Common Load Testing Pitfalls in Apache JMeter
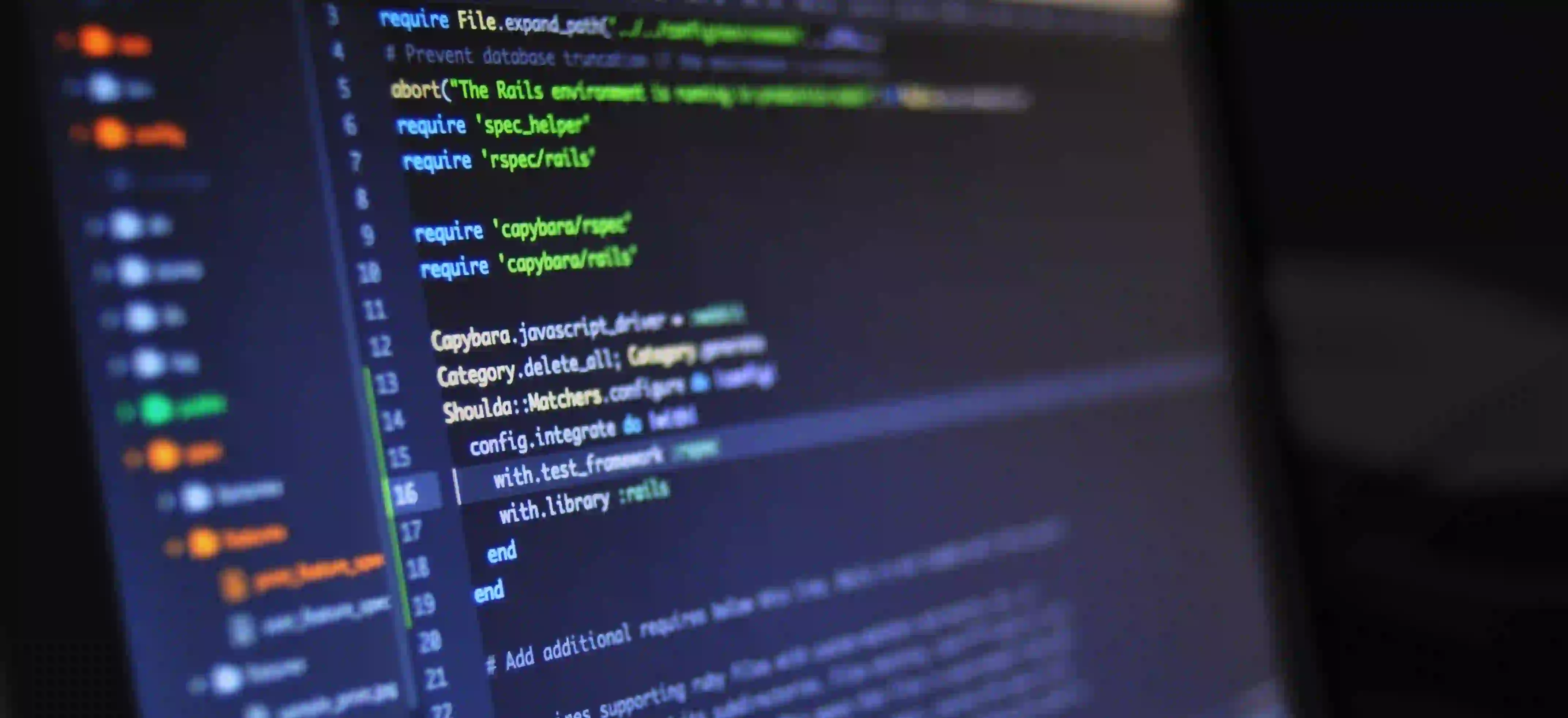
Common Load Testing Pitfalls in Apache JMeter
Load testing is a critical practice for ensuring that your application's performance meets user expectations under varying conditions. Apache JMeter is a popular open-source tool used for load testing, but like any tool, it comes with its pitfalls. Understanding these common mistakes can lead to more effective testing and better application performance. In this post, we will explore these pitfalls in detail while utilizing best practices with JMeter.
Why Load Testing Matters
Load testing helps verify how an application behaves under expected and peak loads. It ensures that your application can handle user activity effectively, maintaining performance and stability. The impact of not conducting sufficient load testing can be severe, ranging from poor user experience to complete application failure.
