Mastering Timeouts in Apache Ivy: A Guide to Resolvers
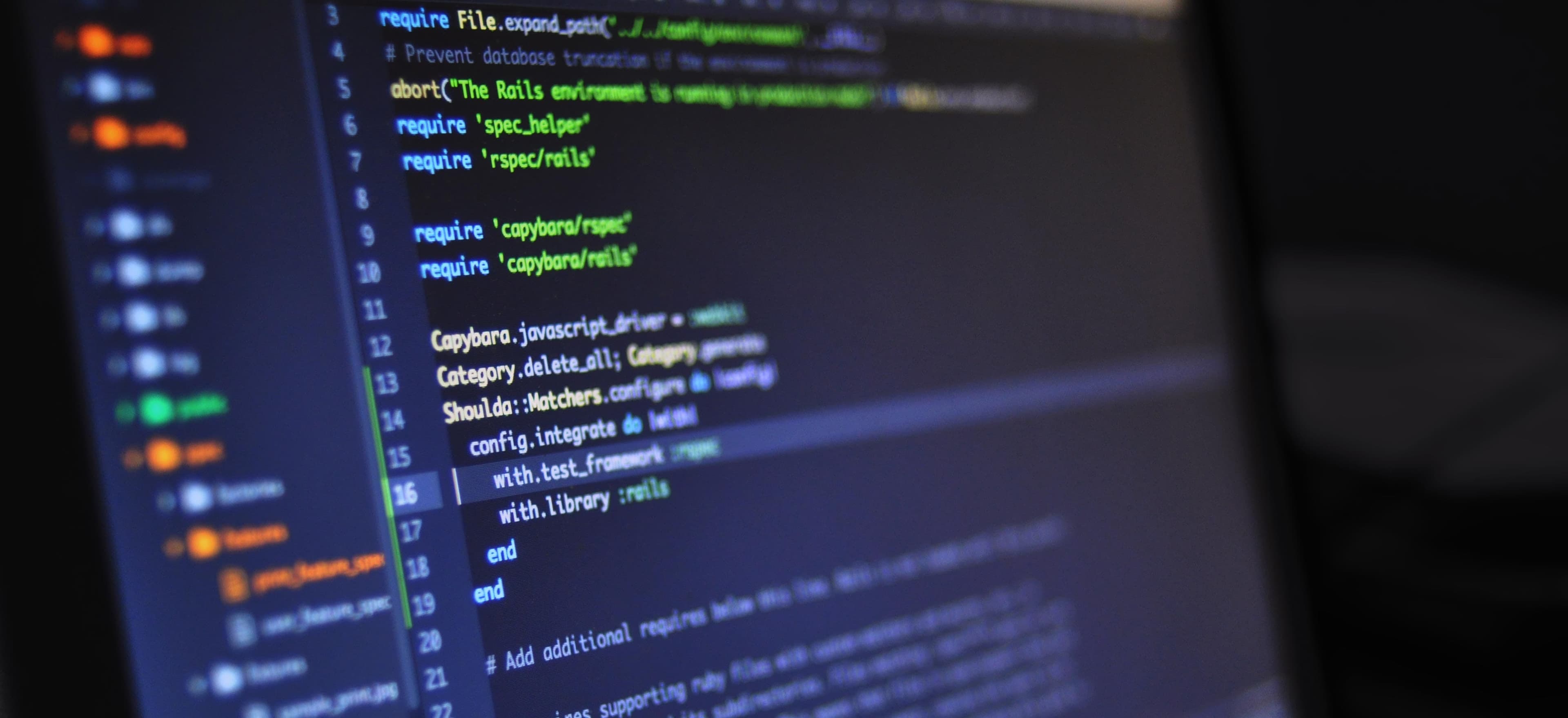
- Published on
Mastering Timeouts in Apache Ivy: A Guide to Resolvers
Apache Ivy is a powerful dependency management tool that integrates seamlessly with Apache Ant. It provides a flexible and advanced way to manage project dependencies. One of the critical aspects of using Ivy is configuring resolvers, which are responsible for retrieving dependencies from various sources like Maven repositories, Ivy repositories, or even custom repositories. Among the various configurations available, managing timeouts effectively can significantly enhance reliability, particularly in networks with variable performance.
In this blog post, we will dive deep into working with resolvers in Apache Ivy and demonstrate how to effectively set timeouts. We will walk through code snippets, configurations, and example use cases to ensure you have a solid grasp of this crucial feature.
Understanding Resolvers in Apache Ivy
A resolver is a configuration component in Ivy that specifies how and from where dependencies will be resolved. Different types of resolvers can be configured based on the source of dependencies. Some common resolvers include:
- Ivy Resolver: For Ivy-specific repositories.
