Solving Display Challenges in Java Web Frameworks
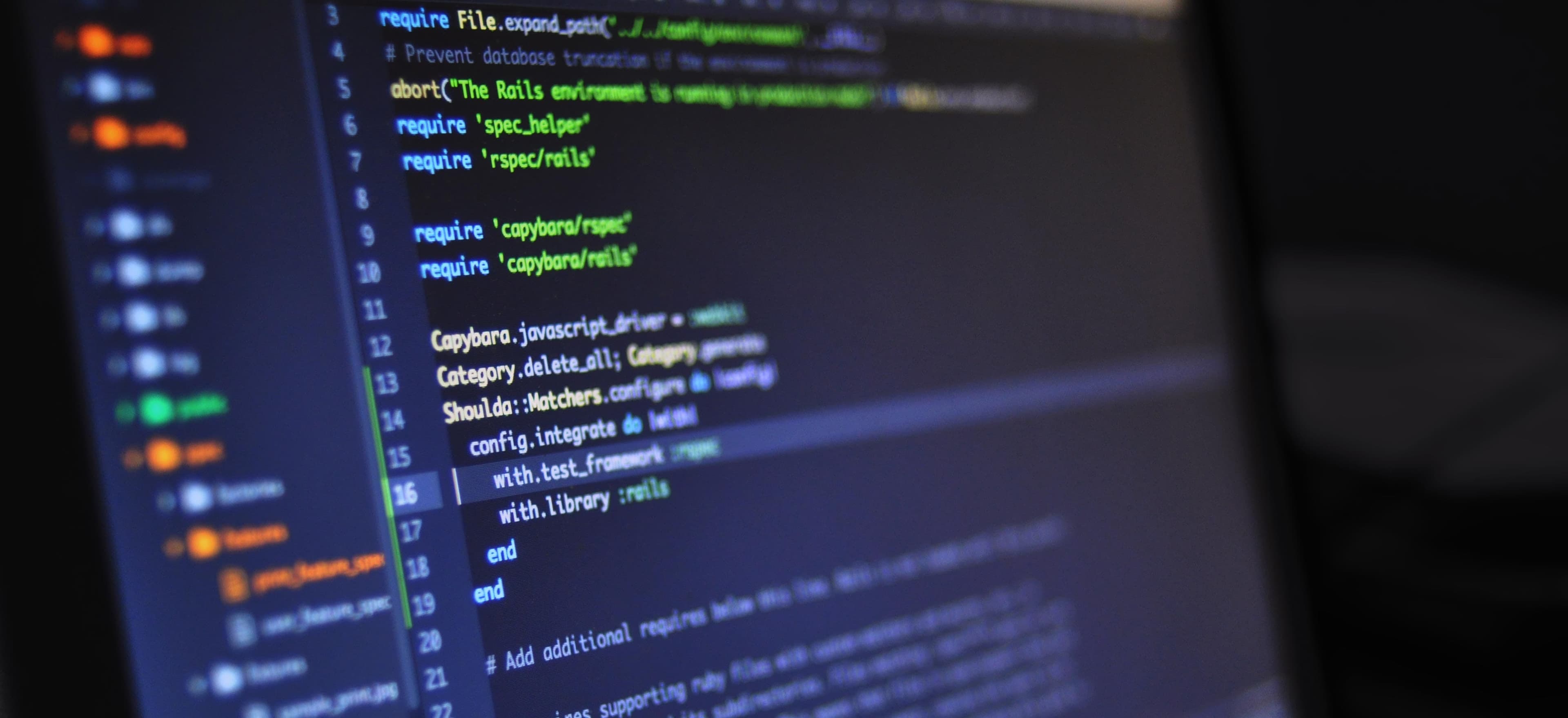
- Published on
Solving Display Challenges in Java Web Frameworks
In the rapidly evolving world of web development, displaying content effectively has become a cornerstone for user experience. Java web frameworks offer robust solutions for developing complex applications, but they often encounter display challenges that can hinder performance or lead to content visibility issues. This blog post aims to dissect common display challenges in Java web frameworks and provide practical solutions to enhance user experience.
Understanding Display Challenges
Display challenges arise due to several factors such as short content, incorrect layout configuration, and inadequate responsiveness across devices. For instance, if short content is not handled correctly, it can lead to empty spaces or improperly aligned elements on a webpage. As discussed in the article Display Issues: Fixing Short Content on Your Webpage, appropriately addressing these issues is imperative for an optimal user experience.
