Eliminating Lombok Compilation Errors in Your Java Projects
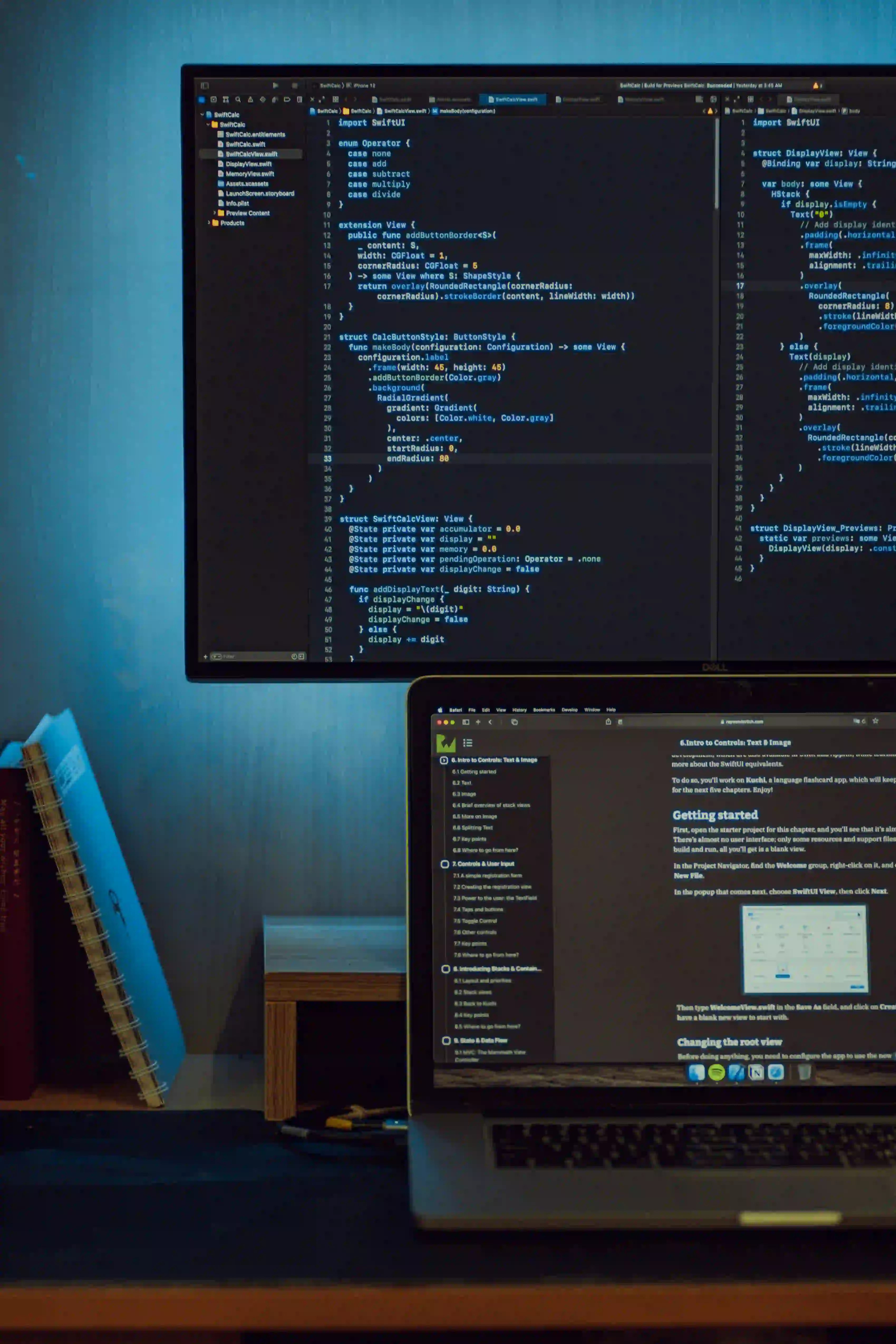
Eliminating Lombok Compilation Errors in Your Java Projects
Lombok is a popular Java library that enhances the coding experience by reducing boilerplate code. It generates necessary methods (like getters, setters, equals, hashCode, and toString) from annotations at compile time, allowing developers to write cleaner and more maintainable code. However, working with Lombok can sometimes lead to compilation errors or other issues, especially if you are new to the library or don’t have the proper setup. In this blog post, we'll explore common Lombok compilation errors, how to troubleshoot them, and best practices to avoid these pitfalls.
What is Lombok?
