Mastering Array Initialization: Common Pitfalls in Java
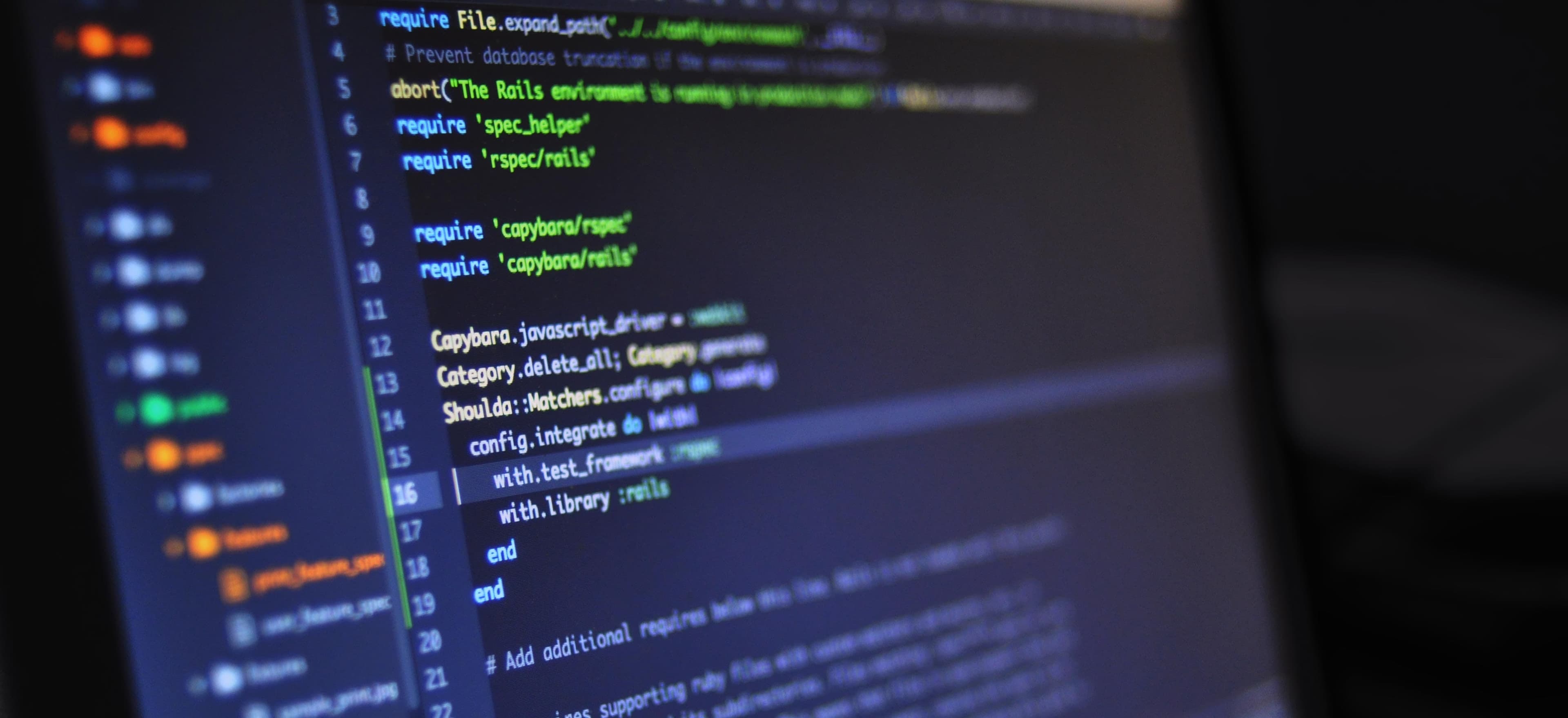
- Published on
Mastering Array Initialization: Common Pitfalls in Java
Java arrays are fundamental to storing fixed-size collections of elements of the same type. Mastering array initialization may seem trivial at first glance, yet subtle pitfalls can pose challenges even for experienced programmers. In this blog post, we will explore various aspects of array initialization in Java, identify common pitfalls, and provide tips to help you avoid them.
Table of Contents
- Understanding Java Arrays
- Basic Array Initialization
- Common Pitfalls in Array Initialization
- Pitfall 1: Wrong Array Declaration
- Pitfall 2: Array Index Out of Bounds
- Pitfall 3: Ignoring Default Values
- Multi-Dimensional Arrays
- Best Practices for Array Initialization
- Conclusion
1. Understanding Java Arrays
Arrays in Java are a collection of elements identified by index or key. Each element in an array is accessed using an integer index, with the first element at index 0. Arrays can hold any type of data: primitives, objects, and even other arrays!
