Choosing the Right Java Framework: A Developer's Dilemma
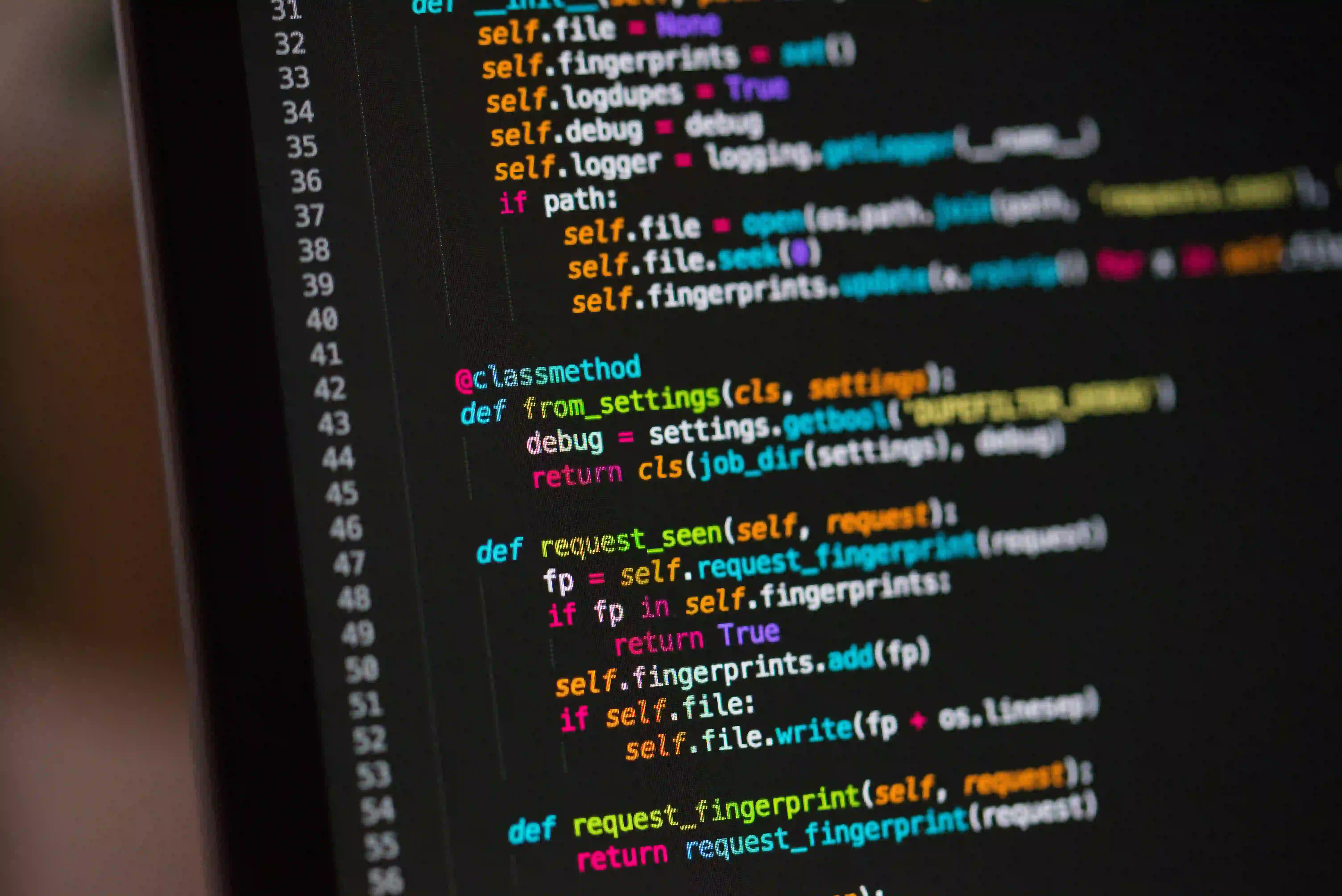
Choosing the Right Java Framework: A Developer's Dilemma
Java, one of the oldest and most robust programming languages, continues to embody versatility and reliability. As organizations grow and technology advances, developers face a crucial decision: which Java framework to use. This choice can significantly influence project scalability, maintainability, and performance. In this blog post, we’ll explore the essential factors to consider when choosing a Java framework, delve into popular Java frameworks, and provide insights on making an informed choice.
Understanding Java Frameworks
Before diving into specifics, it's essential to understand what a framework is. A Java framework is a set of libraries and tools that provide reusable components, making it easier to build applications. Frameworks simplify development processes by providing a structure and common functionalities, allowing developers to focus on business logic rather than underlying infrastructure.
