How to Prevent Your App from Auto-Closing Old Box Objects
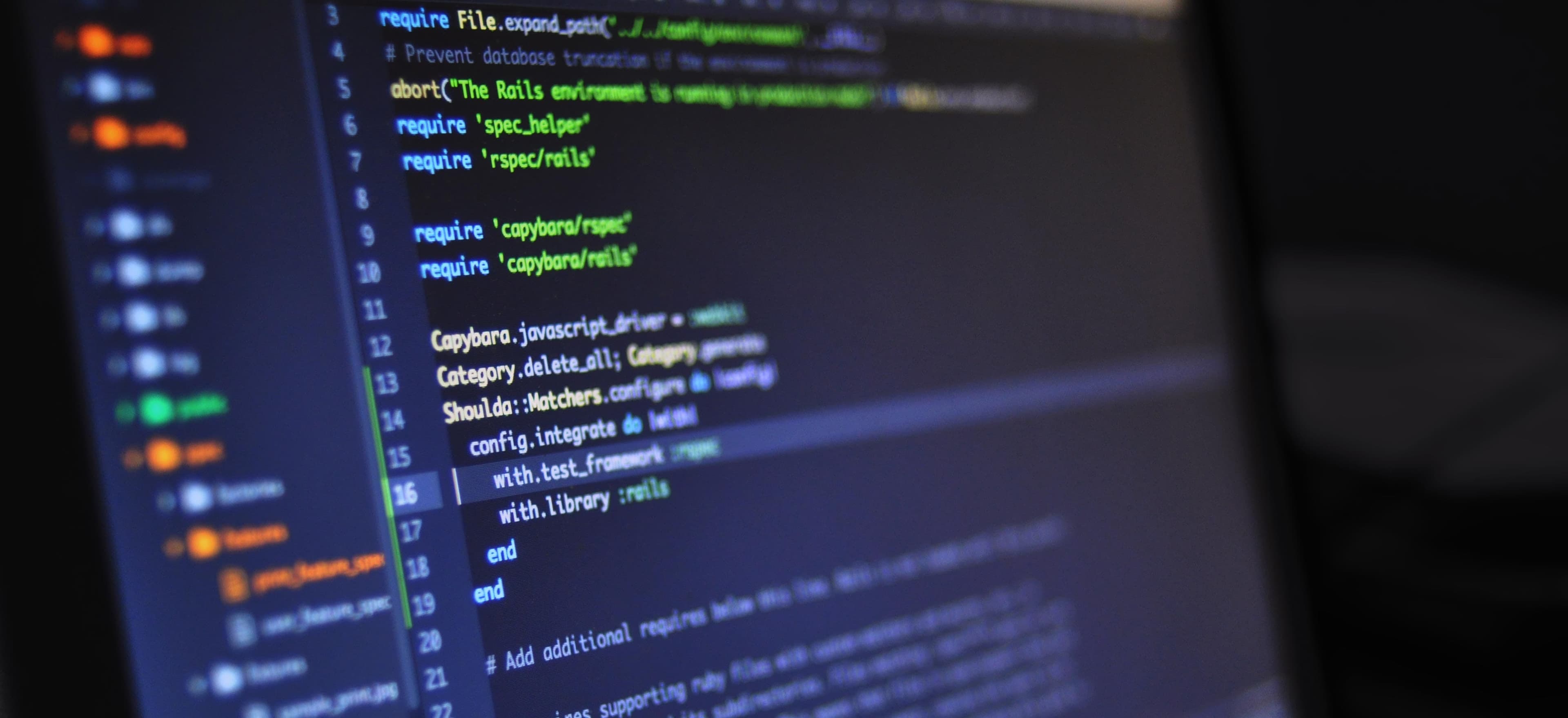
- Published on
How to Prevent Your App from Auto-Closing Old Box Objects in Java
Java, as a versatile programming language, provides developers with a range of features to manage memory effectively. One common concern while working with box objects (such as Optional
, Box
, or custom container classes) is ensuring that they don't get auto-closed or garbage collected prematurely. In this blog post, we will delve into understanding what box objects are, why they might get auto-closed, and how to manage their lifecycle effectively in your application.
Understanding Box Objects in Java
In Java, box objects typically refer to wrapper classes that encapsulate primitive types. For instance, instead of using an int
, you can use Integer
. This encapsulation allows you to treat primitives as objects, which is necessary in many scenarios, particularly in collections:
Integer boxedInt = Integer.valueOf(42);
Moreover, recent Java versions introduced the Optional
class, designed to handle potentially null values more effectively and prevent NullPointerExceptions
. Here’s a simple example:
Optional<String> optionalString = Optional.of("Hello, World");
However, these box objects may face issues with premature closure or being garbage-collected. This can happen when there are no more references to the object, leading to unexpected results or application crashes.
