Unraveling JDK 12's String: Common Confusions Explained
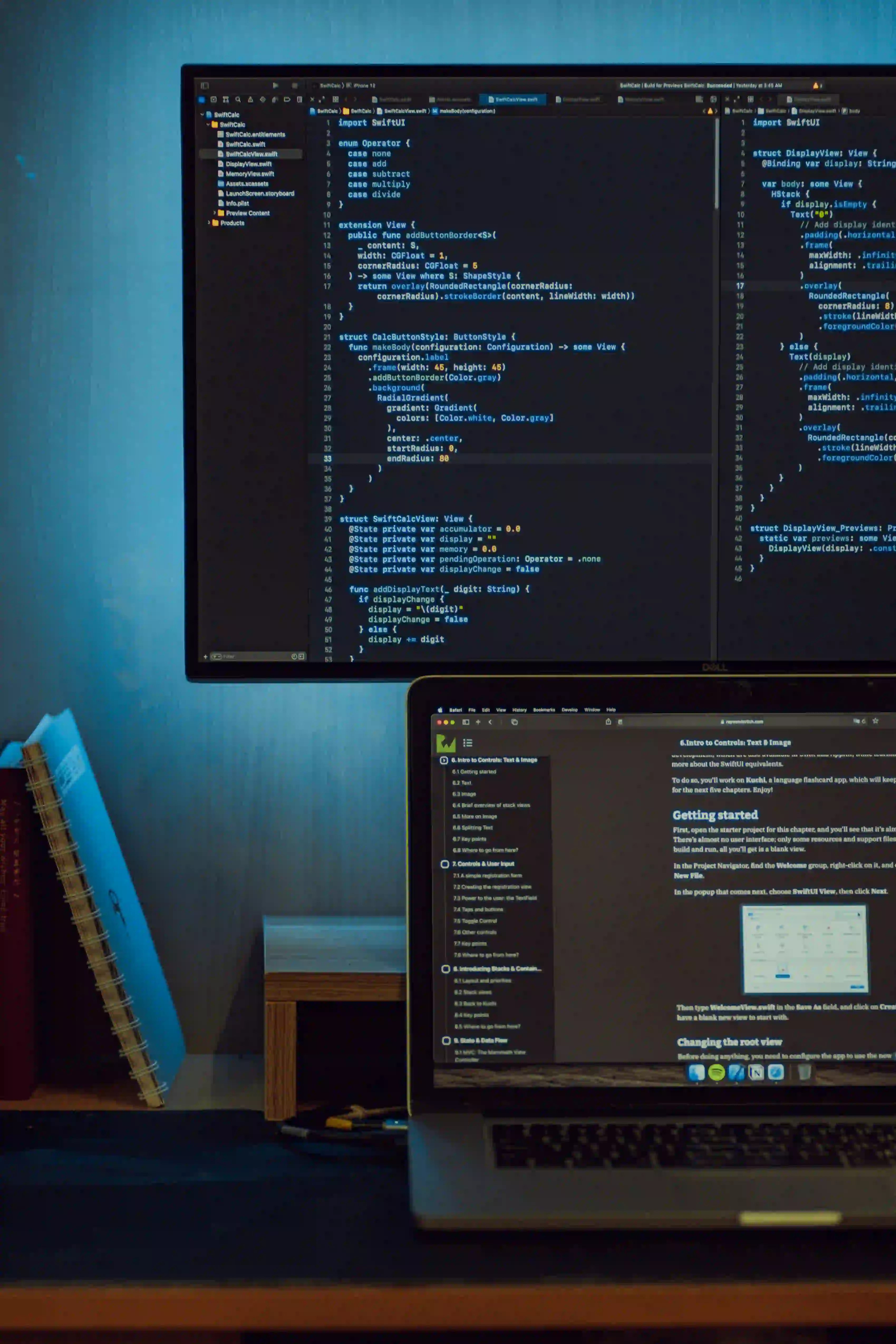
Unraveling JDK 12's String: Common Confusions Explained
Java Development Kit (JDK) 12 brought significant enhancements to the Java programming language, including improvements to the often-used String
class. While these changes are intended to simplify coding and enhance performance, they have also led to some confusion among developers. In this blog post, we will clarify these common misunderstandings about String in JDK 12, offering clear explanations along with practical examples.
Overview of String Changes in JDK 12
To set the stage, the String
class in Java has long been a fundamental aspect of the language. JDK 12 introduced a couple of notable enhancements:
- String Factory Methods: New factory methods for creating String objects.
