Mastering Spy Beans: Common Pitfalls in Spring
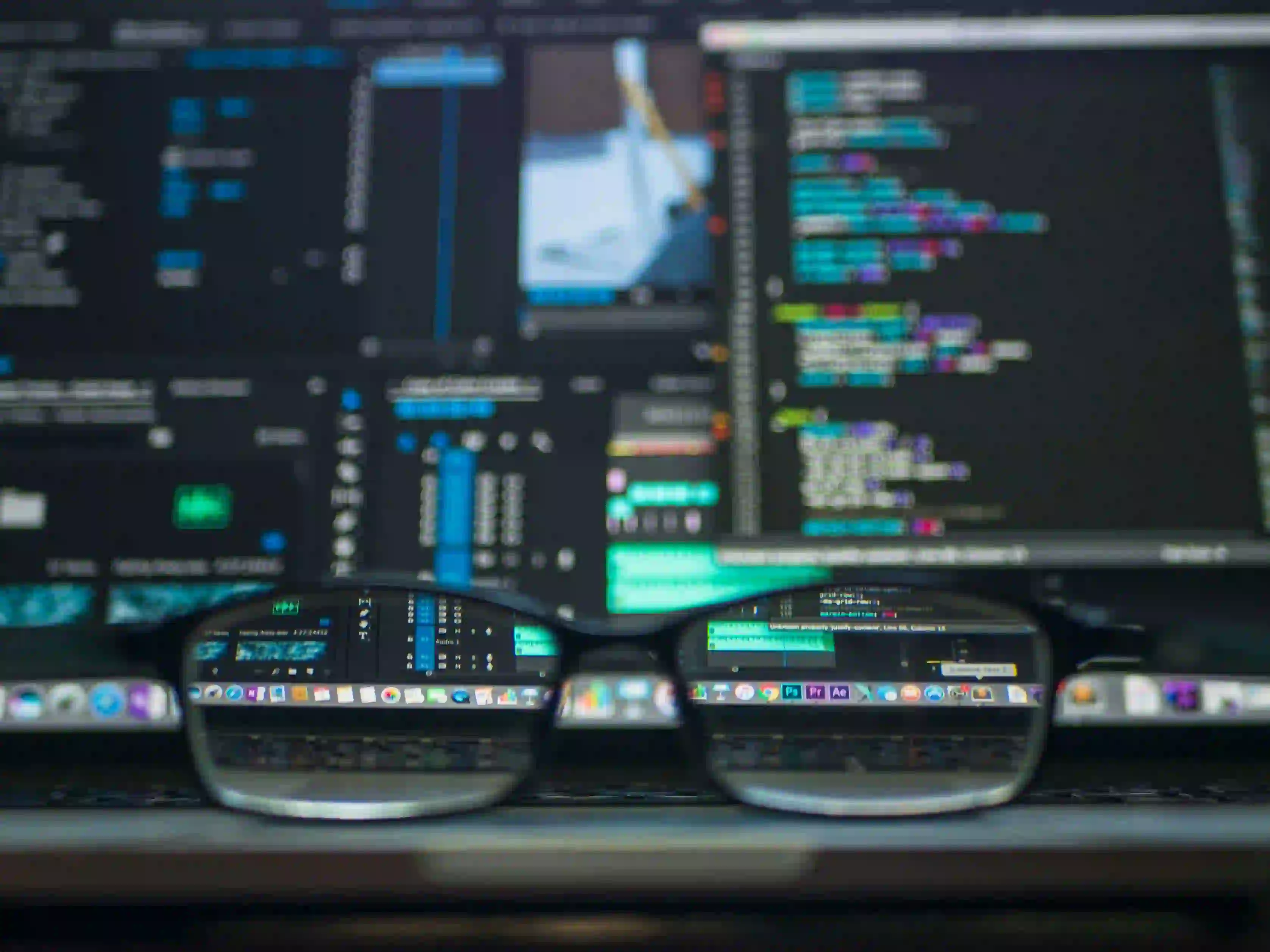
Mastering Spy Beans: Common Pitfalls in Spring
Spring Framework is an expansive and powerful ecosystem for developing Java applications. One of its more advanced features is the Spy Bean mechanism, which enables developers to monitor and analyze beans, facilitating optimization and debugging.
However, diving into the world of Spy Beans can come with its challenges. In this blog post, we will explore common pitfalls when using Spy Beans in Spring, providing you with insights and strategies to avoid these traps and harness the full potential of this feature.
Understanding Spy Beans
Before we discuss the pitfalls, it's crucial to understand what Spy Beans are. Spy Beans are a type of Spring bean specifically created for monitoring and spying on existing beans in your Spring application context. They enable developers to gather metrics such as invocation counts, execution times, or error rates without modifying the original bean's code.