- Maven Resolver: For Maven-based repositories.
- URL Resolver: For HTTP/HTTPS based repositories.
- File Resolver: For local file storage.
The Importance of Timeout Configurations
Timeout settings in resolvers enable you to control how long your application will wait for a response from a dependency repository before it gives up and retries or fails altogether. This is crucial for maintaining the stability of your build processes, especially when dependencies are fetched over network connections that can be slow or unreliable.
Setting Up a Basic Ivy Configuration
Before we configure timeouts, let's set up a basic Ivy configuration. Here is an example ivy.xml
file:
<ivy-module version="2.0">
<info organisation="com.example" module="myproject" />
<dependencies>
<dependency org="commons-lang" name="commons-lang" rev="2.6" />
</dependencies>
</ivy-module>
Ivy Settings (ivysettings.xml
)
To dictate how Ivy should resolve its dependencies, create an ivysettings.xml
file. Below is a simple example with a Maven resolver:
<ivy-settings>
<settings defaultResolver="maven-repo"/>
<resolvers>
<ibiblio name="maven-repo" m2compatible="true" root="https://repo1.maven.org/maven2/">
<!-- Configuring timeouts as parameters -->
<artifact pattern="[organisation]/[module]/[revision]/[artifact]-[revision](-[classifier]).[ext]" />
<!-- Timeout Settings -->
<timeout connect="5000" read="10000" />
</ibiblio>
</resolvers>
</ivy-settings>
Analyzing Timeout Configuration
In the above configuration, we define a Maven resolver named maven-repo
with some timeout settings. Let's break down the timeout parameters:
-
connect: These milliseconds define how long the resolver will wait while trying to connect to a repository before timing out. A value of 5000ms means it will wait a maximum of 5 seconds.
-
read: This defines the maximum time (in milliseconds) Ivy will wait to read data from the repository after the connection has been established. A value of 10000ms indicates a 10-second window.
Setting the connect and read timeouts appropriately will help ensure that your dependency resolution process does not hang indefinitely during slow network conditions.
Example of Custom Resolver with Timeout
Let's say you have a custom HTTP repository for your internal dependencies. Here’s how to set it up with timeout configurations:
<ivy-settings>
<settings defaultResolver="internal-repo"/>
<resolvers>
<url name="internal-repo" m2compatible="true" root="https://internal-repo.example.com/artifacts/">
<artifact pattern="[organisation]/[module]/[revision]/[artifact]-[revision](-[classifier]).[ext]" />
<!-- Custom Timeouts -->
<timeout connect="7000" read="15000" />
</url>
</resolvers>
</ivy-settings>
Using the Configured Resolvers
Now that you have configured a resolver with timeouts in ivysettings.xml
, you need to execute the dependency resolution process. You can do this through an Ant build script. Here is a sample build script that uses the Ivy configuration:
<project name="MyProject" default="resolve" xmlns:ivy="ant:org.apache.ivy.ant">
<ivy:retrieve pattern="lib/[artifact]-[revision].[ext]" />
<target name="resolve">
<ivy:resolve />
</target>
</project>
Best Practices for Timeout Configuration
-
Analyze Network Performance: When setting timeout values, always assess the performance of your network. Avoid setting values that are too low, as this could lead to premature failures.
-
Incremental Changes: Start with conservative timeout values and make incremental adjustments based on your build history and network performance.
-
Monitor Performance: Use monitoring tools to track the success rate of dependency resolutions. Adjust your settings based on observed issues.
-
Fallback Resolvers: Consider configuring fallback resolvers in case the primary resolver fails. This can be particularly useful when dealing with external repositories.
Debugging Timeout Issues
If you find you are consistently running into timeout issues, there are several ways to investigate and troubleshoot:
-
Increase Timeout: Start by increasing the configured timeout values to see if the issues persist.
-
Check Network Connectivity: Ensure that you can access the repository URLs using tools like
curl
orping
. -
Review Logs: Ivy logs detailed information on dependency resolution. Pay close attention to these logs to identify what might be causing the delays.
-
Update Ivy: Always ensure you are using the latest version of Apache Ivy, as performance improvements and bug fixes may be available.
The Bottom Line
Managing resolvers and their timeout settings is an essential part of using Apache Ivy effectively. Proper configuration represents a significant step toward ensuring stable and reliable build processes. Whether you are using Ivy with Maven repositories, custom resolvers, or a combination of both, employing an understanding of timeout mechanics will safeguard your projects from unexpected network issues.
For further reading on Ivy and its configurations, check out the official Apache Ivy documentation.
Now, it's your turn to implement and experiment with timeout configurations in Apache Ivy. By mastering this aspect, you're well on your way to a more robust dependency management strategy!
Checkout our other articles