Top 5 Tips to Speed Up Your Selenium Test Cases
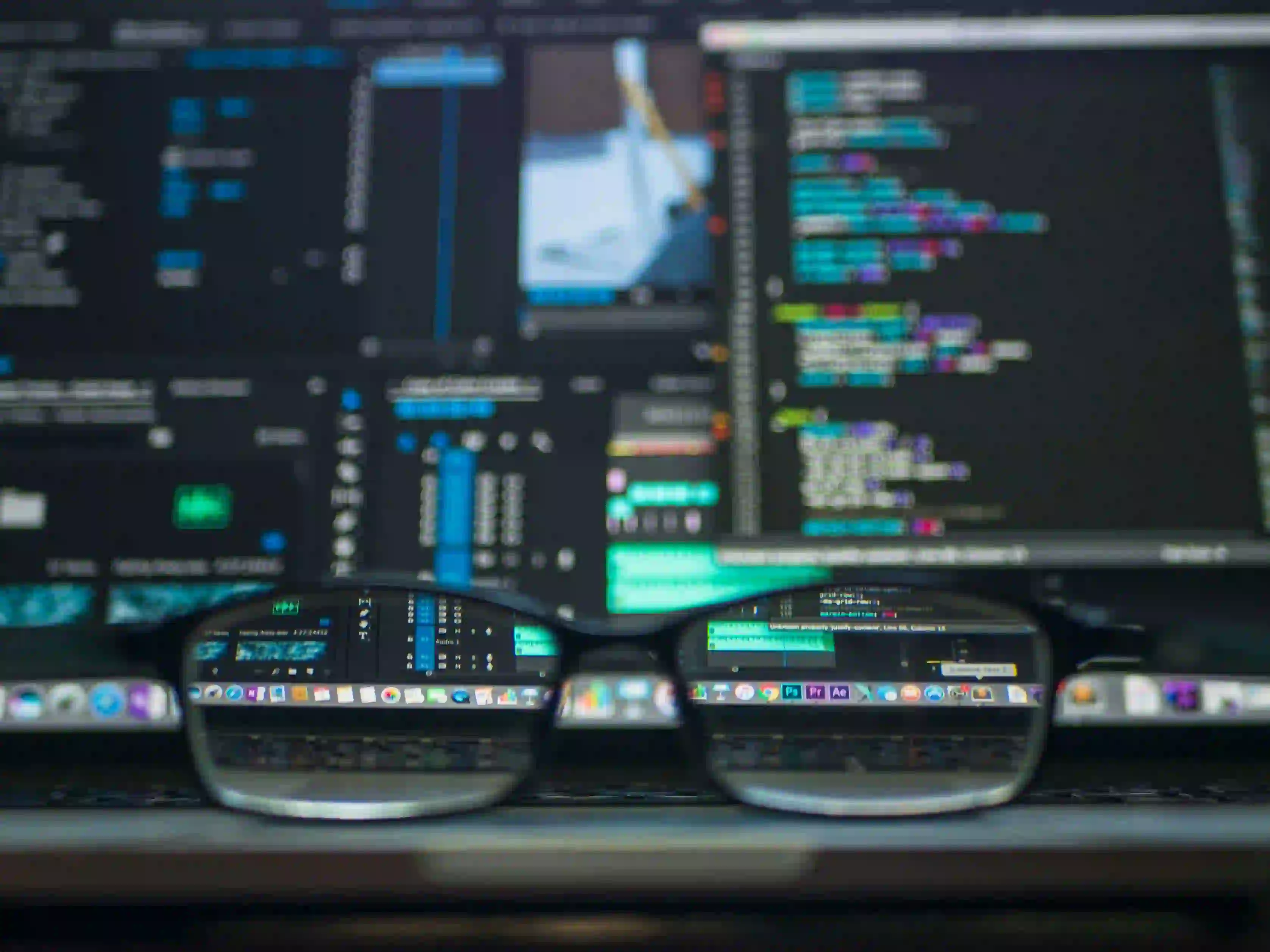
Top 5 Tips to Speed Up Your Selenium Test Cases
Selenium is a widely used open-source framework for automating web applications for testing purposes. While it’s powerful, one of the consistent challenges developers and testers face is the execution speed of Selenium test cases. Slower tests can lead to increased feedback times, which can hinder the agile development process.
