
System.out.println("Available processors: " + numberOfCores);
- For I/O-bound tasks, such as reading from databases or making HTTP requests, you can use a higher number of threads to ensure that while some threads are blocked waiting for I/O operations, others can continue processing. A common strategy is to use a number of threads significantly greater than the number of cores.
3. Utilize Thread Pools
Thread pools help manage the number of threads more efficiently by recycling existing threads and controlling their lifecycle. The ExecutorService
framework in Java provides a robust solution for managing thread pools. For example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
ExecutorService executorService = Executors.newFixedThreadPool(numberOfCores);
for (int i = 0; i < 10; i++) {
executorService.submit(new MyRunnable());
}
executorService.shutdown();
Why Use ExecutorService
?
Using ExecutorService
allows you to specify the number of threads required, submit tasks asynchronously, and manage their lifecycle while minimizing the resource overhead associated with creating and destroying threads.
4. Conduct Performance Testing
After you have an initial configuration, it's critical to conduct performance tests. Use profiling tools to monitor the application's performance and resource usage, adjusting the number of threads according to the results you observe.
Some popular Java performance profiling tools include:
- VisualVM
- Eclipse MAT (Memory Analyzer Tool)
- YourKit
5. Monitor and Optimize Continuously
Even after deployment, remember that user loads and application demands can change over time. Continuously monitor the performance and resource usage of your application to make necessary adjustments to the thread count as needed.
Example of a Full Program
Incorporating everything discussed so far, let's create a simple Java application using thread pools to run concurrent tasks:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
class MyTask implements Runnable {
private final int taskId;
MyTask(int taskId) {
this.taskId = taskId;
}
@Override
public void run() {
System.out.println("Running Task ID: " + taskId + " in " + Thread.currentThread().getName());
try {
// Simulating task duration
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
System.out.println("Task ID: " + taskId + " was interrupted.");
}
}
}
public class ThreadPoolExample {
public static void main(String[] args) {
int numberOfCores = Runtime.getRuntime().availableProcessors();
ExecutorService executorService = Executors.newFixedThreadPool(numberOfCores);
for (int i = 0; i < 10; i++) {
executorService.submit(new MyTask(i));
}
executorService.shutdown();
System.out.println("All tasks submitted.");
}
}
In this example, we define a MyTask
class implementing Runnable
. In the main
method, we create a fixed thread pool based on the number of available cores and submit ten tasks. Finally, we shut down the executor service.
The Closing Argument
Finding the right number of threads for your Java application is essential for maximizing performance. It involves understanding your application's workload, considering the type of tasks, utilizing thread pools, conducting performance testing, and continually monitoring your application.
Implementing a thoughtful approach to threading can make a significant difference. Remember, it's not just about using more threads—it's about using the right number of threads effectively.
For additional context, check out Java Concurrency in Practice and Thread Pools in Java.
By adopting the techniques discussed in this blog post, you can significantly optimize the performance of your Java applications while ensuring they remain responsive and efficient under various workloads.