Embracing Failure: The Key Fear for Aspiring Developers
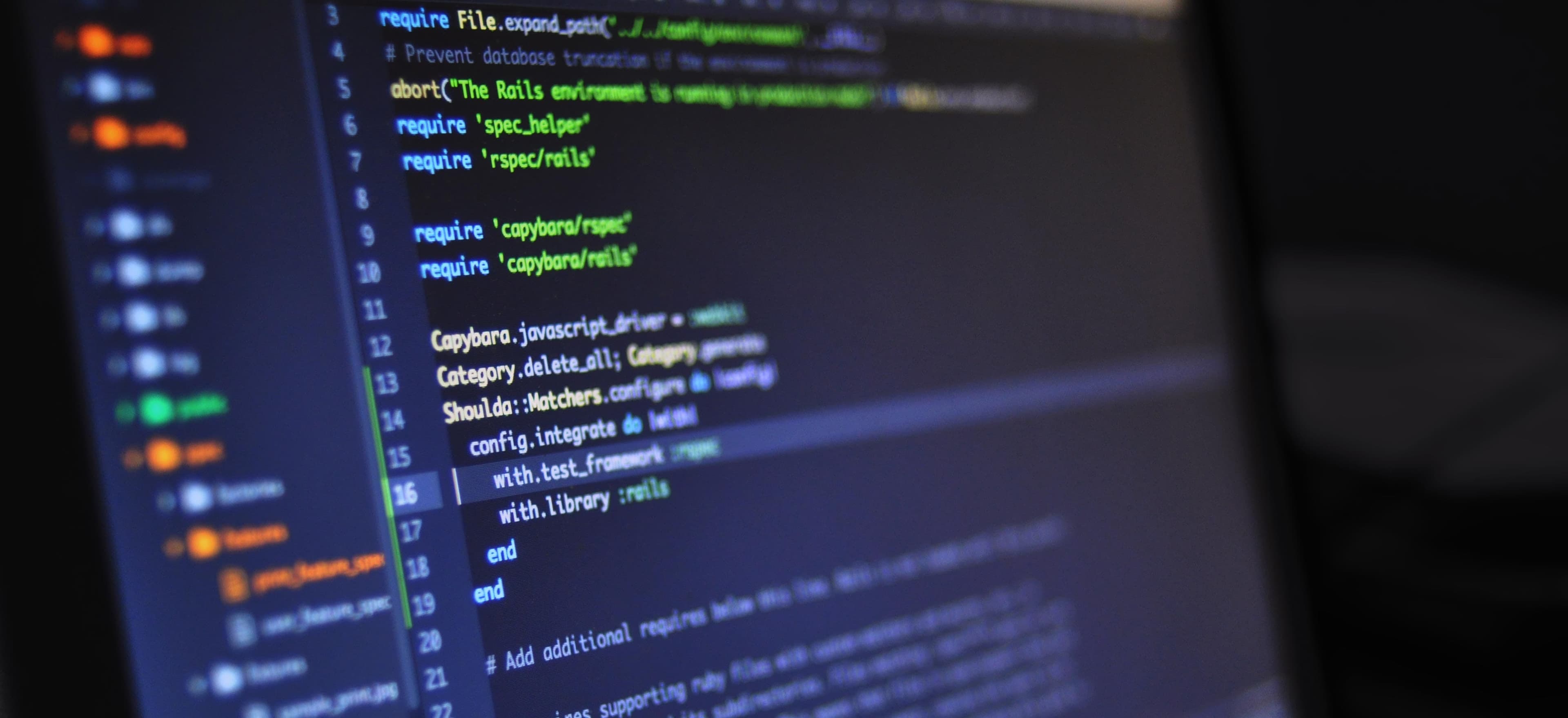
- Published on
Embracing Failure: The Key Fear for Aspiring Developers
In the fast-evolving world of technology, failure can often feel like a shadow looming over aspiring developers. Unlike success, failure tends to be stigmatized, creating an atmosphere of fear rather than one of growth. However, embracing failure is paramount for any developer striving to excel in their craft. In this blog post, we'll explore the significance of failure, dissect common fears associated with it, and provide actionable strategies to overcome these hurdles.
The Inevitability of Failure
First and foremost, it’s crucial to understand that failure is an integral part of the learning process. In programming, errors are not only common; they are expected. Every developer, no matter their level of experience, has encountered bugs and unexpected results. This journey often starts with a simple assignment or project. Hundreds of lines of code are written, only to be met with compilation errors or runtime exceptions.