Short Content Handling
When dealing with short content, developers often face the challenge of ensuring that it still fits contextually within the layout. If not handled well, it can create large, blank areas on the screen. Using CSS for a flexible layout can help alleviate this issue.
.card {
display: flex;
flex-direction: column;
justify-content: center;
height: 100px; /* can adjust based on requirements */
margin: 10px;
background: lightgray;
}
.card-content {
padding: 10px;
}
Why This CSS?
- Flexbox: By utilizing CSS Flexbox, we allow our layouts to adapt fluidly to content size. The
justify-content: center;
style will align any content vertically in the center—even if the content is minimal. - Responsive Design: This approach ensures that regardless of the screen size, content remains visually appealing without unnecessary empty spaces.
Optimal HTML Structure
To further enhance the presentation, it’s important to structure your HTML appropriately. Here’s an example of how you can structure a card:
<div class="card">
<div class="card-content">
<h4>Short Title</h4>
<p>Some brief description.</p>
</div>
</div>
Why This Structure?
- Semantic HTML: The use of proper headings and paragraphs makes your content accessible and clear, improving SEO effectiveness.
- Simplicity: This structure is straightforward and focuses on core content without unnecessary complexity.
Handling Layout Issues with Java Frameworks
Java frameworks like Spring MVC and JSF enable developers to create powerful web applications. However, they can occasionally lead to intricate display challenges due to misconfigured views.
Example: Spring MVC with Thymeleaf
Consider a situation where you collectively retrieve data with an entity and need to display it in a paginated format.
@Controller
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping("/products")
public String getProducts(Model model, @RequestParam(defaultValue = "0") int page) {
Page<Product> productPage = productService.getProducts(PageRequest.of(page, 5));
model.addAttribute("products", productPage.getContent());
model.addAttribute("totalPages", productPage.getTotalPages());
return "product/list";
}
}
Why This Code?
- Pagination Logic: Using Spring's pagination capabilities allows users to navigate through large datasets without overwhelming them.
- Model Attribute: The
model.addAttribute
method passes data to the view seamlessly, ensuring an organized flow of data.
Thymeleaf Template
Here’s how you might structure the Thymeleaf template to display products:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Product List</title>
</head>
<body>
<div>
<h2>Products</h2>
<ul>
<li th:each="product : ${products}">
<span th:text="${product.name}"></span>
</li>
</ul>
<div>
<span th:if="${page > 0}">
<a th:href="@{/products(page=${page - 1})}">Previous</a>
</span>
<span th:if="${page < totalPages - 1}">
<a th:href="@{/products(page=${page + 1})}">Next</a>
</span>
</div>
</div>
</body>
</html>
Why This Template?
- Thymeleaf Syntax: Leverages Thymeleaf’s features for rendering lists, making it dynamic and straightforward to manage.
- Conditional Pagination Links: Provides a better user experience by only showing navigation links when applicable, avoiding confusion.
Utilizing CSS Frameworks for Responsive Design
CSS frameworks like Bootstrap can significantly aid in addressing display issues by providing pre-styled components and responsive grid layouts.
Example: Using Bootstrap in a Java Application
To implement Bootstrap, ensure your HTML file includes the necessary CDN link:
<head>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
</head>
Utilizing Bootstrap's card component can transform the way you present information:
<div class="card" style="width: 18rem;">
<img src="image.jpg" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Card Title</h5>
<p class="card-text">Some quick example text to build on the card title.</p>
<a href="#" class="btn btn-primary">Go somewhere</a>
</div>
</div>
Why Use Bootstrap?
- Pre-styled Components: Reduces the amount of custom CSS needed, allowing developers to focus on functionality rather than aesthetics.
- Mobile Responsiveness: Automatically adds responsive design features, ensuring the application is accessible across all devices.
To Wrap Things Up
In summary, displaying content effectively in Java web frameworks requires a balanced approach of structural integrity, agile coding practices, and responsive design. By implementing flexible CSS layouts, optimal HTML structures, and leveraging powerful frameworks like Bootstrap, developers can create applications that not only function well but also look appealing.
Remember that understanding the 'why' behind each decision made during development plays a crucial role in crafting effective web solutions. For further insights on avoiding display issues, consider diving into the article Display Issues: Fixing Short Content on Your Webpage.
By mastering these principles, you will ensure that your Java applications remain user-friendly, visually appealing, and maintain high standards of content delivery.