Common Mistakes in Spring MVC Hibernate CRUD Implementation
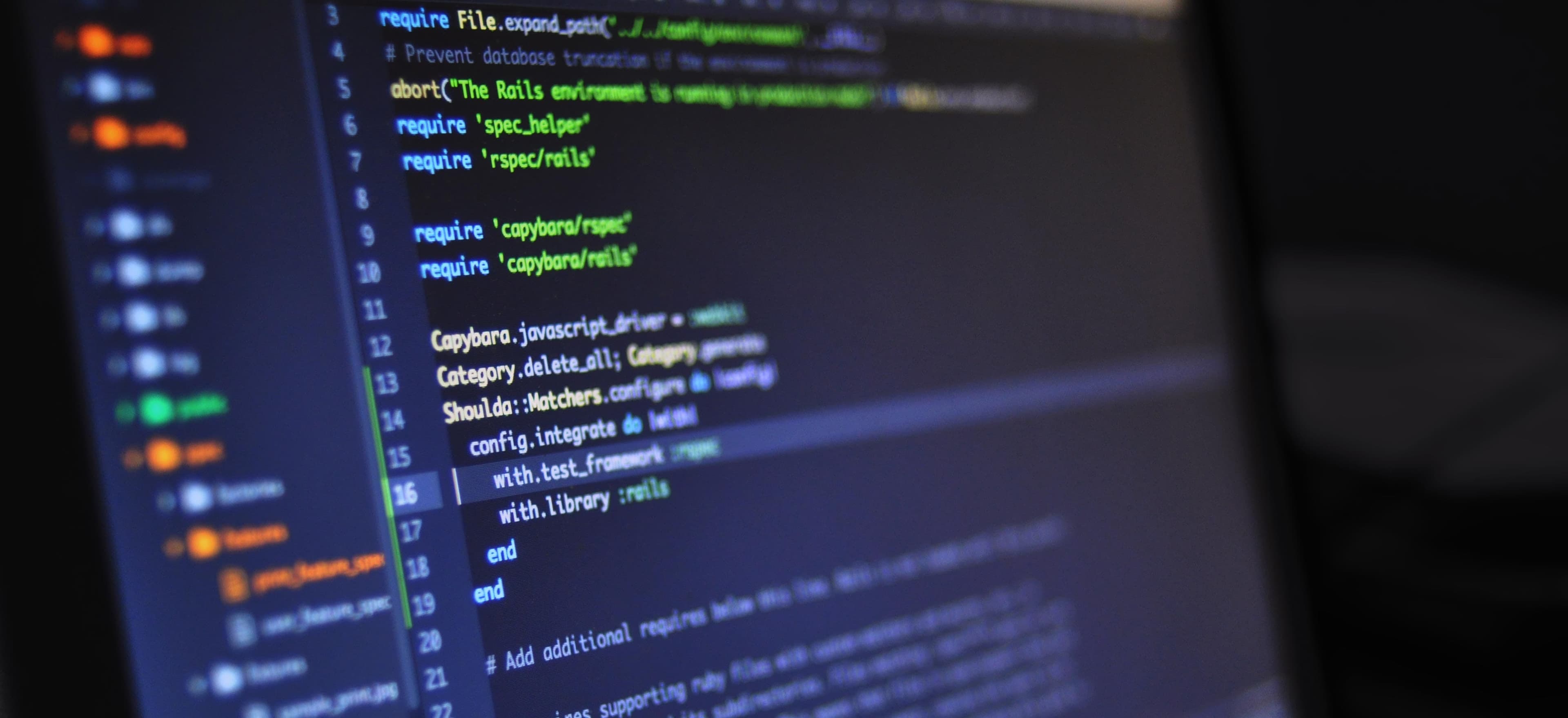
- Published on
Common Mistakes in Spring MVC Hibernate CRUD Implementation
Spring MVC and Hibernate are quintessential technologies in the Java ecosystem, and when combined, they create powerful web applications. However, navigating the implementation of CRUD (Create, Read, Update, Delete) operations using these frameworks can be rife with pitfalls. This post will elucidate the common mistakes developers make when integrating Spring MVC with Hibernate and provide best practices to avoid these errors.
