3 Java Pitfalls Every Developer Must Avoid
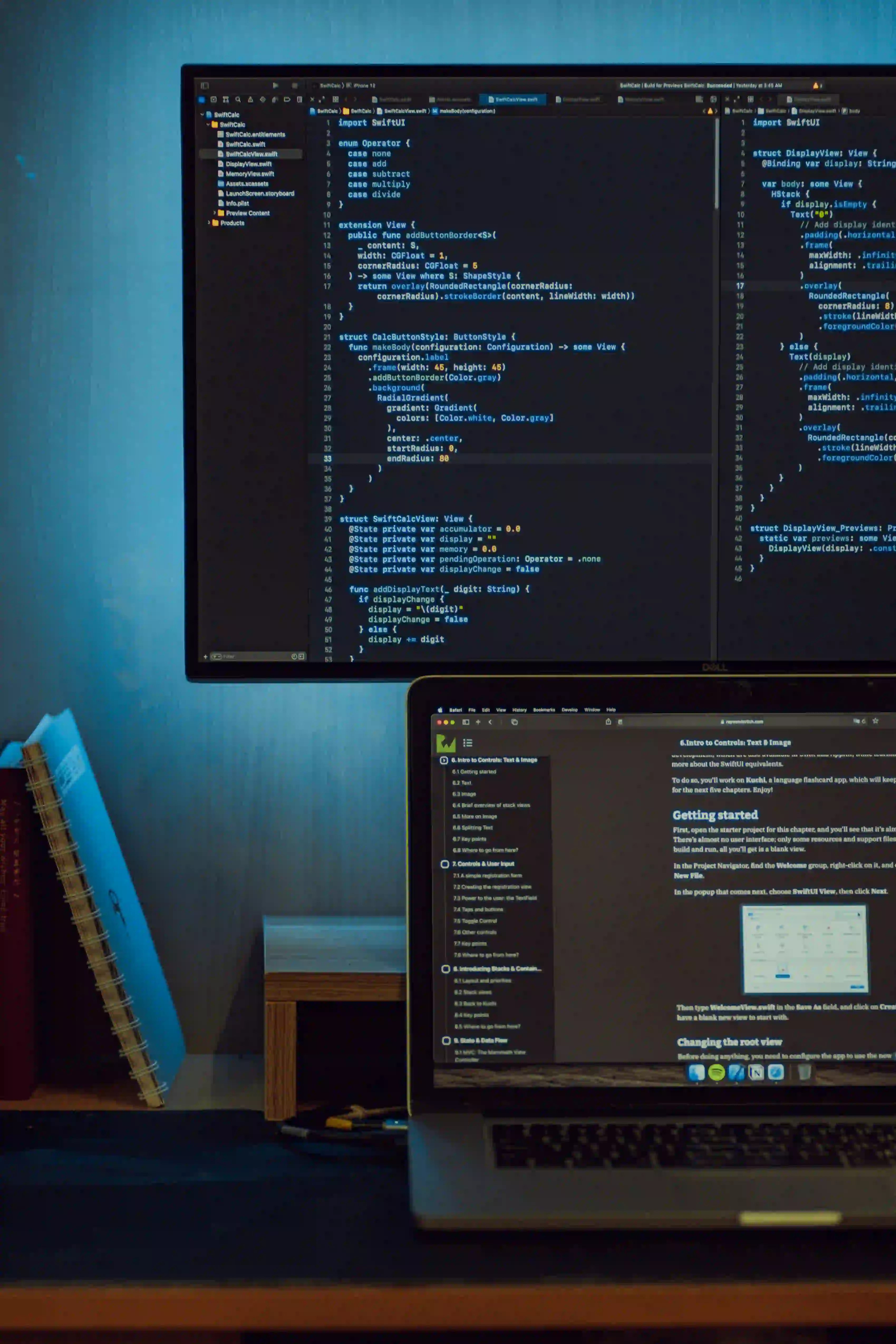
3 Java Pitfalls Every Developer Must Avoid
Java is one of the most widely-used programming languages, cherished for its portability, performance, and object-oriented features. However, like any language, it has its traps that can ensnare even experienced developers. Understanding these pitfalls can save you from headaches and improve your coding practices. In this article, we'll discuss three common Java pitfalls and how to avoid them.
1. Null Pointer Exceptions
One of the most notorious issues in Java programming is the dreaded Null Pointer Exception (NPE). It strikes when you attempt to use an object that has not been initialized or is set to null.
Why is it a Problem?
NPEs can lead to runtime crashes, which diminish your application’s reliability. They often surface in production environments, confusing users and complicating the debugging process.
How to Avoid It
You can avoid NPEs in several ways:
- Use Initialization: Always initialize your variables.
String message = "Hello, World!"; // Initialized correctly
- Use Optional: Java 8 introduced
Optional
, a container object which may or may not contain a non-null value.
Optional<String> optionalMessage = Optional.ofNullable(message);
optionalMessage.ifPresent(System.out::println); // Will only print if present
- Check for Nulls: Always perform null checks before using objects.
if (message != null) {
System.out.println(message);
} else {
System.out.println("Message is null.");
}
2. Overusing Static Members
Creating overly static classes or members is another common pitfall that sometimes leads to poor design and testing issues. While static members can be convenient, they can introduce global state, making the code harder to manage and test.
Why is this an Issue?
Static members limit flexibility and scalability. They can lead to dependencies between classes that make unit testing particularly challenging. If your methods rely heavily on static state, isolating them for testing becomes complicated.
How to Avoid It
- Favor Instance Members: Use instance members as much as possible to promote encapsulation and reduce dependencies.
public class User {
private String name;
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
- Use Singleton Carefully: If you need global access but still want to maintain some integrity, consider using the Singleton pattern, ensuring that it is thread-safe.
public class Singleton {
private static Singleton instance;
private Singleton() {} // Private constructor
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
- Leverage Dependency Injection: With frameworks like Spring, dependency injection can manage object creation and lifecycle, promoting good design patterns.
3. Ignoring Exception Handling
Many developers underestimate the importance of proper exception handling. Java encourages this through checked exceptions, yet it is common to see overly simplistic exception handling or even entirely neglected areas of code.
Why It Matters
Ignoring exceptions can lead to unpredictable application behavior, data corruption, and security vulnerabilities. Poorly handled exceptions prevent the system from responding gracefully in case of an error, which could lead to a negative user experience.
How to Avoid It
- Use Specific Exceptions: Avoid generic exception handling. Catch specific exceptions to manage failure more effectively.
try {
String data = getDataFromApi();
} catch (IOException e) {
System.err.println("Network error: " + e.getMessage());
} catch (ParseException e) {
System.err.println("Error parsing data: " + e.getMessage());
}
- Logging Exceptions: Always log exceptions for a deeper insight into issues that occur during runtime.
import java.util.logging.Logger;
public class Example {
private static final Logger LOGGER = Logger.getLogger(Example.class.getName());
public void handleData() {
try {
// Code that might throw exceptions
} catch (Exception e) {
LOGGER.severe("An error occurred: " + e.getMessage());
}
}
}
- Clean Up Resources: Use try-with-resources to automatically close resources like streams or connections, thus saving you from resource leaks.
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
To Wrap Things Up
Avoiding common pitfalls in Java can significantly enhance both the reliability and maintainability of your applications. Implementing robust error handling, initialization practices, and thoughtful use of static members will elevate your code quality.
For more insights into best coding practices, check out this Java Best Practices Guide and explore the Java Documentation for further learning.
Make sure to take these strategies into account on your next project, and watch your productivity and code quality soar! Happy coding!