Common Issues with Spring Data Couchbase 1.0 GA Release
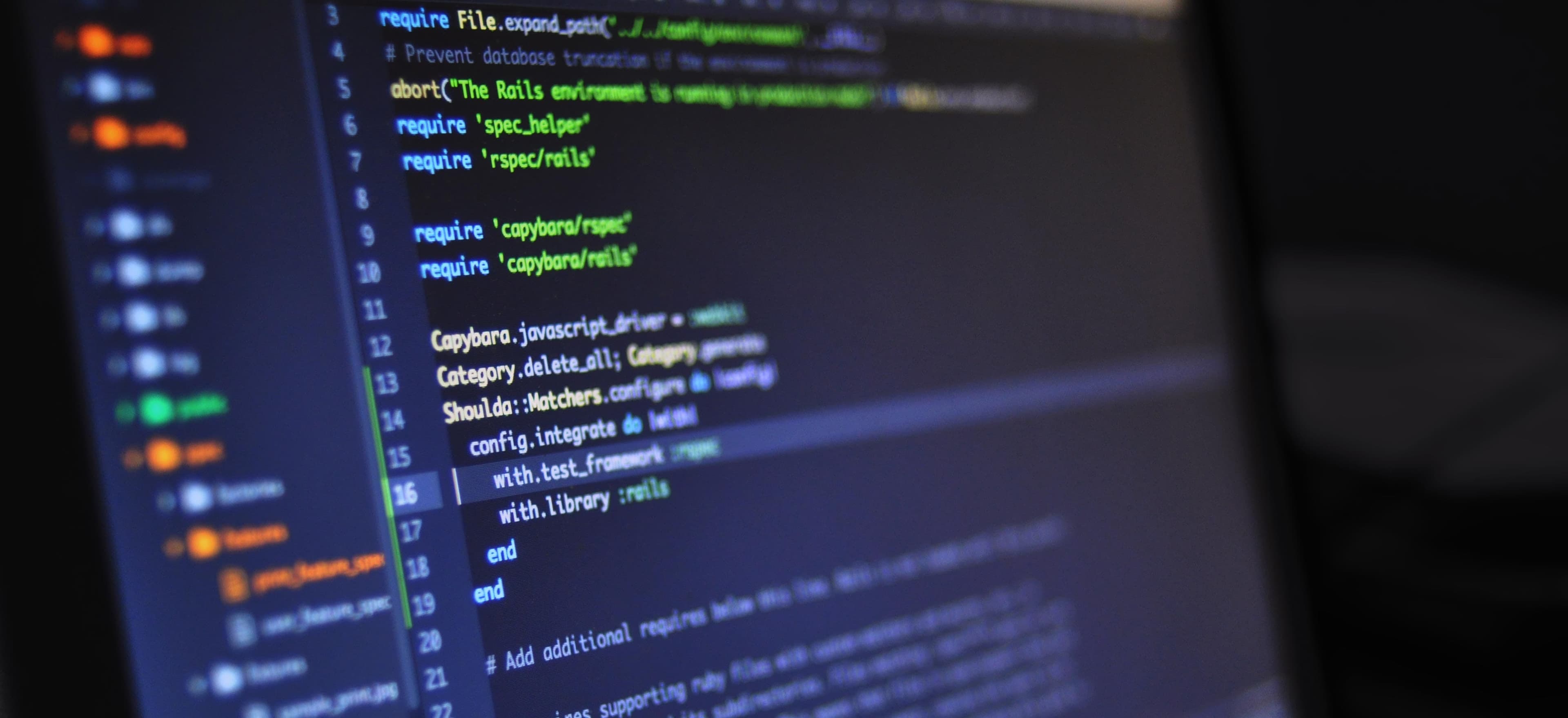
- Published on
Common Issues with Spring Data Couchbase 1.0 GA Release
Spring Data Couchbase provides a powerful and seamless way to interact with Couchbase databases using Spring's familiar programming model. Despite its advantages, developers may encounter various challenges when using the 1.0 GA release. In this post, we will discuss common issues along with useful tips and workarounds, ensuring you can leverage the full potential of Spring Data Couchbase for your applications.
