Mastering Load Tests: The Power of JUnit's Repeat Annotation
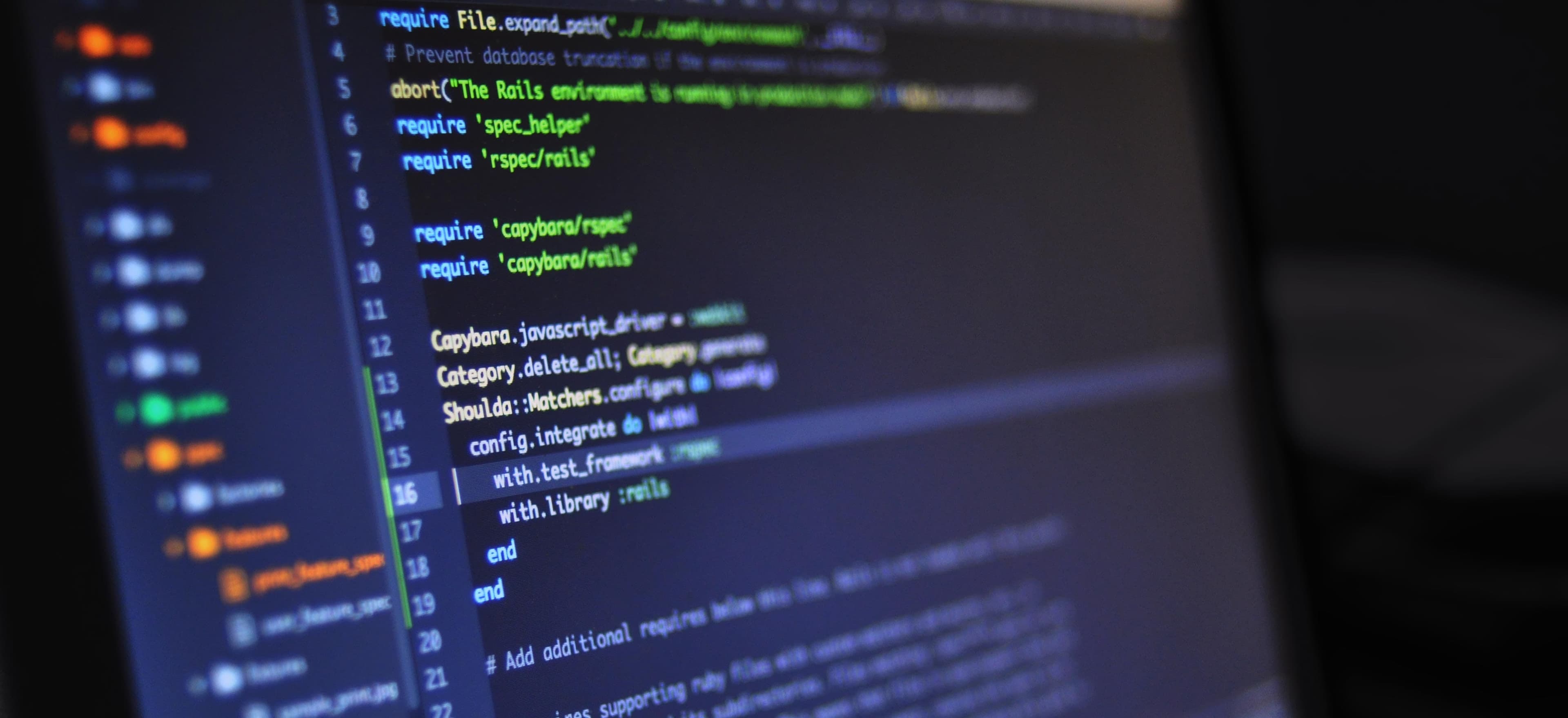
- Published on
Mastering Load Tests: The Power of JUnit's Repeat Annotation
Software performance is a non-negotiable requirement in today's fast-paced tech environment. As application complexity grows, so does the need for rigorous load testing. In conjunction with standard unit tests, load tests allow developers to simulate the expected usage patterns and performance under different conditions. One tool that is exceptionally helpful in this context, particularly for Java developers, is JUnit's @Repeat
annotation. In this post, we will explore how to effectively leverage this annotation for efficient load testing.
Understanding Load Testing
Load testing is designed to evaluate how a system behaves under a specific load. This load can involve multiple users, transaction volumes, or data sizes. The primary objectives of load testing are to identify performance bottlenecks, ensure stability, and validate scalability.
