Resolving Auto-Increment Issues in Maven Release Plugin
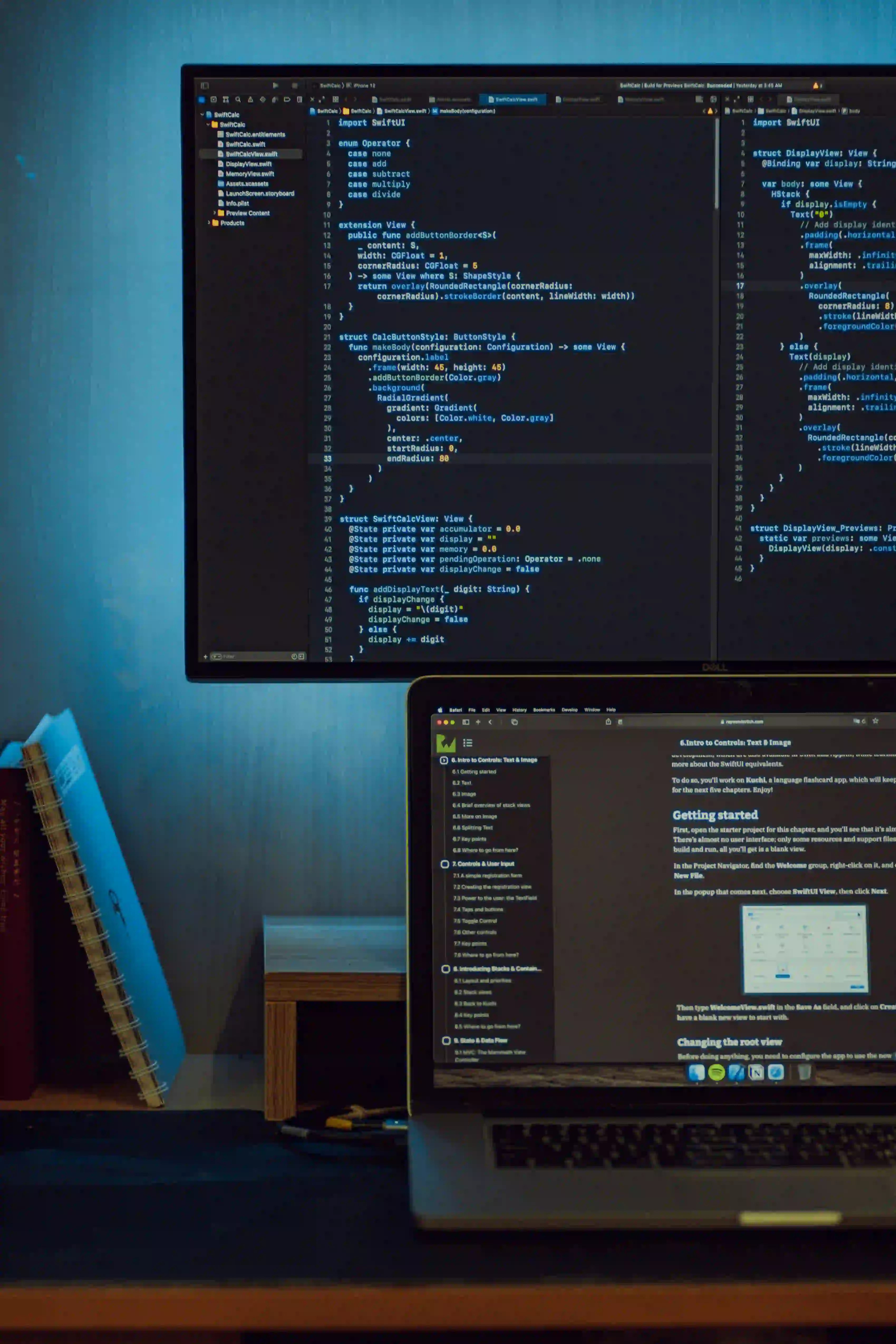
Resolving Auto-Increment Issues in Maven Release Plugin
The Maven Release Plugin is a powerful tool that facilitates the release of projects in Java. It automatically manages version increments, tagging, and deploying artifacts to repositories. However, users frequently encounter issues with auto-incrementing versions, especially when working in collaborative environments. In this blog post, we'll explore the common auto-increment issues that arise in the Maven Release Plugin, and how to effectively resolve them.