In this blog post, we will discuss five key tips to speed up your Selenium test cases effectively. With these strategies, you will not only improve the efficiency of your tests but also enhance the overall developer experience.
1. Optimize WebDriver Usage
Commentary:
Using WebDriver optimally can significantly reduce test execution time. This involves strategically opening and closing browser instances and reusing WebDriver sessions whenever possible.
Code Snippet:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class WebDriverManager {
private static WebDriver driver;
private WebDriverManager() { }
public static WebDriver getDriver() {
if (driver == null) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
driver = new ChromeDriver();
}
return driver;
}
public static void closeDriver() {
if (driver != null) {
driver.quit();
driver = null;
}
}
}
Why:
By using the singleton pattern in the above code, we ensure that only one instance of WebDriver is created. This reduces the overhead of starting and stopping the browser multiple times, leading to faster execution of test cases.
2. Use Implicit and Explicit Waits Judiciously
Commentary:
Wait strategies in Selenium dictate how the WebDriver waits for elements to become available before proceeding. Overusing them can slow down tests, while underusing them can lead to test failures.
Code Snippet:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class WaitExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("http://example.com");
WebDriverWait wait = new WebDriverWait(driver, 20);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("myElement")));
// Perform actions on the element
element.click();
driver.quit();
}
}
Why:
In this example, we combine implicit and explicit waits to have a well-rounded approach. Implicit waits set a default waiting time for the entire session, while explicit waits are tailored to specific instances. Adjusting these strategically will reduce execution time while ensuring test reliability.
3. Parallel Testing with TestNG
Commentary:
Running tests in parallel can dramatically reduce the overall execution time. Tools like TestNG make it easy to configure parallel test execution for Selenium tests.
Code Snippet:
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="Parallel Tests" parallel="tests" thread-count="5">
<test name="Test1">
<classes>
<class name="testScripts.Test1"/>
</classes>
</test>
<test name="Test2">
<classes>
<class name="testScripts.Test2"/>
</classes>
</test>
</suite>
Why:
The above XML configuration allows for multiple tests to run simultaneously on five different threads. This essentially means while one test is loading or executing, another can do the same, resulting in a considerable decrease in total test duration. For more on TestNG, check out the official TestNG Documentation.
4. Reduce the Number of Browser Interactions
Commentary:
Every interaction with the browser (clicks, navigations, etc.) consumes time. Reducing unnecessary interactions can significantly shorten the run-time of your tests.
Code Snippet:
public void performActions() {
// Instead of multiple findElement calls
WebElement button = driver.findElement(By.id("buttonId"));
// Perform all actions in one go
button.click();
// Use JavaScriptExecutor if applicable
((JavascriptExecutor) driver).executeScript("arguments[0].click();", button);
}
Why:
In the above code snippet, we minimize the number of times we locate elements. Further, using JavaScript for actions can eliminate some of the wait time, especially for complex operations or elements that behave inconsistently.
5. Headless Browser Execution
Commentary:
Running tests in a headless browser (like Chrome or Firefox) can significantly speed up execution since the GUI does not need to be rendered. This is particularly beneficial in continuous integration/continuous deployment (CI/CD) environments.
Code Snippet:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
public class HeadlessExample {
public static void main(String[] args) {
ChromeOptions options = new ChromeOptions();
options.addArguments("--headless"); // Enable headless mode
WebDriver driver = new ChromeDriver(options);
driver.get("http://example.com");
System.out.println("Title: " + driver.getTitle());
driver.quit();
}
}
Why:
The example above demonstrates setting up a headless Chrome browser. The absence of a GUI means faster load times and reduced memory usage. This is particularly useful when running a large suite of tests as part of an automated build process. For more details on configuring Selenium for headless operation, visit this Selenium documentation.
The Closing Argument
Speeding up your Selenium test cases is crucial for maintaining an efficient development workflow. By applying these five tips—optimizing WebDriver usage, effectively managing waits, utilizing parallel testing, minimizing browser interactions, and opting for headless execution—you can significantly enhance the performance of your testing suite.
Remember, the goal of your tests is not just to run them but to run them efficiently to get quality feedback in a timely manner. Embrace these best practices and watch your test execution speed soar. Happy testing!
By following these strategies and continuously refining your testing approach, you will find that your Selenium test cases become not only faster but also more reliable. If you found these tips useful, feel free to share your experiences or other tips you might have!