Testing Code in Production: Managing Risks Effectively
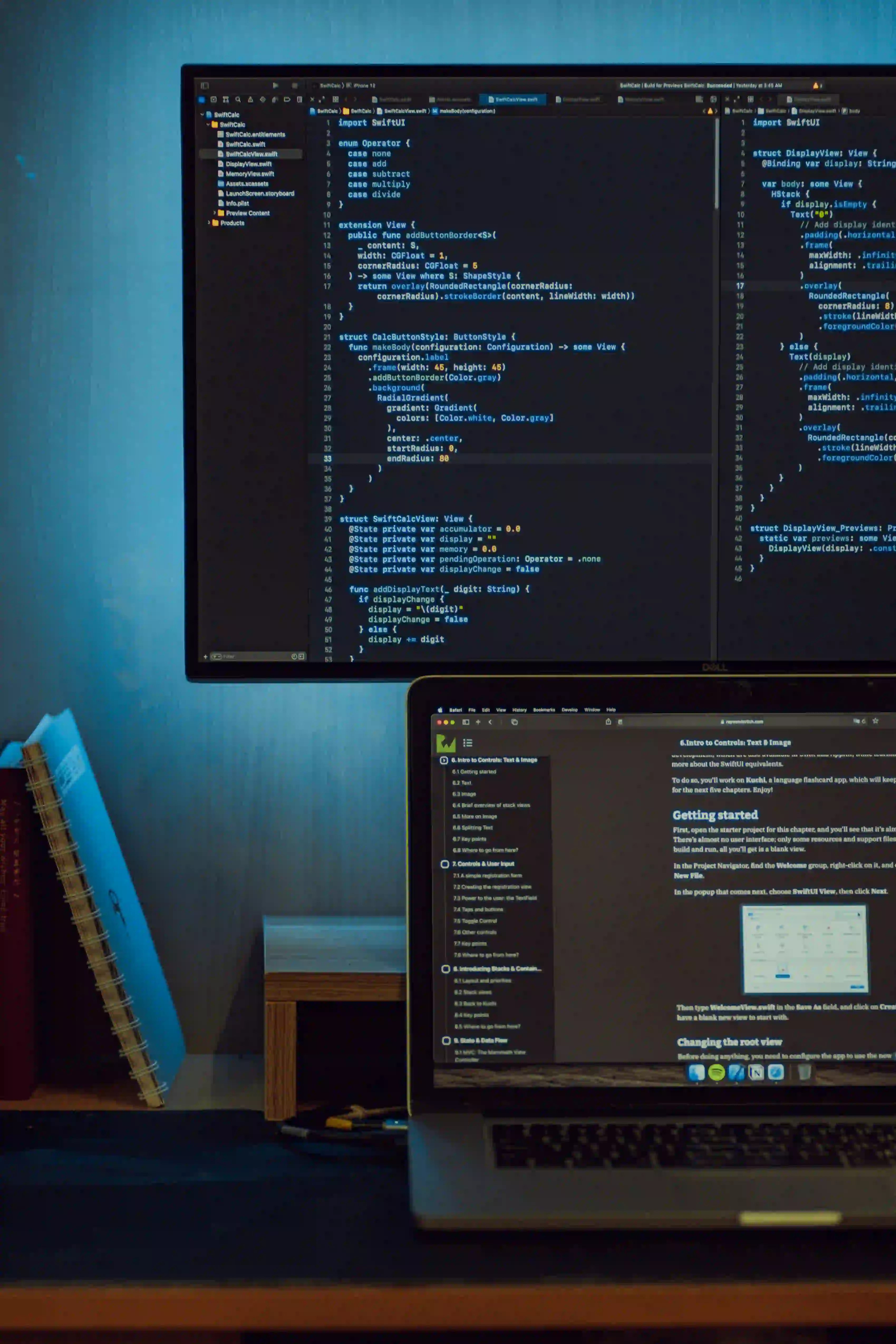
Testing Code in Production: Managing Risks Effectively
In today's fast-paced world of software development, the approach towards code testing has evolved significantly. Traditionally, developers conducted extensive tests in a separate staging environment, ensuring that all bugs were ironed out before deployment. However, as the demand for immediate updates grows, so does the trend of testing code in production. This blog post delves into the strategies for effectively managing risks inherent in production testing.
The Shift Toward Production Testing
The notion of testing in production (TiP) may raise eyebrows at first. Code once reserved for safe environments is now deployed in real-time, leading to many advantages but also unavoidable risks. Why consider this approach?
-
Immediate Feedback: When you test in production, you instantly gather data on how the system behaves in a live environment.
-
Real User Interaction: Testing in production allows developers to see how real users interact with new features and to collect valuable usage statistics.