The Common Pitfalls
1. Ignoring Proper Test Planning
Why It Matters: Effective load testing starts long before executing your tests. Failing to define the scope, objectives, and test scenarios can lead to meaningless results.
How to Avoid It:
- Define clear objectives, such as the number of concurrent users and the metrics you want to gather (e.g., response time, throughput).
- Identify key scenarios that reflect real-world usage.
Here’s an example of a basic test plan structure in JMeter:
Test Plan
|- Thread Group
|- HTTP Request Defaults
|- HTTP Request (for each scenario)
|- Listeners (e.g., Summary Report, View Results Tree)
2. Overlooking Environment Consistency
Why It Matters: Conducting load tests in different environments can yield inconsistent results. If you're testing your application in a development environment while users are in production, you're likely assessing an inaccurate representation of your application's performance.
How to Avoid It:
- Always run load tests in an environment that closely mirrors your production setup.
- Ensure that database connections, APIs, and other dependencies are configured similarly to avoid skewed results.
3. Neglecting to Measure the Right Metrics
Why It Matters: Collecting metrics without focus can complicate the analysis processes, making it harder to identify performance bottlenecks.
How to Avoid It:
- Focus on critical metrics such as response time, error rate, and throughput.
- Utilize JMeter’s various listener options, like the View Results Tree, to gain insights into your endpoints.
Consider using JMeter's Summary Report for concise data that summarizes key metrics:
// Configure Summary Report Listener in JMeter
SummaryReport summaryReport = new SummaryReport();
summaryReport.setFilename("summaryReport.jtl");
4. Not Setting Realistic User Simulation
Why It Matters: Simulating users too aggressively or too conservatively can lead to misleading results. If the virtual users act in perfect synchrony, that won't reflect how real users behave.
How to Avoid It:
- Use timers, such as Constant Timer or Gaussian Random Timer, to mimic realistic user delays and interactions.
Here’s an example of how to add a Constant Timer in JMeter:
// Add a Constant Timer to simulate user think time
ConstantTimer timer = new ConstantTimer();
timer.setDelay(2000); // delays 2 seconds
5. Skipping User-Centric Testing
Why It Matters: Testing should be based on how users actually interact with your application, rather than theoretical or assumed access patterns.
How to Avoid It:
- Use a tool like Google Analytics to understand user behavior and tailor your test scenarios accordingly.
- Focus on critical functionalities from a user perspective, such as logging in, checking out, or navigating your site.
6. Ignoring Resource Limitations
Why It Matters: Underestimating the resource limitations of the testing machine will lead to incorrect bottleneck identification. The machine running JMeter should have sufficient CPU and RAM to handle the generated load.
How to Avoid It:
- Analyze the hardware on which JMeter runs and conduct tests using appropriate loads.
- Consider executing distributed testing with JMeter Master-Slave setup for better scalability.
// Starting a JMeter slave instance from the command line
jmeter-server -Djava.rmi.server.hostname=<slave-ip-address>
7. Failing to Parse and Analyze the Data
Why It Matters: Collecting data without proper analysis can result in missing crucial performance insights. It is essential to thread the collected data into actionable feedback.
How to Avoid It:
- After running your tests, regularly analyze your data using JMeter's reporting tools.
- Use JMeter Dashboard Reports for comprehensive visual representations of your load testing results.
8. Not Conducting Post-Test Cleanup
Why It Matters: After running tests, inconsistency in data can linger with scenarios not correctly handling sessions or states. This can lead to inaccurate data in future tests.
How to Avoid It:
- Ensure your testing environment resets after each test. This includes cleaning temporary sessions, cache, and data.
- Use appropriate tear-down mechanisms or scripts tailored for cleanup.
Closing Remarks
Apache JMeter is a powerful tool for conducting load testing, but avoiding common pitfalls is essential for effective and meaningful results. By implementing structured test plans, realistic user simulation, and thorough metric selection, you can improve your load testing strategy significantly.
Further Reading
For more in-depth information, check out:
- The Complete Guide to Load Testing with JMeter
- JMeter User Documentation
Final Thoughts
Load testing is an art and a science. With careful planning, the right strategies, and lessons from common pitfalls, your organization can ensure that your application endures success in a high-traffic world. Happy testing!