How to Quickly Identify Breaks After Rapid Code Deployments
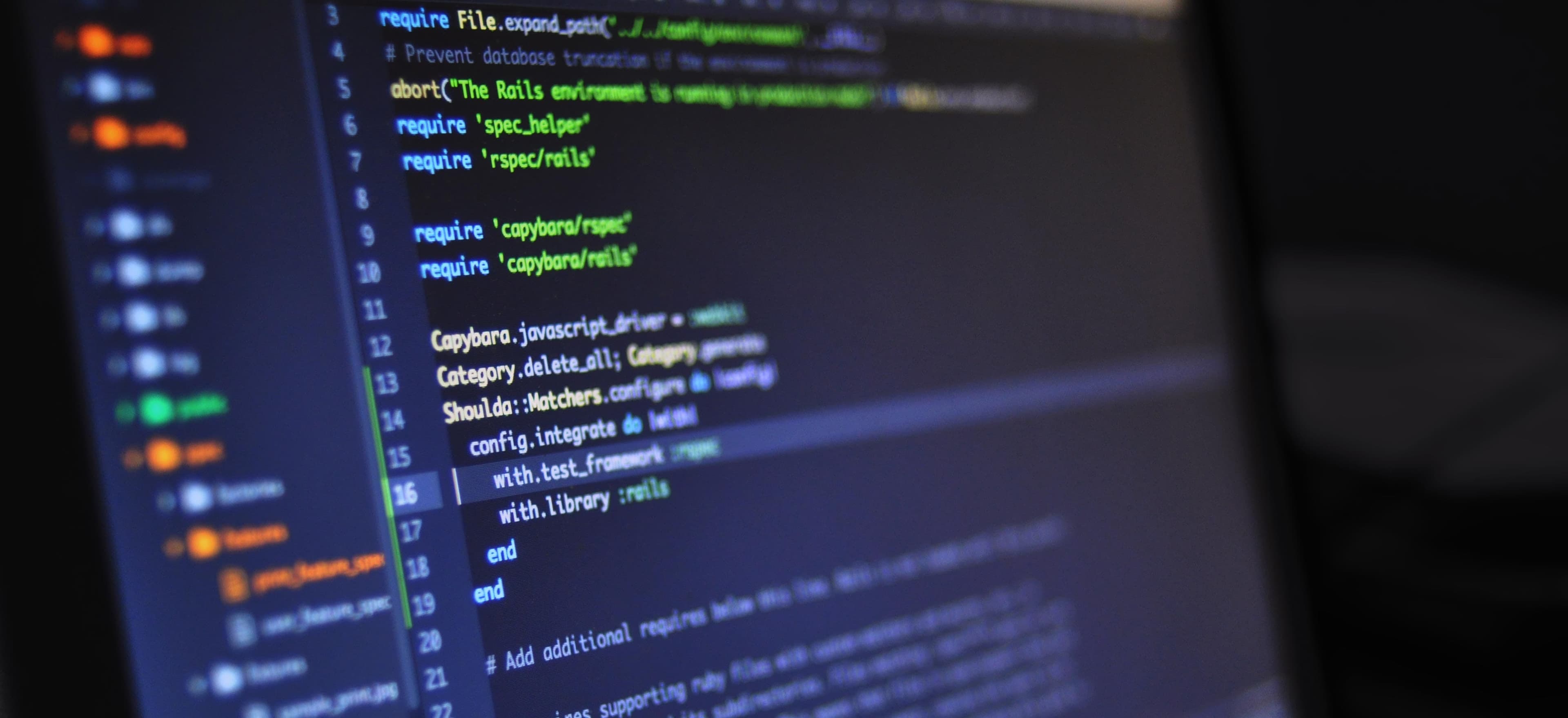
- Published on
How to Quickly Identify Breaks After Rapid Code Deployments
In the fast-paced world of software development, rapid code deployments are commonplace. These continuous integrations aim to deliver new features and fixes rapidly, but they also increase the risk of breaking changes. Identifying those issues quickly is crucial. In this blog post, we will explore strategies for efficiently identifying breaks following rapid code deployments. We will cover both automated and manual techniques to ensure that you can rapidly rectify any issues.
The Importance of Rapid Backtracking
The essence of rapid deployments is agility, but with this agility comes the heightened era of constant vigilance. When a code deployment introduces bugs or breaks functionality, immediate attention is imperative. Being able to identify these breaks reduces downtime, improves user experience, and empowers developers to maintain a steady rhythm of code improvement.
