Avoiding Deadlocks: The Concurrency Bug You Can Fix Today
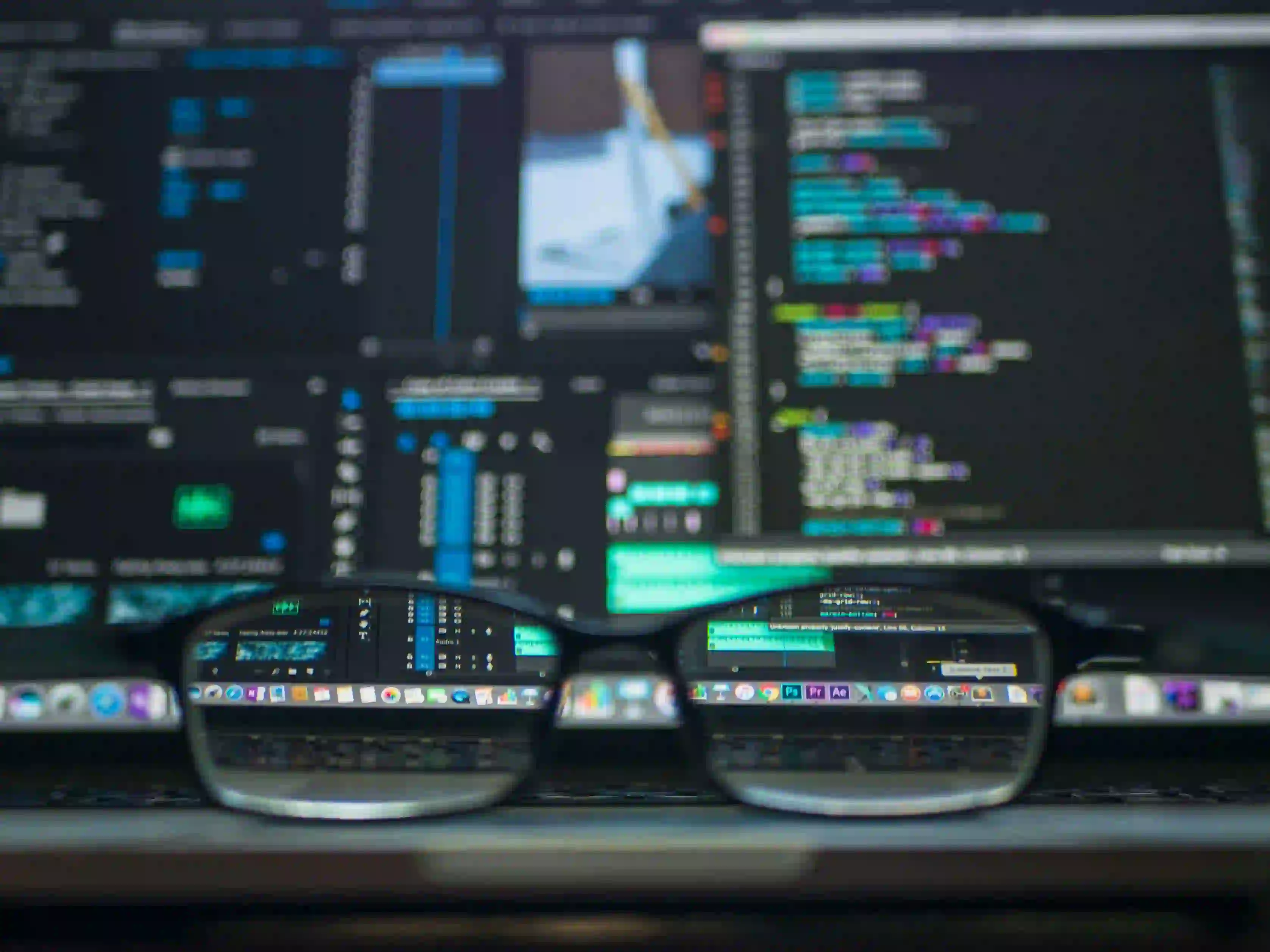
Avoiding Deadlocks: The Concurrency Bug You Can Fix Today
Deadlocks are one of the most challenging issues developers face when building concurrent applications. They occur when two or more threads are waiting indefinitely for resources held by each other. In this blog post, we will explore what deadlocks are, why they happen, and how to avoid them using best practices and effective coding strategies in Java.
What is a Deadlock?
A deadlock can be defined as a state in a multi-threaded environment where two or more processes cannot proceed because each is waiting for the other to release a resource. Imagine two threads:
- Thread 1 locks Resource A and wants to lock Resource B.