-
Performance Bottlenecks: These refer to the parts of the program that reduce throughput, leading to slow response times or application crashes.
-
Stability: The application must consistently meet performance criteria over time.
-
Scalability: The application should function seamlessly as user demand increases.
For a deeper dive into load testing concepts, check out the Load Testing Documentation.
JUnit: A Testing Framework for Java
JUnit is a popular testing framework for Java, used widely in the industry. It provides an easy way to create and run tests in Java applications. With its built-in assertions and annotations, JUnit enhances test structure and readability.
What is the @Repeat Annotation?
JUnit has introduced several annotations that simplify test execution. Among them, the @Repeat
annotation stands out. It allows you to run a single test method multiple times. This can be particularly useful in load testing scenarios, where you may want to execute the same operation under varying conditions. By automating this process, you can gather performance data more efficiently.
The standard way to include the @Repeat
annotation is as follows:
import org.junit.jupiter.api.RepeatedTest;
import org.junit.jupiter.api.Assertions;
class LoadTest {
@RepeatedTest(5)
void testLoad() {
// Simulate a task
Assertions.assertTrue(true);
}
}
In the above example, testLoad()
will execute five times.
Why Use @Repeat in Load Testing?
-
Simplicity: It eliminates the need for writing elaborate loops or using other utilities to repeat tests. A single annotation suffices.
-
Clarity: Tests remain easy to understand; the intent is clear.
-
Consistency: By running tests multiple times, you can observe performance metrics consistently, reducing the impact of transient issues.
Setting Up JUnit 5
To utilize the @Repeat
annotation, you need to set up JUnit 5 in your Java project. If you are using Maven, add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
For Gradle users, this is how you can declare it in your build.gradle
file:
testImplementation 'org.junit.jupiter:junit-jupiter:5.7.0'
Example: Implementing a Load Test with @Repeat
In this section, we will create a simple load test on an API endpoint or service method. We will test its performance under repeated access.
Simulating API Load Testing
Let’s assume you have a method that fetches user data from a database. The getUserData
method might look like this:
public class UserService {
public String getUserData(int userId) {
// Simulated database call
return "UserData for ID: " + userId;
}
}
Now, let’s write our load test to call this method multiple times using the @Repeat
annotation.
import org.junit.jupiter.api.RepeatedTest;
import static org.junit.jupiter.api.Assertions.assertNotNull;
class UserServiceTest {
private final UserService userService = new UserService();
@RepeatedTest(100)
void loadTestGetUserData() {
String userData = userService.getUserData(1);
assertNotNull(userData, "UserData should not be null");
System.out.println(userData);
}
}
Understanding the Code
In this example:
- We create an instance of
UserService
for our tests. - The test method
loadTestGetUserData
runs 100 times, simulating load on thegetUserData()
method. - We verify that the returned data is not null, indicating a successful response.
Adding System.out.println(userData);
enables you to see the actual output during the test runs, offering insights into what's happening under the load.
Gathering Performance Data
While the above implementation successfully simulates load, it does not capture actual performance metrics like execution time.
To gauge performance, you can modify the test as follows:
import org.junit.jupiter.api.RepeatedTest;
class UserServiceTest {
private final UserService userService = new UserService();
@RepeatedTest(10)
void loadTestPerformance() {
long startTime = System.currentTimeMillis();
userService.getUserData(1);
long endTime = System.currentTimeMillis();
long duration = endTime - startTime;
System.out.println("Execution Time: " + duration + " ms");
}
}
Why Track Performance?
- Bottleneck Identification: If execution time increases with repeated tests, it's a clear signal to investigate potential bottlenecks.
- Data Comparisons: Tracking performance across different versions helps refine and optimize your application.
Running JUnit Tests
You can run JUnit tests directly from your IDE (like IntelliJ, Eclipse) or via the command line. If using Maven, the command is:
mvn test
For Gradle:
gradle test
A Final Look
Using JUnit’s @Repeat
annotation simplifies the process of load testing in Java applications. It allows for efficient test execution while providing actionable insights into performance metrics. By integrating this into your testing suite, you can ensure your applications remain performant under various loads, a crucial aspect in today’s demanding environments.
For more information about performance testing and practices, you may want to explore the article on Load Testing Best Practices.
As you continue to refine your testing strategy, remember that effective load testing serves as a fundamental pillar in your quality assurance process. Happy testing!