Table of Contents
The Starting Line to Spring Data Couchbase
Spring Data Couchbase is part of the larger Spring Data family that aims to simplify data access in Java applications. It provides an abstraction over the native Couchbase SDK, allowing developers to utilize Spring's powerful features such as dependency injection, transaction management, and declarative data access in a straightforward way.
When adopting a new technology, especially a GA release, it’s common to face some hurdles. This post aims to identify these challenges and provide solutions.
Common Issues
1. Configuration Problems
One significant issue that developers encounter is misconfiguration. This can stem from incorrect connection settings or Bean definitions.
How to Address Configuration Problems
- Consistency Checks: Ensure your
application.properties
orapplication.yml
files are correctly set up.
spring.couchbase.connection-string=127.0.0.1
spring.couchbase.bucket.name=my_bucket
spring.couchbase.username=admin
spring.couchbase.password=password
Always test your connections independently of the Spring application to confirm they are functioning correctly. Also, confirm that your block-specific settings are correctly set for versioning and document expiration to avoid runtime errors.
2. Repository Issues
Spring Data Couchbase allows you to define Repository
interfaces to simplify CRUD operations. However, developers frequently run into issues with method naming conventions and resulting queries.
Why This Happens
The underlying issues typically revolve around misunderstandings of how Spring interprets method names to create database queries.
Resolution
- Double-check Method Names: Review your repository interfaces to ensure they follow the correct naming conventions.
public interface UserRepository extends CouchbaseRepository<User, String> {
List<User> findByLastName(String lastName);
}
If you face problems with method resolution, consider using the @Query
annotation to explicitly state your query.
@Query("#{#n1ql.selectEntity} WHERE lastName = $1")
List<User> findByLastName(String lastName);
This way, you gain more control over the query and reduce potential pitfalls related to method naming conventions.
3. Query Language Challenges
Couchbase uses N1QL as its query language, which can sometimes lead to unexpected results when developing applications. Misunderstandings around this could result in poorly performing queries or runtime exceptions.
Best Approach for N1QL
-
Familiarize Yourself with N1QL: If coming from a SQL background, spend some time getting accustomed to N1QL.
-
Validate Your Queries: Utilize the Couchbase Query Workbench to experiment with your queries before implementing them in your Spring application.
Here is an example that queries a user based on email:
SELECT META(`my_bucket`).id, a.*
FROM `my_bucket` AS a
WHERE a.type = "user" AND a.email = "user@example.com";
This query is more precise than attempting to construct it through method naming alone and gives better performance.
4. Performance Bottlenecks
As applications scale, performance becomes a pressing concern. Couchbase is designed to be performant, but certain configurations can lead to slowdowns.
Tips to Optimize Performance
- Indexing: Ensure indexes are properly configured for N1QL queries. Missing indexes can severely degrade performance.
CREATE INDEX idx_last_name ON `my_bucket`(lastName);
- Connection Pooling: Review your connection pool configurations within Spring to avoid exhaustion or delays.
5. Version Compatibility
When using Spring Data Couchbase, keep an eye on the compatibility between the library version and the Couchbase server version. Issues often arise when updating your dependencies without verifying compatibility.
Suggested Approach
Check the Spring Data release notes and the Couchbase documentation for comprehensive compatibility matrices. This ensures that you are using the right library versions with your server.
Best Practices
-
Document Everything: Maintain good documentation on how your application interfaces with Couchbase. This will help in minimizing configuration issues.
-
Update Dependencies Regularly: Subscribing to releases and updates will help manage bugs and security vulnerabilities.
-
Performance Testing: Conduct thorough tests, especially around N1QL queries, to ensure that your application can handle your expected load.
-
Use the Community: Join the Couchbase and Spring Data communities to stay informed on issues and solutions.
A Final Look
The Spring Data Couchbase 1.0 GA release comes with its set of challenges that developers must navigate. From configuration issues to repository misunderstandings, being aware of these potential pitfalls will enable you to develop robust applications efficiently.
By understanding the underlying technology, utilizing best practices, and seeking support when needed, you can harness the full power of Spring Data Couchbase. With careful attention and proactive measures, your journey with this framework will lead to successful implementations.
For more resources, consider checking out the official Spring Data Couchbase documentation and the Couchbase documentation. Happy coding!
Checkout our other articles