Overcoming Common Challenges with Adapter Design Patterns
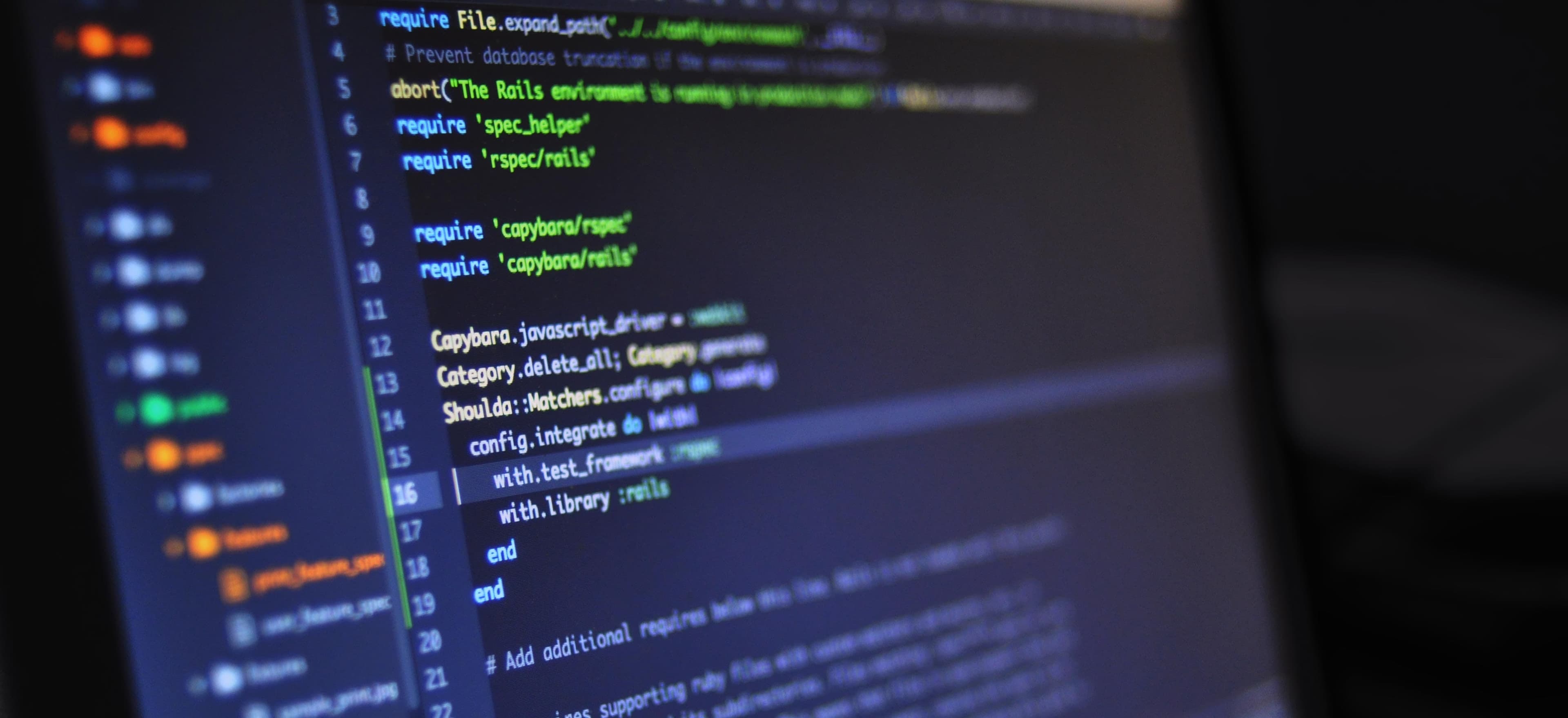
- Published on
Overcoming Common Challenges with Adapter Design Patterns in Java
The Adapter Design Pattern is fundamental in software development, especially when dealing with incompatible interfaces. This pattern acts as a bridge between two incompatible interfaces, allowing them to work together. In this blog post, we will dive deep into the Adapter Design Pattern in Java, explore common challenges, and provide practical solutions to overcome them.
What is the Adapter Design Pattern?
The Adapter Design Pattern falls under the category of structural design patterns. It allows two incompatible interfaces to communicate with each other. By using this pattern, we can create a 'wrapper' that translates requests from one interface to another.
A classical example can be drawn from everyday life. Think about a person (the client) who speaks English wanting to communicate with someone who speaks French. An interpreter acts as the adapter, translating English into French.
