Common Caching Pitfalls in Java and How to Avoid Them
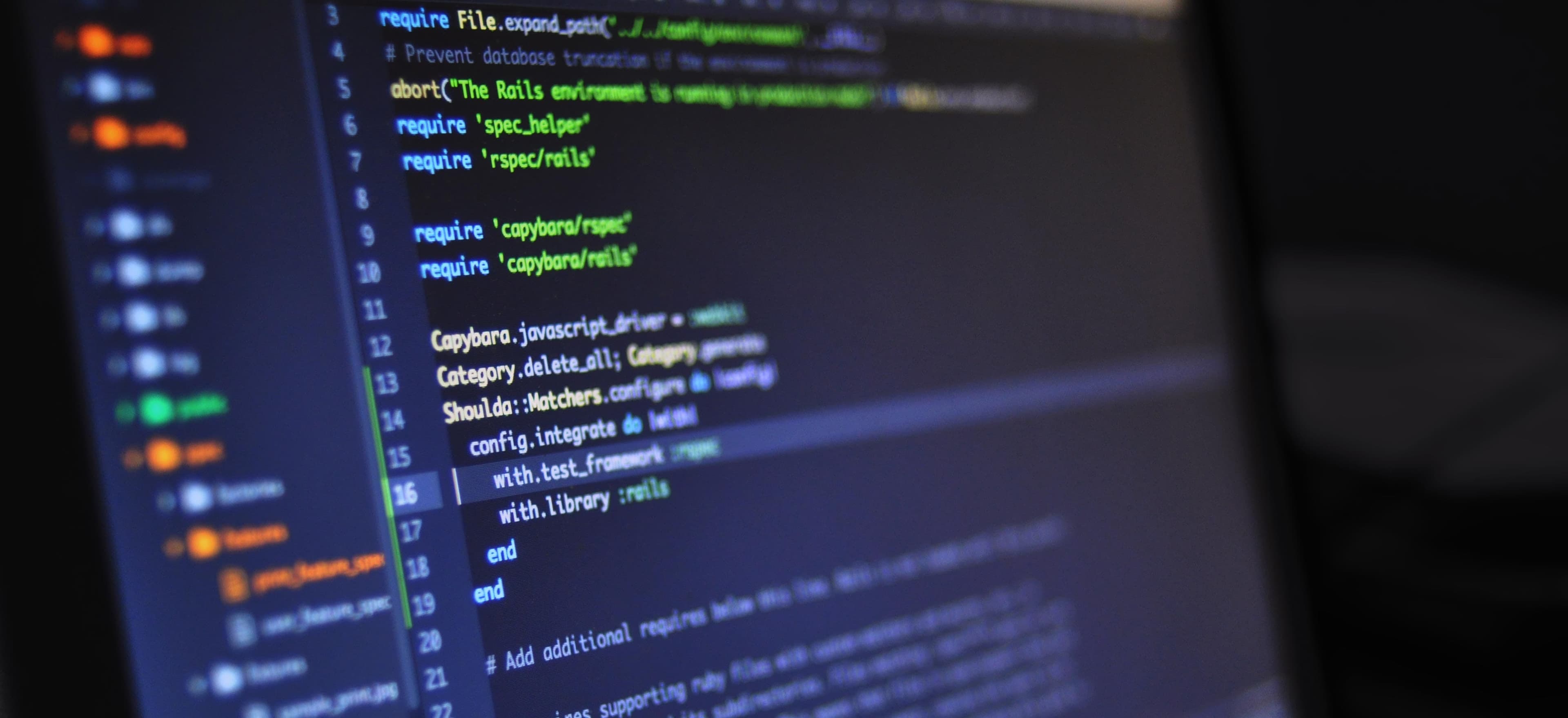
- Published on
Common Caching Pitfalls in Java and How to Avoid Them
Caching plays a critical role in application performance, especially for Java-based systems. By temporarily storing frequently accessed data, caching reduces latency and load on databases. However, improper caching can lead to inefficiencies and bugs. This blog post discusses some common caching pitfalls in Java and offers strategies to avoid them.
What is Caching?
Before diving into pitfalls, let’s clarify what caching is. Caching is the process of storing data in a location that allows for faster retrieval. For instance, if your application frequently queries a user database, caching these user profiles temporarily can significantly reduce database load and improve response times.
Common Caching Pitfalls
1. Over-Caching
What is it?
Over-caching refers to the tendency to store too much data in the cache, which ultimately leads to performance degradation. It can happen when developers cache data that isn't frequently accessed or when too many unique objects are stored.