- Thread 2 locks Resource B and wants to lock Resource A.
Since neither thread can proceed without acquiring the resource held by the other, both become blocked, and the application hangs.
Why Do Deadlocks Occur?
Deadlocks often arise due to:
- Mutual Exclusion: At least one resource must be held in a non-shareable mode. If another thread requests that resource, it must be blocked until the resource is released.
- Hold and Wait: A thread is holding at least one resource and is waiting to acquire additional resources that are currently being held by other threads.
- No Preemption: Resources cannot be forcibly removed from a thread holding them until the resource is voluntarily released.
- Circular Wait: There exists a set of threads, each of which is waiting for a resource held by the next thread in the cycle.
Understanding these conditions is key to devising a solution.
Strategies to Avoid Deadlocks
Now that we’ve established what deadlocks are and how they occur, let’s discuss some effective strategies to avoid them.
1. Lock Ordering
One of the simplest approaches to avoid deadlocks is to enforce a strict order in acquiring locks. By ensuring that all threads acquire locks in the same order, you can prevent the circular wait condition from occurring.
Example Code:
public class LockOrderingExample {
private final Object resourceA = new Object();
private final Object resourceB = new Object();
public void method1() {
synchronized (resourceA) {
System.out.println("Locked resource A");
synchronized (resourceB) {
System.out.println("Locked resource B");
}
}
}
public void method2() {
synchronized (resourceA) {
System.out.println("Locked resource A");
synchronized (resourceB) {
System.out.println("Locked resource B");
}
}
}
}
Why this works: Both method1
and method2
acquire resourceA
before resourceB
, ensuring that circular waiting doesn't occur.
2. Use Timeouts
Implementing timeouts when trying to acquire locks can be a practical method to avoid deadlocks. If a thread cannot acquire a lock within a defined timeout period, it can back off and attempt to release held resources.
Example Code:
public class TimeoutExample {
private final Object resourceA = new Object();
private final Object resourceB = new Object();
public void methodWithTimeout() {
boolean aLocked = false;
boolean bLocked = false;
try {
aLocked = tryLock(resourceA, 1000); // Try to lock resource A
bLocked = tryLock(resourceB, 1000); // Try to lock resource B
} finally {
if (aLocked) {
releaseLock(resourceA);
}
if (bLocked) {
releaseLock(resourceB);
}
}
}
private boolean tryLock(Object resource, long timeout) {
// Simulated acquire with timeout logic here
return true; // placeholder
}
private void releaseLock(Object resource) {
// Simulated unlock logic here
}
}
Why this works: By allowing the thread to back out after a timeout, it reduces the chances of getting stuck perpetually waiting for a resource.
3. Use Higher-Level Concurrency Utilities
Java provides higher-level concurrency utilities in the java.util.concurrent
package. These utilities, like ReentrantLock
, CountDownLatch
, or Semaphore
, often incorporate solutions to avoid deadlocks inherently.
Example Code:
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class ReentrantLockExample {
private final Lock lockA = new ReentrantLock();
private final Lock lockB = new ReentrantLock();
public void methodA() {
try {
if (lockA.tryLock() && lockB.tryLock()) {
System.out.println("Acquired resources A and B");
}
} finally {
// ensure locks are released if held
if (lockA.isHeldByCurrentThread()) lockA.unlock();
if (lockB.isHeldByCurrentThread()) lockB.unlock();
}
}
}
Why this works: tryLock
allows threads to attempt to acquire locks without indefinitely blocking if the locks are not available, thus avoiding deadlocks.
4. Deadlock Detection and Recovery
In some situations, it may be appropriate to implement a detection algorithm that identifies circular dependencies in threads and resources. Upon detection, the system can safely terminate one or more processes to resolve the deadlock.
- Algorithms for deadlock detection usually involve constructing a resource allocation graph.
- If a cycle is detected, you can choose to preempt resources from one or more waiters.
Note: This method can be complex and is used primarily in critical systems where high availability is essential.
Bringing It All Together
Deadlocks can pose significant challenges in concurrent programming, but understanding them and employing purposeful coding strategies can lead to sound solutions. By practicing lock ordering, implementing timeouts, leveraging high-level concurrency utilities, and considering detection and recovery mechanisms, you can build robust Java applications that are resilient against deadlocks.
Further Reading:
By staying aware of these practices while developing, you can prevent deadlocks and ensure that your applications run smoothly and efficiently. Happy coding!