Why Use a Framework?
- Efficiency: Frameworks provide pre-existing solutions for common tasks.
- Standardization: They enforce coding standards, making it easier for teams to collaborate.
- Community Support: Popular frameworks often have active communities, offering forums, documentation, and third-party tools.
Factors to Consider When Choosing a Java Framework
1. Project Requirements
Your project type plays a significant role in framework selection. For example, are you building a web application, mobile app, or microservices? Each project domain may require different frameworks tailored for specific functionalities.
2. Performance
Consider the performance benchmarks of each framework. Some frameworks are lightweight, optimizing resource consumption, while others are feature-rich but may introduce overhead. For performance-sensitive applications, frameworks like Spring or Micronaut might be ideal candidates due to their efficient resource management.
3. Community and Documentation
The health of a framework's community can significantly impact your development process. Well-documented frameworks with strong community support can be invaluable when troubleshooting issues or seeking guidance.
4. Learning Curve
Evaluate how quickly your team can adapt to a new framework. While powerful features can be tempting, a steep learning curve may hinder development speed. Frameworks like Spring Boot are known for their simplicity and quick onboarding, making them ideal for new projects.
5. Long-term Support
Frameworks that offer long-term support (LTS) tend to be more stable. They get regular updates and are less likely to become obsolete. Java EE (Jakarta EE) is a great example of a framework that emphasizes long-term viability.
Popular Java Frameworks
1. Spring Framework
Spring is arguably the most popular Java framework, known for its comprehensive ecosystem. It supports various application types, from monolithic applications to microservices, through Spring Boot.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
Why Spring? The annotation-based configuration makes it intuitive. The @SpringBootApplication
annotation is a convenience annotation that combines three necessary annotations: @Configuration
, @EnableAutoConfiguration
, and @ComponentScan
. This helps in creating a Spring application with minimal setup.
2. Hibernate
When it comes to Object-Relational Mapping (ORM), Hibernate continues to reign supreme. It simplifies database interactions through object-oriented paradigms.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// Getters and setters
}
Why Hibernate? With just a few lines of code, you can map a database table to a Java object. This abstraction allows developers to interact with the database using Java objects instead of tedious SQL queries, accelerating development.
3. JavaServer Faces (JSF)
JSF is a component-based framework for building user interfaces for Java web applications. It facilitates the construction of complex web applications with a rich set of UI components.
<h:form>
<h:inputText value="#{userBean.name}" />
<h:commandButton value="Submit" action="#{userBean.submit}" />
</h:form>
Why JSF? The integration of UI components and seamless event-driven programming makes it attractive for applications with a rich user interface. The component-based model helps in reusing components across different applications.
4. Micronaut
Micronaut is designed for building modern, modular applications, especially in cloud environments. It promises fast startup time and low memory consumption.
import io.micronaut.runtime.Micronaut;
public class Application {
public static void main(String[] args) {
Micronaut.run(Application.class);
}
}
Why Micronaut? Its dependency injection is compile-time instead of runtime, which enhances performance. This framework is a perfect fit for microservices and serverless applications.
5. Jakarta EE
Formerly known as Java EE, Jakarta EE provides a robust platform for large-scale enterprise applications. It supports a variety of APIs and features, ensuring extensive specification coverage.
Why Jakarta EE? It emphasizes standards, allowing for vendor independence. You can switch between implementations without major code changes, making it ideal for enterprise solutions.
Making Your Choice
With various frameworks available, how do you decide?
-
Analyze the Project Scope: Identify your specific needs such as performance, scalability, and security.
-
Prototype: Build small prototypes with the frameworks you are considering. This practical experience can highlight strengths and weaknesses.
-
Gather Team Feedback: Discuss with your development team. They will be the end-users of the framework, so their comfort level is crucial.
-
Consider Future Needs: Think about your project’s scalability and future requirements. Will the framework be able to adapt as your application grows?
A Final Look
Choosing a Java framework is often a developer's dilemma, but it doesn't have to be daunting. By evaluating your project's requirements, understanding the strengths of various frameworks like Spring, Hibernate, and Micronaut, and considering your team's expertise, you can make an informed choice.
Frameworks are not just tools; they shape your development experience and project trajectory. Take your time with this decision, as it can pay dividends in future development cycles. Remember, there is no "one-size-fits-all" solution, and the best choice is the one that aligns with your specific project goals and team strengths.
For more detailed information on Java frameworks and their comparisons, you can explore Baeldung's Java Frameworks Guide and Oracle’s Java EE Documentation.
Happy coding!