Optimize Java Web Applications to Avoid Content Display Issues
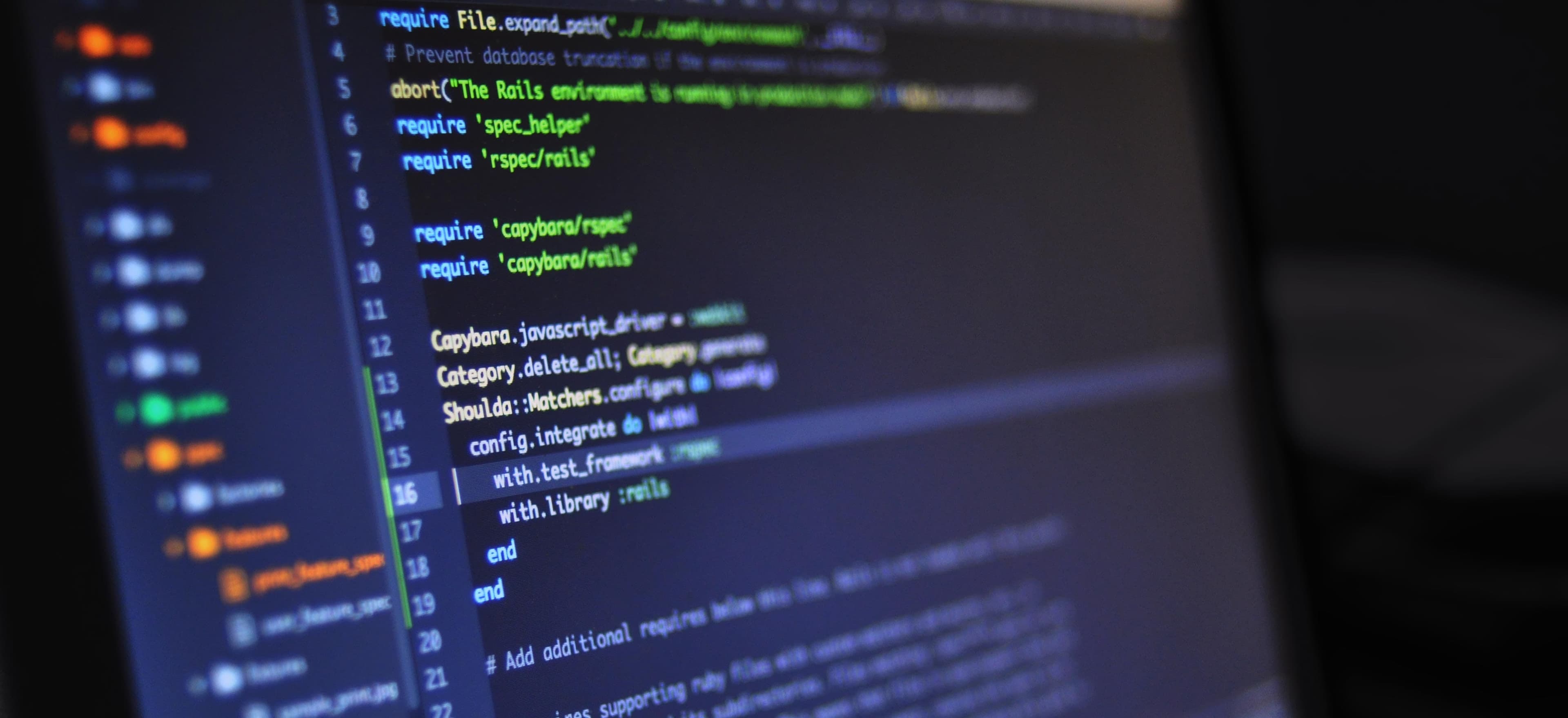
- Published on
Optimize Java Web Applications to Avoid Content Display Issues
In the world of web applications, user experience is paramount. One of the most crucial aspects of user experience is how content is displayed. A well-structured layout enhances readability and engagement, while poorly displayed content can lead to frustration and reduced user interaction. This is especially true in Java web applications, where developers often face unique challenges in content rendering.
In this blog post, we'll delve into how to optimize Java web applications to avoid content display issues. Along the way, we'll discuss common pitfalls, best practices, and provide code snippets to exemplify how to implement these strategies effectively.
