Solving Infinispan Caching Issues in Apache Camel Transactions
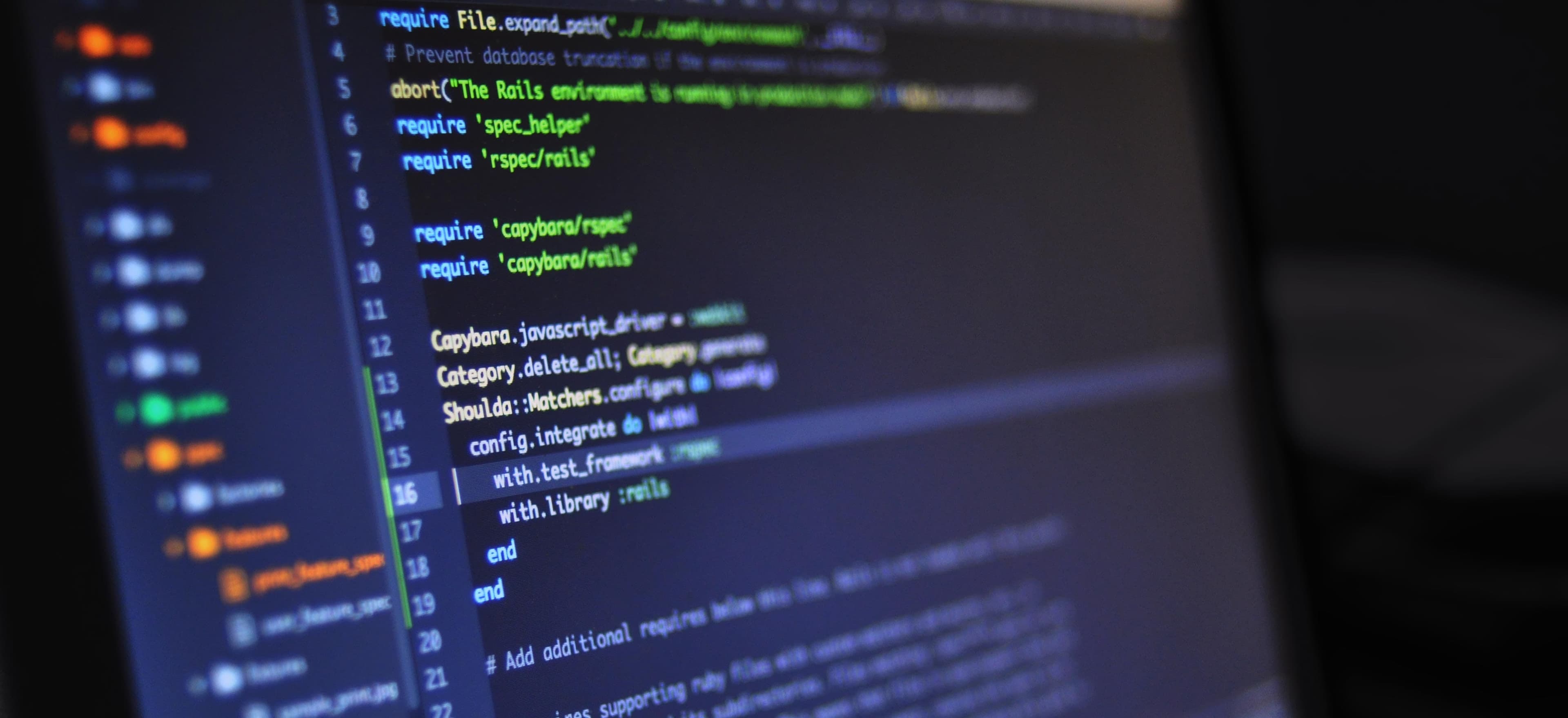
- Published on
Solving Infinispan Caching Issues in Apache Camel Transactions
Caching is a common design pattern used in software development to improve the performance of applications. When combined with transaction management in Apache Camel, caching can lead to intricate issues that require careful handling. In this article, we will explore Infinispan’s caching solutions in the context of Apache Camel transactions. We aim to provide a clear understanding of potential pitfalls and efficient solutions through insightful discussions and practical code snippets.
What Is Apache Camel?
Apache Camel is a powerful open-source integration framework that allows developers to integrate various systems easily. It provides an array of components for connecting applications through various protocols and data formats. A key aspect of Camel is its ability to handle complex transaction scenarios seamlessly.
What Is Infinispan?
Infinispan is a distributed in-memory key/value data store and cache developed by Red Hat, built for scalability and high availability. Infinispan is often used in conjunction with Java applications to provide fast data access and caching capabilities. This allows distributed applications to achieve surprisingly high performance.
Why Use Infinispan with Apache Camel?
Using Infinispan with Apache Camel can help improve the speed of data access in various scenarios. For example, within a microservices architecture, you may want to cache request and response data between services to reduce latency and increase throughput.
