Overcoming Backpressure Issues in RxJava File Loading
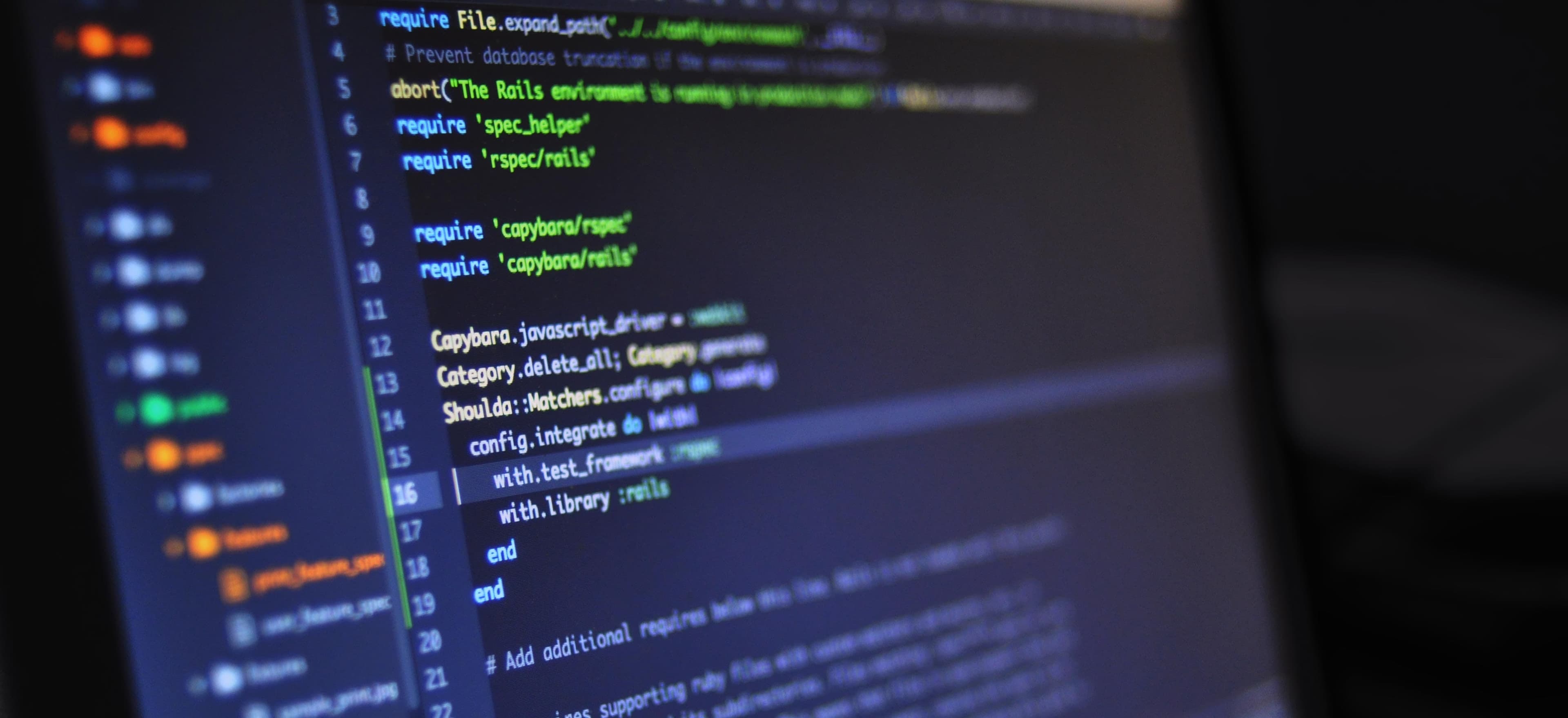
- Published on
Overcoming Backpressure Issues in RxJava File Loading
In the realm of reactive programming, backpressure represents a common yet often misunderstood phenomenon. As systems grow more complex, developers may find themselves grappling with performance bottlenecks, especially when handling asynchronous file loading. This blog post dives deep into conquering backpressure challenges in RxJava while loading files.
Understanding Backpressure
Backpressure occurs when the data producer generates items faster than the consumer can process them. In RxJava, this situation can become detrimental, leading to memory overflows or dropped events. Imagine an application that reads large files, producing observable data points at a high speed. Without proper control, the reading and processing of data can spiral out of control.
To get a clearer picture, let’s break down our task: loading a large file, processing its contents, and ensuring that our system handles data flows gracefully.