Understanding the Maven Release Plugin
Before digging into the issues, letβs briefly understand what the Maven Release Plugin does. It helps automate the process of preparing your project for release by:
- Updating Versions: It can increment the version in your
pom.xml
. - Tagging Releases: It tags your code in version control systems like Git.
- Deploying Artifacts: It builds and deploys your project artifacts as needed.
Usually, when you run the command:
mvn release:prepare
It will prompt for a new version and automatically update the version numbers. But when you're working with multiple branches or teams, conflicts can arise, particularly related to version increments.
Common Issues with Auto-Increment
1. Conflicting Versions
When multiple team members are working on different branches, they may independently change the version number in the pom.xml
. This can lead to conflicts when the Maven Release Plugin attempts to auto-increment the version.
Solution: Communication and Version Management
A simple solution is to establish a clear versioning scheme within your team. Tools like Semantic Versioning can help manage expectations about version increments.
2. Non-Standard Version Format
The Maven Release Plugin expects versions to follow a specific format, usually in the form of x.y.z
(major.minor.patch). When a non-standard version is used, the plugin can fail to auto-increment correctly.
Solution: Validate Versioning
Ensure that versioning rules are followed before running the Maven Release Plugin. Here is how you can validate your version:
<project>
...
<properties>
<project.version>1.0.0</project.version>
</properties>
...
</project>
If versions do not comply, you can use a script to check this during your continuous integration (CI) process.
3. Uncommitted Changes
Running the Maven Release Plugin with uncommitted changes can break the process since it modifies the pom.xml
. The plugin might not be able to auto-increment because it doesn't have a clean working directory.
Solution: Ensure a Clean State
Before invoking the release process, always check your Git status:
git status
If there are uncommitted changes, either commit them or stash them. A simple command to stash changes is:
git stash
Run the release commands only after confirming that your working directory is clean.
4. Incorrect Deployment Configuration
Sometimes, the auto-increment may seem to fail because the artifact is not being deployed correctly due to misconfigured settings in the pom.xml
.
Solution: Verify Distribution Management
Ensure that your pom.xml
includes the correct distribution management configuration:
<distributionManagement>
<repository>
<id>releases</id>
<url>http://your-repo-url/releases</url>
</repository>
</distributionManagement>
This ensures that the plugin has a valid target for deploying releases.
Step-by-Step: Running Maven Release Plugin
Here is a step-by-step guideline to use the Maven Release Plugin effectively:
-
Clean Your Workspace: Make sure that your workspace is clean.
π§snippet.shgit status
-
Prepare for Release: Run the prepare command. This will allow you to define the next version number.
π§snippet.shmvn release:prepare
-
Perform the Release: This command will handle the tagging and deployment.
π§snippet.shmvn release:perform
-
Clean Up: After a successful release, check if you can get your workspace back to normal, for example:
π§snippet.shgit stash pop
Example: Updating Version in POM
Here is an example to illustrate how to increment the version dynamically within your CI/CD pipeline using Maven's properties. You can define your project's version in the pom.xml
:
<project>
...
<version>${project.version}</version>
...
</project>
You can update the version using a profile in your pom.xml
based on the release type:
<profiles>
<profile>
<id>release</id>
<properties>
<release.version>1.0.1</release.version>
</properties>
</profile>
</profiles>
Run the following command to set the new version:
mvn versions:set -DnewVersion=${release.version} -P release
After setting the new version, remember to commit your changes.
Key Takeaways
Resolving auto-increment issues in the Maven Release Plugin is crucial for maintaining a smooth development and release cycle. By ensuring clean working states, adhering to versioning standards, and validating deployment configurations, you can mitigate most common issues.
For more information about Maven and releases, refer to the Maven Release Plugin documentation and Maven Guides.
Managing releases is not just about automation; it's about creating a culture of collaboration. By following best practices and maintaining clear communication within your team, you can take full advantage of the powerful capabilities that Maven has to offer. Happy coding!