Harnessing Java for Astrology Calculations in Your Apps
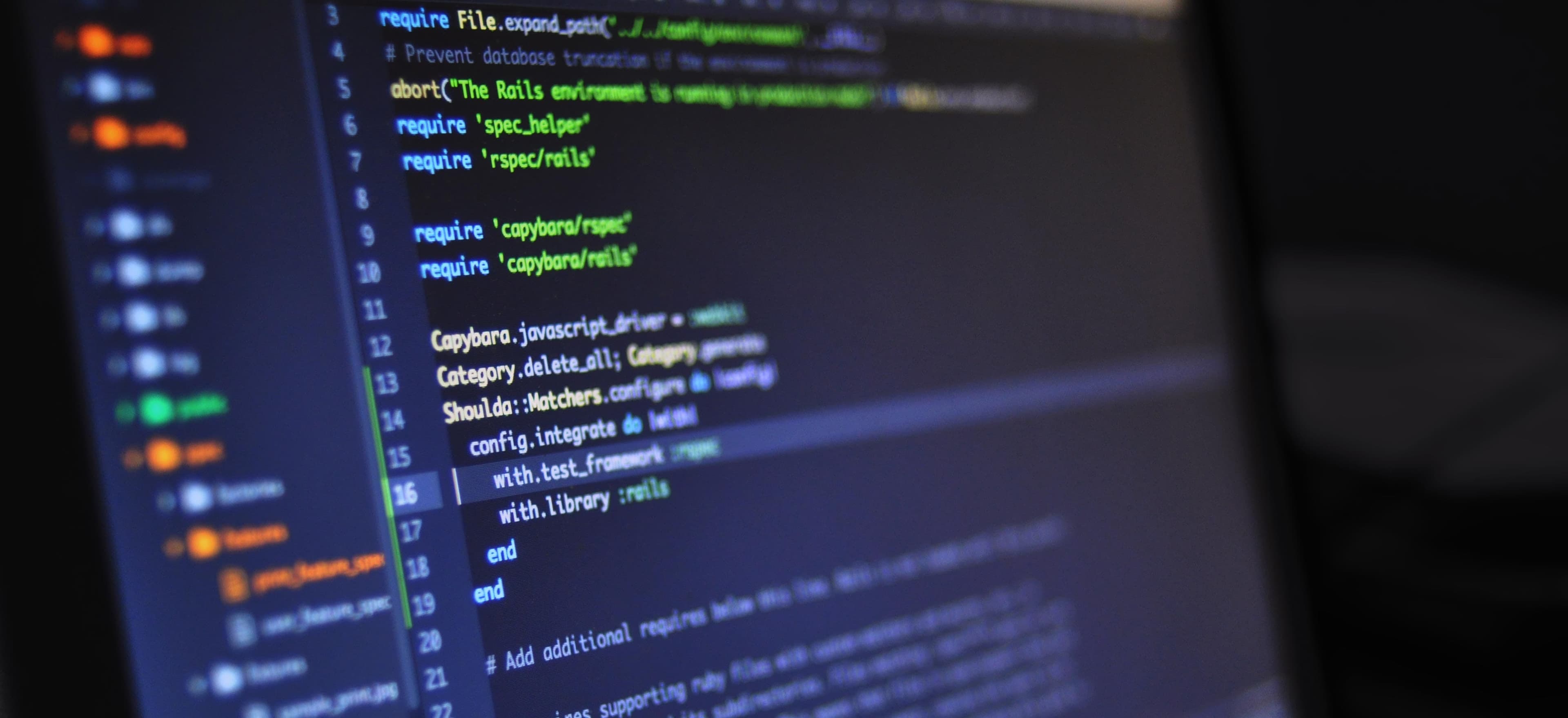
- Published on
Harnessing Java for Astrology Calculations in Your Apps
Astrology, a craft both ancient and fascinating, has captivated humanity for millennia. With the rise of technology, this field has found its way into modern applications. By using Java, developers can create applications that perform intricate astrology calculations. This article will guide you through understanding how to harness Java for astrology calculations, showcasing methods, tips, and exemplary code snippets.
Understanding the Basics of Astrology
Before diving into the technical details, let's understand the basics of astrology. It revolves around the positions of celestial bodies and their influence on human affairs. Key components of astrology include:
- Zodiac Signs: The twelve divisions of the celestial sphere.
- Planets: Each planet rules specific attributes and influences personality traits.
- Houses: Divisions of the sky that correlate with areas of life.
For a more comprehensive understanding, check out the engaging article "Astrologie: Entdecke die zeitlose Weisheit der Sterne" on auraglyphs.com.
Setting Up Your Java Development Environment
To begin coding, ensure you have a Java Development Kit (JDK) and a compatible Integrated Development Environment (IDE) such as IntelliJ IDEA or Eclipse.
Step 1: Install Java
- Visit the official Java SE Downloads page.
- Download and install the JDK that suits your operating system.