Why Do Box Objects Get Auto-Closed?
Box objects can be auto-closed due to various factors:
-
Scope Limitations: When box objects are defined inside a method, they will be eligible for garbage collection once the method execution is complete, and there are no more references to them.
-
Weak References: If you use weak references to hold box objects, they can be collected by the garbage collector at any time. This is suitable for caching but unsuitable for persistent states.
-
Lack of Lifecycle Management: If you do not explicitly manage the lifecycle of your box objects (e.g., keeping references in long-lived collections), they can vanish unexpectedly.
Understanding this behavior is key to preventing your application from mismanaging these objects.
Best Practices to Prevent Auto-Closing of Box Objects
1. Maintain Strong References
The simplest way to prevent box objects from being auto-closed is to maintain strong references to them as long as needed.
import java.util.ArrayList;
import java.util.List;
public class ReferenceKeeper {
private List<Integer> boxObjects = new ArrayList<>();
public void addBoxObject(int value) {
boxObjects.add(value); // Strong reference is maintained
}
public List<Integer> getBoxObjects() {
return boxObjects;
}
}
In this example, the ReferenceKeeper
class holds strong references to Integer
objects. As long as the ReferenceKeeper
instance exists, the box objects are preserved.
2. Utilize Singleton Pattern for Long-Lived Objects
If your application needs certain box objects throughout its lifecycle, consider the Singleton pattern. This design restricts the instantiation of a class to one object, ensuring that the objects persist.
public class SingletonBox {
private static SingletonBox instance;
private Integer boxObject;
private SingletonBox() {
// private constructor
}
public static SingletonBox getInstance() {
if (instance == null) {
instance = new SingletonBox();
}
return instance;
}
public void setBoxObject(int value) {
this.boxObject = value;
}
public Integer getBoxObject() {
return boxObject;
}
}
Here, SingletonBox
maintains its box object as long as the application runs.
3. Using Java Collections
Java Collections such as ArrayList
, HashMap
, or custom data structures are excellent for managing box objects' lifecycles. You can safely store your box objects in these collections to prevent them from closing prematurely.
import java.util.HashMap;
import java.util.Map;
public class BoxManager {
private Map<String, Integer> boxMapping = new HashMap<>();
public void addBox(String key, int value) {
boxMapping.put(key, value); // Keeps box object referenced
}
public Integer getBox(String key) {
return boxMapping.get(key);
}
}
4. Implement Reference Counting
For scenarios where box objects may have multiple users, consider implementing a reference counting mechanism. This allows you to track how many references exist for a given object and only release it when no references are left.
import java.util.HashMap;
import java.util.Map;
public class RefCountBox {
private Map<Integer, Integer> boxRefCount = new HashMap<>();
public void addBox(int value) {
boxRefCount.put(value, boxRefCount.getOrDefault(value, 0) + 1);
}
public void releaseBox(int value) {
if (boxRefCount.containsKey(value)) {
int count = boxRefCount.get(value);
if (count <= 1) {
boxRefCount.remove(value); // remove if no references left
} else {
boxRefCount.put(value, count - 1);
}
}
}
}
In this implementation, RefCountBox
tracks the number of references to each box object, managing their lifecycle based on usage.
5. Use SoftReferences or WeakHashMap for Caching
In particular scenarios, such as caching, you can utilize SoftReference
or WeakHashMap
to allow the garbage collector to reclaim objects when memory is low, while still offering control over their lifecycle.
import java.lang.ref.SoftReference;
import java.util.HashMap;
import java.util.Map;
public class SoftBoxCache {
private Map<String, SoftReference<Integer>> cache = new HashMap<>();
public void put(String key, int value) {
cache.put(key, new SoftReference<>(value));
}
public Integer get(String key) {
SoftReference<Integer> ref = cache.get(key);
return (ref != null) ? ref.get() : null; // Returns null if object has been collected
}
}
In this caching mechanism, SoftReference
allows you to create a cache that the garbage collector can reclaim if necessary, reducing memory usage while providing access to the box objects when available.
Wrapping Up
As we can see, properly managing the lifecycle of box objects in a Java application is crucial to prevent them from auto-closing. By maintaining strong references, implementing design patterns like Singleton, using Java Collections wisely, and even leveraging caching techniques, developers can ensure that their applications run smoothly without unexpected closures.
Understanding and applying these best practices not only helps create a more stable application but also enhances performance and reliability. For a deeper dive into Java concepts, consider checking out Oracle’s Official Java Documentation or exploring other educational resources. Happy coding!
Feel free to explore additional topics like memory management, design patterns, and Java collection frameworks to enhance your understanding and skill set further.