Navigating NullPointerExceptions in Java: A Developer's Guide
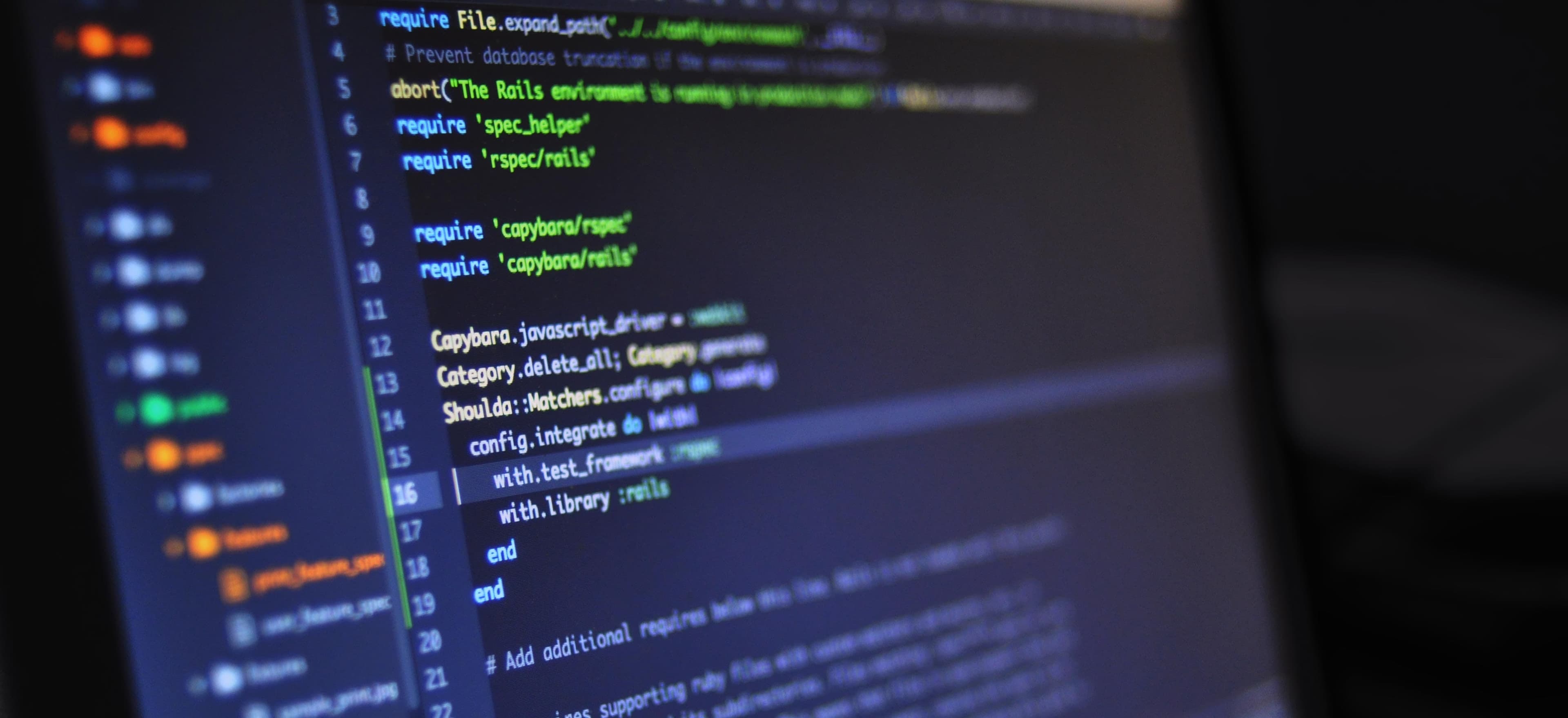
- Published on
Navigating NullPointerExceptions in Java: A Developer's Guide
Java developers often encounter NullPointerException (NPE) during application development. This runtime exception can lead to application crashes and a frustrating debugging process. Understanding its causes and how to prevent or handle these exceptions is crucial for writing robust Java code.
In this comprehensive guide, we will explore:
- What is NullPointerException?
- Common scenarios that lead to NPE.
- Best practices for avoiding NPE.
- Techniques for handling NPE when it arises.
- Example code snippets with explanations.
What is NullPointerException?
A NullPointerException is thrown when the Java Virtual Machine (JVM) attempts to access an object or call a method on a reference that points to null
. In essence, it indicates that your code is trying to access an object that doesn't exist.