Why You Need a Monitoring Strategy
Understanding how your application behaves in production is key. Proper monitoring can automatically alert your team when something goes wrong, allowing you to address issues before they escalate. A solid monitoring strategy should encompass:
- Application Performance Monitoring (APM): Tools like New Relic, Datadog, or Dynatrace provide insights into application health.
- Error Tracking: Services such as Sentry or Rollbar help track and manage exceptions.
- Real User Monitoring (RUM): Tools that capture real-time user interactions and performance issues can provide valuable insights.
Establishing a Baseline
Before deploying code changes, it is essential to establish a baseline performance metric of your application. This can be done through:
- Load Testing: Identify how your application performs under load.
- Performance Benchmarks: Run routine checks on speed, response time, and resource usage.
These baselines help developers quickly differentiate between acceptable performance and problematic behavior after a code change.
The Role of Automated Testing
Automated testing is a crucial component of any deployment strategy. It enables teams to catch bugs before they hit production. Below are some key facets of automated testing:
Unit Tests
Unit tests validate the smallest parts of an application. A robust suite of unit tests can reveal issues during development and ensure that code functionality remains intact.
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
Why Use Unit Tests? Unit tests are straightforward to write. They help isolate the functionality being tested and provide rapid feedback upon code changes.
Integration Tests
These tests check how different components of the application work together. They are crucial for identifying issues in interactions between modules that unit tests might miss.
import static org.mockito.Mockito.*;
import org.junit.Test;
public class UserServiceTest {
@Test
public void testUserCreation() {
UserRepository userRepository = mock(UserRepository.class);
UserService userService = new UserService(userRepository);
User user = new User("test@example.com");
userService.createUser(user);
verify(userRepository).save(user);
}
}
Why Use Integration Tests? Integration tests help ensure that multiple components work seamlessly together, which is particularly important as more code is added.
End-to-End Tests
End-to-end (E2E) tests simulate real user scenarios from start to finish. These tests can uncover issues that unit and integration tests might miss.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class LoginTest {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("http://yourapp.com/login");
WebElement usernameField = driver.findElement(By.name("username"));
WebElement passwordField = driver.findElement(By.name("password"));
WebElement loginButton = driver.findElement(By.name("login"));
usernameField.sendKeys("user");
passwordField.sendKeys("password");
loginButton.click();
// Add assertions here to confirm successful login
driver.quit();
}
}
Why Use E2E Tests? These tests demonstrate how the application performs in an environment that closely mirrors production, ensuring a more comprehensive check.
Leveraging Continuous Integration/Continuous Deployment (CI/CD) Pipelines
Implementing a CI/CD pipeline automates the testing and deployment process. When integrated with testing frameworks, it can catch issues early in the development cycle.
- Automated Testing and Building: Every push to the repository triggers a test suite, allowing immediate feedback.
- Automated Deployment: Successful tests can automatically deploy code, minimizing manual intervention.
Popular CI/CD tools include Jenkins, CircleCI, and GitHub Actions.
Example of a Simple CI/CD Configuration Using GitHub Actions
name: CI/CD Pipeline
on:
push:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
- name: Set up JDK
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Build with Maven
run: mvn clean install
Why Use CI/CD? The immediate integration of testing and deployment processes provides rapid feedback loops, allowing teams to catch issues faster.
Manual Verification Techniques
Sometimes, automated processes may identify issues but require further investigation. Manual verification techniques can be employed to facilitate this.
Log Analysis
Error logs can provide deep insights into what went wrong during a deployment. Regularly checking logs and using tools like ELK Stack (Elasticsearch, Logstash, Kibana) can aggregate and analyze log data effectively.
Peer Reviews and Pair Programming
Implementing pair programming or code reviews fosters collaboration and knowledge sharing. A fresh set of eyes on the code may reveal issues that the original author missed.
User Feedback
Finally, always consider getting feedback from your users. They can offer insights and opinions that quantitative testing may not reveal. The use of tools like Hotjar can facilitate this user feedback collection.
Lessons Learned
The rapid deployment of code necessitates an acute awareness of potential breakage in functionalities. By employing a diverse strategy that incorporates automated testing, CI/CD practices, and manual verification, teams can quickly and efficiently identify and address breaks in their applications.
Implementing the discussed practices not only ensures a smoother deployment cycle but also empowers developers to maintain agility in today’s fast-paced development environment.
With a proactive approach to monitoring and testing, you can build resiliency into your deployments, allowing your team to continue delivering value to end-users with confidence.
For further reading on enhancing your deployment strategy, check out Continuous Integration: Improving Software Quality and Best Practices for Continuous Deployment.
By following the methods discussed in this guide, you are well on your way to mastering the complexities of identifying breaks after rapid code deployments. Happy coding!