Java's Exception Handling: Avoiding Common Missteps
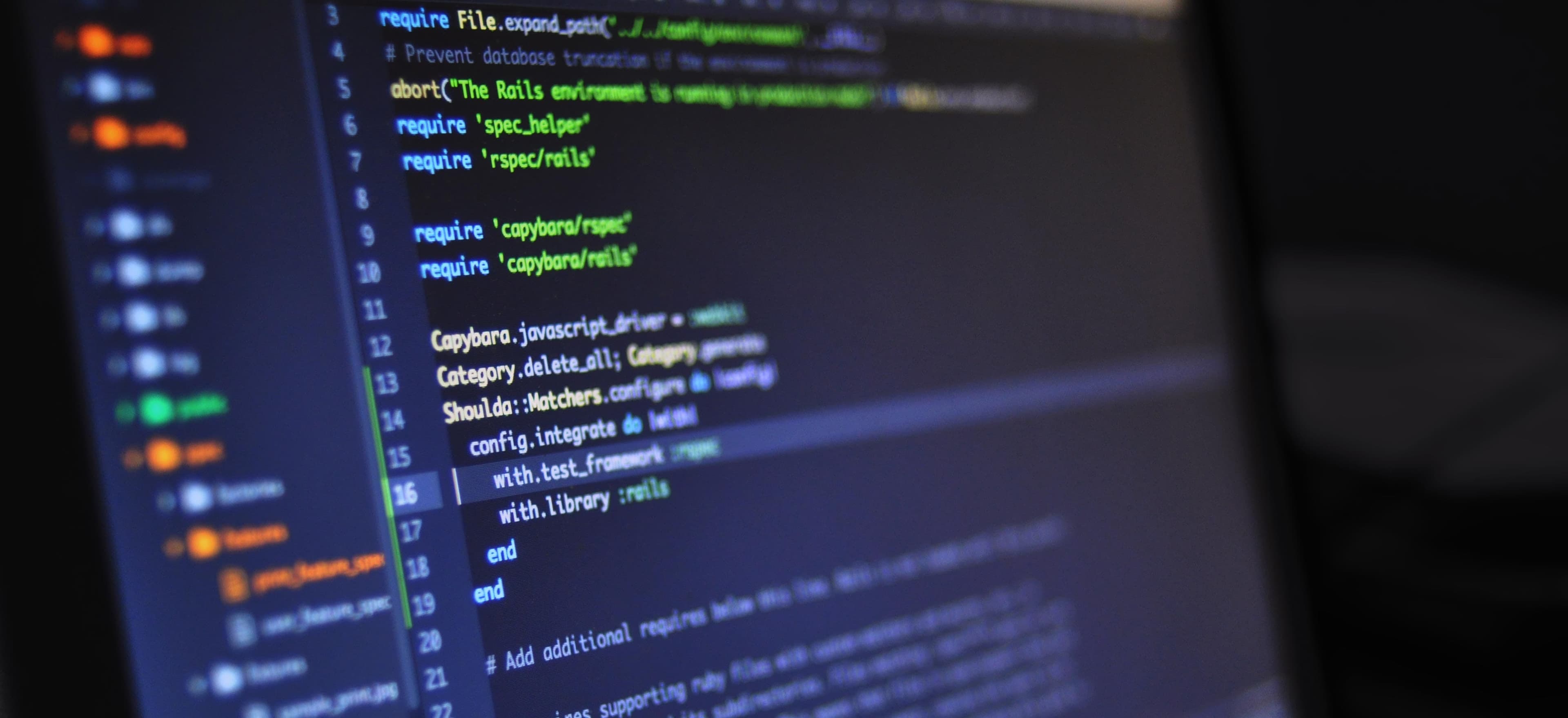
- Published on
Java's Exception Handling: Avoiding Common Missteps
Java, one of the most widely-used programming languages, offers a robust exception handling mechanism. Properly managing exceptions is crucial for building resilient applications. In this blog post, we will explore common pitfalls in Java's exception handling and provide practical tips to enhance your error management strategy. We will also discuss how these concepts mirror those found in other languages like Rust, which we touched on in the article "Navigating Rust's Error Handling: Common Pitfalls to Avoid" (check it out here).
What is Exception Handling?
Exception handling is a programming construct that enables you to respond to runtime errors in a controlled manner. In Java, exceptions are represented as objects that describe an unusual or erroneous situation that occurs during the execution of a program. The primary goal of exception handling is to maintain normal program flow even when unexpected events occur.