To create a Spy Bean, you typically use the @SpyBean
annotation from Spring's testing framework:
import org.springframework.boot.test.mock.mockito.SpyBean;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class MyServiceTest {
@SpyBean
private MyService myService;
// Test methods go here
}
In this example, MyService
is being spied upon. The Spy Bean will record interactions with myService
, allowing you to analyze how it is being used during tests.
Common Pitfalls When Using Spy Beans
1. Misunderstanding Proxy Behavior
One of the most common pitfalls developers encounter is misunderstanding how proxies work in Spring. When a bean is spied upon, Spring uses proxies. Consequently, if you try to call the spied method directly on the bean, you may unintentionally bypass the Spy functionality.
Why This Matters: Developers often expect that using the instance will invoke the spy's behavior, but it won't if they call a method directly on the bean.
Solution: Always access the method through the proxy. You can inject the spied bean like this:
@Autowired
private MyService myService;
@Test
public void givenSpyBean_whenMethodCalled_thenSpyingWorks() {
myService.someMethod(); // Correct way
// Use Mockito's verification to check interactions
}
2. Not Configuring the Test Context Properly
Another common mistake is not properly configuring the Spring Test context. It can lead to scenarios where the Spy Bean is not effective, or worse, doesn't exist altogether.
Why This Matters: If Spring can't correctly initialize the application context with your beans, you'll miss valuable spying capabilities.
Solution: Make sure your test class is annotated with @SpringBootTest
(or an appropriate configuration) and includes the necessary configuration classes or properties files.
@SpringBootTest(classes = {Application.class})
public class MyServiceTest {
// Test methods here
}
3. Overusing Spy Beans
While Spy Beans are an excellent tool for monitoring, overusing them can lead to unnecessary complexity and performance issues. Developers may find themselves creating Spy Beans for nearly every component, which can clutter the tests and degrade performance.
Why This Matters: Each Spy Bean adds overhead. They should be used judiciously to maintain performance and test clarity.
Solution: Use Spy Beans when you need to understand critical interactions in the application. It's best to limit their usage to services where you specifically require insight.
4. Forgetting to Reset Spy Beans Between Tests
Spy Beans retain state between test methods if not reset. If a test modifies the spy's state, it can inadvertently affect subsequent tests, leading to flaky tests that may pass/fail unpredictably.
Why This Matters: Flaky tests hinder the development process and reduce confidence in the codebase.
Solution: Utilize Mockito's reset
function to clear state after each test.
@BeforeEach
public void setUp() {
MockitoAnnotations.openMocks(this);
}
@Test
public void testMethod1() {
// Test logic here
// Reset spy beans
reset(myService);
}
@Test
public void testMethod2() {
// Further tests here
}
5. Ignoring Exception Handling in Spy Beans
Spy Beans do not automatically handle exceptions thrown by the proxied bean. If a spied-upon method throws an exception, it may adversely affect your test logic or outcomes.
Why This Matters: Unhandled exceptions can lead to incomplete test coverage and missed interactions.
Solution: Always wrap your Spy Bean interactions in a try-catch block to manage exceptions gracefully.
@Test
public void givenSpyBean_whenThrowsException_thenHandled() {
try {
myService.methodThatThrows();
} catch (ExpectedException e) {
// Handle exception
}
verify(myService).methodThatThrows();
}
6. Failing to Verify Interactions
The entire point of Spy Beans is to monitor interactions. Forgetting to verify these interactions renders the Spy Bean ineffective. Developers need to explicitly check how many times a method was called or whether it was called with specific parameters.
Why This Matters: Without verification, you don't validate the efficacy of your application logic.
Solution: Utilize Mockito's verification methods to assert that the expected interactions occurred.
@Test
public void testMethodCallCount() {
myService.someMethod();
verify(myService, times(1)).someMethod();
}
Best Practices for Using Spy Beans
-
Limit Usage: Only use Spy Beans where necessary for monitoring critical behaviors.
-
Reset State: Always reset or reinitialize Spy Beans before each test to maintain isolation.
-
Utilize Proxies: Access methods through the spy proxy rather than directly on the bean instance.
-
Verify Interactions: Regularly verify interactions to ensure your methods are invoked as expected.
-
Keep Exception Handling: Always handle exceptions wisely around spied scenarios.
Closing the Chapter
Mastering Spy Beans in Spring can significantly enhance your application's observability, but they require careful consideration to avoid common pitfalls. By understanding how Spy Beans work, acknowledging the implications of proxy behavior, and strictly verifying interactions, you can leverage this powerful tool without running into the traps that so many developers encounter.
By adhering to the best practices outlined in this blog post, you will be well on your way to using Spring's Spy Beans effectively and efficiently. Embrace this feature with the confidence that comes from understanding its intricacies, and you'll find yourself better equipped to write robust, maintainable, and high-quality tests.
For further reading on Spring Boot testing strategies, you might find these resources helpful:
- Spring Boot Testing Documentation
- Mockito Documentation
- Effective Testing with Spring
Happy coding!