Lombok is a Java annotation processor that uses annotations to automatically generate boilerplate code, reducing the amount of code a developer must write by hand. Here are some common Lombok annotations you will encounter:
@Getter
: Automatically generates getter methods for the annotated field.@Setter
: Automatically generates setter methods for the annotated field.@ToString
: Generates atoString()
method that includes all fields.@EqualsAndHashCode
: Generatesequals()
andhashCode()
methods.@NoArgsConstructor
and@AllArgsConstructor
: Generates constructors with no and all parameters, respectively.
Setting Up Lombok in Your Project
Before diving into troubleshooting compilation errors, it’s essential to ensure Lombok is correctly set up in your project. Here’s how you can do that:
Maven Dependency
If you are using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.24</version> <!-- Check for the latest version -->
<scope>provided</scope>
</dependency>
Gradle Dependency
For Gradle users, add this to your build.gradle
:
dependencies {
compileOnly 'org.projectlombok:lombok:1.18.24' // Check for the latest version
annotationProcessor 'org.projectlombok:lombok:1.18.24'
}
IDE Configuration
In addition to adding the dependency, some IDEs require additional configuration.
-
IntelliJ IDEA: Ensure that you have the Lombok plugin installed. You can check this by going to Settings > Plugins.
-
Eclipse: Ensure you have installed the Lombok jar and configured your
eclipse.ini
file by adding-javaagent:path/to/lombok.jar
.
Common Lombok Compilation Errors
Let’s discuss common compilation errors you may encounter while using Lombok and how to resolve them.
1. Compilation Errors in IDE
Error Message: "Cannot resolve symbol 'Getter'".
Possible Causes:
- Lombok is not correctly set up in your IDE.
- The Lombok plugin is not installed or enabled.
Solution:
- Make sure the Lombok plugin is installed and enabled in your IDE.
- Reimport your project or restart the IDE to refresh the annotations.
2. Missing Annotation Processor
Error Message: “AnnotationProcessor not found”.
Possible Causes:
- Missing annotation processing configuration.
Solution:
- In Maven, ensure you have specified the
annotationProcessor
scope when adding Lombok. - In Gradle, include
annotationProcessor
in your dependencies.
Example Gradle dependency setup:
dependencies {
compileOnly 'org.projectlombok:lombok:1.18.24'
annotationProcessor 'org.projectlombok:lombok:1.18.24'
}
3. Version Compatibility Issues
Error Message: “Lombok version is not compatible with JDK version”.
Possible Causes:
- Using an outdated Lombok version that is not compatible with the Java version you are using.
Solution:
- Always check the Lombok documentation for version compatibility. Update your Lombok version to the most recent one that works with your JDK.
4. Gradle and Lombok Issues
If you encounter issues in Gradle that do not allow Lombok to compile:
Possible Causes:
- Improper gradle settings.
Solution: Use the built-in support for Java in Gradle to ensure that it knows how to handle Lombok.
apply plugin: 'java'
dependencies {
compileOnly 'org.projectlombok:lombok:1.18.24'
annotationProcessor 'org.projectlombok:lombok:1.18.24'
}
5. IDE Build Path Issues
Error Message: “Could not find the Lombok library”.
Possible Causes:
- Incorrect library setup in your project's build path.
Solution:
- Ensure that the Lombok jar is included in the project's build path. In IDEs like IntelliJ, check under Project Structure > Libraries.
Best Practices to Avoid Lombok Errors
While Lombok is a powerful tool, it is essential to follow best practices to avoid common pitfalls.
1. Keep Dependencies Updated
Regularly check for updates to Lombok and other dependencies you may be using. Keeping dependencies updated can often resolve compatibility problems.
2. Use Appropriate Annotations
Use only the annotations you need to avoid unnecessary complications. Overusing annotations or mixed annotations can lead to confusion and potential errors.
3. Write Unit Tests
Write unit tests for your classes that make use of Lombok. This ensures that the generated code works as intended and isn’t causing underlying issues in your application logic.
Example unit test using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class UserTest {
@Test
public void testUser() {
User user = new User();
user.setName("John Doe");
assertEquals("John Doe", user.getName());
}
}
4. Regularly Clean Project
Regularly cleaning your project (especially in IDEs) can help eliminate cached data that might be causing issues. This can be done in IDEs via Project menu > Clean.
5. Use IDE Assistance
Make use of IDE features that assist with annotation processing. For example, many IDEs provide options to enable/disable annotation processing which can resolve issues automatically.
Wrapping Up
Lombok is a fantastic library that can significantly reduce boilerplate code in your Java projects. However, like any tool, it comes with its own set of challenges. By understanding common Lombok compilation errors and following best practices, you can leverage the power of this library without getting bogged down by issues.
For further reading, consider visiting the Lombok Project Page for official documentation and troubleshooting tips.
Incorporating Lombok into your Java projects will streamline your coding process and enhance productivity. Happy coding!