Exception Hierarchy in Java
Java's exception hierarchy is divided mainly into two branches: checked exceptions and unchecked exceptions.
-
Checked Exceptions: These exceptions are checked at compile-time. The compiler enforces handling of checked exceptions, which means the developer must either catch them or declare them in the method signature using the
throws
keyword.public void riskyMethod() throws IOException { // Your code that might throw IOException }
-
Unchecked Exceptions: These exceptions are checked at runtime and include errors and runtime exceptions. The compiler does not require handling of these exceptions.
public void method() { int result = 10 / 0; // This will throw ArithmeticException }
Understanding this hierarchy is crucial for deciding how to handle potential errors in your code.
Common Missteps in Exception Handling
Now, let's delve into some of the critical missteps to avoid when handling exceptions in Java.
1. Catching Generic Exceptions
One of the most significant pitfalls is catching overly broad exceptions, like Exception
or Throwable
. This practice diminishes the clarity and effectiveness of error handling.
Why is this a problem?
Catching generic exceptions can lead to silent failures, where the program continues running despite an error occurring. It makes debugging difficult because it obscures the root cause of the problem.
Example:
try {
// Some risky operation
} catch (Exception e) {
// Bad practice: catching general exception
e.printStackTrace(); // Too vague
}
Better Approach:
Instead, catch specific exceptions to enhance clarity.
try {
// Some risky operation
} catch (IOException e) {
System.err.println("IO exception occurred: " + e.getMessage());
} catch (ArithmeticException e) {
System.err.println("Mathematical error occurred: " + e.getMessage());
}
2. Ignoring Exceptions
Another common mistake is ignoring exceptions. Simply logging an error message without taking corrective action can lead to data corruption or application instability.
Example:
try {
// Some risky operation
} catch (IOException e) {
// Ignored
}
Better Approach:
Handle the exception appropriately. If it's a recoverable error, take steps to fix the issue, or if necessary, fail gracefully.
try {
// Some risky operation
} catch (IOException e) {
// Handling with corrective action
System.err.println("Error occurred, retrying...");
// retry logic or alternative approach
}
3. Overusing Exception Handling for Control Flow
Using exceptions for regular control flow, rather than for error management, is also a significant misstep. This approach can lead to performance degradation and less readable code.
Example:
public int divide(int a, int b) {
try {
return a / b; // Unintentional misuse
} catch (ArithmeticException e) {
return 0; // Control flow error handling
}
}
Better Approach:
Instead of relying on exceptions for control flow, check for potential issues before performing operations.
public int divide(int a, int b) {
if (b == 0) {
return 0; // Graceful handling
}
return a / b;
}
4. Not Using Finally or Try-With-Resources
Failing to release resources after usage can lead to memory leaks. For example, forget to close file streams or database connections.
Example:
public void readFile(String path) {
FileInputStream fis = null;
try {
fis = new FileInputStream(path);
// Read the file
} catch (IOException e) {
e.printStackTrace();
}
// fis might not get closed properly
}
Better Approach:
Utilize the finally
block or the try-with-resources statement to ensure that resources are always released.
public void readFile(String path) {
try (FileInputStream fis = new FileInputStream(path)) {
// Read the file
} catch (IOException e) {
e.printStackTrace();
} // fis will automatically be closed
}
5. Not Logging Exception Details
When exceptions occur, it is essential to log them with enough detail. Just logging the exception message can lead to a lack of context.
Example:
try {
// Some risky operation
} catch (SQLException e) {
System.err.println("SQL error");
}
Better Approach:
Log detailed messages that include context to assist in debugging.
try {
// Some risky operation
} catch (SQLException e) {
System.err.println("SQL error occurred while executing query: " + e.getSQLState());
e.printStackTrace(); // Log stack trace for debugging
}
Final Thoughts
Mastering Java’s exception handling can significantly enhance your application's stability and maintainability. Avoiding common pitfalls—such as catching generic exceptions, ignoring exceptions, overusing exceptions for control flow, neglecting resource management, and failing to log exceptions—can lead to more robust and user-friendly applications.
For developers looking to expand their understanding of error handling across various languages, the insights from Rust’s error handling can also be beneficial, as discussed in the article "Navigating Rust's Error Handling: Common Pitfalls to Avoid" (available here).
By implementing best practices for exception handling, you can ensure that your Java applications not only handle errors gracefully but continue functioning efficiently in the face of issues. Happy coding!
Checkout our other articles