Why is it a problem?
An overloaded cache can reduce efficiency. It may cause increased memory consumption, forcing Java's Garbage Collector (GC) to work harder and leading to potential performance bottlenecks.
How to avoid it?
Be selective about what you cache:
- Only cache the data that is frequently used and expensive to fetch.
- Implement cache size limitations so that only a subset of data can be stored. Here’s a simple implementation using Guava Caches:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
public class ExampleCache {
private final LoadingCache<String, String> cache;
public ExampleCache() {
cache = CacheBuilder.newBuilder()
.maximumSize(100) // Limit cache size
.expireAfterWrite(10, TimeUnit.MINUTES) // Set expiration time
.build(new CacheLoader<String, String>() {
public String load(String key) {
return fetchDataFromDatabase(key); // Method to fetch from DB
}
});
}
private String fetchDataFromDatabase(String key) {
// Simulate a database fetch operation
return "Data for " + key;
}
public String getValue(String key) throws ExecutionException {
return cache.get(key);
}
}
2. Ignoring Cache Expiration
What is it?
Cache expiration involves removing expired data from the cache to ensure that stale data is not served to users. Not handling this properly leads to serving outdated information.
Why is it a problem?
Stale cache can cause inconsistencies between the cached data and the original data source, leading to flawed business logic and a poor user experience.
How to avoid it?
Incorporate expiration policies in your caching strategy:
- Use time-based expiration—data should become outdated after a certain interval.
- Implement write-through caching that refreshes the cache when the underlying data changes. For example, the Guava library's settings allow you to manage expiration effectively.
3. Not Handling Cache Misses Effectively
What is it?
A cache miss occurs when the requested data is not found in the cache, prompting a backend lookup, usually from a database. Many developers overlook efficient handling of cache misses.
Why is it a problem?
If not managed well, cache misses can lead to performance hitches. Repeatedly querying the database can create a bottleneck and negate the benefits of caching.
How to avoid it?
Implement strategies to handle cache misses effectively:
- Use lazy-loading techniques to store data the first time it is requested and cache it for subsequent requests.
- Consider using a fallback mechanism for miss cases. This will enable your application to handle failures gracefully.
Here’s a simple implementation to demonstrate lazy loading:
public String getData(String key) {
// Assuming a cache field exists
String value = cache.getIfPresent(key);
if (value == null) {
value = fetchDataFromDatabase(key); // Fetch from DB if not in cache
cache.put(key, value); // Cache the newly fetched data
}
return value;
}
4. Poor Cache Key Design
What is it?
Cache keys are identifiers that point to specific cached values. Poorly designed cache keys can lead to collisions or inefficient lookups.
Why is it a problem?
If cache keys are not unique enough, they can overwrite each other leading to loss of data. Additionally, overly complex keys can drastically reduce performance.
How to avoid it?
Design cache keys carefully:
- Ensure cache keys are unique and follow a defined naming convention.
- Avoid using objects as keys due to the inherent complexity. Instead, prefer simple string representations.
5. Not Considering Thread Safety
What is it?
In multi-threaded Java environments, cache operations must be thread-safe to avoid race conditions, which can lead to data integrity issues.
Why is it a problem?
If several threads try to access or modify the cache simultaneously, it can lead to unpredictable behavior and data corruption.
How to avoid it?
Leverage concurrent data structures designed for thread safety. For example, use ConcurrentHashMap
for simpler cache implementations. Libraries like Caffeine or Guava also provide thread-safe cache implementations.
import java.util.concurrent.ConcurrentHashMap;
public class SimpleThreadSafeCache {
private final ConcurrentHashMap<String, String> cache = new ConcurrentHashMap<>();
public String getValue(String key) {
return cache.getOrDefault(key, fetchDataFromDatabase(key));
}
public void putValue(String key, String value) {
cache.put(key, value);
}
private String fetchDataFromDatabase(String key) {
return "Data for " + key; // Simulated database fetch
}
}
Closing Remarks
Cachings, when correctly implemented, offer tremendous performance benefits. However, as we've discussed, pitfalls such as over-caching, stale data, unhandled cache misses, poor key design, and thread safety issues can easily undermine these advantages. By following this guide and implementing the discussed strategies, you can significantly enhance your Java application's caching effectiveness.
For further reading on caching solutions in Java, you may explore Java Caching Best Practices or check out Caching with Spring for detailed approaches aligned with popular frameworks.
Ultimately, keep the core principle of caching in mind: it’s about delivering speed while ensuring data integrity. Happy coding!