Here’s a simple illustration:
int[] numbers = {1, 2, 3, 4, 5};
In this example, we declare and initialize an array of integers. Understanding this basic structure is crucial for tackling more complex scenarios.
2. Basic Array Initialization
Static Initialization
The simplest way to initialize an array is through static initialization, where you can declare and assign values in one line.
String[] fruits = {"Apple", "Banana", "Cherry"};
Dynamic Initialization
In cases where you need to assign values to the array at a later point, dynamic initialization comes into play:
int[] ages = new int[3]; // Declares an array of size 3
ages[0] = 25; // Assigns value to the first element
ages[1] = 30; // Second element
ages[2] = 22; // Third element
Dynamic initialization is particularly beneficial when the size of the array is determined at runtime.
3. Common Pitfalls in Array Initialization
Understanding the common pitfalls in array initialization will help you avoid common mistakes. Let’s dive into these pitfalls:
Pitfall 1: Wrong Array Declaration
One common mistake is failing to distinguish between the array's data type and an ordinary variable declaration. In Java, you need to specify brackets when declaring an array.
// Incorrect Declaration
int fruits; // This declares an int variable, not an array
// Correct Declaration
int[] nums; // This declares an array of integers
Why is it important? Misdeclaring an array type can lead to compilation errors, unexpected behavior, or runtime exceptions.
Pitfall 2: Array Index Out of Bounds
One of the tastiest fruits of learning Java arrays is the joy of accessing elements. However, attempting to access an index that is out of bounds will lead to a runtime error.
int[] scores = {10, 20, 30};
// Incorrect Access
int score = scores[3]; // Throws ArrayIndexOutOfBoundsException
Why should you be cautious? Always ensure your index is within the bounds of the array (0 to length-1). This often happens when dynamically initializing the array based on user input, or when looping through its elements.
Pitfall 3: Ignoring Default Values
In Java, when you create an array, the elements are automatically initialized to default values: 0 for numeric types, false for booleans, and null for reference types.
boolean[] flags = new boolean[5]; // Array of five booleans
// All flags[0] to flags[4] are initialized to false
Why is this crucial? Ignoring default values may lead to logical errors in your program. Always check the state of an array to avoid unexpected behaviors.
4. Multi-Dimensional Arrays
Java supports multi-dimensional arrays, allowing you to create a matrix of arrays. However, initializing them can be tricky.
Static Multi-Dimensional Initialization
int[][] matrix = {
{1, 2, 3},
{4, 5, 6}
};
Dynamic Multi-Dimensional Initialization
To dynamically create a two-dimensional array:
int[][] matrix = new int[2][3]; // This creates a 2x3 matrix
matrix[0][0] = 1; // First row, first column
matrix[1][2] = 6; // Second row, third column
Important Note: Be aware of irregular array lengths across the rows, which is allowed in Java:
int[][] irregular = {
{1, 2, 3},
{4, 5}
}; // This creates a 2D array with different lengths
5. Best Practices for Array Initialization
Here are some best practices to optimize your experience while working with arrays:
-
Use Length Property: Always use the
.length
property of an array to prevent out-of-bounds errors.for (int i = 0; i < scores.length; i++) { System.out.println(scores[i]); }
-
Immutable Lists: Consider using
List
(e.g.,ArrayList
) from the Java Collections Framework for variable size and easier manipulation.List<Integer> list = new ArrayList<>(Arrays.asList(1, 2, 3));
-
Initialization in a Loop: Dynamically initialize arrays within loops for changes in size or content.
int[] dynamicArray = new int[n]; // n determined at runtime for (int i = 0; i < n; i++) { dynamicArray[i] = i * 2; // Filling the array with even numbers }
-
Error Handling: Implement try-catch blocks around your array access code to gracefully handle potential errors.
try { System.out.println(scores[3]); } catch (ArrayIndexOutOfBoundsException e) { System.err.println("Index out of bounds! Please check your access."); }
6. Conclusion
Array initialization might appear simple, but the subtleties in its manipulation can lead to bugs that are hard to trace back. By recognizing common pitfalls such as wrong declarations, index out of bounds exceptions, and default values, you can effectively avoid them in your code.
Embracing best practices can further enhance your coding prowess and lead to cleaner, safer, and more understandable code. For deeper insights and further reading, check out resources like GeeksforGeeks or Java Documentation.
Successful coding is all about clarity and understanding - keep mastering those arrays, and enjoy the journey. Happy coding!
This blog post has walked you through the challenges and best practices of array initialization in Java. With practice and caution, mastering arrays will become second nature. Continue exploring Java and uncovering its deep functionalities!
Checkout our other articles