Understanding Content Display Issues
Before we jump into solutions, it's essential to comprehend what constitutes content display issues. These problems can range from simple layout misalignments to more complex scenarios such as JavaScript errors that interfere with rendering. A relevant resource that discusses these issues in detail is the article titled “Display Issues: Fixing Short Content on Your Webpage”. This article outlines common display issues and offers techniques to handle short content optimally.
Identifying Common Content Display Problems
-
Excessive Use of JavaScript: While JavaScript can enhance interactivity, excessive use can lead to problems with how content gets displayed. JavaScript failures or delays can prevent content from rendering altogether.
-
CSS Misconfigurations: In many cases, CSS settings can cause layout issues. Whether it's improper use of flexbox, grid, or floats, CSS can make or break the visual arrangement of content.
-
HTML Structure: An improper HTML structure can lead to issues where content is not displayed in the desired manner.
-
Server-Side Rendering Issues: Java applications often employ frameworks that may not render pages effectively.
Now that we've identified potential culprits, let’s explore how to tackle these issues effectively.
1. Simplifying JavaScript Usage
JavaScript should enhance your application, but it should not hinder it. Optimize your JavaScript usage by eliminating unnecessary libraries and scripts. The principle of "progressively enhancing" your site rather than relying solely on JavaScript can also yield benefits.
Example: Defer Non-critical JavaScript
By deferring non-essential scripts, you can improve loading times, which ultimately helps in rendering the content faster.
<script src="example.js" defer></script>
Why This Matters
Using the defer
attribute allows the script to be executed after the document has been fully parsed. This leads to a smoother loading experience for users, minimizing display issues related to content appearing late or not at all.
2. Correcting CSS Layout Issues
Proper implementation of CSS can vastly improve how your content is displayed.
Example: Flexbox for Responsive Design
Make use of CSS Flexbox to create a responsive design that adjusts based on screen size.
.container {
display: flex;
flex-wrap: wrap;
justify-content: space-around;
}
.item {
flex: 1 1 300px; /* Grow and shrink with a base size */
margin: 10px;
}
Why This Matters
Flexbox offers a more efficient way to lay out, align, and distribute space among items in a container, even when their size is unknown or dynamic. This approach ensures better alignment and spacing between elements, reducing layout problems that affect content display.
3. Structuring HTML Properly
A robust HTML structure is vital for content to render correctly. It’s important to utilize semantic HTML tags to enhance accessibility and maintainability.
Example: Utilizing Semantic Tags
<main>
<article>
<h1>Understanding HTML Structure</h1>
<p>Proper HTML structure can greatly enhance content display.</p>
</article>
</main>
Why This Matters
Using semantic tags not only improves SEO but also allows browsers to render your content more appropriately. This prevents content rendering issues related to improper markup.
4. Optimizing Server-Side Rendering
Java applications often benefit from server-side rendering (SSR). However, improper SSR practices can lead to significant content display issues.
Example: Using JSP for Dynamic Content
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Dynamic Content</title>
</head>
<body>
<h1>Welcome, <%= userName %></h1>
</body>
</html>
Why This Matters
Using JSP effectively allows for seamless integration of dynamic content on your webpages. Gradually loading the critical content first can improve the perceived loading times and mitigate display issues.
5. Leveraging Content Delivery Networks (CDN)
Using a CDN can significantly reduce content loading times. When your static assets like CSS and JavaScript are stored on a CDN, users can download them faster, leading to speedy content rendering.
Example: Including a CSS File from a CDN
<link rel="stylesheet" href="https://cdn.example.com/styles.css">
Why This Matters
A CDN serves content from a location closer to the user, drastically cutting down loading time and improving the overall display of your web application’s content.
6. Testing and Monitoring
Finally, testing your web application is essential for identifying content display issues.
Example: Using Google Lighthouse
Running your web application through tools like Google Lighthouse provides in-depth reports on performance, accessibility, and SEO.
Why This Matters
Regular audits with such tools can help catch bottlenecks or misconfigurations before they become major display issues.
The Bottom Line
Optimizing Java web applications to avoid content display issues is an ongoing process, requiring careful attention to JavaScript, CSS, HTML structure, server-side rendering, and testing. By implementing the strategies discussed in this blog, you are taking proactive steps to enhance the user experience and ensure that your content is displayed correctly.
For more in-depth information on content display issues, consider reading the article “Display Issues: Fixing Short Content on Your Webpage” at infinitejs.com/posts/display-issues-fix-short-content-webpage.
By making informed choices and consistently monitoring your applications, you can build web applications that are not only visually appealing but also exceptionally functional.