Compiler vs Interpreter: The Performance Dilemma Explained
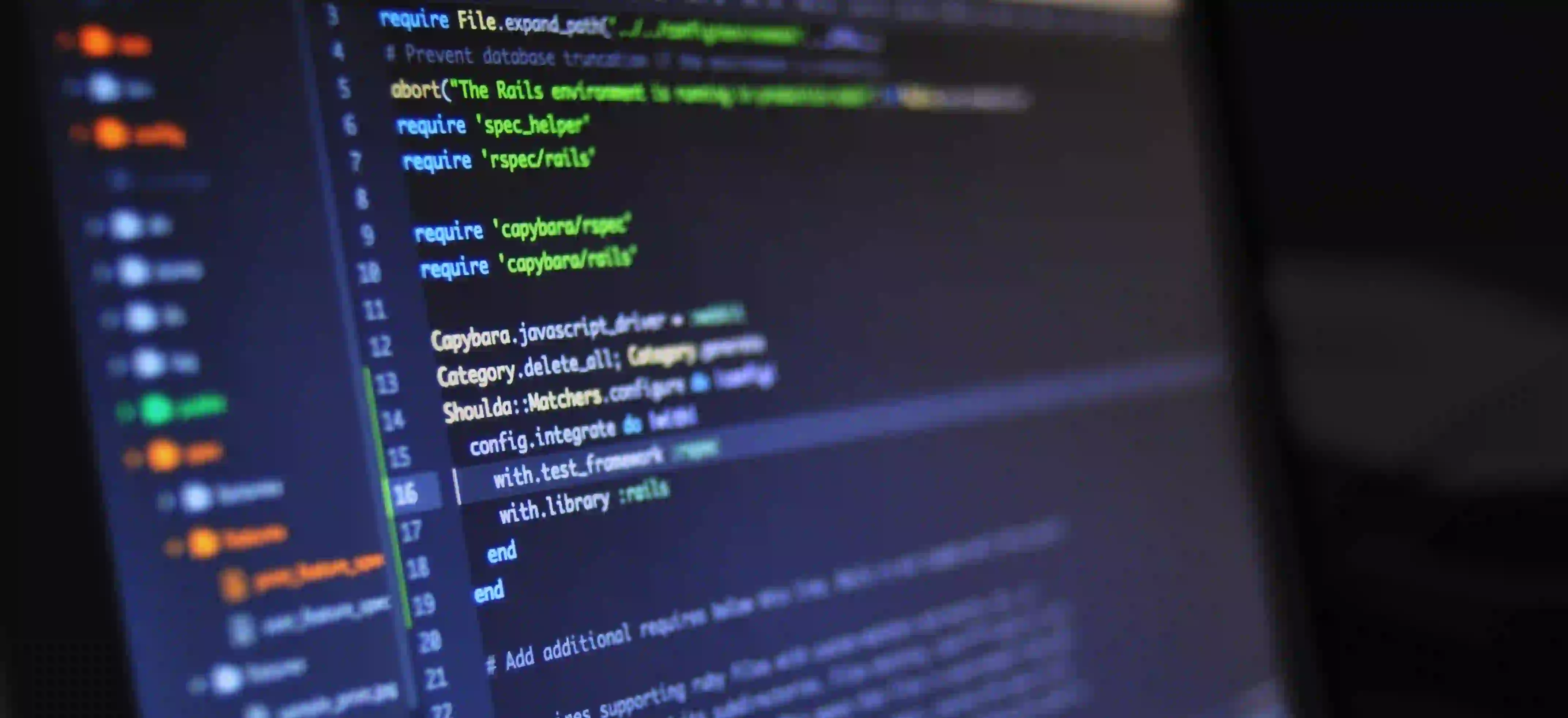
Compiler vs Interpreter: The Performance Dilemma Explained
When it comes to programming languages, two fundamental types of translation mechanisms often come into play: compilers and interpreters. Both serve the crucial function of translating high-level programming code into machine code that a computer can understand. However, they do so in distinctly different manners, which affects performance, development speed, and debugging processes. In this post, we will examine the major differences between compilers and interpreters, their performance implications, and when to use each.
