Bridging the Gap: Managers vs. Individual Contributors in Code
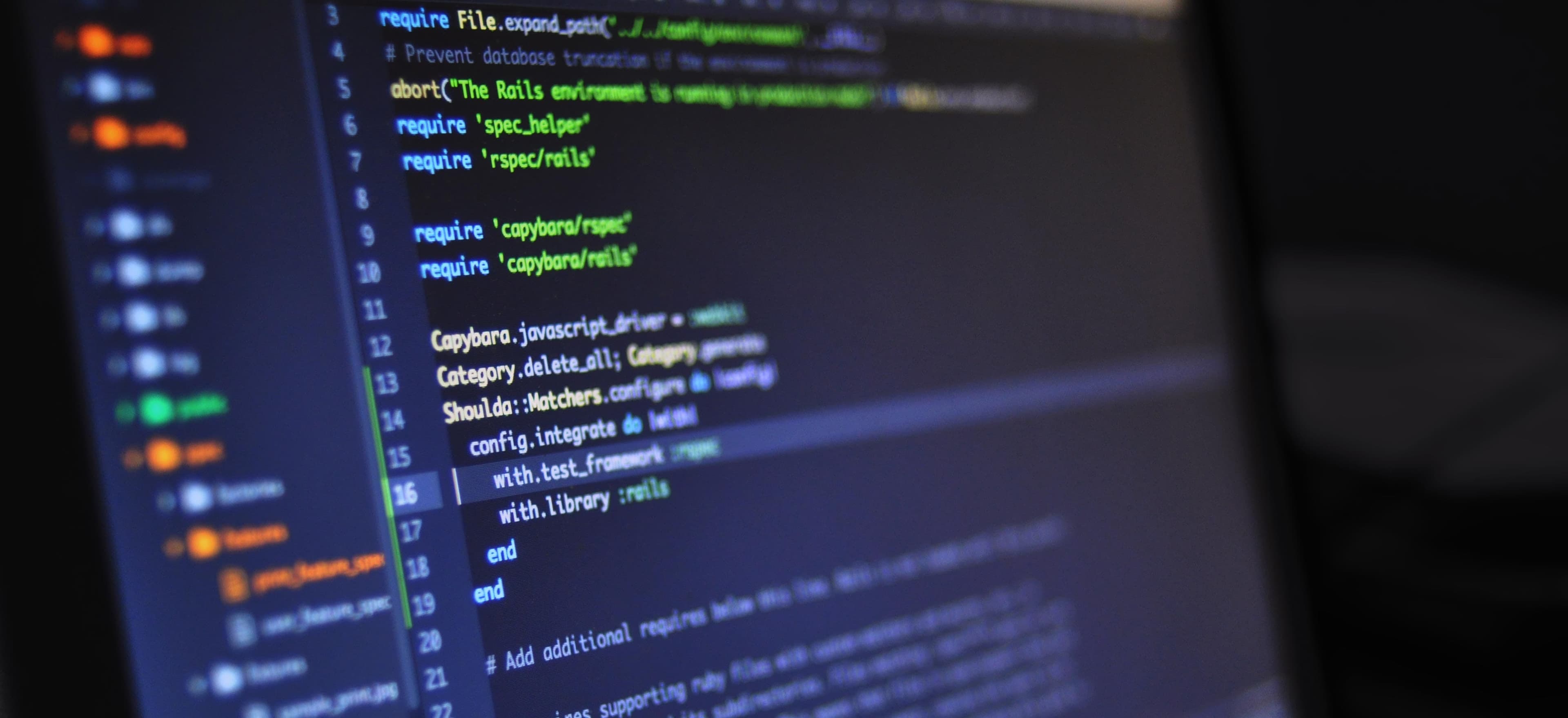
- Published on
Bridging the Gap: Managers vs. Individual Contributors in Code
In the fast-evolving technology landscape, the collaboration between managers and individual contributors (ICs) is crucial for the successful delivery of projects. This post aims to explore the distinct roles of managers and individual contributors within software development, particularly focusing on how each can lead to better code quality and, ultimately, successful project outcomes.
Table of Contents
- Understanding Roles
- Challenges Faced
- Fostering Collaboration
- Code Quality and Best Practices
- Tools for Effective Communication
- Conclusion
Understanding Roles
Managers
In software development, managers are responsible for overseeing projects, while also ensuring that team members have the resources they need to succeed. They take on a variety of responsibilities:
- Project Management: Managers plan timelines, allocate resources, and monitor progress.