1. Ignoring Dependency Management
Mistake
One of the primary mistakes is neglecting dependency management within your project. This often leads to version mismatches between Spring, Hibernate, and other libraries, resulting in compatibility issues.
Solution
Utilize a build management tool like Maven or Gradle. This way, you can manage dependencies efficiently and also scope their versions. Here’s an example of how to set up dependencies in Maven:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.9</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.32.Final</version>
</dependency>
<dependency>
<groupId>javax.transaction</groupId>
<artifactId>javax.transaction-api</artifactId>
<version>1.3</version>
</dependency>
By adhering to this configuration, you can preemptively tackle issues related to library compatibility.
2. Incorrect Database Configuration
Mistake
Not configuring the database properties properly can lead to connection failures or inefficiencies in data transactions.
Solution
Make sure your application.properties
or application.yml
file is configured correctly. Here’s a sample configuration:
spring.datasource.url=jdbc:mysql://localhost:3306/your_database
spring.datasource.username=root
spring.datasource.password=password
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5Dialect
The properties ddl-auto
and show-sql
help with development and debugging, while the dialect is essential for translating SQL commands compatible with your database.
3. Poorly Defined Entity Classes
Mistake
A common oversight occurs when developers define entity classes without adequately specifying relationships, leading to lazy loading issues or incorrect data fetching.
Solution
Define your entities with clear annotations and relationships. Here’s an example of a simple User
entity:
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String username;
@Column(nullable = false)
private String password;
// Getters and Setters
}
This code snippet defines a clean entity with essential annotations that Hibernate can interpret for database mapping.
4. Not Handling Transactions Properly
Mistake
Failing to handle transactions properly can result in data inconsistency and application errors. For instance, not using @Transactional
in service methods can lead to unexpected behavior.
Solution
Use the @Transactional
annotation effectively. For example:
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void createUser(User user) {
userRepository.save(user);
}
}
By annotating the method with @Transactional
, you ensure that the transaction management is handled automatically, creating a safer execution context for your operations.
5. Inefficient Fetching Strategies
Mistake
Using default fetching strategies can lead to performance bottlenecks, particularly with large datasets. Many developers overlook the importance of settings like FetchType.LAZY
and FetchType.EAGER
.
Solution
Define fetching strategies explicitly within your entity relationships. Here’s how you can do that:
@Entity
public class Post {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long postId;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "user_id", nullable = false)
private User user;
// Getters and Setters
}
In this case, setting fetch = FetchType.LAZY
ensures that the associated user is fetched only when needed, which can significantly enhance performance.
6. Lack of Exception Handling
Mistake
Many developers fail to implement proper exception handling in their CRUD operations, leading to uncaught exceptions and poor user experience.
Solution
Encapsulate your logic in try-catch
blocks. You can create a custom exception handler:
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception exception) {
return new ResponseEntity<>(exception.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
This creates a centralized way to manage exceptions, reducing redundancy and improving maintainability.
7. Not Validating Input Data
Mistake
Neglecting to validate input data before processing can lead to data integrity issues. Failing to ensure that the incoming data adheres to the expected format can result in challenges later on.
Solution
Use validation annotations in your entity class along with the @Valid
annotation in your controller. An example is shown below:
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
public class User {
@NotNull
@Size(min = 2, message = "Username should be at least 2 characters")
private String username;
// Other fields
}
By applying constraints, you can avoid potential failures and ensure data integrity right from the start.
Closing the Chapter
Implementing CRUD operations with Spring MVC and Hibernate is a rewarding experience when done correctly. By avoiding common pitfalls such as poor dependency management, incorrect database configuration, or lack of validation, you can create a robust application that delivers a seamless user experience.
Leveraging best practices, such as effective transaction management and strategic entity relationships, will not only enhance your application's performance but also its maintainability. For additional context on Spring and Hibernate integrations, feel free to explore Spring Documentation and Hibernate Documentation.
Ultimately, understanding these common mistakes allows developers to build more resilient applications that are scalable and efficient. Happy coding!
Checkout our other articles