The Programmer's Lifecycle
The process of programming is a continuous cycle of trying, failing, learning, and improving. Here’s a simple breakdown of this lifecycle:
- Planning: Define what you want to build.
- Development: Start coding based on your plans.
- Testing: Attempt to run your code and identify any problems.
- Debugging: Fix the issues your tests reveal.
- Learning: Understand why the mistakes occurred and how to avoid them in the future.
- Iteration: Use the insights gained to improve your work.
Each failure in this cycle serves as a stepping stone to mastery, showcasing the importance of a growth mindset.
The Fear Factor
So, what exactly creates this fear of failure among aspiring developers? Several factors may contribute:
-
Perfectionism: Many new developers feel the pressure to write perfect code and may avoid taking risks as a result. However, perfection is an elusive target. Instead of fearing mistakes, aim for progress.
-
Comparison: Social platforms and coding communities can create a sense of inadequacy when comparing your journey to others. Remember, every expert was once a beginner. Celebrate your unique path.
-
Imposter Syndrome: Many developers struggle with feeling like frauds despite their accomplishments. This can prevent them from seeking help or taking on ambitious projects.
-
Career Implications: The tech industry can be highly competitive. Some may fear that failure will hinder career advancement or job prospects. It’s vital to remember that companies value problem-solving skills—an attribute sharpened through failure.
Transforming Fear into Fuel
Failure can be a potent teacher when embraced correctly. Here are some strategies to turn fear into motivation and growth:
1. Adopt a Growth Mindset
A growth mindset recognizes that abilities can be cultivated through effort. Viewing failures as opportunities to learn encourages you to take risks without the fear of negative judgment.
Example: Rather than saying, “I’m bad at Java because I couldn’t solve this bug,” opt for, “I’m learning how to debug Java, and this failure will teach me how to improve.”
2. Build a Supportive Network
Surround yourself with fellow aspiring developers, mentors, or a supportive community that understands the challenges of coding. Sharing your fears and experiences can lessen the burden, making it clear that you are not alone.
- Local Meetups: Joining local programming meetups can help you connect with others in similar situations.
- Online Forums: Platforms like Stack Overflow or Dev.to are excellent for asking questions and learning from the experiences of others.
3. Practice Mindfulness
Mindfulness can help you detach from the fear of failure. Techniques like meditation can improve focus, reduce anxiety, and build resilience in the face of challenges.
4. Celebrate Small Wins
Instead of focusing solely on big goals, acknowledge and celebrate small wins. Every bug you fix, every new concept you master, is a step forward in your journey.
public class Greeting {
// Main method that serves as the entry point of the program
public static void main(String[] args) {
String name = "Developer";
System.out.println(createGreeting(name));
}
// A method that creates a greeting message
public static String createGreeting(String name) {
return "Hello, " + name + "! Embrace your coding journey, failures and all.";
}
}
In this code snippet, we use a method to create a personalized greeting, reinforcing that every line of code, whether successful or erroneous, contributes to a broader goal.
5. Learn from the Failures
Whenever you encounter an error or bug, take the time to understand what went wrong. This is a fundamental practice in coding.
public class Division {
public static void main(String[] args) {
int numerator = 10;
int denominator = 0; // This will lead to an exception
try {
System.out.println(divide(numerator, denominator));
} catch (ArithmeticException e) {
System.out.println("Caught an exception: " + e.getMessage());
}
}
public static int divide(int num, int den) {
return num / den; // This division will throw an exception if denominator is zero
}
}
In the example above, we simulate an arithmetic failure (division by zero). Instead of avoiding this scenario, we handle it using a try-catch block, demonstrating how to manage and learn from failures in a structured way.
Closing the Chapter
Embracing failure is not merely an encouraging notion; it is a transformative approach to personal and professional development. For aspiring developers, understanding that failures are stepping stones to success can pave the way for innovation, learning, and ultimately, expertise.
By adopting a growth mindset, building a supportive community, practicing mindfulness, celebrating small wins, and learning from each setback, developers can turn fear into a source of strength and resilience.
The path to becoming a proficient developer is riddled with challenges, but each failure brings us one step closer to mastery. So, the next time you hit a roadblock in your code, remember to embrace it. After all, it’s not about avoiding failure; it’s about learning to thrive in spite of it.
For more insights on navigating your coding journey, check out resources like Codecademy and FreeCodeCamp.
Happy Coding!
Checkout our other articles