Key Benefits of Using the Adapter Pattern
- Encapsulation: The adapter hides the complexities of converting one interface to another.
- Single Responsibility Principle: The client code remains unaffected when an adapter is used.
- Reusability: Existing code can be reused without modification.
Challenges Faced with Adapter Design Patterns
While the Adapter Design Pattern is highly advantageous, developers often face specific challenges when implementing it:
- Multiple Adapters: Using multiple adapters for various components can increase complexity.
- Performance Overhead: Introducing abstraction can incur performance costs.
- Difficulty in Unit Testing: It may add complexity to unit tests if not managed correctly.
Let's explore each of these challenges and their solutions one by one.
Challenge 1: Managing Multiple Adapters
When implementing the Adapter Design Pattern, especially in large frameworks or applications, it's common to encounter multiple adapters. This can lead to confusion and increased maintenance overhead.
Solution: Aggregate Adapters
Instead of creating individual adapters scattered throughout the application, consider aggregating them into a single interface. This strategy provides a unified point of access.
// An existing interface
interface Target {
void request();
}
// Adapter A
class AdapterA implements Target {
private AdapteeA adapteeA;
public AdapterA(AdapteeA adapteeA) {
this.adapteeA = adapteeA;
}
public void request() {
adapteeA.specificRequest();
}
}
// Adapter B
class AdapterB implements Target {
private AdapteeB adapteeB;
public AdapterB(AdapteeB adapteeB) {
this.adapteeB = adapteeB;
}
public void request() {
adapteeB.anotherSpecificRequest();
}
}
// Unified Access Point
class UnifiedAdapter implements Target {
private List<Target> adapters = new ArrayList<>();
public UnifiedAdapter(List<Target> adapters) {
this.adapters = adapters;
}
public void request() {
for (Target adapter : adapters) {
adapter.request();
}
}
}
Commentary
The UnifiedAdapter
consolidates multiple adapter implementations. By using a list of Target
instances, it calls the request
method for every adapter in the collection. This simplifies the client code and allows it to interact with various components seamlessly through a single interface.
Challenge 2: Performance Overhead
Abstraction through adapters can sometimes introduce performance overhead, particularly in performance-sensitive applications.
Solution: Optimize the Adapter implementation
To alleviate performance issues, ensure that the adapter performs minimal operations. Here are some techniques:
- Caching: If the adapter is performing resource-intensive operations, consider caching the results.
- Lazy Initialization: Delay the creation of the adapted object until it is explicitly needed.
class CachingAdapter implements Target {
private Adaptee adaptee; // Lazy-loaded
private String cachedResult;
public void request() {
if (cachedResult == null) {
cachedResult = adaptee.specificRequest(); // Call only on first request
}
System.out.println(cachedResult);
}
}
Commentary
In the CachingAdapter
, the costly operation of fetching data from Adaptee
is only executed when the request is made for the first time. Subsequent requests utilize the cached result, effectively reducing performance overhead.
Challenge 3: Unit Testing Complexity
Adapters can complicate unit testing, as they may introduce additional dependencies that need to be mocked.
Solution: Use Dependency Injection
Dependency Injection can alleviate unit testing challenges by giving you control over the dependencies of an adapter, making it easier to test.
class Service {
private final Target adapter;
public Service(Target adapter) {
this.adapter = adapter;
}
public void serve() {
adapter.request();
}
}
// Enhanced Testing
class ServiceTest {
@Test
void testService() {
Target mockAdapter = mock(Target.class);
Service service = new Service(mockAdapter);
service.serve();
verify(mockAdapter).request(); // Verify the request was made
}
}
Commentary
In the Service
class, dependency injection allows us to pass any adapter we wish during testing. In the test case, we use a mocking framework to create a mock adapter, ensuring that we can verify the interaction without relying on the actual implementation.
A Final Look
The Adapter Design Pattern is invaluable for creating flexible and maintainable code in Java. By effectively addressing challenges like managing multiple adapters, performance overhead, and testing complexity, developers can maximize the advantages this pattern offers.
For more information on the Adapter Design Pattern and other design patterns, check out Refactoring Guru for deep dives and illustrative examples.
Incorporating the Adapter Design Pattern into your projects can pave the way for more robust architectures. With the right strategies, you can overcome common challenges and leverage the full potential of this versatile design pattern. Here’s to bridging gaps and ensuring seamless communication between incompatible interfaces!
Checkout our other articles