Step 2: Configure Your IDE
After installing, launch your chosen IDE and configure it to use your new JDK. This setup enables you to start writing Java code effectively.
Basic Astronomy Calculations Using Java
Astrological applications require fundamental astronomical calculations. We will create methods to compute the position of the Sun and the Moon using Java.
Example 1: Calculating the Position of the Sun
Here's a simple class to calculate the Sun's position based on the date.
import java.util.Calendar;
public class SunPositionCalculator {
public static void main(String[] args) {
Calendar calendar = Calendar.getInstance();
calendar.set(2023, Calendar.OCTOBER, 14); // Set date
double sunPosition = calculateSunPosition(calendar);
System.out.println("Sun position on " + calendar.getTime() + ": " + sunPosition + " degrees");
}
public static double calculateSunPosition(Calendar date) {
// Calculate the day of the year
int dayOfYear = date.get(Calendar.DAY_OF_YEAR);
// Use a simplified formula for the example
double sunPosition = (dayOfYear * 360.0) / 365.0; // Basic solar position calculation
return sunPosition;
}
}
Explanation of the Code
- Calendar Class: It allows you to set and manipulate dates easily in Java.
- Day of Year Calculation: We retrieve the day of the year, which is vital in determining the Sun's position.
- Simplified Formula: Here, we use a basic calculation for illustration. In real applications, you'd want to consider more complex elements such as the Earth's axial tilt and orbit.
Example 2: Calculating the Moon's Position
The Moon's position can be calculated in a similar manner, but it requires different parameters.
import java.util.Calendar;
public class MoonPositionCalculator {
public static void main(String[] args) {
Calendar calendar = Calendar.getInstance();
calendar.set(2023, Calendar.OCTOBER, 14); // Set date
double moonPosition = calculateMoonPosition(calendar);
System.out.println("Moon position on " + calendar.getTime() + ": " + moonPosition + " degrees");
}
public static double calculateMoonPosition(Calendar date) {
int dayOfYear = date.get(Calendar.DAY_OF_YEAR);
double moonPosition = (dayOfYear * 360.0) / 27.32; // Basic lunar cycle calculation
return moonPosition;
}
}
Why This Approach?
- Modularity: Having separate classes for calculations promotes better organization and allows for easier debugging.
- Simplicity Justifies Clarity: Though this example uses simplified formulas, it highlights the principle of calculating celestial positions which can be expanded with real-world algorithms.
Advanced Astrology Calculations
Now that we have our fundamental calculations, we can dive deeper into astrology with advanced calculations, such as planetary aspects and houses.
Computing Planetary Aspects
When planets form angles to each other, they create aspects. Here's a basic calculation for these aspects:
public class AstrologyAspectCalculator {
public static void main(String[] args) {
double sunPosition = 60; // degrees
double moonPosition = 120; // degrees
String aspect = determineAspect(sunPosition, moonPosition);
System.out.println("Aspect between Sun and Moon: " + aspect);
}
public static String determineAspect(double position1, double position2) {
double difference = Math.abs(position1 - position2);
if (difference < 8) {
return "Conjunction";
} else if (difference < 8 || difference > 172) {
return "Opposition";
} else {
return "No significant aspect";
}
}
}
What Makes This Code Work?
- Aspects: The method checks the angular distance between the two celestial bodies.
- Condition Check: We provide conditions to identify particular aspects. This foundational logic can be extended with more aspects like trine, square, etc.
Building User Interfaces for Astrology Apps
An engaging user interface (UI) is paramount in app development. Java offers various libraries for building UIs. Two popular options are JavaFX and Swing.
Example UI Code Snippet with JavaFX
Here's a simple UI where users can input a date and get the Sun's position:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class AstrologyApp extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
DatePicker datePicker = new DatePicker();
Button calculateButton = new Button("Calculate Sun Position");
calculateButton.setOnAction(e -> {
double position = SunPositionCalculator.calculateSunPosition(datePicker.getValue());
System.out.println("Sun Position: " + position);
});
VBox layout = new VBox(10, datePicker, calculateButton);
Scene scene = new Scene(layout, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Astrology App");
primaryStage.show();
}
}
Explanation of the UI Code
- JavaFX: Allows you to build rich client applications. The DatePicker facilitates date selection.
- Event Handling: The action method responds to the button click, triggering the calculation.
Wrapping Up
Creating astrology calculations using Java is an engaging way to meld ancient wisdom with modern technology. While this guide outlines basic and advanced techniques, remember, astrology is intricate. For deeper insights and more complex algorithms, consider researching existing libraries or collaborating with professional astrologers.
As technology continues to evolve, integrating astrology into applications provides an opportunity to engage users on a personal level. Whether designing a fun astrology app or creating a serious platform for astrological study, Java offers the tools needed to make it happen.
For additional inspiration on astrology, revisit the insightful article "Astrologie: Entdecke die zeitlose Weisheit der Sterne" at auraglyphs.com.
Resources for Further Learning
Feel free to use this knowledge to kickstart your journey into astrology with Java. Happy coding!
Checkout our other articles