Setting Up the Basics
First, let's go over a basic example of how to read a file using RxJava. We will leverage Flowable
, a suitable type for handling backpressure in RxJava.
import io.reactivex.Flowable;
import io.reactivex.schedulers.Schedulers;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
public class FileLoader {
public static void main(String[] args) {
String filePath = "path/to/largefile.txt";
Flowable<FileLine> fileLineFlowable = Flowable.create(emitter -> {
List<String> lines = Files.readAllLines(Paths.get(filePath));
for (String line : lines) {
if (!emitter.isCancelled()) {
emitter.onNext(new FileLine(line));
}
}
emitter.onComplete();
}, BackpressureStrategy.BUFFER);
fileLineFlowable
.subscribeOn(Schedulers.io())
.observeOn(Schedulers.computation())
.subscribe(
line -> processLine(line),
Throwable::printStackTrace,
() -> System.out.println("File processing complete.")
);
}
private static void processLine(FileLine line) {
// Simulate processing each line
System.out.println("Processing: " + line.getContent());
try {
Thread.sleep(100); // Simulate time-consuming processing
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
class FileLine {
private final String content;
public FileLine(String content) {
this.content = content;
}
public String getContent() {
return content;
}
}
Code Explanation
- File Loading: The
Flowable.create
method is used to read the file line by line. Each line is then emitted as aFileLine
object. - Backpressure Strategy: We use
BackpressureStrategy.BUFFER
, allowing us to store emitted lines in a queue until they are processed. This is a straightforward strategy, but it has its limits. - Thread Management: Using
subscribeOn(Schedulers.io())
helps in performing IO-bound tasks on an I/O thread, whileobserveOn(Schedulers.computation())
shifts processing to computation threads.
Enhancing Backpressure Handling
To gain more control over backpressure, we can switch to Flowable
operators designed specifically for it, such as onBackpressureBuffer
, onBackpressureDrop
, or custom buffering strategies.
Improved Handling with onBackpressureDrop
When using onBackpressureDrop
, we tell our system to drop items if the buffer is full. This can be useful for non-critical data where prompt action isn't necessary.
fileLineFlowable
.onBackpressureDrop() // Drop data if the consumer is slow
.subscribeOn(Schedulers.io())
.observeOn(Schedulers.computation())
.subscribe(
line -> processLine(line),
Throwable::printStackTrace,
() -> System.out.println("File processing complete.")
);
Use of Throttling with throttleLast
Another effective way to combat backpressure is to employ throttling. This approach will limit the rate at which items are processed.
fileLineFlowable
.throttleLast(100, TimeUnit.MILLISECONDS) // Process the latest line every 100 milliseconds
.subscribeOn(Schedulers.io())
.observeOn(Schedulers.computation())
.subscribe(
line -> processLine(line),
Throwable::printStackTrace,
() -> System.out.println("File processing complete.")
);
Integrating Error Handling
In real-world applications, files may not always be accessible. We must implement proper error handling to manage exceptions that can arise during file reading or processing.
fileLineFlowable
.onErrorResumeNext(throwable -> {
System.err.println("Error during file loading: " + throwable);
return Flowable.empty(); // Return an empty observable on error
})
.subscribeOn(Schedulers.io())
.observeOn(Schedulers.computation())
.subscribe(
line -> processLine(line),
Throwable::printStackTrace,
() -> System.out.println("File processing complete.")
);
Summary of Error Handling Techniques
onErrorResumeNext
: This operator allows your stream to continue, even after an error has occurred. You can catch the exception and respond to it gracefully.- Try-Catch within the Subscriber: Even though not specific to RxJava, implementing a try-catch block within your subscriber can help manage specific use cases where you expect issues.
Final Touches
Having implemented strategies to control backpressure, it is now vital to test your application thoroughly in conditions that mimic production. Load tests can reveal how your system behaves under pressure and ensure that your backpressure mechanisms are functioning correctly.
Effective Testing Practices
- Unit tests with RxJava's
TestObserver
: This will allow you to simulate various scenarios easily. - Load testing with tools like JMeter: This will help you assess the performance and behavior of your file loading under different load conditions.
The Last Word
Backpressure in RxJava can be complex, especially when dealing with asynchronous file loading. However, by understanding its mechanisms and implementing robust handling strategies such as backpressure strategies, throttling, and error handling, we can create responsive and efficient applications.
For more insight on reactive programming concepts, check out RxJava Official Documentation and Backpressure in Reactive Streams.
By mastering these concepts, you can enhance your RxJava applications, turning potential pitfalls into strategic advantages. Happy coding!
Checkout our other articles