Potential Caching Issues in Transactions
While caching can add a significant performance boost, it is important to recognize the issues it may introduce, especially in a transactional context. Here are some common pitfalls:
- Data Consistency: Transactions rely on consistent data. If your cache is stale, you may read outdated information, leading to inconsistent states.
- Transaction Scope: Understanding the boundaries of transactions is essential. If you fetch data from the cache outside of the transaction, you risk rolling back updates that inappropriately rely on state.
- Synchronization Overhead: Using caches in a distributed system can lead to synchronization issues. Without utilizing transactional semantics appropriately, you'll face race conditions.
Strategies for Handling Caching Issues in Apache Camel
To optimize your application while avoiding common pitfalls, consider the following strategies:
- Use Caching Annotations: Annotations can help manage caching behavior directly in your code.
- Leverage Infinispan's Transactions: Infinispan supports optimistic locking and can work with transactions in a way that maintains consistency.
- Transaction Isolation Levels: Adjust the isolation level according to your requirements, ensuring that transactions do not interfere with each other.
Sample Code Snippet: Basic Camel Route with Caching
Let's look at a simple example of integrating Infinispan with an Apache Camel route.
import org.apache.camel.CamelContext;
import org.apache.camel.builder.RouteBuilder;
import org.apache.camel.impl.DefaultCamelContext;
import org.infinispan.Cache;
import org.infinispan.configuration.cache.ConfigurationBuilder;
import org.infinispan.manager.DefaultCacheManager;
public class CachingExample {
public static void main(String[] args) throws Exception {
// Create an Infinispan cache manager
DefaultCacheManager cacheManager = new DefaultCacheManager(new ConfigurationBuilder().build());
Cache<String, String> cache = cacheManager.getCache("myCache");
// Initialize a Camel Context
CamelContext camelContext = new DefaultCamelContext();
// Define the route
camelContext.addRoutes(new RouteBuilder() {
@Override
public void configure() throws Exception {
from("direct:start")
.process(exchange -> {
String key = exchange.getIn().getBody(String.class);
// Try to get cached value
String value = cache.get(key);
if (value == null) {
value = expensiveOperation(key); // Placeholder for expensive data fetching
cache.put(key, value); // Cache the result
}
exchange.getIn().setBody(value);
})
.to("log:info");
}
// Simulate an expensive operation
private String expensiveOperation(String key) {
// Simulates a delay
try {
Thread.sleep(500); // Simulating expensive operation (e.g., database call)
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Value for " + key;
}
});
camelContext.start();
// Send a test message
camelContext.createProducerTemplate().sendBody("direct:start", "test-key");
// Stop the context
camelContext.stop();
cacheManager.stop();
}
}
Explanation of the Code
-
Initialization: We start by creating an Infinispan
CacheManager
and fetching a specific cache named "myCache." This is critical; without proper cache management, we risk memory leaks or cache misses. -
Camel Route: The route continuously processes incoming requests from the
direct:start
endpoint. It attempts to retrieve a value from the cache first. -
Cache Lookup: If the value is absent in the cache, an "expensive" operation is invoked (simulating a slow database query). The resulting value is then stored in the cache for future retrieval.
-
Logging: The output is logged to confirm that the cache mechanism is working efficiently.
Handling Data Consistency
One of the paramount concerns when using caching within a transaction context is ensuring the data remains consistent. Infinispan provides a variety of transactional capabilities, such as using transaction managers or local locking to maintain data integrity.
Implementing Transactional Cache Operations
You can also implement a transactional mechanism as shown in the code snippet below:
import javax.transaction.TransactionManager;
import javax.transaction.UserTransaction;
...
UserTransaction userTransaction = ...; // Obtain the user transaction
TransactionManager transactionManager = ...; // Obtain transaction manager
try {
// Start transaction
userTransaction.begin();
// Perform cache operations
String key = "myKey";
cache.put(key, "myValue"); // This will be part of the transaction
// Prepare to commit
userTransaction.commit();
} catch (Exception e) {
// Roll back transactions upon failure
userTransaction.rollback();
throw e; // Rethrow for application logic to handle
}
Pro Tip: Use Infinispan’s Cache Listeners
Infinispan supports cache listeners that allow you to perform specific actions when changes occur in the cache. This can be useful for auditing changes or refreshing data sources based on cache updates.
Closing the Chapter
By integrating Infinispan with Apache Camel, developers can effectively manage caching within transaction scenarios. However, developers must be cautious about potential issues such as data consistency, transaction scope, and synchronization overhead.
By implementing the strategies discussed in this article, you can harness the full potential of caching while ensuring data integrity.
For further reading, you might want to check the official documentation on Infinispan Transactions and Apache Camel.
Next Steps
Apply these concepts in your own projects and experiment with the configurations. Test and monitor the performance of your caching strategy in various scenarios. This exploration can lead to a more robust application architecture. Happy coding!