What is a Compiler?
A compiler translates an entire high-level programming program (source code) into machine code at once. Once this translation is complete, the resulting executable file can run independently and repeatedly without needing the original source code or compiler.
Benefits of Using a Compiler
-
Performance:
- Executables created by compilers generally run faster than interpreted code since the translation is done beforehand.
- This is particularly important for performance-critical applications.
-
Error Checking:
- A compiler performs a thorough syntax analysis before producing machine code.
- This means many potential bugs can be caught at compile-time rather than at runtime.
-
Optimization:
- Compilers offer various levels of optimization, allowing the developer to fine-tune performance.
Example: Java Compiler
In Java, the source code is first compiled into an intermediate bytecode using the Java Compiler (javac
):
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
To compile this code, you would run:
javac HelloWorld.java
This generates a HelloWorld.class
file, which contains bytecode executable by the Java Virtual Machine (JVM).
What is an Interpreter?
An interpreter translates high-level programming code into machine code line-by-line or statement-by-statement. It executes the source code directly without producing a separate executable.
Benefits of Using an Interpreter
-
Ease of Debugging:
- Errors are caught one line at a time, making it easier to locate and fix issues in the code.
- This is ideal during development for quick iterations and testing.
-
Platform Independence:
- Interpreted languages often run on different platforms without the need for recompilation since they rely on an interpreter compatible with that platform.
Example: Python Interpreter
Python is a prime example of an interpreted language. Consider the same "Hello, World!" program:
print("Hello, World!")
To run this code, simply execute it through the Python interpreter:
python3 hello_world.py
As the interpreter processes one line at a time, any error that occurs will immediately halt execution, allowing for rapid troubleshooting.
Compilers vs Interpreters: Performance Comparison
The choice between a compiler and an interpreter can significantly impact performance, especially for extensive applications.
-
Execution Speed:
- Compiled code typically executes much faster than interpreted code due to pre-translated instructions.
- However, the initial compilation phase can take time, making it slower during the development phase.
-
Memory Usage:
- Compiled programs can be more memory-efficient than their interpreted counterparts, which may need to hold the entire source code in memory during execution.
-
Deployment Considerations:
- Compiled programs create a standalone executable, allowing for simpler distribution.
- In contrast, interpreted programs require the presence of the interpreter on the target machine, adding an additional layer of dependency.
Real-World Use Cases
When to Use a Compiler
-
Performance-Critical Applications: Applications like video games and graphic processing software benefit from compiled languages like C or C++.
-
System-Level Programming: Operating systems and drivers require optimal performance, hence compiled languages are commonly utilized.
When to Use an Interpreter
-
Rapid Development: Languages like Python and Ruby allow for quick iterations and immediate feedback, making them ideal for web development and scripting tasks.
-
Cross-Platform Development: Interpreters allow the same codebase to run on various operating systems without modification.
Combining Both Approaches: The JVM
Java is unique in its approach to combining compilation and interpretation. As mentioned earlier, Java code is first compiled into bytecode. This bytecode is then interpreted by the JVM, allowing for a balance between performance and platform independence.
The JVM also optimizes performance with techniques like Just-In-Time (JIT) compilation, where frequently executed bytecode segments are compiled into machine code during runtime.
This dual approach shines a light on the possibilities of combining the strengths of both compilers and interpreters, and highlights a growing trend in modern programming languages.
To Wrap Things Up: Making the Right Choice
In the enduring debate of compilers vs interpreters, the choice ultimately hinges on specific project needs. Performance-critical applications lean towards compilers, while ease of use and rapid debugging favors interpreters.
As technology evolves, so do these translation mechanisms. Understanding when and how to leverage each can significantly impact the functionality and efficiency of your application. For developers, staying informed about these differences is essential not just for choosing a programming language, but also for optimizing the workflow.
For more information on programming languages and development strategies, check out W3Schools and GeeksforGeeks.
By leveraging both the strategic advantages of compilers and interpreters, developers can enhance their workflows and build more efficient, robust software solutions.