- Team Leadership: They motivate and mentor team members, helping to foster an environment focused on growth and learning.
- Stakeholder Communication: Managers act as a bridge between the technical team and stakeholders, ensuring that expectations are effectively managed.
Individual Contributors
On the other hand, individual contributors are the technical hands-on specialists who write the code and build the product. Their responsibilities include:
- Coding: ICs transform requirements into functional code.
- Problem-Solving: They diagnose, troubleshoot, and resolve issues during the development process.
- Collaboration: ICs often work in tandem with other team members, reviewing code and providing feedback.
Challenges Faced
Both managers and ICs face unique challenges that can heighten the tension between them. Here are some common hurdles:
-
Communication Gaps: Sometimes, managers may lack a technical background, which can lead to misunderstandings about project challenges. Similarly, ICs may not always understand management concerns around timelines and resources.
-
Different Metrics for Success: Managers often measure success by project timelines, while ICs might focus on code quality and technical challenges. This divergence can lead to conflicting priorities.
-
Time Allocation: Managers might prioritize meetings and documentation, while ICs may prefer to spend time coding. Balancing these approaches requires understanding and compromise.
Fostering Collaboration
To bridge the gap between managers and individual contributors, companies must foster a culture of collaboration. Here are a few strategies to consider:
-
Regular Check-ins: Hold weekly or bi-weekly meetings where both managers and ICs can share updates, challenges, and feedback. This builds a better understanding of each other's perspectives and priorities.
-
Encourage Feedback: Managers should solicit continuous feedback from ICs on project processes and alleviating bottlenecks. This helps to create a culture of open communication.
-
Involve ICs in Planning: Including ICs in the project planning phase can provide valuable insights. Developers have firsthand knowledge of technical challenges that can impact timelines, and they appreciate having their input valued.
Code Quality and Best Practices
Quality code is essential for the success of any software project. Both managers and individual contributors can play a role in ensuring code quality through best practices.
Establishing Coding Standards
Using consistent coding standards helps maintain clarity and ease of maintenance throughout a project. Below is an example of a simple Java class illustrating best practices:
public class User {
private String username;
private String email;
public User(String username, String email) {
this.username = username;
this.email = email;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
return "User{" +
"username='" + username + '\'' +
", email='" + email + '\'' +
'}';
}
}
Comments:
- Encapsulation: The private variables ensure that data is only accessible through getter and setter methods.
- Constructor Initialization: The constructor uses mandatory parameters to initialize a
User
object, ensuring that crucial information is provided upon creation. - toString() Method: Overriding the
toString()
method adds clarity when logging user information, making it easier to debug.
Code Reviews
Encouraging code reviews promotes collaboration and enhances code quality. Each review provides an opportunity to share knowledge and identify potential pitfalls.
public class PasswordValidator {
public boolean isValid(String password) {
if (password.length() < 8) {
return false; // Password too short
}
return password.chars().anyMatch(Character::isUpperCase)
&& password.chars().anyMatch(Character::isLowerCase)
&& password.chars().anyMatch(Character::isDigit); // Check for upper, lower, and digit
}
}
Comments:
- Early Return Pattern: The method checks password length first to quickly exit invalid cases, promoting efficient coding practices.
- Stream API Usage: Using Java 8's Stream API for concise checks demonstrates modern Java programming approaches.
Tools for Effective Communication
Technology plays a key role in bridging gaps between managers and individual contributors. Various tools can facilitate communication and project management:
-
Project Management Tools: Tools like JIRA or Trello allow both managers and ICs to visualize task progress and priorities.
-
Version Control Systems: Using Git and platforms like GitHub promotes collaboration by allowing code-sharing and version control among team members.
-
Collaborative Documentation: Maintain shared documentation on platforms like Confluence to ensure that both managers and ICs have access to essential project information.
Bringing It All Together
The relationship between managers and individual contributors is vital in software development. While both roles come with unique responsibilities and challenges, fostering effective communication and collaboration can create a harmonious environment conducive to success.
By embracing coding standards, instituting regular check-ins, and employing effective tools for communication, organizations can alleviate discrepancies and achieve seamless integration between management and technical teams.
Ultimately, when managers and individual contributors work together toward common goals, they bridge the gap between strategy and execution, ensuring that high-quality code leads to successful products.
If you have any strategies or experiences that have helped you bridge this gap in your own work environment, feel free to share in the comments!
Checkout our other articles