- New
String
Methods: Enhanced methods for richer functionality.
String Factory Methods
Factory methods provide a neat way to instantiate objects. In JDK 12, several new factory methods were introduced to streamline String creation. Some commonly used methods include:
String.of(char[] array)
String.of(char[] array, int offset, int count)
String.copyValueOf(char[] array)
Example 1: Utilizing Factory Methods
public class StringFactoryExample {
public static void main(String[] args) {
char[] charArray = {'H', 'e', 'l', 'l', 'o'};
// Using String.of() to create a String directly from the character array
String hello = String.of(charArray);
System.out.println(hello); // Output: Hello
// Using String.copyValueOf() as an alternative
String helloCopy = String.copyValueOf(charArray);
System.out.println(helloCopy); // Output: Hello
}
}
Commentary: Here, String.of() simplifies the creation process for strings from character arrays. It enhances readability and makes code less verbose.
Common Confusions Regarding String Factory Methods
Confusion 1: What's the Difference Between String.of
and String.copyValueOf
?
Many developers wonder about the differences between these two methods. Both can create strings from a character array, but:
String.of()
can create a string directly from a character array.String.copyValueOf()
is tailored for copying a portion of the char array, returning the processed result in String format.
Enhanced String Methods
JDK 12 also introduced a couple of new methods that broaden the capabilities of the String
class. Notably, one of these methods is String.strip()
, which eliminates leading and trailing whitespace.
Example 2: Utilizing String.strip
public class StringStripExample {
public static void main(String[] args) {
String message = " Hello, World! ";
// Using the strip method to remove whitespace
String strippedMessage = message.strip();
System.out.println("'" + strippedMessage + "'"); // Output: 'Hello, World!'
}
}
Commentary: The strip()
method enhances string manipulation by ensuring that any unwanted whitespace is easily removed without affecting the actual content of the string.
Common Confusions About Enhanced String Methods
Confusion 2: String.strip()
vs. String.trim()
A prevalent question is whether String.strip()
is simply a replacement for String.trim()
. While both remove whitespace, their operational differences are key:
String.strip()
removes Unicode whitespace, whileString.trim()
only cleans ASCII whitespace.
To illustrate, consider the following example:
public class StringTrimExample {
public static void main(String[] args) {
String unicodeWhitespaces = "\u2003Hello, World!\u2003"; // Enclosed with Unicode whitespace
System.out.println("Using trim: '" + unicodeWhitespaces.trim() + "'"); // May not remove all
System.out.println("Using strip: '" + unicodeWhitespaces.strip() + "'"); // Removes all
}
}
Commentary: The difference in how these methods function underlines the importance of choosing the correct method based on your needs.
Performance Enhancements in JDK 12
Another important aspect of the JDK 12 release is the enhancement in performance regarding immutable strings. While these changes are not often discussed, they can have a notable impact on the efficiency of string operations.
Understanding Immutability
Java's String
class is immutable—once created, a string cannot be changed. This characteristic ensures that string data is consistent and thread-safe. However, when performing numerous string operations, such as concatenation, new strings are created internally, leading to potential inefficiencies.
Example 3: String Concatenation Performance
public class StringPerformance {
public static void main(String[] args) {
String result = "";
for (int i = 0; i < 1000; i++) {
result += "Hello"; // This creates a new string on each iteration
}
System.out.println("Result length: " + result.length());
}
}
Commentary: Relying on +=
can lead to excessive memory use due to the immutable nature of strings. Utilizing StringBuilder
is often suggested for concatenation performance:
public class EfficientStringConcatenation {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("Hello"); // More efficient
}
String result = sb.toString();
System.out.println("Result length: " + result.length());
}
}
Conclusion: Using StringBuilder
provides performance benefits by minimizing string object creation during concatenation.
A Final Look
In summary, JDK 12's updates to the String
class include factory methods and new string manipulation methods such as strip()
. These changes aim to improve usability and performance but can lead to confusions, particularly regarding the functionalities of similar methods. Being aware of how each works can vastly improve both your coding speed and efficiency.
In your journey of mastering the Java programming language, W3Schools can be a helpful resource to understand basic Java syntax better. For further information on Java’s string handling, Oracle’s official documentation is invaluable. Explore effective string usage and make the most of your Java applications!
Remember, coding is about clarity and precision. Understanding the tools at your disposal allows you to write cleaner, more efficient code—why wouldn't you take advantage of that? Happy coding!