-
Reduced Time to Market: Code can move from development to live production much faster, facilitating more agile development methodologies.
However, balancing these benefits with the potential drawbacks is crucial.
Understanding the Risks
Testing in production can result in several risks:
-
Service Disruptions: Bugs can directly affect user experience, potentially causing downtime or degraded service quality.
-
Data Integrity Issues: Errant production code can compromise data integrity, leading to corruption or loss of user data.
-
Security Vulnerabilities: Bugs introduced in the testing phase may create security holes that expose sensitive user information.
To engage in testing in production responsibly, it's imperative to adopt a strategy that mitigates these risks.
Key Strategies for Effective Production Testing
1. Feature Flags
Feature flags, also known as feature toggles, allow teams to enable or disable features dynamically in a production environment. This offers a level of control that can significantly reduce risk.
Example Code Snippet
public class FeatureToggle {
private boolean isFeatureEnabled;
public FeatureToggle(boolean isEnabled) {
this.isFeatureEnabled = isEnabled;
}
public void execute() {
if (isFeatureEnabled) {
// New feature code here
System.out.println("Feature is enabled.");
} else {
// Old feature code here
System.out.println("Feature is disabled.");
}
}
}
// Usage
FeatureToggle featureToggle = new FeatureToggle(true);
featureToggle.execute();
Why Use Feature Flags: This code illustrates how to control the execution flow based on the status of a feature. If a new feature encounters significant problems post-launch, it can be quickly disabled without needing an entirely new deployment.
2. Gradual Rollouts
Gradual rollouts involve releasing a feature to a small segment of your user base before expanding the deployment. This approach allows potential issues to be identified and addressed without affecting the entire user community.
Example Code Snippet
public class GradualRollout {
private static final int TOTAL_USERS = 1000;
public static void rolloutFeature(int userId) {
if (userId <= TOTAL_USERS * 0.1) { // First 10% of users
// Deploy feature
System.out.println("Feature is rolled out to user " + userId);
} else {
// Keep the old version
System.out.println("Old version for user " + userId);
}
}
}
// Simulating user rollouts
for (int i = 1; i <= 1000; i++) {
GradualRollout.rolloutFeature(i);
}
Why Gradual Rollouts: This snippet illustrates how only 10% of users are exposed to the new feature. This minimizes the risk of widespread failure, allowing teams to gather feedback and address issues before fully rolling out the new feature.
3. Monitoring and Metrics
Monitoring production environments is non-negotiable when testing code in production. Real-time monitoring tools can track application performance and health, ensuring that if something goes wrong, the team is immediately aware.
Example Code Snippet
public class ApplicationMonitor {
public void logPerformanceMetrics(int responseTime) {
System.out.println("Current response time: " + responseTime + "ms");
if (responseTime > 200) {
System.out.println("Warning: Response time exceeds threshold!");
// Trigger alert or remediation processes
}
}
}
// Usage
ApplicationMonitor monitor = new ApplicationMonitor();
monitor.logPerformanceMetrics(250); // Simulated response time
Why Monitoring Matters: The code above illustrates tracking performance metrics. Monitoring response times in real-time allows teams to respond proactively to performance issues before they impact users.
4. Robust Rollback Procedures
Even with extensive testing and risk management, issues can still arise. Therefore, a comprehensive rollback procedure is essential. Prepare the ability to revert to a previous stable version of your application quickly.
Example Code Snippet
public class RollbackManager {
public void rollback(String version) {
// Logic to revert to a stable version
System.out.println("Rolling back to version: " + version);
// Code to execute rollback
}
}
// Usage
RollbackManager rollbackManager = new RollbackManager();
rollbackManager.rollback("1.0.0"); // Example version
Why Rollbacks are Critical: This code snippet emphasizes the importance of having a rollback mechanism in place. Even with careful planning, the ability to revert to a stable configuration can save time and maintain user trust.
Closing Remarks
Testing code in production is not without its challenges, but with the right strategies, organizations can effectively manage risks. The emphasis on methods such as feature flags, gradual rollouts, real-time monitoring, and robust rollback procedures can ensure a smoother transition from development to production.
By embracing a careful, calculated approach to production testing, teams can harness the advantages of immediate feedback and faster delivery while keeping users' interests at the forefront. For more advanced strategies on feature flags and monitoring tools, consider exploring resources like LaunchDarkly for feature management and Datadog for monitoring solutions.
Ultimately, the goal is to foster a development culture where innovation meets stability—where risk management aids in delivering better products faster. Instrumenting an effective production testing strategy sets the stage for continuous improvement, allowing teams to adapt and thrive in an ever-changing digital landscape.