Here's a simple illustration:
String str = null;
int length = str.length(); // This line throws NullPointerException
In this example, since str
is null
, attempting to call the method length()
results in a NullPointerException.
Quick Facts
- NPE is a runtime exception.
- It signifies that an application tried to access an object or a property that hasn't been initialized.
- Java does not allow primitive types (like
int
,char
, etc.) to be null; only reference types can be null.
Common Scenarios that Lead to NullPointerException
Understanding how NPEs arise can help you recognize potential pitfalls in your coding practices. Here are some common scenarios:
-
Missing Object Initialization: Forgetting to instantiate an object leads to NPE.
MyClass myObject; // Declared but not initialized myObject.doSomething(); // NullPointerException
-
Returning null from Methods: If a method returns
null
and its result is not handled properly, this could lead to NPE.public String getValue() { return null; // Intentionally returning null } String value = getValue(); System.out.println(value.length()); // NullPointerException
-
Using Collections: Accessing a collection element that hasn't been initialized.
List<String> list = null; String item = list.get(0); // NullPointerException
-
Chain Method Calls: If any object in a chain of method calls is
null
, an NPE will occur.String result = getPerson().getName(); // NullPointerException if getPerson() returns null
Best Practices for Avoiding NullPointerException
To bolster your defenses against NPE, consider implementing the following best practices:
1. Use Optional<T>
Starting from Java 8, you can utilize the Optional<T>
class to represent a value that may or may not be present. This approach effectively communicates the possibility of null
and allows the developer to handle such cases gracefully.
Here’s how you can implement it:
import java.util.Optional;
public Optional<String> getName() {
return Optional.ofNullable(name);
}
Optional<String> nameOpt = getName();
nameOpt.ifPresent(name -> System.out.println(name.length())); // Safe access
2. Use Explicit Null Checks
Before accessing objects, always check if they are null
:
if (myObject != null) {
myObject.doSomething();
} else {
System.out.println("Object is not initialized!");
}
3. Adopt Defensive Programming Practices
Think ahead about potential null references, and code defensively. For instance, consider default values or throw exceptions where appropriate.
public void process(String input) {
if (input == null) {
throw new IllegalArgumentException("Input cannot be null");
}
// continue processing
}
4. Avoid Chain Calls
Whenever possible, avoid chaining method calls unless you're absolutely sure each method will return a non-null object.
// Risky
String personName = getPerson().getName();
// Safer approach
Person person = getPerson();
String personName = (person != null) ? person.getName() : "Unknown";
5. Initialize Collections
Always initialize collections like lists and maps at the time of declaration. This practice helps prevent null-related issues.
List<String> list = new ArrayList<>();
list.add("Hello");
System.out.println(list.get(0)); // Safe
Techniques for Handling NullPointerException
As much as we aim to avoid them, NPEs can still occur. Here’s how to manage these exceptions more gracefully.
1. Try-Catch Block
You can catch NPEs and handle them gracefully. However, use this approach judiciously as overuse can hide underlying issues.
try {
String str = null;
System.out.println(str.length());
} catch (NullPointerException e) {
System.out.println("Caught a NullPointerException: " + e.getMessage());
}
2. Logging Frameworks
Utilize logging frameworks to log exceptions when they occur, providing insight into what went wrong. Frameworks like SLF4J or Log4j can be highly useful.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyClass {
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
public void riskyMethod() {
try {
// Code that may throw NPE
} catch (NullPointerException e) {
logger.error("Caught a NullPointerException", e);
}
}
}
3. Unit Testing
Incorporate testing to ensure your code handles null references properly. Frameworks like JUnit can help automate this process.
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class MyClassTest {
@Test
void testNullInput() {
MyClass myObj = new MyClass();
assertThrows(IllegalArgumentException.class, () -> {
myObj.process(null);
});
}
}
Lessons Learned
NullPointerExceptions are an unavoidable aspect of Java programming, but by applying defensive programming techniques, making use of Optional
, and properly handling potential NPEs, you can significantly mitigate their impact.
As a developer, it’s essential to remain vigilant and proactive rather than reactive when it comes to null references. Ultimately, robust handling of nullability leads to cleaner, more maintainable code.
For further insights into handling nulls in Java, refer to Java's Optional Documentation and Effective Java by Joshua Bloch.
By following the practices outlined in this guide, you'll be well-equipped to safeguard your code against NPEs and improve overall application stability. Stay alert, write safe code, and happy coding!